Knight Moves(广搜BFS)
Description
Of course you know that it is vice versa. So you offer him to write a program that solves the "difficult" part.
Your job is to write a program that takes two squares a and b as input and then determines the number of knight moves on a shortest route from a to b.
Input
Output
Sample Input
Sample Output
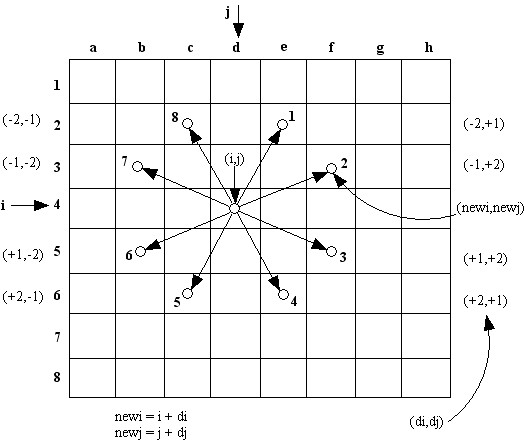
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std;
char s1[],s2[];
struct node
{
int x;
int y;
int step;
};
int vis[][];
int to[][]= {{-,},{-,},{,},{,},{,-},{,-},{-,-},{-,-}};
int ex,ey;
int judge(int x,int y)
{
if(x>=&&y>=&&x<&&y<&&!vis[x][y])
{
return ;
}
else
{
return ;
}
}
int BFS()
{
int i;
queue<node>q;
node p,a;
p.x=s1[]-'a';
p.y=s1[]-'';
p.step=;
ex=s2[]-'a';
ey=s2[]-'';
vis[p.x][p.y]=;
q.push(p);
while(!q.empty())
{
a=q.front();
if(a.x==ex&&a.y==ey)
{
return a.step;
}
for(i=;i<;i++)
{
node b;
b=a;
b.x+=to[i][];
b.y+=to[i][];
if(judge(b.x,b.y))
{
b.step++;
vis[b.x][b.y]=;
q.push(b);
}
}
q.pop();
}
}
int main()
{
while(scanf("%s%s",s1,s2)!=EOF)
{
printf("To get from %s to %s takes %d knight moves.\n",s1,s2,BFS());
memset(vis,,sizeof(vis));
memset(s1,sizeof(s1),);
memset(s2,sizeof(s2),);
}
return ;
}
Knight Moves(广搜BFS)的更多相关文章
- UVA 439 Knight Moves --DFS or BFS
简单搜索,我这里用的是dfs,由于棋盘只有8x8这么大,于是想到dfs应该可以过,后来由于边界的问题,TLE了,改了边界才AC. 这道题的收获就是知道了有些时候dfs没有特定的边界的时候要自己设置一个 ...
- poj3126 Prime Path 广搜bfs
题目: The ministers of the cabinet were quite upset by the message from the Chief of Security stating ...
- hdu 1253:胜利大逃亡(基础广搜BFS)
胜利大逃亡 Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submi ...
- hdu 2717 Catch That Cow(广搜bfs)
题目链接:http://i.cnblogs.com/EditPosts.aspx?opt=1 Catch That Cow Time Limit: 5000/2000 MS (Java/Others) ...
- 算法学习笔记(六) 二叉树和图遍历—深搜 DFS 与广搜 BFS
图的深搜与广搜 复习下二叉树.图的深搜与广搜. 从图的遍历说起.图的遍历方法有两种:深度优先遍历(Depth First Search), 广度优先遍历(Breadth First Search),其 ...
- luoguP4112 [HEOI2015]最短不公共子串 SAM,序列自动机,广搜BFS
luoguP4112 [HEOI2015]最短不公共子串 链接 luogu loj 思路 子串可以用后缀自动机,子序列可以用序列自动机. 序列自动机是啥,就是能访问到所有子序列的自动机. 每个点记录下 ...
- Knight Moves (双向bfs)
# 10028. 「一本通 1.4 例 3」Knight Moves [题目描述] 编写一个程序,计算一个骑士从棋盘上的一个格子到另一个格子所需的最小步数.骑士一步可以移动到的位置由下图给出. [算法 ...
- POJ 3126 Prime Path 简单广搜(BFS)
题意:一个四位数的质数,每次只能变换一个数字,而且变换后的数也要为质数.给出两个四位数的质数,输出第一个数变换为第二个数的最少步骤. 利用广搜就能很快解决问题了.还有一个要注意的地方,千位要大于0.例 ...
- HDU 5336——XYZ and Drops——————【广搜BFS】
XYZ and Drops Time Limit: 3000/1500 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others)Tot ...
随机推荐
- javascript 中x++和++x的不同
x++和++x都是给x加一,但是前者是完成赋值之后再递增x,后者相反. 例如:如果x是5,y=x++会将y设置为5,x设置为6:而y=++x会将x和y都设置为6.
- LVS负载均衡机制之LVS-DR模式工作原理以及简单配置
本博文主要简单介绍一下LVS负载均衡集群的一个基本负载均衡机制:LVS-DR:如有汇总不当之处,请各位在评论中多多指出. LVS-DR原理: LVS的英文全称是Linux Virtual Server ...
- echarts通过ajax动态获取数据的方法
echarts表格的数据一般都需要动态获取,所以总结了一下通过ajax动态获取数据的操作: 插入的方法应该不止一种,我也是接触不久,所以刚学会了一种插入方法: 灵感和经验来自:https://www. ...
- MongoDB的高级使用
MongoDB的高级使用 1. Mongdb的索引备份以及和python交互 t255为mongodb中的集合 1.1 创建索引 索引的特点:提高查找的效率 不创建索引的情况下的查询: for(i=0 ...
- 有限差分法解矩形波导内场值、截止频率 MATLAB
利用有限差分法,解矩形波导内场解和截止频率: 这里以解TM11模为例,利用双重迭代法,每4次场值,更新一次Kc: %% % 求矩形波导中TM11模 截面内场分布.截止频率kc和特性阻抗Zc % // ...
- C语言中while语句里使用scanf的技巧
今天友人和我讨论了一段代码,是HDU的OJ上一道题目的解,代码如下 #include<stdio.h> { int a,b; while(~scanf("%d%d",& ...
- 第三次预作业20155231邵煜楠:虚拟机上的Linux学习
java第三次预作业--虚拟机初体验(学习记录) 学习在笔记本上安装Linux操作系统 通过老师给予的官网链接,下载了VirtualBox-5.1.14-112924-win和Ubuntu-16.04 ...
- linux IPC机制学习博客
要求 研究Linux下IPC机制:原理,优缺点,每种机制至少给一个示例,提交研究博客的链接 - 共享内存 - 管道 - FIFO - 信号 - 消息队列 研究博客 管道(PIPE) 管道(PIPE): ...
- jq移除最后一个class的值
$(".his_pg_jl li").on("click",function() {//挂一个点击事件 $(this).addClass('back_img') ...
- OpenCV中Mat操作clone() 与copyto()的区别
OpenCV中Mat操作clone() 与copyto()的区别 // Mat is basically a class with two data parts: the matrix header ...