UVA 11374 Airport Express SPFA||dijkstra
http://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&problem=2369
Description
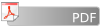
Problem D: Airport Express |
In a small city called Iokh, a train service, Airport-Express, takes residents to the airport more quickly than other transports. There are two types of trains in Airport-Express, the Economy-Xpressand the Commercial-Xpress.
They travel at different speeds, take different routes and have different costs.
Jason is going to the airport to meet his friend. He wants to take the Commercial-Xpress which is supposed to be faster, but he doesn't have enough money. Luckily he has a ticket for the Commercial-Xpress which can take him one station forward. If he used the
ticket wisely, he might end up saving a lot of time. However, choosing the best time to use the ticket is not easy for him.
Jason now seeks your help. The routes of the two types of trains are given. Please write a program to find the best route to the destination. The program should also tell when the ticket should be used.
Input
The input consists of several test cases. Consecutive cases are separated by a blank line.
The first line of each case contains 3 integers, namely N, S and E (2 ≤ N ≤ 500, 1 ≤ S, E ≤ N),
which represent the number of stations, the starting point and where the airport is located respectively.
There is an integer M (1 ≤ M ≤ 1000) representing the number of connections between the stations of the Economy-Xpress. The next Mlines give the information of the routes of the Economy-Xpress.
Each consists of three integers X, Y and Z (X, Y ≤ N, 1 ≤ Z ≤ 100). This means X and Y are
connected and it takes Z minutes to travel between these two stations.
The next line is another integer K (1 ≤ K ≤ 1000) representing the number of connections between the stations of the Commercial-Xpress. The next K lines contain the information of
the Commercial-Xpress in the same format as that of the Economy-Xpress.
All connections are bi-directional. You may assume that there is exactly one optimal route to the airport. There might be cases where you MUST use your ticket in order to reach the airport.
Output
For each case, you should first list the number of stations which Jason would visit in order. On the next line, output "Ticket Not Used" if you decided NOT to use the ticket; otherwise, state the station where Jason should get on the train
of Commercial-Xpress. Finally, print the total time for the journey on the last line. Consecutive sets of output must be separated by a blank line.
Sample Input
4 1 4
4
1 2 2
1 3 3
2 4 4
3 4 5
1
2 4 3
Sample Output
1 2 4
2
5
Problemsetter: Raymond Chun
Originally appeared in CXPC, Feb. 2004
题目大意:
机场快线分为经济线和商业线。两种路线价格、路线、速度不同。给你初始地点和目标地点,还有所有的经济线和商业线,要你求出从到目标地点最快的路线,这条路线有一个要求就是最多坐一条商业线,当然也可以不做,速度最快就好。
要求输出所经过的路径、在哪个站点使用商业线、以及总的时间。
思路:
我们可以先调用两次dijkstra或者两次SPFA,求出起点和终点到所有点的最短路径,然后商业线一一枚举。
example:
INPUT:
5 5 1
3
1 2 4
2 3 4
3 4 4
5
1 2 2
2 3 2
1 3 2
2 4 2
4 5 10
OUT:
5 4 3 2 1
5
22
判断商业线的时候,要特别注意。看下面的代码,要判断双向的。我一开始一直WA就是这样。。
dijkstra版本:0.019s
#include<cstdio>
#include<cstring>
#include<queue>
const int INF=999999;
const int MAXN=520;
const int MAXM=2520;
using namespace std;
struct edge
{
int to;
int val;
int next;
}e[MAXM]; struct node
{
int from;
int val;
node(int f,int v){from=f;val=v;}
bool operator < (const node& b)const
{
return val > b.val;
}
}; int head[MAXN],len;
int dis_s[MAXN],dis_r[MAXN],path_s[MAXN],path_r[MAXN]; void add(int from,int to,int val)
{
e[len].to=to;
e[len].val=val;
e[len].next=head[from];
head[from]=len++;
} int n,s,en;
void dijkstra(int start,int dis[],int path[])
{
bool vis[MAXN]={0};
dis[start]=0; priority_queue<node> q;
