POJ 2177 Ghost Busters(三维几何)
Description
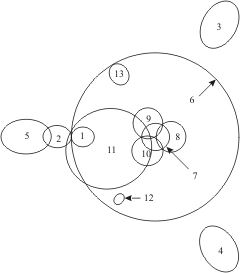
Ghosts are detected by ectoplasmic scanner and are represented as floating spheres. The coordinates of their centers and radii are delivered from the ectoplasmic scanner to the targeting software. The coordinate system is aligned is such a way, that the proton gun fires from the point (0, 0, 0) anywhere into X ≥ 0, Y ≥ 0, Z ≥ 0 trihedral angle. The gun fires a proton ray in a straight line and is so powerful, that even a touch of its ray is enough to kill a ghost. The ray of the proton gun is able to kill a virtually unlimited number of ghosts on its way.
For the first prototype for the targeting software, you are asked to write a program that determines the maximal number of ghosts that can be killed with a single shot of the proton gun.
Input
Output
#include <cstdio>
#include <algorithm>
#include <cstring>
#include <iostream>
#include <cmath>
using namespace std;
typedef long long LL; const double EPS = 1e-;
const double INF = 1e50;
const double PI = acos(-1.0); inline int sgn(double x) {
return (x > EPS) - (x < -EPS);
} inline double zero(double x) {
if(sgn(x) == ) return ;
else return x;
} inline double sqr(double x) {
return x * x;
} struct Point3D {
double x, y, z;
Point3D() {}
Point3D(double x, double y, double z): x(x), y(y), z(z) {}
void read() {
scanf("%lf%lf%lf", &x, &y, &z);
}
double operator * (const Point3D &rhs) const {
return x * rhs.x + y * rhs.y + z * rhs.z;
}
Point3D operator + (const Point3D &rhs) const {
return Point3D(x + rhs.x, y + rhs.y, z + rhs.z);
}
Point3D operator - (const Point3D &rhs) const {
return Point3D(x - rhs.x, y - rhs.y, z - rhs.z);
}
Point3D operator * (double rhs) const {
return Point3D(x * rhs, y * rhs, z * rhs);
}
Point3D operator / (double rhs) const {
return Point3D(x / rhs, y / rhs, z / rhs);
}
bool operator == (const Point3D &rhs) const {
return sgn(x - rhs.x) == && sgn(y - rhs.y) == && sgn(z - rhs.z) == ;
}
double length() const {
return sqrt(x * x + y * y + z * z);
}
Point3D unit() const {
return *this / length();
}
}; struct Line3D {
Point3D st, ed;
Line3D() {}
Line3D(Point3D st, Point3D ed): st(st), ed(ed) {}
}; struct Plane3D {
Point3D a, b, c;
Plane3D() {}
Plane3D(Point3D a, Point3D b, Point3D c): a(a), b(b), c(c) {}
void read() {
a.read(), b.read(), c.read();
}
}; struct Circle3D {
Point3D c;
double r;
Circle3D() {}
Circle3D(Point3D c, double r): c(c), r(r) {}
void read() {
c.read();
scanf("%lf", &r);
}
}; double dist(const Point3D &a, const Point3D &b) {
return (a - b).length();
}
//叉积
Point3D cross(const Point3D &u, const Point3D &v) {
Point3D ret;
ret.x = u.y * v.z - u.z * v.y;
ret.y = u.z * v.x - u.x * v.z;
ret.z = u.x * v.y - u.y * v.x;
return ret;
}
//点到直线距离
double point_to_line(const Point3D &p, const Line3D &l) {
