The Stereo Action Dimension
Network MIDI on iOS - Part 1
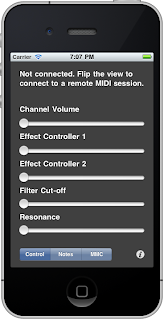
- Composing outgoing MIDI data in response to user input
- Processing incoming MIDI data in real time (with semaphores and lock free buffers)
- Finding network services with Bonjour
Please note you need to run this on a device rather than the simulator.
(Now seems to work OK on the iOS 7 simulator at least).
Network MIDI on iOS - Part 2
In this part, I will discuss finding and publishing network MIDI services using Bonjour, and creating the MIDI client using the CoreMIDI API. The source code for this project is available for download in
Part 1 of this article.
The network MIDI services in OS X and iOS can be discovered in the same way as any other Bonjour service. In the initialiser of the MIDIController class (see MIDIController.m) an instance of
NSNetServiceBrowser is created, and instructed to search for services of the type MIDINetworkBonjourServiceType i.e. @"_apple-midi._udp". By implementing the delegate protocol for this browser, a MIDIController instance can monitor available services of
this type on the network.
These services are exposed to the remainder of the application as a dictionary. In addition, various operations are provided to allow connection, disconnection etc. (see the section marked "Connection Management" in the implementation for details). The sample
application uses these methods to provide a simple UI to connect to any detected services. The settings page is displayed by flipping the main view. From here, we can select one or more of the remote MIDI services available on the current LAN.
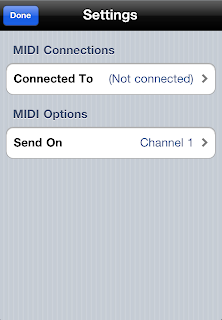
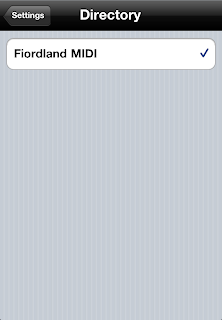
Note that connections can also be made from the other end. By opening Audio MIDI Setup on a Mac on the same LAN, we can browse to the iPhone and initiate a connection from the computer. In this instance, the MIDIController instance will inform the UI that a
connection has been made via an NSNotification. If multiple connections are made to or from the same device, they are bridged in that:
- All incoming data from the network is merged as if it was coming from a single device
- Outgoing data from the device is sent to all network services
The MIDIController initialiser also sets up the shared MIDINetworkSession instance, which acts as a bridge between the network services and the CoreMIDI API. A MIDI client, input port and output port are then created. The input port is connected to the source endpoint of the MIDINetworkSession instance. Note
that these endpoints are named from the perspective of the iOS application; the "source" endpoint is where data will be received from the network, and the "destination" endpoint is where the application will send data to the network.
To send data to the output port once a connection has been made, the app invokes the methods in the section marked Sending in the implementation file, namely:
-(void) allNotesOffOnChannel:(NSUInteger)channel;
-(void) sendChangeForController:(NSUInteger)controller onChannel:(NSUInteger)channel withValue:(NSUInteger)value;
-(void) sendNote:(NSUInteger)note on:(BOOL)on onChannel:(NSUInteger)channel withVelocity:(NSUInteger)velocity;
-(void) sendMMCCommand:(NSUInteger)command toDevice:(NSUInteger)device;
data (for instance where the packet count is set too high) will typically result in disconnection by the remote service.
The sendNote:on:onChannel:withVelocity: method is invoked when the user presses the "keys" on the Notes tab:
The sendMMCCommand:toDevice: method is invoked in response to button presses on the MMC tab:
Please note that the MMC command output hasn't been tested as I don't have any devices that respond to this part of the MIDI protocol. Let me know via the comments if you have any problems.
The operation of the input port is described in Part 3 of this article.
Network MIDI on iOS - Part 3
In this part I will discuss how incoming MIDI is received into the application and subsequently processed. The source code for this project is available for download in
Part 1 of this article.
The MIDI controller class (see MIDIController.h) provides a formal protocol, MIDIReceivedDelegate, and a corresponding delegate property. This defines the following methods:
- (void) midiControllerUpdated:(Byte)controller onChannel:(Byte)channel toValue:(Byte)value;
- (void) midiNoteOnOff:(Byte)note onChannel:(Byte)channel withVelocity:(Byte)velocity on:(BOOL)on;
These methods encapsulate the complexity of receiving MIDI data - but how is this accomplished behind the scenes?
When we created the MIDI client in part 2 of this article, we also created a MIDI input port. This was passed a pointer to a callback function, MIDIInputReadProc. This callback function is called by the operating system when MIDI data is received at the port.
As a real time callback function which may be re-entrant when the system is under load, certain restrictions should be adhered to. In particular, this function should avoid:
- Allocating and deallocating memory
- Acquiring locks
- Performing lengthy operations
The function walks the list of MIDI packets received as follows:
For each MIDI packet received:
- The packet's length and data are written into a structured circular buffer (see MIDIPacketBuffer.h)
- A Mach semaphore is incremented to signal the rest of the application that another packet is available for processing.
Note that the structured buffer in this example disregards the timestamp of the MIDI packet. This will be covered in a later example.
