UVA 116 Unidirectional TSP(DP最短路字典序)
Description
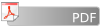
Unidirectional TSP |
Background
Problems that require minimum paths through some domain appear in many different areas of computer science. For example, one of the constraints in VLSI routing problems is minimizing wire length. The Traveling Salesperson Problem (TSP) -- finding whether all the cities in a salesperson's route can be visited exactly once with a specified limit on travel time -- is one of the canonical examples of an NP-complete problem; solutions appear to require an inordinate amount of time to generate, but are simple to check.
This problem deals with finding a minimal path through a grid of points while traveling only from left to right.
The Problem
Given an matrix of integers, you are to write a program that computes a path of minimal weight. A path starts anywhere in column 1 (the first column) and consists of a sequence of steps terminating in column n (the last column). A step consists of traveling from column i to column i+1 in an adjacent (horizontal or diagonal) row. The first and last rows (rows 1 and m) of a matrix are considered adjacent, i.e., the matrix ``wraps'' so that it represents a horizontal cylinder. Legal steps are illustrated below.
The weight of a path is the sum of the integers in each of the n cells of the matrix that are visited.
For example, two slightly different matrices are shown below (the only difference is the numbers in the bottom row).
The minimal path is illustrated for each matrix. Note that the path for the matrix on the right takes advantage of the adjacency property of the first and last rows.
The Input
The input consists of a sequence of matrix specifications. Each matrix specification consists of the row and column dimensions in that order on a line followed by integers where m is the row dimension and n is the column dimension. The integers appear in the input in row major order, i.e., the first n integers constitute the first row of the matrix, the second n integers constitute the second row and so on. The integers on a line will be separated from other integers by one or more spaces. Note: integers are not restricted to being positive. There will be one or more matrix specifications in an input file. Input is terminated by end-of-file.
For each specification the number of rows will be between 1 and 10 inclusive; the number of columns will be between 1 and 100 inclusive. No path's weight will exceed integer values representable using 30 bits.
The Output
Two lines should be output for each matrix specification in the input file, the first line represents a minimal-weight path, and the second line is the cost of a minimal path. The path consists of a sequence of n integers (separated by one or more spaces) representing the rows that constitute the minimal path. If there is more than one path of minimal weight the path that is lexicographically smallest should be output.
Sample Input
5 6
3 4 1 2 8 6
6 1 8 2 7 4
5 9 3 9 9 5
8 4 1 3 2 6
3 7 2 8 6 4
5 6
3 4 1 2 8 6
6 1 8 2 7 4
5 9 3 9 9 5
8 4 1 3 2 6
3 7 2 1 2 3
2 2
9 10 9 10
Sample Output
1 2 3 4 4 5
16
1 2 1 5 4 5
11
1 1
19
/*
从前往后推,把所有解找出来再找字典序最小的,WA了。
从后往前推,直接是字典序最小的。
*/
#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
using namespace std; const int INF=;
int d[][],dir[][]={,-,-,-,,-};
int m,n,a[][],next[][]; int main()
{
int i,j,k;
while(~scanf("%d%d",&n,&m))
{
for(i=;i<=n;i++)for(j=;j<=m;j++) scanf("%d",&a[i][j]);
memset(next,-,sizeof(next));
for(i=;i<=n;i++) d[i][m]=a[i][m];
for(i=;i<=n;i++) for(j=;j<m;j++) d[i][j]=INF;
for(i=m;i>=;i--)
{
for(j=;j<=n;j++)
{
for(k=;k<;k++)
{
int x=((j+dir[k][]-)%n+n)%n+;
int y=i+dir[k][];
if(d[x][y]>d[j][i]+a[x][y])
{
d[x][y]=d[j][i]+a[x][y];
next[x][y]=j;
}
else if(d[x][y] == d[j][i]+a[x][y] && next[x][y] > j)
next[x][y] = j; }
}
}
int ansm=INF,ansi;
for(i=;i<=n;i++)
if(ansm>d[i][])
{
ansm=d[i][];ansi=i;
}
for(i=;i<=m;i++)
{
printf(i==?"%d":" %d",ansi);
ansi=next[ansi][i];
}
printf("\n%d\n",ansm);
}
return ;
}
UVA 116 Unidirectional TSP(DP最短路字典序)的更多相关文章
- UVA 116 Unidirectional TSP(dp + 数塔问题)
Unidirectional TSP Background Problems that require minimum paths through some domain appear in ma ...
