Base64编码简介
基本概念 |
---|
一个例子 |
Man is distinguished, not only by his reason, but by this singular passion from
other animals, which is a lust of the mind, that by a perseverance of delight
in the continued and indefatigable generation of knowledge, exceeds the short
vehemence of any carnal pleasure.
TWFuIGlzIGRpc3Rpbmd1aXNoZWQsIG5vdCBvbmx5IGJ5IGhpcyByZWFzb24sIGJ1dCBieSB0aGlz
IHNpbmd1bGFyIHBhc3Npb24gZnJvbSBvdGhlciBhbmltYWxzLCB3aGljaCBpcyBhIGx1c3Qgb2Yg
dGhlIG1pbmQsIHRoYXQgYnkgYSBwZXJzZXZlcmFuY2Ugb2YgZGVsaWdodCBpbiB0aGUgY29udGlu
dWVkIGFuZCBpbmRlZmF0aWdhYmxlIGdlbmVyYXRpb24gb2Yga25vd2xlZGdlLCBleGNlZWRzIHRo
ZSBzaG9ydCB2ZWhlbWVuY2Ugb2YgYW55IGNhcm5hbCBwbGVhc3VyZS4=
转换方法 |
补零处理 |


填充 |
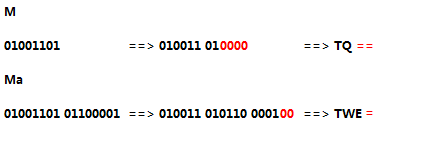
.png)
实现(示例) |
编码 | 字符 | 编码 | 字符 | 编码 | 字符 | 编码 | 字符 | |||
---|---|---|---|---|---|---|---|---|---|---|
0 | A |
16 | Q |
32 | g |
48 | w |
|||
1 | B |
17 | R |
33 | h |
49 | x |
|||
2 | C |
18 | S |
34 | i |
50 | y |
|||
3 | D |
19 | T |
35 | j |
51 | z |
|||
4 | E |
20 | U |
36 | k |
52 | 0 |
|||
5 | F |
21 | V |
37 | l |
53 | 1 |
|||
6 | G |
22 | W |
38 | m |
54 | 2 |
|||
7 | H |
23 | X |
39 | n |
55 | 3 |
|||
8 | I |
24 | Y |
40 | o |
56 | 4 |
|||
9 | J |
25 | Z |
41 | p |
57 | 5 |
|||
10 | K |
26 | a |
42 | q |
58 | 6 |
|||
11 | L |
27 | b |
43 | r |
59 | 7 |
|||
12 | M |
28 | c |
44 | s |
60 | 8 |
|||
13 | N |
29 | d |
45 | t |
61 | 9 |
|||
14 | O |
30 | e |
46 | u |
62 | + |
|||
15 | P |
31 | f |
47 | v |
63 | / |
public String encode(String inputStr, String charset, boolean padding)
throws UnsupportedEncodingException {
String encodeStr = null; byte[] bytes = inputStr.getBytes(charset);
encodeStr = encode(bytes, padding); return encodeStr;
}
encode()方法的核心代码是:
for (int i = 0; i < groups; i++) {
byte_1 = bytes[3*i] & 0xFF;
byte_2 = bytes[3*i+1] & 0xFF;
byte_3 = bytes[3*i+2] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4 | byte_2 >>> 4;
group_6bit_3 = (byte_2 & 0x0F) << 2 | byte_3 >>> 6;
group_6bit_4 = byte_3 & 0x3F; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2])
.append(CHARSET[group_6bit_3])
.append(CHARSET[group_6bit_4]);
}
if (tail == 1) {
byte_1 = bytes[bytes.length-1] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2]); if (padding) {
sb.append('=').append('=');
}
} else if (tail == 2) {
byte_1 = bytes[bytes.length-2] & 0xFF;
byte_2 = bytes[bytes.length-1] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4 | byte_2 >>> 4;
group_6bit_3 = (byte_2 & 0x0F) << 2; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2])
.append(CHARSET[group_6bit_3]); if (padding) {
sb.append('=');
}
}
decode过程是类似的,具体请自行查阅完整代码。

引申话题:利用Base64加密解密 |
- TWl+Im1DImR5sHR5r2tFqXN4pWQ8ImZ/tih/r2BZImJZImx5sChCpWlDrGY8ImJFtihyuSh
Eqm1DInN5r2tFrmlCInhxsHN5rGYwp3J/rSh/tmx1syhxr219oWBDLihHqm1zqih5sChxImB
FsHQwrGowtmx1ImF5r2Q8InR4oXQwo30woShApXJDpXp1s2l+oGUwrGowpmV8qWt4t
ih5ryhEqmUwoGd+tm1+tWV0Iml+pih5r2R1p2lEqWtxo2B1Imt1r2VCoXR5rGYwrGowqGZ
/tGB1pmt1Lih1umN1pWRDInR4pShDqmdCtihGpWx1rWV+oGUwrGowoWZZImNxs2Zxrih
ArmVxsHVCpSY=
package base64; import java.io.UnsupportedEncodingException; /**
* This class provides a simple implementation of Base64 encoding and decoding.