q.push(node(start,0));
int num=0;
while(!q.empty())
{
node temp=q.top();
q.pop(); if(vis[temp.from])
continue; vis[temp.from]=true; if(num==n)
break;
for(int i=head[temp.from];i!=-1;i= e[i].next)
{
if( !vis[e[i].to] &&
dis[temp.from] + e[i].val < dis[e[i].to])
{
dis[e[i].to]=dis[temp.from] + e[i].val ;
path[e[i].to] = temp.from;
q.push(node(e[i].to,dis[e[i].to]));
}
}
}
} void print(int cur)
{
if(path_s[cur]!=-1)
print(path_s[cur]); if(cur!=en)
printf("%d ",cur);
else
printf("%d\n",cur);
}
int main()
{
bool not_first=false;
while(~scanf("%d%d%d",&n,&s,&en))
{
if(not_first)
printf("\n");
not_first=true; len=0;
for(int i=1;i<=n;i++)
{
dis_s[i]=dis_r[i]=INF;
head[i]=path_r[i]=path_s[i]=-1;
} int m;
scanf("%d",&m);
for(int i=0;i<m;i++)
{
int from,to,val;
scanf("%d%d%d",&from,&to,&val);
add(from,to,val);
add(to,from,val);
}
dijkstra(s,dis_s,path_s);
dijkstra(en,dis_r,path_r); int k;
scanf("%d",&k);
int ans_from=-1,ans_to,ans_val,ans=dis_s[en]; for(int i=0;i<k;i++)
{
int from,to,val,temp;
scanf("%d%d%d",&from , &to , &val );
temp=dis_s[from] + dis_r[to] + val;
if(temp < ans)
{
ans=temp;
ans_from=from;
ans_to=to;
ans_val=val;
}
temp=dis_s[to] + dis_r[from] + val;
if(temp < ans)
{
ans=temp;
ans_from=to;
ans_to=from;
ans_val=val;
}
}
if(ans_from==-1)
print(en);
else
{
print(ans_from);
int cur=ans_to;
while(cur!=en)
{
printf("%d ",cur);
cur=path_r[cur];
}
printf("%d\n",en);
}
if(ans_from==-1)
printf("Ticket Not Used\n");
else
printf("%d\n",ans_from);
printf("%d\n",ans); } return 0;
}
采用SLF优化的SPFA版本:0.015s
#include<cstdio>
#include<cstring>
#include<queue>
const int INF=999999;
const int MAXN=520;
const int MAXM=2520;
using namespace std;
struct edge
{
int to;
int val;
int next;
}e[MAXM]; int head[MAXN],len;
int dis_s[MAXN],dis_r[MAXN],path_s[MAXN],path_r[MAXN]; void add(int from,int to,int val)
{
e[len].to=to;
e[len].val=val;
e[len].next=head[from];
head[from]=len++;
} int n,s,en;
void SPFA(int start,int dis[],int path[])
{
bool vis[MAXN]={0};
dis[start]=0; deque<int> q;
q.push_back(start);
vis[start]=true;
while(!q.empty())
{
int cur=q.front();
q.pop_front();
vis[cur]=false;
for(int i=head[cur];i!=-1;i=e[i].next)
{
int id=e[i].to;
if( dis[cur] + e[i].val < dis[id] )
{
path[id]=cur;
dis[id]=dis[cur] + e[i].val;
if(!vis[id])
{
if(!q.empty() && dis[id] <dis[q.front()] )
q.push_front(id);
else
q.push_back(id);
vis[id]=true;
}
}
}
}
}
void print(int cur)
{
if(path_s[cur]!=-1)
print(path_s[cur]); if(cur!=en)
printf("%d ",cur);
else
printf("%d\n",cur);
}
int main()
{
bool not_first=false;
while(~scanf("%d%d%d",&n,&s,&en))
{
if(not_first)
printf("\n");
not_first=true; len=0;
for(int i=1;i<=n;i++)
{
dis_s[i]=dis_r[i]=INF;
head[i]=path_r[i]=path_s[i]=-1;
} int m;
scanf("%d",&m);
for(int i=0;i<m;i++)
{
int from,to,val;
scanf("%d%d%d",&from,&to,&val);
add(from,to,val);
add(to,from,val);
}
SPFA(s,dis_s,path_s);
SPFA(en,dis_r,path_r); int k;
scanf("%d",&k);
int ans_from=-1,ans_to,ans_val,ans=dis_s[en]; for(int i=0;i<k;i++)
{
int from,to,val,temp;
scanf("%d%d%d",&from , &to , &val );
temp=dis_s[from] + dis_r[to] + val;
if(temp < ans)
{
ans=temp;
ans_from=from;
ans_to=to;
ans_val=val;
}
temp=dis_s[to] + dis_r[from] + val;
if(temp < ans)
{
ans=temp;
ans_from=to;
ans_to=from;
ans_val=val;
}
}
if(ans_from==-1)
print(en);
else
{
print(ans_from);
int cur=ans_to;
while(cur!=en)
{
printf("%d ",cur);
cur=path_r[cur];
}
printf("%d\n",en);
}
if(ans_from==-1)
printf("Ticket Not Used\n");
else
printf("%d\n",ans_from);
printf("%d\n",ans); } return 0;
}
UVA 11374 Airport Express SPFA||dijkstra的更多相关文章
- UVA - 11374 - Airport Express(堆优化Dijkstra)
Problem UVA - 11374 - Airport Express Time Limit: 1000 mSec Problem Description In a small city c ...
