UVA1601-The Morning after Halloween(双向BFS)
Problem UVA1601-The Morning after Halloween
Accept: 289 Submit: 3136
Time Limit: 12000 mSec
Problem Description
Input
Output
For each dataset in the input, one line containing the smallest number of steps to restore ghosts into the positions where they are supposed to be should be output. An output line should not contain extra characters such as spaces.
Sample Input
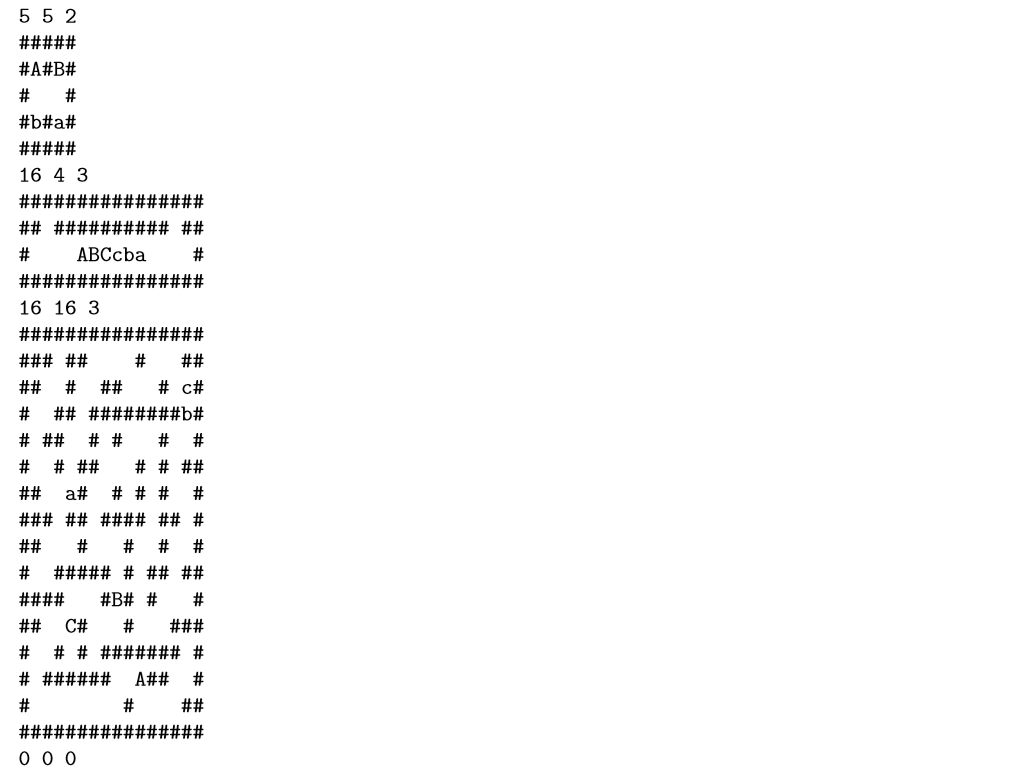
Sample Ouput
7
36
77
题解:双向BFS经典题目。
抛开双向BFS,这个题的预处理也很值得学习。节点个数太多16*16=256,并且有三个鬼,所以状态总数几乎是256^3,不管是时间还是空间,肯定都要炸,所以就要把状态算的精确一些,省去一些不可能的情况,首先最外层都是'#',这样这个图就变成了最大14*14 = 196,还是有点大,注意题目条件,每2*2中至少有一个障碍,所以这196个点至多有75%是空格,196*0.75 = 147。这就差不多了,由于后面可能有加点的操作,不过最多加2个点,也就是说总数不会超过150,这个数字就比较理想了,时间上和空间上都不错。判重的问题,刚才分析过了,每个鬼能够出现的格子数目不超过150,八位二进制数就能表示,因此三个就可以用24位二进制数表示,和int还有一段距离,所以转换成一个int型整数判重是再好不过的了。将所有空格连接起来用的是类似邻接表的操作,不过lrj没有用vector,可能还是效率原因吧。
之后就是双向BFS的模板了,两个队列,分别入队出发点和终点,vis数组肯定是三维的,只是原来的0、1变成了现在的0、1、2,因为要区分是在哪一边扩展的过程中遍历到的这个点。当一个点在两个队列中都出现了,就说明找到了,返回dis的和,别忘了+1.还有一个需要注意的是双向BFS每次扩展一层而不是扩展一个节点。原因参考下面的链接:
http://www.cppblog.com/Yuan/archive/2011/02/23/140553.aspx
先来一个单向BFS,680ms
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cstdlib>
#include <queue>
using namespace std; const int maxs = ;
const int maxn = ; int n,m,cnt,sum;
int deg[maxn],G[maxn][maxn];
int x[maxn],y[maxn],id[maxn][maxn];
int d[maxn][maxn][maxn];
int s[],t[];
int dx[] = {,,,-,};
int dy[] = {,,-,,}; int find_ID(int a,int b,int c){
return (a<<)|(b<<)|c;
} bool conflict(int a,int b,int a2,int b2){
if((a2==b && a==b2) || a2==b2) return true;
return false;
} int bfs(){
queue<int> que;
d[s[]][s[]][s[]] = ;
que.push(find_ID(s[],s[],s[]));
while(!que.empty()){
int top = que.front();que.pop();
int a = (top>>)&0xff,b = (top>>)&0xff,c = (top&0xff);
if(a==t[] && b==t[] && c==t[]) return d[a][b][c];
for(int i = ;i < deg[a];i++){
int a2 = G[a][i];
for(int j = ;j < deg[b];j++){
int b2 = G[b][j];
if(conflict(a,b,a2,b2)) continue;
for(int k = ;k < deg[c];k++){
int c2 = G[c][k];
if(d[a2][b2][c2] != -) continue;
if(conflict(a,c,a2,c2)) continue;
