Widget Size and Position !!!!!!!!!!!!!!!!!!
https://medium.com/@diegoveloper/flutter-widget-size-and-position-b0a9ffed9407
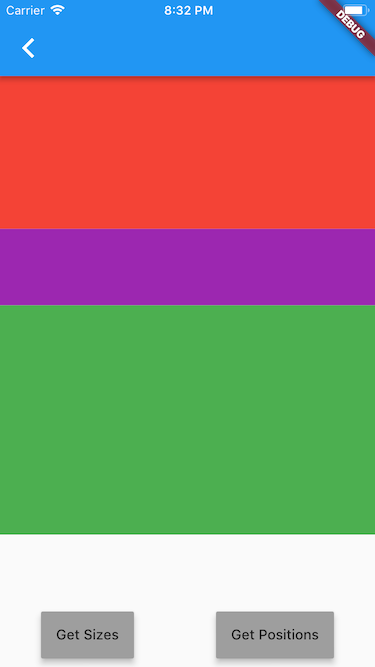
I have read many questions about how we can obtain the dimensions or positions of the widgets that we have on screen.
In some cases we find ourselves in situations in which we want to achieve that for any reason.
The widget doesn’t have position or size by itself, in order to achieve this it’s necessary that we obtain the RenderBox associated with the context of our Widget.
But how do we do this?
Let’s start building a demo app which has 3 panels of different colors, Red, Purple and Green inside a Column and we have two buttons at the bottom to get the Size and Position.
This is the code of the demo app:
And the result:
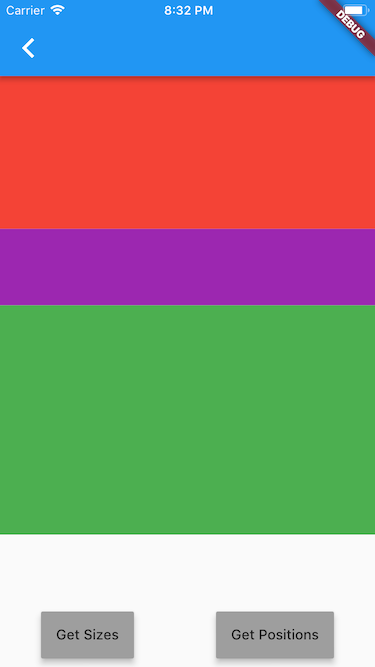
Ok so now the question is : How can I get the size and position of each panel?
Let’s focus on just one panel for this post (Red panel) , after we know how to get the size and position for one panel it should be easy for the others.
Get the size of a Widget
In order to do that, we need our Widget to have a Key, for this we create a GlobalKey and assign it to our Widget.
//creating Key for red panel
GlobalKey _keyRed = GlobalKey();
...
//set key
Flexible(
flex: 2,
child: Container(
key: _keyRed,
color: Colors.red,
),
),
Once our Widget already has a Key, we can use this Key to be able to obtain the size in the following way:
_getSizes() {
final RenderBox renderBoxRed = _keyRed.currentContext.findRenderObject();
final sizeRed = renderBoxRed.size;
print("SIZE of Red: $sizeRed");
}
If we press the Get Sizes button, you’ll get this result in the console:
flutter: SIZE of Red: Size(375.0, 152.9)
now we know that our Red panel has 375.0 as width and 152.9 as height
It was easy, right?
Let’s go to obtain the position in which our Widget is located.
Get the position of a Widget
In the same way that we did previously, our Widget must have an associated Key.
Now we update the method to obtain the position of the Widget relative to the top-left of the defined position (in this case we are using 0.0 it means the top-left corner of our current screen).
_getPositions() {
final RenderBox renderBoxRed = _keyRed.currentContext.findRenderObject();
final positionRed = renderBoxRed.localToGlobal(Offset.zero);
print("POSITION of Red: $positionRed ");
}
If we press the Get Positions button, you’ll get this result in the console:
flutter: POSITION of Red: Offset(0.0, 76.0)
It means our Widget start from 0.0 from the X axis and 76.0 from the Y axis from TOP-LEFT.
Why 76.0? That’s because there is an AppBar above that has a height of 76.0.