return cross(p - l.st, l.ed - l.st).length() / dist(l.ed, l.st);
}
//求两直线间的距离
double line_to_line(const Line3D u, const Line3D v) {
Point3D n = cross(u.ed - u.st, v.ed - v.st);
return fabs((u.st - v.st) * n) / n.length();
}
//取平面法向量
Point3D vector_of_plane(const Plane3D &s) {
return cross(s.a - s.b, s.b - s.c);
}
//判断两直线是否平行
bool isParallel(const Line3D &u, const Line3D &v) {
return sgn(cross(u.ed - u.st, v.ed - v.st).length()) <= ;
}
//判断直线是否与球相交
bool isIntersect(const Line3D &l, const Circle3D &cir) {
return sgn(point_to_line(cir.c, l) - cir.r) <= ;
}
//直线与平面的交点
Point3D intersect(const Line3D &l, const Plane3D &s) {
Point3D ret = vector_of_plane(s);
double t = (ret * (s.a - l.st)) / (ret * (l.ed - l.st));
return l.st + (l.ed - l.st) * t;
}
//在原点上看,两个球的交点
int intersect(const Circle3D &u, const Circle3D &v, Point3D &p1, Point3D &p2) {
double d = dist(u.c, v.c);
if(u.c == v.c || sgn(d - u.r - v.r) > || sgn(fabs(u.r - v.r) - d) > ) return ;
double t = (sqr(d) + sqr(u.r) - sqr(v.r)) / ( * d);
Point3D mid = u.c + (v.c - u.c).unit() * t;
Point3D vec = cross(mid, v.c - u.c).unit() * sqrt(zero(sqr(u.r) - sqr(t)));
p1 = mid + vec;
p2 = mid - vec;
return + sgn(vec.length());
} const int MAXN = ; Circle3D cir[MAXN];
Point3D p[MAXN * MAXN], ansVec;
int maxAns, pcnt;
int n; int count(const Point3D &vec) {
int ret = ;
for(int i = ; i < n; ++i)
ret += (sgn(point_to_line(cir[i].c, Line3D(Point3D(, , ), vec)) - cir[i].r) <= );
return ret;
} void output(const Point3D &vec) {
bool flag = false;
for(int i = ; i < n; ++i) {
if(sgn(point_to_line(cir[i].c, Line3D(Point3D(, , ), vec)) - cir[i].r) <= ) {
if(flag) putchar(' ');
flag = true;
printf("%d", i + );
}
}
printf("\n");
} int main() {
scanf("%d", &n);
for(int i = ; i < n; ++i) cir[i].read();
for(int i = ; i < n; ++i) {
double t = / cir[i].c.length();
cir[i].c = cir[i].c * t;
cir[i].r = cir[i].r * t;
}
pcnt = ;
for(int i = ; i < n; ++i)
for(int j = i + ; j < n; ++j) pcnt += intersect(cir[i], cir[j], p[pcnt], p[pcnt + ]);
maxAns = ;
for(int i = ; i < n; ++i) {
int t = count(cir[i].c);
if(t > maxAns) {
maxAns = t;
ansVec = cir[i].c;
}
}
for(int i = ; i < pcnt; ++i) {
int t = count(p[i]);
if(t > maxAns) {
maxAns = t;
ansVec = p[i];
}
}
printf("%d\n", maxAns);
output(ansVec);
}
POJ 2177 Ghost Busters(三维几何)的更多相关文章
- POJ 2251 Dungeon Master --- 三维BFS(用BFS求最短路)
POJ 2251 题目大意: 给出一三维空间的地牢,要求求出由字符'S'到字符'E'的最短路径,移动方向可以是上,下,左,右,前,后,六个方向,每移动一次就耗费一分钟,要求输出最快的走出时间.不同L层 ...
- hdu 5839(三维几何)
Special Tetrahedron Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Othe ...
- POJ 2251 Dungeon Master (三维BFS)
题目链接:http://poj.org/problem?id=2251 Dungeon Master Time Limit: 1000MS Memory Limit: 65536K Total S ...
- poj 2031Building a Space Station(几何判断+Kruskal最小生成树)
/* 最小生成树 + 几何判断 Kruskal 球心之间的距离 - 两个球的半径 < 0 则说明是覆盖的!此时的距离按照0计算 */ #include<iostream> #incl ...