if(conflict(b,c,b2,c2)) continue;
d[a2][b2][c2] = d[a][b][c]+;
que.push(find_ID(a2,b2,c2));
}
}
}
}
return -;
} int main()
{
//freopen("input.txt","r",stdin);
//freopen("output.txt","w",stdout);
while(~scanf("%d%d%d\n",&m,&n,&sum) && (m||n||sum)){
char gra[maxs][maxs];
cnt = ;
memset(d,-,sizeof(d));
for(int i = ;i < n;i++) fgets(gra[i],maxs,stdin); for(int i = ;i < n;i++){
for(int j = ;j < m;j++){
if(gra[i][j] != '#'){
x[cnt] = i,y[cnt] = j,id[i][j] = cnt;
if(islower(gra[i][j])) s[gra[i][j]-'a'] = cnt;
else if(isupper(gra[i][j])) t[gra[i][j]-'A'] = cnt;
cnt++;
}
}
} for(int i = ;i < cnt;i++){
int X = x[i],Y = y[i];
deg[i] = ;
for(int k = ;k < ;k++){
int xx = X+dx[k],yy = Y+dy[k];
if(gra[xx][yy] != '#') G[i][deg[i]++] = id[xx][yy];
}
} if(sum <= ){
s[] = t[] = G[cnt][] = cnt;
deg[cnt++] = ;
}
if(sum <= ){
s[] = t[] = G[cnt][] = cnt;
deg[cnt++] = ;
} printf("%d\n",bfs());
}
return ;
}
再来一个双向BFS,530ms
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cstdlib>
#include <queue>
using namespace std; const int maxs = ;
const int maxn = ; int n,m,cnt,sum;
int deg[maxn],G[maxn][maxn];
int x[maxn],y[maxn],id[maxn][maxn];
int d[maxn][maxn][maxn];
int vis[maxn][maxn][maxn];
int s[],t[];
int dx[] = {,,,-,};
int dy[] = {,,-,,}; int find_ID(int a,int b,int c){
return (a<<)|(b<<)|c;
} bool conflict(int a,int b,int a2,int b2){
if((a2==b && a==b2) || a2==b2) return true;
return false;
} int bfs(){
queue<int> que,anti_que;
d[s[]][s[]][s[]] = ;
d[t[]][t[]][t[]] = ; que.push(find_ID(s[],s[],s[]));
anti_que.push(find_ID(t[],t[],t[])); vis[s[]][s[]][s[]] = ;
vis[t[]][t[]][t[]] = ; while(!que.empty() && !anti_que.empty()){
int num = que.size(),anti_num = anti_que.size();
while(num--){
int top = que.front();que.pop();
int a = (top>>)&0xff,b = (top>>)&0xff,c = (top&0xff); for(int i = ;i < deg[a];i++){
int a2 = G[a][i];
for(int j = ;j < deg[b];j++){
int b2 = G[b][j];
if(conflict(a,b,a2,b2)) continue;
for(int k = ;k < deg[c];k++){
int c2 = G[c][k];
if(conflict(a,c,a2,c2)) continue;
if(conflict(b,c,b2,c2)) continue; if(vis[a2][b2][c2] == ){
