Spring报错:java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist
感谢:http://blog.chinaunix.net/uid-20681545-id-184633.html提供的解决方案,非常棒 !
问题说明:
新建一个Spring项目,新建一个Bean类:HelloWorld类,Main.java是主程序,xml是Spring配置文件。
项目结构如下:
打开文件夹,src目录下的结构如下:
打开bin文件夹,目录如下
HelloWorld.java代码:
package com.tt.spring.beans; public class HelloWorld { private String name; public void setName(String name) {
this.name = name;
} public void hello(){
System.out.println("hello: "+name);
} }
Main.java
package com.tt.spring.beans; import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext; public class Main { public static void main(String[] args){
/*
//创建HelloWorld的一个对象
HelloWorld helloWorld = new HelloWorld();
//为name属性赋值
helloWorld.setName("tt");
*/
//调用hello方法 //1.创建Spring 的 IOC 容器对象
ApplicationContext ctx = new ClassPathXmlApplicationContext("applicationContext.xml"); //2.从IOC容器中获取Bean实例
HelloWorld helloWorld = (HelloWorld) ctx.getBean("helloWorld"); //3.调用Hello方法
helloWorld.hello(); }
}
applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd"> <!-- 配置bean -->
<bean id="helloWorld" class="com.tt.spring.beans.HelloWorld">
<property name="name" value="Spring"></property>
</bean>
</beans>
运行Main.java程序,结果显示报错。报错如下:
信息: Loading XML bean definitions from class path resource [applicationContext.xml]
Exception in thread "main" org.springframework.beans.factory.BeanDefinitionStoreException: IOException parsing XML document from class path resource [applicationContext.xml]; nested exception is java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.loadBeanDefinitions(XmlBeanDefinitionReader.java:343)
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.loadBeanDefinitions(XmlBeanDefinitionReader.java:303)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:180)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:216)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:187)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:251)
at org.springframework.context.support.AbstractXmlApplicationContext.loadBeanDefinitions(AbstractXmlApplicationContext.java:127)
at org.springframework.context.support.AbstractXmlApplicationContext.loadBeanDefinitions(AbstractXmlApplicationContext.java:93)
at org.springframework.context.support.AbstractRefreshableApplicationContext.refreshBeanFactory(AbstractRefreshableApplicationContext.java:129)
at org.springframework.context.support.AbstractApplicationContext.obtainFreshBeanFactory(AbstractApplicationContext.java:540)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:454)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:139)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:83)
at com.tt.spring.beans.Main.main(Main.java:18)
Caused by: java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist
at org.springframework.core.io.ClassPathResource.getInputStream(ClassPathResource.java:158)
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.loadBeanDefinitions(XmlBeanDefinitionReader.java:329)
... 13 more
问题分析:
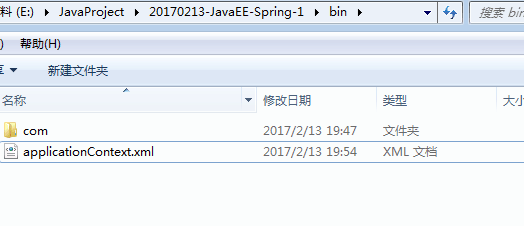
现在目录树如下:
1 package com.tt.spring.beans;
2
3 public class Car {
4
5 private String brand;
6 private String corp;
7 private int price;
8 private int maxSpeed;
9
10
11 public Car() {
12 System.out.println("Car's Constructor");
13 }
14
15 public Car(String brand, String corp, int price) {
16 super();
17 this.brand = brand;
18 this.corp = corp;
19 this.price = price;
20 }
21
22 @Override
23 public String toString() {
24 return "Car [brand=" + brand + ", corp=" + corp + ", price=" + price + ", maxSpeed=" + maxSpeed + "]";
25 }
26
27 }
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd"> <context:component-scan base-package="com.tt.spring.beans"></context:component-scan>
<!--
配置bean
class:bean的全类名,通过反射的方式在IOC容器中创建Bean实例,所以要求Bean中必须有无参的构造器
id:标识容器中的bean,id唯一
-->
<bean id="helloWorld" class="com.tt.spring.beans.HelloWorld">
<property name="name" value="Spring"></property>
</bean> <!-- 通过构造方法来配置bean的属性 -->
<bean id="car" class="com.tt.spring.beans.Car">
<constructor-arg value="Audi"></constructor-arg>
<constructor-arg value="chengdu"></constructor-arg>
<constructor-arg value="30000"></constructor-arg>
</bean> </beans>
Main.java
控制台打印信息如下:
看到没?只能获取一个bean实例,不能获取两个。
原因分析:
在项目里,applicationContext.xml是在src最里面一层的,不在src的那一层,所以一旦运行Main.java文件,相应的就会在bin的目录下生成对应的applicationContext.xml文件。
但是我们又在bin文件夹里做过粘贴applicationContext.xml。
所以说applicationContext.xml的目录压根就是混乱的!!!既是处于根目录,又处于com最里面的目录。
要么在项目上将xml移到src那一层,那么bin底下的xml也对应着-------xml就处于根目录。
要么在项目上仍然位于com的最里面,那么bin那里就不要将xml移出来----xml就处于最里面的目录
至于xml文件究竟在哪个目录,就该用相应的读取方式。
ClassPathXmlApplicationContext和FileSystemXmlApplicationContext读取xml
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml")
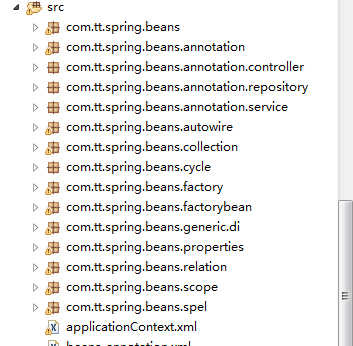
Spring报错:java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist的更多相关文章
- nested exception is java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be opened because it does not exist
org.apache.ibatis.exceptions.PersistenceException: ### Error building SqlSession. ### The error may ...