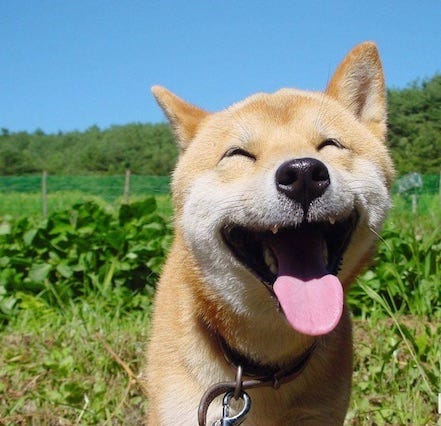
So we already know how to get the size and position of our Widgets, well so far. Yay!!
But what happens if I’m interested in getting the size or position at the beginning, without having to press a button for it.
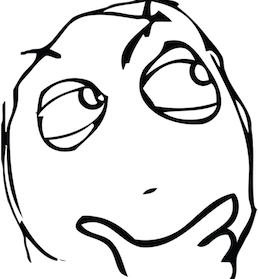
Ok then let’s call our methods in our constructor.
_MainSizeAndPositionState(){
_getSizes();
_getPositions();
}
Run the app and …. oh we have an error here:
flutter: The following NoSuchMethodError was thrown building Builder:
flutter: The method 'findRenderObject' was called on null.
flutter: Receiver: null
flutter: Tried calling: findRenderObject()
Ok let’s try calling the methods from the initState , it should work ….. or no
@override
void initState() {
_getSizes();
_getPositions();
super.initState();
}
Run the app and … a little different but the same error:
flutter: Another exception was thrown: NoSuchMethodError: The method 'findRenderObject' was called on null.
So, we have a problem, we can not get the size or position at the beginning, so how do I do it?
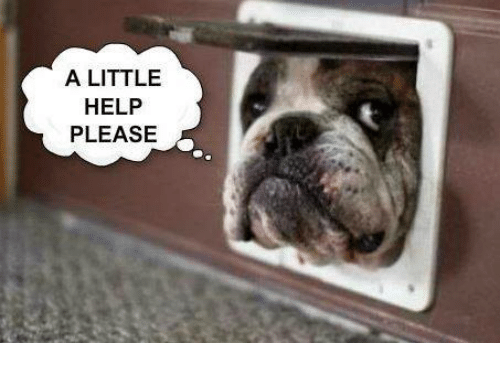
This happens because the context is not yet associated with our state.
You can find more information about the lifecycle of the Widgets here: https://medium.com/flutter-community/widget-state-buildcontext-inheritedwidget-898d671b7956
So we have to wait for our Widget to finish rendering, but how can achieve that?
There is a simple way, as I show you below.
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_afterLayout);
super.initState();
}
_afterLayout(_) {
_getSizes();
_getPositions();
}
With this you make sure to call your methods after the layout is completed.
If we run our app again, this is the result:
flutter: SIZE of Red: Size(375.0, 152.9)
flutter: POSITION of Red: Offset(0.0, 76.0)
Finally!!!
You can also review this package created by my friend Simon Lightfoot here: https://pub.dartlang.org/packages/after_layout
Conclusion
Many times, we get complicated by things that are very simple, it is necessary to read the documentation that Flutter provides, anyway every day we learn new things.
You can check the source code in my flutter-samples repo https://github.com/diegoveloper/flutter-samples
Widget Size and Position !!!!!!!!!!!!!!!!!!的更多相关文章
- 【Cocos2d-x】源代码分析之 2d/ui/Widget
从今天開始 咱也模仿 红孩儿这些大牛分析源代码 ,因为水平有限 不正确之处欢迎狂喷.哈哈. #ifndef __UIWIDGET_H__ #define __UIWIDGET_H__ #include ...
- Android学习笔记_63_手机安全卫士知识点归纳(3)分享 程序锁 服务 进程管理 widget
1.分享: Intent shareIntent = new Intent(); shareIntent.setAction(Intent.ACTION_SEND); shareIntent.setT ...
- OSG Qt Widget加载三维模型
graphicswindowqt.h #ifndef GRAPHICSWINDOWQT_H #define GRAPHICSWINDOWQT_H #include <QGLWidget> ...
- iOS-CALayer中position与anchorPoint详解
iOS-CALayer中position与anchorPoint详解 属性介绍 CALayer通过四个属性来确定大小和位置, 分别为:frame.bounds.position.anchorPoint ...