d[a2][b2][c2] = d[a][b][c]+;
vis[a2][b2][c2] = ;
que.push(find_ID(a2,b2,c2));
}
else if(vis[a2][b2][c2] == ){
return d[a][b][c]+d[a2][b2][c2]+;
}
}
}
}
} while(anti_num--){
int top = anti_que.front();anti_que.pop();
int a = (top>>)&0xff,b = (top>>)&0xff,c = (top&0xff); for(int i = ;i < deg[a];i++){
int a2 = G[a][i];
for(int j = ;j < deg[b];j++){
int b2 = G[b][j];
if(conflict(a,b,a2,b2)) continue;
for(int k = ;k < deg[c];k++){
int c2 = G[c][k];
if(conflict(a,c,a2,c2)) continue;
if(conflict(b,c,b2,c2)) continue; if(vis[a2][b2][c2] == ){
d[a2][b2][c2] = d[a][b][c]+;
vis[a2][b2][c2] = ;
anti_que.push(find_ID(a2,b2,c2));
}
else if(vis[a2][b2][c2] == ){
return d[a][b][c]+d[a2][b2][c2]+;
}
}
}
}
}
}
return -;
} int main()
{
//freopen("input.txt","r",stdin);
//freopen("output.txt","w",stdout);
while(~scanf("%d%d%d\n",&m,&n,&sum) && (m||n||sum)){
char gra[maxs][maxs];
cnt = ;
memset(d,-,sizeof(d));
memset(vis,,sizeof(vis));
for(int i = ;i < n;i++) fgets(gra[i],maxs,stdin); for(int i = ;i < n;i++){
for(int j = ;j < m;j++){
if(gra[i][j] != '#'){
x[cnt] = i,y[cnt] = j,id[i][j] = cnt;
if(islower(gra[i][j])) s[gra[i][j]-'a'] = cnt;
else if(isupper(gra[i][j])) t[gra[i][j]-'A'] = cnt;
cnt++;
}
}
} for(int i = ;i < cnt;i++){
int X = x[i],Y = y[i];
deg[i] = ;
for(int k = ;k < ;k++){
int xx = X+dx[k],yy = Y+dy[k];
if(gra[xx][yy] != '#') G[i][deg[i]++] = id[xx][yy];
}
} if(sum <= ){
s[] = t[] = G[cnt][] = cnt;
deg[cnt++] = ;
}
if(sum <= ){
s[] = t[] = G[cnt][] = cnt;
deg[cnt++] = ;
} printf("%d\n",bfs());
}
return ;
}
UVA1601-The Morning after Halloween(双向BFS)的更多相关文章
- UVa1601 - The Morning after Halloween [单向bfs]
解题思路: 1.注意到2*2方格中必有一个#,那么最多只有192条通道,可以将所有非‘#’的位置提取出来用邻接表的方式建图,通过bfs搜索目标位置. 2.将三个ghost的位置(a,b,c)作为状态量 ...
- UVa 1601 || POJ 3523 The Morning after Halloween (BFS || 双向BFS && 降维 && 状压)
题意 :w*h(w,h≤16)网格上有n(n≤3)个小写字母(代表鬼).要求把它们分别移动到对应的大写字母里.每步可以有多个鬼同时移动(均为往上下左右4个方向之一移动),但每步结束之后任何两个鬼不能占 ...
- UVA-1601 The Morning after Halloween(BFS或双向BFS)
题目大意:在一张图中,以最少的步数将a,b,c移到对应的A,B,C上去.其中,每个2x2的方格都有障碍并且不能两个小写字母同时占据一个格子. 题目分析:为避免超时,先将图中所有能联通的空格建起一张图, ...