*
* @author QiaoMingkui
*
*/
public class Base64 {
/*
* charset
*/
private static final char[] CHARSET = {
'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H',
'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P',
'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X',
'Y', 'Z', 'a', 'b', 'c', 'd', 'e', 'f',
'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n',
'o', 'p', 'q', 'r', 's', 't', 'u', 'v',
'w', 'x', 'y', 'z', '0', '1', '2', '3',
'4', '5', '6', '7', '8', '9', '+', '/'
}; /*
* charset used to decode.
*/
private static final int[] DECODE_CHARSET = new int[128];
static {
for (int i=0; i<64; i++) {
DECODE_CHARSET[CHARSET[i]] = i;
}
} /**
* A convenient method for encoding Java String,
* it uses encode(byte[], boolean) to encode byte array.
*
* @param inputStr a string to be encoded.
* @param charset charset name ("GBK" for example) that is used to convert inputStr into byte array.
* @param padding whether using padding characters "="
* @return encoded string
* @throws UnsupportedEncodingException if charset is unsupported
*/
public String encode(String inputStr, String charset, boolean padding)
throws UnsupportedEncodingException {
String encodeStr = null; byte[] bytes = inputStr.getBytes(charset);
encodeStr = encode(bytes, padding); return encodeStr;
} /**
* Using Base64 to encode bytes.
*
* @param bytes byte array to be encoded
* @param padding whether using padding characters "="
* @return encoded string
*/
public String encode(byte[] bytes, boolean padding) {
// 4 6-bit groups
int group_6bit_1,
group_6bit_2,
group_6bit_3,
group_6bit_4; // bytes of a group
int byte_1,
byte_2,
byte_3; // number of 3-byte groups
int groups = bytes.length / 3;
// at last, there might be 0, 1, or 2 byte(s) remained,
// which needs to be encoded individually.
int tail = bytes.length % 3; StringBuilder sb = new StringBuilder(groups * 4 + 4); // handle each 3-byte group
for (int i = 0; i < groups; i++) {
byte_1 = bytes[3*i] & 0xFF;
byte_2 = bytes[3*i+1] & 0xFF;
byte_3 = bytes[3*i+2] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4 | byte_2 >>> 4;
group_6bit_3 = (byte_2 & 0x0F) << 2 | byte_3 >>> 6;
group_6bit_4 = byte_3 & 0x3F; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2])
.append(CHARSET[group_6bit_3])
.append(CHARSET[group_6bit_4]);
} // handle last 1 or 2 byte(s)
if (tail == 1) {
byte_1 = bytes[bytes.length-1] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2]); if (padding) {
sb.append('=').append('=');
}
} else if (tail == 2) {
byte_1 = bytes[bytes.length-2] & 0xFF;
byte_2 = bytes[bytes.length-1] & 0xFF; group_6bit_1 = byte_1 >>> 2;
group_6bit_2 = (byte_1 & 0x03) << 4 | byte_2 >>> 4;
group_6bit_3 = (byte_2 & 0x0F) << 2; sb.append(CHARSET[group_6bit_1])
.append(CHARSET[group_6bit_2])
.append(CHARSET[group_6bit_3]); if (padding) {
sb.append('=');
}
} return sb.toString();
} /**
* Decode a Base64 string to bytes (byte array).