- QT把widget转换成图片后打印
from PyQt5.QtWidgets import (QApplication, QWidget, QTableWidget,QPushButton, QVBoxLayout, QTableWid ...
- Qt 【widget如何铺满窗口】
刚接触qt不是很长时间,都是使用ui拖拽控件实现界面,然后发现有些问题就是控件一旦多了起来,拖拽就不好控制了,然后就转而使用纯代码开发. 一下是碰到第一个问题: 创建一个MainWidget; Mai ...
- 第15.30节 PyQt编程实战:通过eventFilter监视QScrollArea的widget()的Paint消息画出scrollAreaWidgetContents的范围矩形
老猿Python博文目录 专栏:使用PyQt开发图形界面Python应用 老猿Python博客地址 一.引言 在<PyQt(Python+Qt)学习随笔:QScrollArea滚动区域详解> ...
- 带你实现开发者头条APP(五)--RecyclerView下拉刷新上拉加载
title: 带你实现开发者头条APP(五)--RecyclerView下拉刷新上拉加载 tags: -RecyclerView,下拉刷新,上拉加载更多 grammar_cjkRuby: true - ...
- Android之ListView&ViewPager模拟新闻界面
模拟新闻 APP 的界面 1)写 ListView 之前先写布局: 这里有两种 Item 的布局: <?xml version="1.0" encoding="ut ...
随机推荐
- iwms后台编辑器无法粘贴word格式的解决方法
iwms后台编辑器用的是tiny_mce,默认会自动过滤word粘贴中的格式,以减小数据库的占用,但在word中辛苦做的字体和格式都不见了,可采用下方法关闭编辑器的自动清除格式功能. 编辑文件:\ti ...
- CS224n学习笔记(三)
语言模型 对于一个文本中出现的单词 \(w_i\) 的概率,他更多的依靠的是前 \(n\) 个单词,而不是这句话中前面所有的单词. \[ P\left(w_{1}, \ldots, w_{m}\rig ...
- Service Function Chaining Resource Allocation: A Survey
摘要: 服务功能链(SFC)是未来Internet的一项关键技术. 它旨在克服当前部署模型的僵化和静态限制. 该技术的应用依赖于可以将SFC最佳映射到衬底网络的算法. 这类算法称为"服务功能 ...
- A3C 算法资料收集
A3C 算法资料收集 2019-07-26 21:37:55 Paper: https://arxiv.org/pdf/1602.01783.pdf Code: 1. 超级马里奥:https://gi ...
- python去除BOM头\ufeff等特殊字符
1.\ufeff 字节顺序标记 去掉\ufeff,只需改一下编码就行,把UTF-8编码改成UTF-8-sigwith open(file_path, mode='r', encoding='UTF-8 ...
- spring 技术内幕读书笔记1
1 在 java 应用开发中,往往会涉及复杂的对象耦合关系,在 代码中处理这些耦合关系,对代码的维护性和应用扩展性会带来许多不便.通过使用spring 的 IOC 容器,可以对这些耦合关系实现一个文本 ...
- 微信小程序开发——微信小程序下拉刷新真机无法弹回
开发工具中下拉之后页面回弹有一定的延迟,这个时间也有点久.真机测试,下拉后连回弹都没有,这个问题要解决,就得在下拉函数里加上停止下拉刷新的API,如下: /** * 下拉刷新 */ onPullDow ...
- filebeat获取nginx的access日志配置
filebeat获取nginx的access日志配置 产生nginx日志的服务器即生产者服务器配置: 拿omp.chinasoft.com举例: .nginx.conf主配置文件添加日志格式 log_ ...
- Qt编写气体安全管理系统27-设备调试
一.前言 设备调试核心就是将整个系统中的所有打印数据统一显示到一个模块上,一般都会将硬件通信的收发数据和对应的解析信号发出来或者qdebug出来,这个在调试阶段非常有用,可以具体追踪问题出在哪,哪个数 ...
- springboot:redis反序列化发生类型转换错误
明明是同一个类,在反序列时报类型转换错误,真实奇怪.经查找资料,说是引入了devtools的缘故. 注释掉以下内容: <dependency> <groupId>org.spr ...