- UVA - 11374 Airport Express (Dijkstra模板+枚举)
Description Problem D: Airport Express In a small city called Iokh, a train service, Airport-Express ...
- UVA 11374 Airport Express 机场快线(单源最短路,dijkstra,变形)
题意: 给一幅图,要从s点要到e点,图中有两种无向边分别在两个集合中,第一个集合是可以无限次使用的,第二个集合中的边只能挑1条.问如何使距离最短?输出路径,用了第二个集合中的哪条边,最短距离. 思路: ...
- UVa 11374 - Airport Express ( dijkstra预处理 )
起点和终点各做一次单源最短路, d1[i], d2[i]分别代表起点到i点的最短路和终点到i点的最短路,枚举商业线车票cost(a, b); ans = min( d1[a] + cost(a, b ...
- UVA 11374 Airport Express(最短路)
最短路. 把题目抽象一下:已知一张图,边上的权值表示长度.现在又有一些边,只能从其中选一条加入原图,使起点->终点的距离最小. 当加上一条边a->b,如果这条边更新了最短路,那么起点st- ...
- UVA 11374 Airport Express (最短路)
题目只有一条路径会发生改变. 常见的思路,预处理出S和T的两个单源最短路,然后枚举商业线,商业线两端一定是选择到s和t的最短路. 路径输出可以在求最短路的同时保存pa数组得到一棵最短路树,也可以用di ...
- UVA 11374 Airport Express(枚举+最短路)
枚举每条商业线<a, b>,设d[i]为起始点到每点的最短路,g[i]为终点到每点的最短路,ans便是min{d[a] + t[a, b] + g[b]}.注意下判断是否需要经过商业线.输 ...
- 训练指南 UVA - 11374(最短路Dijkstra + 记录路径 + 模板)
layout: post title: 训练指南 UVA - 11374(最短路Dijkstra + 记录路径 + 模板) author: "luowentaoaa" catalo ...
- UVA-11374 Airport Express (dijkstra+枚举)
题目大意:n个点,m条无向边,边权值为正,有k条特殊无向边,起止点和权值已知,求从起点到终点的边权值最小的路径,特殊边最多只能走一条. 题目分析:用两次dijkstra求出起点到任何一个点的最小权值, ...
随机推荐
- jquery12 queue() : 队列方法
<!DOCTYPE HTML> <html> <head> <meta http-equiv="Content-Type" content ...
- Android圆形图片不求人,自定义View实现(BitmapShader使用)
在很多APP当中,圆形的图片是必不可少的元素,美观大方.本文将带领读者去实现一个圆形图片自定View,力求只用一个Java类来完成这件事情. 一.先上效果图 二.实现思路 在定义View 的onMea ...
- Python: PS 滤镜-- Fish lens
本文实现 PS 滤镜中的一种几何变换– Fish lens, 对图像做扭曲,感觉就像通过一个凸镜或者凹镜在观察图像一样. import numpy as np from skimage import ...
- Autoencoders and Sparsity(二)
In this problem set, you will implement the sparse autoencoder algorithm, and show how it discovers ...
- 原生JS实现页面内定位
需求:点击跳转到页面指定位置 <div id="test">点击跳转到此处</div> [法一]: 利用a标签的锚点跳转 <a href=" ...
- sync---强制将被改变的内容立刻写入磁盘
sync命令用于强制被改变的内容立刻写入磁盘,更新超块信息. 在Linux/Unix系统中,在文件或数据处理过程中一般先放到内存缓冲区中,等到适当的时候再写入磁盘,以提高系统的运行效率.sync命令则 ...
- HDU1023 Train Problem II【Catalan数】
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=1023 题目大意: 一列N节的火车以严格的顺序到一个站里.问出来的时候有多少种顺序. 解题思路: 典型 ...
- 跟着辛星用PHP的反射机制来实现插件
我的博文的前一篇解说了PHP的反射机制是怎么回事,假设读者还不清楚反射机制,能够搜索下或者看我的博文,都是不错的选择.我们開始解说一下怎么用PHP来实现插件机制.所谓插件机制.就是我们定义一个接口.即 ...
- 9.使用 npm 命令安装模块
转自:http://www.runoob.com/nodejs/nodejs-tutorial.html npm 安装 Node.js 模块语法格式如下: $ npm install <Modu ...
- vue ---webpack 打包上线
先来描述一下期间遇到的问题有哪些: 1.打包后将 dist 文件夹和 index.html 放到 tomcat,在浏览器中访问时,出现空白页,f12 提示 404. 2.打包好的静态资源均是绝对路径 ...