【转】go里面字符串转成 字节slice, 字节slice转成字符串
原文: https://yourbasic.org/golang/convert-string-to-byte-slice/#convert-string-to-bytes
---------------------------------------------------------------------------
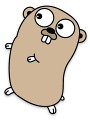
Basics
When you convert between a string and a byte slice (array), you get a brand new slice that contains the same bytes as the string, and vice versa.
- The conversion doesn’t change the data;
- the only difference is that strings are immutable, while byte slices can be modified.
If you need to manipulate the characters (runes) of a string, you may want to convert the string to a rune slice instead. See Convert between rune array/slice and string.
Convert string to bytes
When you convert a string to a byte slice, you get a new slice that contains the same bytes as the string.
b := []byte("ABC€")
fmt.Println(b) // [65 66 67 226 130 172]
Note that the character €
is encoded in UTF-8 using 3 bytes. See the Go rune articlefor more on UTF-8 encoding of Unicode code points.
Convert bytes to string
When you convert a slice of bytes to a string, you get a new string that contains the same bytes as the slice.
s := string([]byte{65, 66, 67, 226, 130, 172})
fmt.Println(s) // ABC€
Performance
These conversions create a new slice or string, and therefore have time complexityproportional to the number of bytes that are processed.
More efficient alternative
In some cases, you might be able to use a string builder, which can concatenate strings without redundant copying:
【转】go里面字符串转成 字节slice, 字节slice转成字符串的更多相关文章
- Python入门篇-基础数据类型之整型(int),字符串(str),字节(bytes),列表(list)和切片(slice)
Python入门篇-基础数据类型之整型(int),字符串(str),字节(bytes),列表(list)和切片(slice) 作者:尹正杰 版权声明:原创作品,谢绝转载!否则将追究法律责任. 一.Py ...
- Java字节、十进制、十六进制、字符串之间的相互转换
1. 字节转10进制 直接使用(int)类型转换. /* * 字节转10进制 */ public static int byte2Int(byte b){ int r = (int) b; retur ...
- java中字节数组byte[]和字符(字符串)之间的转换
转自:http://blog.csdn.net/linlzk/article/details/6566124 Java与其他语言编写的程序进行tcp/ip socket通讯时,通讯内容一般都转换成by ...
- C#//字节数组转16进制字符串
//字节数组转16进制字符串 private static string byteToHexStr(byte[] bytes,int length) { string returnStr = &quo ...
- 《将一个字符串转换成datetime时,先分析该字符串以获取日期,然后再将每个变量放置到datetime对象中》的解决办法
我们在写代码时,稍不注意就收到VS那文不对题的错误提示. 最近在项目上碰到了“将一个字符串转换成datetime时,先分析该字符串以获取日期,然后再将每个变量放置到datetime对象中”的这个错误提 ...
- C++:怎样把一个int转成4个字节?
大家都知道,一个int 或 unsigned int是由4个字节组成的,(<C/C++学习指南>,第3章,第3.2.3节:变量的内存视图) 比如, int n = sizeof( ...
- 字符串A转换到字符串B,只能一次一次转换,每次转换只能把字符串A中的一个字符全部转换成另一个字符,是否能够转换成功
public class DemoTest { public static void main(String[] args) { System.)); } /** * 有一个字符串A 有一个字符串B ...
- JS的slice、substring、substr字符串截取
JS中截取一个字符串的三种方法:字符串.slice(开始索引,结束索引)字符串.substring(开始索引,结束索引)字符串.substr(开始索引,截取的长度) 如果需要截取到该字符串的最后,可以 ...
- 给定一个字符串,把字符串内的字母转换成该字母的下一个字母,a换成b,z换成a,Z换成A,如aBf转换成bCg, 字符串内的其他字符不改变,给定函数,编写函数 void Stringchang(const char*input,char*output)其中input是输入字符串,output是输出字符串
import java.util.Scanner; /*** * 1. 给定一个字符串,把字符串内的字母转换成该字母的下一个字母,a换成b,z换成a,Z换成A,如aBf转换成bCg, 字符串内的其他字 ...
- js字符串长度计算(一个汉字==两个字符)和字符串截取
js字符串长度计算(一个汉字==两个字符)和字符串截取 String.prototype.realLength = function() { return this.replace(/[^\x00-\ ...
随机推荐
- idea查看接口或类的所有方法
第一种: 显示结果: 第二种: 点击左显示栏的Structure: 第三种:ctrl+f12,有的电脑可能需要加fn键
- SourceTree使用图解
看完这篇文档你能做到的是: 1.简单的用Git管理项目. 2.怎样既要开发又要处理发布出去的版本bug情况. SourceTree是一个免费的Git图形化管理工具,mac下也可以安装. 下载地址:ht ...
- linux shell `符号详解
linux shell `符号详解 <pre>[root@iZ23uewresmZ arjianghu]# echo `ls`asss.html common guaji.php imag ...
- Clustering and Exploring Search Results using Timeline Constructions (paper2)
作者:Omar Alonso 会议:CIKM 2009 摘要: 截至目前(2009),通过提取文档中内嵌的时间信息来展现和聚类,这方面的工作并不多. 在这篇文章中,我们将提出一个“小插件”增添到现有的 ...
- 2、1 昨天讲列表缓存,为了让列表更新,我们需要在增、删、改方法之前加 @CacheEvict(value="list",allEntries = true)
package com.bw.service; import java.util.List; import javax.annotation.Resource; import org.springfr ...
- 事务的ACID
事务提供一种机制将一个活动涉及的所有操作纳入到一个不可分割的执行单元,组成事务的所有操作只有在所有操作均能正常执行的情况下方能提交,只要其中任一操作执行失败,都将导致整个事务的回滚. 简单地说,事务提 ...
- C++:标准模板库Sort
一.概述 STL几乎封装了所用的数据结构中的算法,这里主要介绍排序算法的使用,指定排序迭代器区间后,即可实现排序功能. 所需头文件#include <algorithm> sort函数:对 ...
- 数位dp踩坑
前言 数位DP是什么?以前总觉得这个概念很高大上,最近闲的没事,学了一下发现确实挺神奇的. 从一道简单题说起 hdu 2089 "不要62" 一个数字,如果包含'4'或者'62', ...
- 利用Python进行数据分析_Pandas_层次化索引
申明:本系列文章是自己在学习<利用Python进行数据分析>这本书的过程中,为了方便后期自己巩固知识而整理. 层次化索引主要解决低纬度形式处理高纬度数据的问题 import pandas ...
- 控制层解析post请求中json数据的时候,有些属性值为空
原因: 1.默认json数据解析的时候,值会赋给键的首字母是小写的封装的bean中的属性,如果没有首字母小写的属性,也不会报错.即bean中有getXXX方法时,从json到model会增加xxx属性 ...