HDU-1506 Largest Rectangle in a Histogram【单调栈】
Description
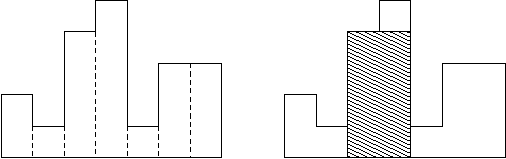
Usually, histograms are used to represent discrete distributions,
e.g., the frequencies of characters in texts. Note that the order of the
rectangles, i.e., their heights, is important. Calculate the area of
the largest rectangle in a histogram that is aligned at the common base
line, too. The figure on the right shows the largest aligned rectangle
for the depicted histogram.
Input
Output
each test case output on a single line the area of the largest
rectangle in the specified histogram. Remember that this rectangle must
be aligned at the common base line.
Sample Input
7 2 1 4 5 1 3 3
4 1000 1000 1000 1000
0
Sample Output
8
4000
Hint
Source
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
#include <deque> using namespace std;
typedef __int64 LL;
const int maxn=1e5+7; LL maxx; struct node{
LL len;
LL h;
}a[maxn]; int main()
{
LL n;
while(~scanf("%I64d",&n)&&n){
for(LL i=0;i<n;i++)
{
scanf("%I64d",&a[i].h);
a[i].len=1;
}
deque <node> q;
q.push_back(a[0]);
maxx=0;
for(LL i=1;i<n;i++)
{
if(a[i].h>=a[i-1].h)
{
q.push_back(a[i]);
}
else
{
LL totlen=0;
LL ae=0;
while(!q.empty()&&a[i].h<q.back().h)
{
totlen+=q.back().len;
ae=totlen*q.back().h;
maxx=max(maxx,ae);
q.pop_back();
}
totlen+=a[i].len;
a[i].len=totlen;
q.push_back(a[i]);
}
} LL totlen=0,ae=0; while(!q.empty())
{
totlen+=q.back().len;
ae=totlen*q.back().h;
maxx=max(maxx,ae);
q.pop_back();
}
printf("%I64d\n",maxx);
}
return 0;
}
HDU-1506 Largest Rectangle in a Histogram【单调栈】的更多相关文章
- hdu 1506 Largest Rectangle in a Histogram(单调栈)
L ...
- HDU - 1506 Largest Rectangle in a Histogram (单调栈/笛卡尔树)
题意:求一个直方图中最大矩形的面积. 很经典的一道问题了吧,可以用单调栈分别求出每个柱子左右两边第一个比它低的柱子(也就相当于求出了和它相连的最后一个比它高的柱子),确定每个柱子的左右边界,每个柱子的 ...
- HDU 1506 Largest Rectangle in a Histogram (dp左右处理边界的矩形问题)
E - Largest Rectangle in a Histogram Time Limit:1000MS Memory Limit:32768KB 64bit IO Format: ...
- HDU 1506 Largest Rectangle in a Histogram set+二分
Largest Rectangle in a Histogram Problem Description: A histogram is a polygon composed of a sequenc ...
- hdu 1506 Largest Rectangle in a Histogram 构造
题目链接:HDU - 1506 A histogram is a polygon composed of a sequence of rectangles aligned at a common ba ...
- HDU 1506 Largest Rectangle in a Histogram(区间DP)
题目网址:http://acm.hdu.edu.cn/showproblem.php?pid=1506 题目: Largest Rectangle in a Histogram Time Limit: ...
- DP专题训练之HDU 1506 Largest Rectangle in a Histogram
Description A histogram is a polygon composed of a sequence of rectangles aligned at a common base l ...
- Hdu 1506 Largest Rectangle in a Histogram 分类: Brush Mode 2014-10-28 19:16 93人阅读 评论(0) 收藏
Largest Rectangle in a Histogram Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 ...
- HDU 1506 Largest Rectangle in a Histogram(DP)
Largest Rectangle in a Histogram Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 ...
- poj 2559 Largest Rectangle in a Histogram - 单调栈
Largest Rectangle in a Histogram Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 19782 ...
随机推荐
- 用pycharm自带的数据库创建项目00
一.生成表格1.创建模型类(在 models.py文件中创建一个person类并且继承models.Models类) 2.生成表格(在项目目录下)(1)生成迁移文件:在pycharm下方的命令行Ter ...
- Git push时不需要总输入密码
遇到问题: 最近因为换了自己的邮箱密码后,每次push的时候都需要填写密码,账号.很烦 解决方法: [戎马半生的答案] (http://www.cnblogs.com/zhaoyu1995/p/650 ...
- Python3标准库:itertools迭代器函数
1. itertools迭代器函数 itertools包括一组用于处理序列数据集的函数.这个模块提供的函数是受函数式编程语言(如Clojure.Haskell.APL和SML)中类似特性的启发.其目的 ...
- JavaScript 基础学习(二)js 和 html 的结合方式
第一种 使用一个标签 <script type="text/javascript"> js代码; </script> 第二种 使用 script 标签,引入 ...
- ViewPager调用notifyDataSetChanged() 刷新问题解决方案
一.问题来由 ViewPager控件很大程度上满足了开发者开发页面左右移动切换的功能,使用非常方便.但是使用中发现,在删除或者修改数据的时候,PagerAdapter无法像BaseAdapter那样仅 ...
- android sdk manager 无法更新,解决连不上dl.google.com的问题
感谢博主的帮助,入口在这:https://www.jianshu.com/p/8fb367a51b9f?utm_campaign=haruki&utm_content=note&utm ...
- Linux部署MongoDB
下载安装包 打开网站 https://www.mongodb.com/download-center/community查找与Linux版本一致的MongoDB安装包.我这里选择安装包格式为tgz压缩 ...
- windows快捷键记录
-1: 装完iis, run -> inetmgr 弹出iis管理器 0.按住Shift键右击鼠标打开命令行窗口 1.ODBC数据源管理器run->odbcad32 2.计算机管理(查看设 ...
- Python——面向对象,简易学生信息管理系统
一.概述 1.1涉及到的知识点 项目开发:创建项目,创建包,导入包: 面向对象:静态方法,继承,内置函数,自定义函数: 数据类型:列表,字典,字符串.列表.字典的转换和自动生成导向: 异常处理:捕获异 ...
- 《手把手教你构建自己的 Linux 系统》学习笔记(4)
汇编链接器(Binutils) 这是一个软件包,这个软件包其实是一个工具集,里面含有了大量的用于汇编程序活着读取二进制文件相关的程序. CC 它是一条命令的别名,这条命令的作用是使用 GCC 的 C ...