- poj 1185 炮兵阵地(三维状态压缩dP)
题目:http://poj.org/problem?id=1185 思路: d[i][j][k]表示第i行的状态为第k个状态,第i-1行的状态为第j个状态的时候 的炮的数量. 1表示放大炮, 地形状态 ...
- POJ 2252 Dungeon Master 三维水bfs
题目: http://poj.org/problem?id=2251 #include <stdio.h> #include <string.h> #include <q ...
- POJ 2653 Pick-up sticks(几何)
Pick-up sticks Time Limit: 3000MS Memory Limit: 65536K Total Submissions: 13377 Accepted: 5039 D ...
- POJ 3304 Segments --枚举,几何
题意: 给n条线段,问有没有一条直线,是每条线段到这条直线上的投影有一个公共点. 解法: 有公共点说明有一条这条直线的垂线过所有线段,要找一条直线过所有线段,等价于从所有线段中任选两端点形成的直线存在 ...
- POJ 2318 TOYS && POJ 2398 Toy Storage(几何)
2318 TOYS 2398 Toy Storage 题意 : 给你n块板的坐标,m个玩具的具体坐标,2318中板是有序的,而2398无序需要自己排序,2318要求输出的是每个区间内的玩具数,而231 ...
随机推荐
- 微信小程序新版用户授权方式处理
最新更新(2018-12-27): 最近做了改版,做成默认进来就是首页,然后去判断有没有用户信息,没有的话再去判断用没授权过,如果授权过直接自动去获取,没有的话再跳转到授权页面.因为用户授权主要就是针 ...
- CodeChef March Lunchtime 2018 div2
地址https://www.codechef.com/LTIME58B?order=desc&sortBy=successful_submissions 简单做了一下,前三题比较水,第四题应该 ...
- 创建在类路径资源[applicationcontext]中定义名为“工厂”的bean时出错。:在设置bean属性“dataSource”时,无法解析对bean“dataSource”的引用;嵌套异常是org.springframe .beans.factory。BeanCreationException:创建名为“数据源”的bean时出错,该名称是在类路径资源[applicationcontext
控制台报错: 创建在类路径资源[applicationcontext]中定义名为“工厂”的bean时出错.:在设置bean属性“dataSource”时,无法解析对bean“dataSource”的引 ...
- mysql学习记录,CASE WHEN THEN ELSE END用法
记mysql,case when then else end用法 用法1:搜索函数 SELECT r.order_no, r.golds, r.pay_tool, , ) ) END AS price ...
- 日常工作之Zabbix源码编译,兼容mysql5.6
原文链接:http://www.leleblog.top/daily/more?id=6 Zabbix源码编译 环境: centOS7.mysql5.6.21(已存在). 任务简述: 服务器搭建zab ...
- layui sleect获取value值
<div class="layui-form-item"> <label for="username" class="layui-f ...
- 帝国cms发布信息时替换正文IMG图片标签里的ALT内容
帝国cms发布信息时替换正文IMG图片标签里的ALT内容 在 e/class/userfun.php 里面增加 //替换正文IMG里的ALT内容 function user_imgalt($mid,$ ...
- 20190112-自定义实现字符串的操作方法,如strip,upper,title,ljust,center,zfill,find,rfind等
1:自定义实现strip()Python strip() 方法用于移除字符串头尾指定的字符(默认为空格或换行符)或字符序列算法:strip()仅移除首尾的指定字符,不能移除中间的先从首部开始移除 de ...
- 使用bison和yacc制作脚本语言(2)
我们先来想一下语法 一般脚本语言不需要定义类型直接在赋值的时候确定 我们主要考虑一下变量的类型 a = 1; b = 1.1; c = "str"; 一般来讲,我们使用这三种类型, ...
- C# 截取屏幕图像
#region 截取屏幕图像 private static Bitmap GetScreenCapture() { Rectangle tScreenRect = , , Screen.Primary ...