POJ 题目1145/UVA题目112 Tree Summing(二叉树遍历)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 8132 | Accepted: 1949 |
Description
other important data structures such as trees.
This problem deals with determining whether binary trees represented as LISP S-expressions possess a certain property.
Given a binary tree of integers, you are to write a program that determines whether there exists a root-to-leaf path whose nodes sum to a specified integer. For example, in the tree shown below there are exactly four root-to-leaf paths. The sums of the paths
are 27, 22, 26, and 18.
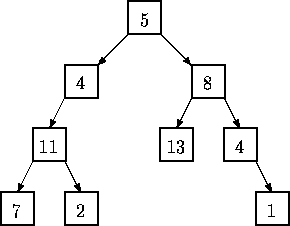
Binary trees are represented in the input file as LISP S-expressions having the following form.
empty tree ::= () tree ::= empty tree (integer tree tree)
The tree diagrammed above is represented by the expression (5 (4 (11 (7 () ()) (2 () ()) ) ()) (8 (13 () ()) (4 () (1 () ()) ) ) )
Note that with this formulation all leaves of a tree are of the form (integer () () )
Since an empty tree has no root-to-leaf paths, any query as to whether a path exists whose sum is a specified integer in an empty tree must be answered negatively.
Input
binary tree S-expressions will be valid, but expressions may be spread over several lines and may contain spaces. There will be one or more test cases in an input file, and input is terminated by end-of-file.
Output
whose sum is I and no if there is no path in T whose sum is I.
Sample Input
22 (5(4(11(7()())(2()()))()) (8(13()())(4()(1()()))))
20 (5(4(11(7()())(2()()))()) (8(13()())(4()(1()()))))
10 (3
(2 (4 () () )
(8 () () ) )
(1 (6 () () )
(4 () () ) ) )
5 ()
Sample Output
yes
no
yes
no
Source
题目大意:给出一个二叉树的表达式,问有没有一个路径和是n
ac代码
Problem: 1145 User: kxh1995
Memory: 164K Time: 0MS
Language: C++ Result: Accepted
#include<stdio.h>
int tree_sum(int n)
{
int ans=0,m;
if(scanf(" (%d",&m))
{
ans=tree_sum(n-m)+tree_sum(n-m);
if(ans<2)
ans=0;
}
else
ans=!n;
scanf(" )");
return ans;
}
int main()
{
int n;
while(scanf("%d",&n)!=EOF)
{
if(tree_sum(n))
printf("yes\n");
else
printf("no\n");
}
}
POJ 题目1145/UVA题目112 Tree Summing(二叉树遍历)的更多相关文章
- UVa 112 - Tree Summing(树的各路径求和,递归)
题目来源:https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&category=3&pa ...
- UVa 112 Tree Summing
题意: 计算从根到叶节点的累加值,看看是否等于指定值.是输出yes,否则no.注意叶节点判断条件是没有左右子节点. 思路: 建树过程中计算根到叶节点的sum. 注意: cin读取失败后要调用clear ...
- PAT 1020 Tree Traversals[二叉树遍历]
1020 Tree Traversals (25)(25 分) Suppose that all the keys in a binary tree are distinct positive int ...
- PAT甲题题解1099. Build A Binary Search Tree (30)-二叉树遍历
题目就是给出一棵二叉搜索树,已知根节点为0,并且给出一个序列要插入到这课二叉树中,求这棵二叉树层次遍历后的序列. 用结构体建立节点,val表示该节点存储的值,left指向左孩子,right指向右孩子. ...
- hdu1710(Binary Tree Traversals)(二叉树遍历)
Binary Tree Traversals Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/O ...
- PAT 甲级 1020 Tree Traversals (二叉树遍历)
1020. Tree Traversals (25) 时间限制 400 ms 内存限制 65536 kB 代码长度限制 16000 B 判题程序 Standard 作者 CHEN, Yue Suppo ...
- UVA题目分类
题目 Volume 0. Getting Started 开始10055 - Hashmat the Brave Warrior 10071 - Back to High School Physics ...
- POJ 1145 Tree Summing
Tree Summing Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 7698 Accepted: 1737 Desc ...
- uva 1556 - Disk Tree(特里)
题目连接:uva 1556 - Disk Tree 题目大意:给出N个文件夹关系,然后依照字典序输出整个文件文件夹. 解题思路:以每一个文件夹名作为字符建立一个字典树就可以,每一个节点的关系能够用ma ...
随机推荐
- python 命令行下的命令参数
本文所介绍的命令或许是在依赖包里使用的,说白了,我不太清除,由于刚开始学习,所以对知识了解不清楚,按说学习本应该学以解惑,可是为了把知识面展开的太广而影响主要知识的学习,我决定抓住主要矛盾,把有些困惑 ...
- Python安装(一)
Python的安装 打开python的官网 进入下载界面 选择下载 安装步骤如下所示: 安装完成进入到dos界面,输入python -V,如下图展示及成功 打开Python工具 1:: print() ...
- 四 过滤模式 map Only - 作业完成 bloomFilter、top10、去重
第四部分所有的模式涉及一个共同点: 不会改变原有的记录. 这些模式都是寻找数据子集的,不管结果集的规模是小(top10)还是大(像去重结果). 与第三部分差异是,他们通过对数据的相思子端坐概要与分组来 ...
- WITH common_table_expression (Transact-SQL)
https://docs.microsoft.com/en-us/sql/t-sql/queries/with-common-table-expression-transact-sql Specifi ...
- js插件---图片裁剪photoClip
js插件---图片裁剪photoClip 一.总结 一句话总结:页面裁剪图片得到base64格式的图片数据,然后把这个数据通过ajax上传给服务器,服务器将base64图片数据解析成图片并且保存到服务 ...
- pig安装配置
pig的安装配置很简单,只需要配置一下环境变量和指向hadoop conf的环境变量就行了 1.上传 2.解压 3.配置环境变量 Pig工作模式 本地模式:只需要配置PATH环境变量${PIG_HOM ...
- 二分法查找 js 算法
二分法查找算法:采用二分法查找时,数据需是排好序的.主要思想是:(设查找的数组区间为array[s, e])(1)确定该区间的中间位置m(2)将查找的值T与array[m]比较,若相等,查找成功返回此 ...
- 11/1 NOIP 模拟赛
11.1 NOIP 模拟赛 期望得分:50:实际得分:50: 思路:暴力枚举 + 快速幂 #include <algorithm> #include <cstring> #in ...
- 【Henu ACM Round#17 D】Hexagons!
[链接] 我是链接,点我呀:) [题意] 在这里输入题意 [题解] 题目的图吓人. 找下规律就会发现从内到外是1,6,12,18 即1,16,26,36... 即1+6(1+2+3+...) 等差求和 ...
- 三种new
http://www.cnblogs.com/zhuyf87/archive/2013/03/23/2976714.html 第一种是正常的new,它是语言内建的,不能重载. new operator ...