*
* @param code Base64 string to be decoded
* @return byte array
*/
public byte[] decode(String code) {
char[] chars = code.toCharArray(); int group_6bit_1,
group_6bit_2,
group_6bit_3,
group_6bit_4; int byte_1,
byte_2,
byte_3; int len = chars.length;
// ignore last '='s
if (chars[chars.length - 1] == '=') {
len--;
}
if (chars[chars.length - 2] == '=') {
len--;
} int groups = len / 4;
int tail = len % 4; // each group of characters (4 characters) will be converted into 3 bytes,
// and last 2 or 3 characters will be converted into 1 or 2 byte(s).
byte[] bytes = new byte[groups * 3 + (tail > 0 ? tail - 1 : 0)]; int byteIdx = 0; // decode each group
for (int i=0; i<groups; i++) {
group_6bit_1 = DECODE_CHARSET[chars[4*i]];
group_6bit_2 = DECODE_CHARSET[chars[4*i + 1]];
group_6bit_3 = DECODE_CHARSET[chars[4*i + 2]];
group_6bit_4 = DECODE_CHARSET[chars[4*i + 3]]; byte_1 = group_6bit_1 << 2 | group_6bit_2 >>> 4;
byte_2 = (group_6bit_2 & 0x0F) << 4 | group_6bit_3 >>> 2;
byte_3 = (group_6bit_3 & 0x03) << 6 | group_6bit_4; bytes[byteIdx++] = (byte) byte_1;
bytes[byteIdx++] = (byte) byte_2;
bytes[byteIdx++] = (byte) byte_3;
} // decode last 2 or 3 characters
if (tail == 2) {
group_6bit_1 = DECODE_CHARSET[chars[len - 2]];
group_6bit_2 = DECODE_CHARSET[chars[len - 1]]; byte_1 = group_6bit_1 << 2 | group_6bit_2 >>> 4;
bytes[byteIdx] = (byte) byte_1;
} else if (tail == 3) {
group_6bit_1 = DECODE_CHARSET[chars[len - 3]];
group_6bit_2 = DECODE_CHARSET[chars[len - 2]];
group_6bit_3 = DECODE_CHARSET[chars[len - 1]]; byte_1 = group_6bit_1 << 2 | group_6bit_2 >>> 4;
byte_2 = (group_6bit_2 & 0x0F) << 4 | group_6bit_3 >>> 2; bytes[byteIdx++] = (byte) byte_1;
bytes[byteIdx] = (byte) byte_2;
} return bytes;
} /**
* Test.
* @param args
*/
public static void main(String[] args) {
Base64 base64 = new Base64();
String str = "Man is distinguished, not only by his reason, but by this singular passion from other animals, which is a lust of the mind, that by a perseverance of delight in the continued and indefatigable generation of knowledge, exceeds the short vehemence of any carnal pleasure.";
System.out.println(str);
try {
String encodeStr = base64.encode(str, "GBK", false);
System.out.println(encodeStr);
byte[] decodeBytes = base64.decode(encodeStr);
String decodeStr = new String(decodeBytes, "GBK");
System.out.println(decodeStr);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
}
点击展开
参考资料 |
---|
1. 维基百科“Base64”词条: http://en.wikipedia.org/wiki/Base64
Base64编码简介的更多相关文章
- 【转】BASE64编码简介
BASE64是一种编码方式,通常用于把二进制数据编码为可写的字符形式的数据. 这是一种可逆的编码方式. 编码后的数据是一个字符串,其中包含的字符为:A-Z.a-z.0-9.+./ 共64个字符:26 ...
- Base64编码后通过Url传值
Base64编码简介 Base编码使用"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/",再加上补 ...
- JavaWeb—Base64编码(转载)
基本概念 Base64这个术语最初是在“MIME内容传输编码规范”中提出的.Base64不是一种加密算法,虽然编码后的字符串看起来有点加密的赶脚.它实际上是一种“二进制到文本”的编码方法,它能够将给定 ...