- 【UVa】1601 The Morning after Halloween(双向bfs)
题目 题目 分析 双向bfs,对着书打的,我还调了好久. 代码 #include<cstdio> #include<cstring> #include<c ...
- UVA - 1601 The Morning after Halloween (双向BFS&单向BFS)
题目: w*h(w,h≤16)网格上有n(n≤3)个小写字母(代表鬼).要求把它们分别移动到对应的大写字母里.每步可以有多个鬼同时移动(均为往上下左右4个方向之一移动),但每步结束之后任何两个鬼不能占 ...
- POJ1915Knight Moves(单向BFS + 双向BFS)
题目链接 单向bfs就是水题 #include <iostream> #include <cstring> #include <cstdio> #include & ...
- HDU 3085 Nightmare II 双向bfs 难度:2
http://acm.hdu.edu.cn/showproblem.php?pid=3085 出的很好的双向bfs,卡时间,普通的bfs会超时 题意方面: 1. 可停留 2. ghost无视墙壁 3. ...
- POJ 3170 Knights of Ni (暴力,双向BFS)
题意:一个人要从2先走到4再走到3,计算最少路径. 析:其实这个题很水的,就是要注意,在没有到4之前是不能经过3的,一点要注意.其他的就比较简单了,就是一个双向BFS,先从2搜到4,再从3到搜到4, ...
- [转] 搜索之双向BFS
转自:http://www.cppblog.com/Yuan/archive/2011/02/23/140553.aspx 如果目标也已知的话,用双向BFS能很大程度上提高速度. 单向时,是 b^le ...
随机推荐
- Java先比较日期再比较时间
package com.bihang.seaya; import lombok.Data; import java.text.ParseException; import java.text.Simp ...
- Http(s)与后台交互方式
前言 Http(s)是前后端交互的主要方式之一,交互技术主要有:Ajax(XMLHttpRequest).Fetch.地址跳转(window.open.location.href).Http(s)与后 ...
- JavaScript是如何工作的:Service Worker的生命周期及使用场景
摘要: 理解Service Worker. 原文:JavaScript 是如何工作的:Service Worker 的生命周期及使用场景 作者:前端小智 Fundebug经授权转载,版权归原作者所有. ...
- qW3xT.2,解决挖矿病毒。
网站在运行期间感觉怪怪的,响应速度慢的不是一丁半点,带宽5M,不该是这样的呀 于是登录Xshell top命令 查看cpu情况如下 PID为3435的进程占用CPU过大,难道被病毒入侵了吗? 查看该进 ...
- DOM技术
DOM概述 DOM:Document Object Model(文档对象模型)(DOM核心就是 文档变对象,标签也变对象,属性也变对象,反正就是把标记文档拆散) 用来将标记型对象封装成对象,并将标记型 ...
- IP 协议
在网络层中,使用的是 ip 协议,它规定网络地址的协议. ip 地址分为两个部分: 网络部分:标识子网 主机部分:标识主机 子网掩码 表示子网络特征的一个参数,它规定 网络部分全部为1,主机部分全部为 ...
- BZOJ3453: tyvj 1858 XLkxc(拉格朗日插值)
题意 题目链接 Sol 把式子拆开,就是求这个东西 \[\sum_{i = 0} ^n \sum_{j = 1}^{a + id} \sum_{x =1}^j x^k \pmod P\] 那么设\(f ...
- Javascript 对象 - 数组对象
JavaScript核心对象 数组对象Array 字符串对象String 日期对象Date 数学对象Math 数组对象 数组对象是用来在单一的变量名中存储一系列的值.数组是在编程语言中经常使用的一种数 ...
- 算法:用两个栈来实现一个队列,完成队列的Push和Pop操作。 队列中的元素为int类型。
算法:用两个栈来实现一个队列,完成队列的Push和Pop操作. 队列中的元素为int类型.<剑指offer> 利用栈来进行操作,代码注释写的比较清楚:首先判断两个栈是否是空的:其次当栈二 ...
- 语句调优基础知识-set statistics time on
set statistics time on --清空缓存数据 dbcc dropcleanbuffers go --清空缓存计划 dbcc freeproccache go set statisti ...