POJ 1955 Rubik's Cube
暴力模拟就好了。。。。
vim写代码真费事,手都写酸了。。。
Time Limit: 1000MS | Memory Limit: 30000K | |
Total Submissions: 638 | Accepted: 324 |
Description
Rummaging through the stuff of your childhood you find an old toy which you identify as the famous Rubik's Cube. While playing around with it you have to acknowledge that throughout the years your ability to solve the puzzle has not improved a bit. But because you always wanted to understand the thing and the only other thing you could do right now is to prepare for an exam, you decide to give it a try. Luckily the brother of your girlfriend is an expert and able to fix the cube no matter how messed-up it is. The problem is that he stays with his girlfriend in the Netherlands most of the time, so you need a solution for long-distance learning. You decide to implement a program which is able to document the state of the cube and the turns to be made.
Problem
A Rubik's Cube is covered with 54 square areas called facelets, 9 facelets on each of its six sides. Each facelet has a certain color. Usually when the cube is in its starting state, all facelets belonging to one side have the same color. For the original cube these are red, yellow, green, blue, white and orange.
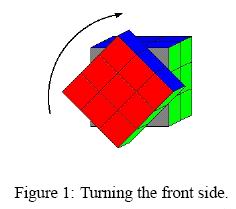
The positions of the facelets can be changed by turning the sides of the cube. This moves nine "little cubes" together with their attached facelets into a new position (see Fig. 1).
The problem is to determine how the facelets of the entire cube are colored after turning different sides in different directions.
Input
The starting state describes the colors of the facelets and where they are positioned. The colors are identified by single characters, and one character is given per facelet. Characters are separated by blanks and arranged in a certain pattern (see Fig. 2). The pattern identifies all six sides of the cube and can be thought of as a folding pattern. As shown in Fig. 2, the description of the top side of the cube is placed right over the description of the front side. This is done by indenting the lines with blanks. The next three lines contain the descriptions of the left, front, right and back side as shown in Fig. 2. The descriptions are simply concatenated with a blank character used as separator. After that the description of the bottom side follows, using the same format as the one used to describe the top side. This concludes the description of the starting state.
Then follows the second section of the scenario containing the turns which have to be performed. The description of the turns starts with a line containing the number of turns t (t > 0). Each turn is given in a separate line and consists of two integer values s and d which are separated by a single blank. The first value s determines the side of the cube which has to be turned. The sides are serially numbered as follows:left '0', front '1', right '2', back '3', top '4', bottom '5'. The second value d determines in which direction
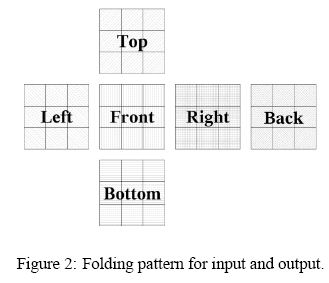
the side s has to be turned and can either be '1' or '-1'. A '1' stands for clockwise and a '-1' for counterclockwise.The direction is given under the assumption that the viewer is looking directly at the specific side of the cube.
Output
Sample Input
2
w w w
w w w
w w w
r r r g g g b b b o o o
r r r g g g b b b o o o
r r r g g g b b b o o o
y y y
y y y
y y y
2
3 1
0 -1
g b b
g w w
g w w
r r r y g g b b y o o w
r r r y g g b b y o o w
w w w r g g b b y o o b
o y y
o y y
o r r
2
0 1
3 -1
Sample Output
Scenario #1:
g b b
g w w
g w w
r r r y g g b b y o o w
r r r y g g b b y o o w
w w w r g g b b y o o b
o y y
o y y
o r r Scenario #2:
w w w
w w w
w w w
r r r g g g b b b o o o
r r r g g g b b b o o o
r r r g g g b b b o o o
y y y
y y y
y y y
Source
#include <iostream>
#include <cstdio>
#include <cstring> using namespace std; int mofang[60]; void SHOW()
{
int cnt=1;printf(" ");
for(int i=0;i<9;i++,cnt++)
{