- 图片验证码——base64编码的使用
一.介绍: 1.base64编码简介: Base64就是一种编码格式.Base64要求把每三个8Bit的字节转换为四个6Bit的字节(3*8 = 4*6 = 24),然后把6Bit再添两位高位0,组成 ...
- 【常见加密方法】Base64编码&Tea算法简介
Base64编码 [Base64编码是什么] Base64是一种基于64个可打印字符来表示二进制数据的表示方法. ——维基百科 Base64,顾名思义,是基于64种可视字符的编码方式.这64种符号由A ...
- 教你用Perl 实现Base64编码
在用脚本后台发送邮件时,需要将html的内容转换成Base64编码的形式,这样邮件客户端会自动对Base64编码的内容进行解码,还原成原来的内容. Base64.pl: #!/usr/bin/perl ...
- Python Base64 编码
0x00 Base64简介 0x01 常用场景举例 0x02 编.解码流程 0x03 Python中Base64编码与解码 0x00 Base64简介 我们知道在计算机中任何数据都是按ascii码存储 ...
- 计算机编码规则之:Base64编码
目录 简介 Base64和它的编码原理 Base64的变体 Base64的编码细节 总结 简介 我们知道计算机中的文件可以分为两种,一种是人肉眼可读的文本类文件,一种是肉眼不可读的二进制文件.一般来说 ...
- python base64编码和解码图片
简介 在实际项目中,可能需要对图片进行大小的压缩,较为常见的方法则是将图片转换为base64的编码,本文就python编码和解码图片做出一定的介绍. 代码 import base64 import o ...
随机推荐
- android 入门-Service
sdk 1.7 package com.example.hellowrold; import java.util.Random; import com.example.hellowrold.R.id; ...
- C#从Image上读取文本
今天通过C#来实现一个读取Image上文本的功能. 1. 环境准备: 1). 下载 Microsoft Office SharePoint Designer 2007. 2). 安装请参考KB:htt ...
- [Skills] 在桌面打开一个BAT文件,CMD窗口不关闭
每次开机都要取得本机IP,然后远程连接上去,屏幕太小,不好输入,想写个bat,执行就能看到IP,并且停留在cmd窗口上,想来简单,以前搜了好久没找到好的办法,今天找到一个贴子,竟然可以,呵呵! 以 ...
- 安卓app设计规范整理和Android APP设计篇(转)
随着安卓智能手机不停的更新换代.安卓手机系统越来越完美,屏幕尺寸也越来越大啦!比如最近小米的miui 6的发布和魅族手机系统的更新等等. 以小米MIUI6的安卓手机来说,MIUI6进行了全新设计,坚持 ...
- java + jni + mingw实例开发(基于命令行窗口模式)
java+ jni + mingw 参考网址: http://wenku.baidu.com/link?url=9aQ88d2ieO7IgKLlNhJi5d3mb3xwzbezLPzSIX3ixz4_ ...
- FastDFS简介
一.FastDFS概述: FastDFS是一个开源的轻量级分布式文件系统,他对文件进行管理,功能包括:文件存储.文件同步.文件访问(文件上传.下载)等,解决了大容量存储和负载均衡的问题,高度追求高性能 ...
- 大端模式 VS 小端模式
简单点说,就是字节的存储顺序,如果数据都是单字节的,那怎么存储无所谓了,但是对于多字节数据,比如int,double等,就要考虑存储的顺序了.注意字节序是硬件层面的东西,对于软件来说通常是透明的.再说 ...
- strust.xml
使用strust2框架,实现跳转,请求对应路径 <?xml version="1.0" encoding="UTF-8" ?> <!DOCTY ...
- PHP之echo/print
1.PHP中有两个基本的输出方式:echo和print: 2.echo和print的区别: **echo:可以输出一个或多个字符串: **print:只允许输出一个字符串,返回值总为1: 3.echo ...
- DSP using MATLAB 示例Example3.23
代码: % Discrete-time Signal x1(n) : Ts = 0.0002 Ts = 0.0002; n = -25:1:25; nTs = n*Ts; x1 = exp(-1000 ...