- Caused by: java.io.FileNotFoundException: class path resource [applicationContext.xml] cannot be ope
1.错误描述 java.lang.IllegalStateException: Failed to load ApplicationContext at org.springframework.tes ...
- parsing XML document from class path resource [applicationtext.xml]; nested exception is java.io.FileNotFoundException: class path resource [applicationtext.xml] cannot be opened because it does not e
控制台异常: parsing XML document from class path resource [applicationtext.xml]; nested exception is java ...
- nested exception is java.io.FileNotFoundException: class path resource [jdbc.properties] cannot be opened because it does not exist
Could not load properties; nested exception is java.io.FileNotFoundException: class path resource [j ...
- java.io.FileNotFoundException class path resource [xxx.xml] cannot be opened
没有找到xxx.xml,首先确定你项目里有这个文件吗,如果没有请添加,或者你已经存在配置文件,只是名字不是xxx.xml,请改正名字.此外还要注意最好把xxx.xml加入到classpath里,就是放 ...
- class path resource [applicationContext.xml] cannot be opened because it does not exis
使用maven创建web工程,将spring配置文件applicationContext.xml放在src/resource下,用eclipse编译时提示class path resource [ap ...
- nested exception is java.io.FileNotFoundException: class path resource [spring/spring-datasource-mog
spring单元測试时发现的问题: org.springframework.beans.factory.BeanDefinitionStoreException: IOException parsin ...
- 关于SpringMVC项目报错:java.io.FileNotFoundException: Could not open ServletContext resource [/WEB-INF/xxxx.xml]
关于SpringMVC项目报错:java.io.FileNotFoundException: Could not open ServletContext resource [/WEB-INF/xxxx ...
- Caused by: java.io.FileNotFoundException: class path resource [spring/springmvc.xml] cannot be opene
Caused by: java.io.FileNotFoundException: class path resource [spring/springmvc. ...
随机推荐
- php 输出 sql语句
第一种方法 $data = M('news')->field("title,date_format(postdate,'%Y-%m-%d') as postdate,content&q ...
- GRUB2 分析 (一)
GRUB是目前较流行启动引导程序.其第二版被主流Linux发行版所包括.本文将探索和分析GRUB的设计和实现机制. boot.S是第一个研究对象,因为boot.S将被编译成boot.img(512字节 ...
- zabbix分布式监控系统安装配置
zabbix简介: zabbix(音同 zæbix)是一个基于WEB界面的提供分布式系统监视以及网络监视功能的企业级的开源解决方案. zabbix能监视各种网络参数,保证服务器系统的安全运营:并提供灵 ...
- Metasploit 学习
知识准备:CCNA/CCNP基础计算机知识框架:操作系统.汇编.数据库.网络.安全 木马.灰鸽子.口令破解.用后门拷贝电脑文件 渗透测试工程师 penetration test engineer &l ...
- HA-web-services
一.HA部署 本次实验的程序选型为heartbeat v1 + hearesources.资源有IP和httpd,filesystem 配置HA集群的前提: (1)各节点资源一致,硬件或软件环境一致 ...
- 项目发布后 Tomcat中只有web-INF文件夹
这是有文件夹没有加载 解决办法如下
- AVL树 - 学习笔记
2017-08-29 14:35:55 writer:pprp AVL树就是带有平衡条件的二叉查找树.每个节点的左子树和右子树高度相差最多为1的二叉查找树 空树的高度定为-1 对树的修正称为旋转 对内 ...
- 在ajax请求下的缓存机制
1.在服务端加 header(“Cache-Control: no-cache, must-revalidate”);2.在ajax发送请求前加上 anyAjaxObj.setRequestHeade ...
- Python基础笔记系列九:变量、自定义函数以及局部变量和全局变量
本系列教程供个人学习笔记使用,如果您要浏览可能需要其它编程语言基础(如C语言),why?因为我写得烂啊,只有我自己看得懂!! 变量在前面的系列中也许就可以发现,python中的变量和C中的变量有些许不 ...
- 理解多线程管理类 CWorkQueue
有些人会觉得多线程无非是,有多少任务就启动多少线程,CreadThread,执行完了自己结束就释放资源了,其实不然.多线程是需要管理的,线程的启动.执行.等待和结束都需要管理,线程间如何通信,如何共享 ...