printf("%c ",mofang[i]);
if(cnt%3==0)
{
if(i!=0) putchar(10);
if(i!=8) printf(" ");
}
}
cnt=1;
for(int i=9;i<45;i++,cnt++)
{
printf("%c ",mofang[i]);
if(cnt%12==0)
putchar(10);
}
cnt=1; printf(" ");
for(int i=45;i<54;i++,cnt++)
{
printf("%c ",mofang[i]);
if(cnt%3==0)
{
putchar(10);
if(i!=53) printf(" ");
}
}
putchar(10);
} void quanCLOCKwise(int a1,int a2,int a3,int a4,int a5,int a6,int a7,int a8,int a9)
{
int a,b,c;
a=mofang[a1]; b=mofang[a2]; c=mofang[a3];
mofang[a1]=mofang[a7]; mofang[a2]=mofang[a4]; mofang[a3]=a;
mofang[a7]=mofang[a9]; mofang[a4]=mofang[a8]; mofang[a8]=mofang[a6];
mofang[a6]=b; mofang[a9]=c;
} void quanFANCLOCKwise(int a1,int a2,int a3,int a4,int a5,int a6,int a7,int a8,int a9)
{
int a=mofang[a1],b=mofang[a2],c=mofang[a3];
mofang[a1]=c; mofang[a2]=mofang[a6]; mofang[a3]=mofang[a9];
mofang[a6]=mofang[a8];mofang[a9]=mofang[a7];
mofang[a7]=a; mofang[a8]=mofang[a4];mofang[a4]=b;
} void huanCLOCKwise(int a1,int a2,int a3,int a4,int a5,int a6,int a7,int a8,int a9,int a10,int a11,int a12)
{
int a=mofang[a1],b=mofang[a2],c=mofang[a3];
mofang[a1]=mofang[a10]; mofang[a2]=mofang[a11];mofang[a3]=mofang[a12];
mofang[a10]=mofang[a7];mofang[a11]=mofang[a8];mofang[a12]=mofang[a9];
mofang[a9]=mofang[a6];mofang[a8]=mofang[a5];mofang[a7]=mofang[a4];
mofang[a6]=c; mofang[a5]=b; mofang[a4]=a;
} void huanFANCLOCKwise (int a1,int a2,int a3,int a4,int a5,int a6,int a7,int a8,int a9,int a10,int a11,int a12)
{
int a=mofang[a1],b=mofang[a2],c=mofang[a3];
mofang[a1]=mofang[a4]; mofang[a2]=mofang[a5]; mofang[a3]=mofang[a6];
mofang[a4]=mofang[a7]; mofang[a5]=mofang[a8]; mofang[a6]=mofang[a9];
mofang[a9]=mofang[a12]; mofang[a8]=mofang[a11]; mofang[a7]=mofang[a10];
mofang[a10]=a; mofang[a11]=b; mofang[a12]=c;
} void QianClockwise()
{
quanCLOCKwise(12,13,14,24,25,26,36,37,38);
huanCLOCKwise(6,7,8,15,27,39,47,46,45,35,23,11);
} void QianFanClockwise()
{
quanFANCLOCKwise(12,13,14,24,25,26,36,37,38);
huanFANCLOCKwise(6,7,8,15,27,39,47,46,45,35,23,11);
} void BackClockwise()
{
quanCLOCKwise(18,19,20,30,31,32,42,43,44);
huanCLOCKwise(2,1,0,9,21,33,51,52,53,41,29,17);
} void BackFanClockwise()
{
quanFANCLOCKwise(18,19,20,30,31,32,42,43,44);
huanFANCLOCKwise(2,1,0,9,21,33,51,52,53,41,29,17);
} void LeftClockwise()
{
quanCLOCKwise(9,10,11,21,22,23,33,34,35);
huanCLOCKwise(0,3,6,12,24,36,45,48,51,44,32,20);
} void LeftFanClockwise()
{
quanFANCLOCKwise(9,10,11,21,22,23,33,34,35);
huanFANCLOCKwise(0,3,6,12,24,36,45,48,51,44,32,20);
} void RightClockwise()
{
quanCLOCKwise(15,16,17,27,28,29,39,40,41);
huanCLOCKwise(8,5,2,18,30,42,53,50,47,38,26,14);
} void RightFanClockwise()
{
quanFANCLOCKwise(15,16,17,27,28,29,39,40,41);
huanFANCLOCKwise(8,5,2,18,30,42,53,50,47,38,26,14);
} void TopClockwise()
{
quanCLOCKwise(0,1,2,3,4,5,6,7,8);
huanCLOCKwise(20,19,18,17,16,15,14,13,12,11,10,9);
} void TopFanClockwise()
{
quanFANCLOCKwise(0,1,2,3,4,5,6,7,8);
huanFANCLOCKwise(20,19,18,17,16,15,14,13,12,11,10,9);
} void BotClockwise()
{
quanCLOCKwise(45,46,47,48,49,50,51,52,53);
huanCLOCKwise(36,37,38,39,40,41,42,43,44,33,34,35);
} void BotFanClockwise()
{
quanFANCLOCKwise(45,46,47,48,49,50,51,52,53);
huanFANCLOCKwise(36,37,38,39,40,41,42,43,44,33,34,35);
} int main()
{
int t,cas=1;
scanf("%d",&t);
while(t--)
{
printf("Scenario #%d:\n",cas++);
for(int i=0;i<54;i++)
{
char c[3];
scanf("%s",c);
mofang[i]=(int)c[0];
}
int m;
scanf("%d",&m);
while(m--)
{
int a,b;
scanf("%d%d",&a,&b);
if(a==0)
{
if(b==1) LeftClockwise();
else LeftFanClockwise();
}
else if(a==1)
{
if(b==1) QianClockwise();
else QianFanClockwise();
}
else if(a==2)
{
if(b==1) RightClockwise();
else RightFanClockwise();
}
else if(a==3)
{
if(b==1) BackClockwise();
else BackFanClockwise();
}
else if(a==4)
{
if(b==1) TopClockwise();
else TopFanClockwise();
}
else if(a==5)
{
if(b==1) BotClockwise();
else BotFanClockwise();
}
}
SHOW();
}
return 0;
}
POJ 1955 Rubik's Cube的更多相关文章
- sdutoj 2606 Rubik’s cube
http://acm.sdut.edu.cn/sdutoj/problem.php?action=showproblem&problemid=2606 Rubik’s cube Time Li ...