- UVa 116 Unidirectional TSP (DP)
该题是<算法竞赛入门经典(第二版)>的一道例题,难度不算大.我先在没看题解的情况下自己做了一遍,虽然最终通过了,思路与书上的也一样.但比书上的代码复杂了很多,可见自己对问题的处理还是有所欠 ...
- uva 116 Unidirectional TSP (DP)
uva 116 Unidirectional TSP Background Problems that require minimum paths through some domain appear ...
- uva 116 Unidirectional TSP【号码塔+打印路径】
主题: uva 116 Unidirectional TSP 意甲冠军:给定一个矩阵,当前格儿童值三个方向回格最小值和当前的和,就第一列的最小值并打印路径(同样则去字典序最小的). 分析:刚開始想错了 ...
- UVA - 116 Unidirectional TSP 多段图的最短路 dp
题意 略 分析 因为字典序最小,所以从后面的列递推,每次对上一列的三个方向的行排序就能确保,数字之和最小DP就完事了 代码 因为有个地方数组名next和里面本身的某个东西冲突了,所以编译错了,后来改成 ...
- UVA 116 Unidirectional TSP 经典dp题
题意:找最短路,知道三种行走方式,给出图,求出一条从左边到右边的最短路,且字典序最小. 用dp记忆化搜索的思想来考虑是思路很清晰的,但是困难在如何求出字典序最小的路. 因为左边到右边的字典序最小就必须 ...
- uva 116 Unidirectional TSP(动态规划,多段图上的最短路)
这道题目并不是很难理解,题目大意就是求从第一列到最后一列的一个字典序最小的最短路,要求不仅输出最短路长度,还要输出字典序最小的路径. 这道题可以利用动态规划求解.状态定义为: cost[i][j] = ...
- UVA - 116 Unidirectional TSP (单向TSP)(dp---多段图的最短路)
题意:给一个m行n列(m<=10, n<=100)的整数矩阵,从第一列任何一个位置出发每次往右,右上或右下走一格,最终到达最后一列.要求经过的整数之和最小.第一行的上一行是最后一行,最后一 ...
- UVa - 116 - Unidirectional TSP
Background Problems that require minimum paths through some domain appear in many different areas of ...
随机推荐
- vs 2012打开vs2013的sln
Project -> Properties -> General -> Platform Toolset (as IInspectable correctly commented)
- cocostudio的bug(1)
今天有个女同事问我一个问题,两个cocostudio的ui同时addChild到一个layer上面,高层级的ui设置visible为false,低层级的ui设置的visible设置为true,然后低层 ...
- 代码块(block)的使用
Objective-C语法之代码块(block)的使用 代码块本质上是和其他变量类似.不同的是,代码块存储的数据是一个函数体.使用代码块是,你可以像调用其他标准函数一样,传入参数数,并得到返回值. 脱 ...
- Vue和MVVM对应关系
Vue和MVVM的对应关系 Vue是受MVVM启发的,那么有哪些相同之处呢?以及对应关系? MVVM(Model-view-viewmodel) MVVM还有一种模式model-view-binder ...
- ubuntu 16.04 + 中文输入法
在桌面右上角设置图标中找到"System Setting",双击打开. 在打开的窗口里找到"Language Support",双击打开. 可能打开会说没有安装 ...
- OI杂记
从今天开始记录一下为数不多天的OI历程 8.25 上 今天举行了难得的五校联考,模拟noip,题目的解压密码竟然是$aKnoIp2o18$,对你没有看错!!! 7:50老师?啊啊啊啊,收不到题目啊,还 ...
- Java-JFrame窗体美化
Java-JFrame窗体美化 JFrame默认的窗体比较土,可以通过一定的美化,让窗体表现的比较漂亮,具体要根据设计的设计图进行美化: JFrame美化的大致思路:先将JFrame去除默认美化效果, ...
- python基础-面向对象的三大特征
继承 单继承 父类 基类 子类 派生类 继承:是面向对象软件技术当中的一个概念,如果一个类别A“继承自”另一个类别B,就把这个A称为“B的子类别”,而把B称为“A的父类别”也可以称“B是A的超类”. ...
- Python中的并发
目录 Python并发 并发三种层次 协程 生成者消费者 新关键字 网络io 线/进程 例子 线程池 进程通信 并发池 future对象 executor对象 参考 Python并发 并发三种层次 个 ...
- ubuntu12.04安装teamviewer
ubuntu 12.04 64位 下载地址:http://downloadap2.teamviewer.com/download/teamviewer_linux_x64.deb 下载之后,选中,右击 ...