Another thread (see midiInputThreadProc) waits on this semaphore. If the semaphore is signalled, the length of the next MIDI packet is retrieved from the circular buffer, and then that amount of data is copied into a regular buffer.
This data is then parsed from this buffer. The following MIDI commands are identified in this example:
- Control changes
- Note on/off
By these means, the application decouples the processing of MIDI data from its receipt, and conforms with the requirements placed on it by the CoreMIDI port model
The Stereo Action Dimension的更多相关文章
- 强化学习_PolicyGradient(策略梯度)_代码解析
使用策略梯度解决离散action space问题. 一.导入包,定义hyper parameter import gym import tensorflow as tf import numpy as ...
- Java基础之处理事件——使用动作Action(Sketcher 6 using Action objects)
控制台程序. 动作Action是任何实现了javax.swing.Action接口的类的对象.这个接口声明了操作Action对象的方法,例如,存储与动作相关的属性.启用和禁用动作.Action接口扩展 ...
- 微软BI 之SSIS 系列 - 数据仓库中实现 Slowly Changing Dimension 缓慢渐变维度的三种方式
开篇介绍 关于 Slowly Changing Dimension 缓慢渐变维度的理论概念请参看 数据仓库系列 - 缓慢渐变维度 (Slowly Changing Dimension) 常见的三种类型 ...
- 【论文笔记】Spatial Temporal Graph Convolutional Networks for Skeleton-Based Action Recognition
Spatial Temporal Graph Convolutional Networks for Skeleton-Based Action Recognition 2018-01-28 15:4 ...
- [libGDX游戏开发教程]使用libGDX进行游戏开发(12)-Action动画
前文章节列表: 使用libGDX进行游戏开发(11)-高级编程技巧 使用libGDX进行游戏开发(10)-音乐音效不求人,程序员也可以DIY 使用libGDX进行游戏开发(9)-场景过渡 ...
- redux-amrc:用更少的代码发起异步 action
很多人说 Redux 代码多,开发效率低.其实 Redux 是可以灵活使用以及拓展的,经过充分定制的 Redux 其实写不了几行代码.今天先介绍一个很好用的 Redux 拓展-- redux-amrc ...
- 尝试asp.net mvc 基于controller action 方式权限控制方案可行性
微软在推出mvc框架不久,短短几年里,版本更新之快,真是大快人心,微软在这种优秀的框架上做了大量的精力投入,是值得赞同的,毕竟程序员驾驭在这种框架上,能够强力的精化代码,代码层次也更加优雅,扩展较为方 ...
- ASP.NET Core 中文文档 第四章 MVC(4.1)Controllers, Actions 和 Action Results
原文:Controllers, Actions, and Action Results 作者:Steve Smith 翻译:姚阿勇(Dr.Yao) 校对:许登洋(Seay) Action 和 acti ...
- java中Action层、Service层和Dao层的功能区分
Action/Service/DAO简介: Action是管理业务(Service)调度和管理跳转的. Service是管理具体的功能的. Action只负责管理,而Service负责实施. DAO只 ...
随机推荐
- Android HTTPS(5)SSL测试工具
Nogotofail: A Network Traffic Security Testing Tool Nogotofail is a tool gives you an easy way to co ...
- java生成随机序列号
1.java生成随机序列号 String deleteUuid = UUID.randomUUID().toString(); 引用Jar包 //java-uuid-generator-3.1.3.j ...
- Intellij IDEA13 创建多模块Maven项目
目标:构建一个类似于如下图所示的这种结构的Maven项目. 首先,需要选中“File”——>“New Project”如下图所示 选中“Maven”,设置项目名称与项目构建地址,点击“Next” ...
- BZOJ 3406 乳草的入侵
BFS. #include<iostream> #include<cstdio> #include<cstring> #include<algorithm&g ...
- [转] gc tips(1)
所有应用软件都需要管理内存,一个应用软件的内存管理系统包括了如下准则:什么时候派发内存,要派发多少内存,什么时候把东西放到回收站,以及什么时候清空回收站.MMgc是Flash Player几乎所有内存 ...
- Task和BackTask
一.总结性知识点: 1.Android应用运行时会创建任务Task,用于存放主窗口 2.每一个任务包含一个堆栈数据结构,用于保存当前应用已创建的窗口对象,这个堆栈即回退栈BackSta ...
- linux apache 配置fastcgi
Redhat 上 FastCGI 安装与配置 软件包 相关软件包: httpd 2.2.14 //注意版本 这个版本不会出问题 注:apache httpd安装 fcgi-2.4.0.t ...
- Java文件下载的几种方式
public HttpServletResponse download(String path, HttpServletResponse response) { try { // path是指欲下载的 ...
- codeforces 340B Maximal Area Quadrilateral(叉积)
事实再一次证明:本小菜在计算几何上就是个渣= = 题意:平面上n个点(n<=300),问任意四个点组成的四边形(保证四条边不相交)的最大面积是多少. 分析: 1.第一思路是枚举四个点,以O(n4 ...
- file的getPath getAbsolutePath和getCanonicalPath的不同
file的这几个取得path的方法各有不同,下边说说详细的区别 概念上的区别:(内容来自jdk,个人感觉这个描述信息,只能让明白的人明白,不明白的人看起来还是有点难度(特别试中文版,英文版稍好些)所以 ...