- The Mathematics of the Rubik’s Cube
https://web.mit.edu/sp.268/www/rubik.pdf Introduction to Group Theory and Permutation Puzzles March ...
- HDU 5836 Rubik's Cube BFS
Rubik's Cube 题目连接: http://acm.hdu.edu.cn/showproblem.php?pid=5836 Description As we all know, Zhu is ...
- hduoj 3459 Rubik 2×2×2
http://acm.hdu.edu.cn/showproblem.php?pid=3459 Rubik 2×2×2 Time Limit: 10000/5000 MS (Java/Others) ...
- squee_spoon and his Cube VI(贪心,找不含一组字符串的最大长度+kmp)
1818: squee_spoon and his Cube VI Time Limit: 1 Sec Memory Limit: 128 MB Submit: 77 Solved: 22Subm ...
- HDU5983Pocket Cube
Pocket Cube Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others) Tota ...
- Pocket Cube
Pocket Cube http://acm.hdu.edu.cn/showproblem.php?pid=5983 Time Limit: 2000/1000 MS (Java/Others) ...
- HDU 5292 Pocket Cube 结论题
Pocket Cube 题目连接: http://acm.hdu.edu.cn/showproblem.php?pid=5292 Description Pocket Cube is the 2×2× ...
- squee_spoon and his Cube VI---郑大校赛(求最长子串)
市面上最常见的魔方,是三阶魔方,英文名为Rubik's Cube,以魔方的发明者鲁比克教授的名字命名.另外,二阶魔方叫Pocket Cube,它只有2*2*2个角块,通常也就比较小:四阶魔方叫Reve ...
随机推荐
- BZOJ 2843: 极地旅行社( LCT )
LCT.. ------------------------------------------------------------------------ #include<cstdio> ...
- C++ cout 如何保留小数输出
参考 : http://upliu.net/how-cout-out-2-precision.html 大家都知道用 C 语言中 printf () 函数可以非常方便控制保留 几位小数输出 不过在 C ...
- HDU 5119 Happy Matt Friends(2014北京区域赛现场赛H题 裸背包DP)
虽然是一道还是算简单的DP,甚至不用滚动数组也能AC,数据量不算很大. 对于N个数,每个数只存在两个状态,取 和 不取. 容易得出状态转移方程: dp[i][j] = dp[i - 1][j ^ a[ ...
- Qt 5.x 全局热键 for windows
Qt 升级到5.x版本后,QAbstractEventDispatcher中函数发生变动,导致libqxt库中的qxtGlobalShortcut挂掉.参考qxtGlobalShortcut写了一个全 ...
- nginx启动过程分析
nginx的启动过程紧紧环绕着ngx_cycle_t的结构体展开,首先通过ngx_get_options()获取命令行參数.然后通过ngx_time_init()进行时间的初始化.如全局变量ngx_c ...
- xp每天定时关机命令
at 00:00 /every:M,T,W,Th,F,S,Su shutdown -s -t 120 能够把00:00改成你想要每天定时关机的时间,120是指关机倒计时的秒数,也能够更改 M,T,W, ...
- Servlet 实现文件的上传与下载
这段时间尝试写了一个小web项目,其中涉及到文件上传与下载,虽然网上有很多成熟的框架供使用,但为了学习我还是选择了自己编写相关的代码.当中遇到了很多问题,所以在此这分享完整的上传与下载代码供大家借鉴. ...
- Inter IPP的一些基本类型对应的vs中类型
来自为知笔记(Wiz)
- c#--foreach遍历的用法与split的用法
一. foreach循环用于列举出集合中所有的元素,foreach语句中的表达式由关键字in隔开的两个项组成.in右边的项是集合名,in左边的项是变量名,用来存放该集合中的每个元素. 该循环 ...
- 【转】java--final
1.final数据 许多程序设计语言都有自己的办法告诉编译器某个数据是“常数”.常数主要应用于下述两个方面: (1) 编译期常数,它永远不会改变 (2) 在运行期初始化的一个值,我们不希望它发生变化 ...