UVA - 12232 Exclusive-OR (并查集扩展偏离向量)
Description
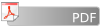
You are not given n non-negative integersX0,X1,...,
Xn-1 less than220, but they do exist, and their values never change.
I'll gradually provide you some facts about them, and ask you some questions.
There are two kinds of facts, plus one kind of question:
Format | Meaning |
I p v |
I tell you Xp = v |
I p q v |
I tell you Xp XOR Xq = v |
Q k p1 p2...pk |
Please tell me the value of Xp1 XOR Xp2 XOR...XOR Xpk |
Input
There will be at most 10 test cases. Each case begins with two integers
n and Q (1n
20,
000, 2Q
40,
000). Each of the following lines contains either a fact or a question, formatted as stated above. Thek parameter in the questions will be a positive integer not greater than 15, and thev
parameter in the facts will be a non-negative integer less than220. The last case is followed byn =
Q = 0, which should not be processed.
Output
For each test case, print the case number on its own line, then the answers, one on each one. If you can't deduce the answer for a particular question, from the facts I provide youbefore that question, print ``I don't know.",
without quotes. If thei-th fact (don't count questions)cannot be consistent with
all the facts before that, print ``The firsti facts are conflicting.", then keep silence for everything after that (including facts and questions). Print a blank line after the output of
each test case.
Sample Input
2 6
I 0 1 3
Q 1 0
Q 2 1 0
I 0 2
Q 1 1
Q 1 0
3 3
I 0 1 6
I 0 2 2
Q 2 1 2
2 4
I 0 1 7
Q 2 0 1
I 0 1 8
Q 2 0 1
0 0
Sample Output
Case 1:
I don't know.
3
1
2 Case 2:
4 Case 3:
7
The first 2 facts are conflicting.
题意:
有n(n<=20000)个未知的整数X0,X1,X2...Xn-1,有下面Q个(Q<=40000)操作:
I p v :告诉你Xp=v
I p q v :告诉你Xp Xor Xq=v
Q k p1 p2 … pk : 询问 Xp1 Xor Xp2 .. Xor Xpk。 k不大于15。
假设当前的I跟之前的有冲突的话,跳出
思路:并查集题目,深深的感到没好好做并查集的无力感,知道是并查集却不知道怎么下手,说一下思路:
1.对于每次的询问,我们并不须要知道每一个数的大小也能够推出来结果,对于这样的: I p v
的我们能够虚拟一个数xn=0,这样就能够有通式p^q=v,由于p^0=p。
虚根xn是不能变的,它的子孙都有确定的值
2.我们如果偏移量val[i]=x[i]^x[fa[i]],还有熟悉a^b = 1 , b^c = 2 , 那么 a^c = 1^2 = 3,还有异或能够互相转化:a^b=c -> a^b^b=c^b -> a = b^c
3.为什么会用到并查集呢,由于对于同一个集合里的话我们能够通过他们与根的偏移量和根的值来知道两个数的异或结果,这样更方便计算,同一时候计算:Q k x1 .. xk 的时候。就能够转化为:(val[x1]^val[x2]..val[xk])^(x[fa[x1]]^x[fa[x2]]..x[fa[xk]]),然后利用异或偶数次不变的原理推断必须是奇数次才有能够得到结果,推断是不是xn根就是了
#include <iostream>
#include <cstdio>
#include <cstring>
#include <map>
#include <algorithm>
using namespace std;
const int MAXN = 20010; int n, m;
int val[MAXN], fa[MAXN]; int find(int x) {
if (x != fa[x]) {
int tmp = fa[x];
fa[x] = find(fa[x]);
val[x] ^= val[tmp];
}
return fa[x];
} int Union(int x, int y, int v) {
int fx = find(x);
int fy = find(y);
if (fx == fy)
return (val[x]^val[y]) == v;
if (fx == n)
swap(fx, fy);
fa[fx] = fy;
val[fx] = val[x]^v^val[y];
return 1;
} int main() {
char str[MAXN];
int p, q, v, k, x;
int cas = 1;
while (scanf("%d%d", &n, &m) != EOF && n+m) {
for (int i = 0; i <= n; i++) {
val[i] = 0;
fa[i] = i;
}
printf("Case %d:\n", cas++);
int facts = 0;
int err = 0;
while (m--) {
scanf("%s", str);
if (str[0] == 'I') {
gets(str);
facts++;
if (err)
continue;
int cnt = sscanf(str, "%d%d%d", &p, &q, &v);
if (cnt == 2) {
v = q;
q = n;
}
if (!Union(p, q, v)) {
err = true;
printf("The first %d facts are conflicting.\n", facts++);
}
} else {
scanf("%d", &k);
int ans = 0;
int is = 1;
map<int, int> mp;
for (int i = 0; i < k; i++) {
scanf("%d", &x);
if (err)
continue;
int f = find(x);
ans ^= val[x];
mp[f]++;
}
if (err)
continue;
map<int, int>::iterator it;
for (it = mp.begin(); it != mp.end(); it++) {
if (it->second % 2) {
if (it->first != n) {
is = 0;
break;
}
else ans ^= val[it->first];
}
}
if (is)
printf("%d\n", ans);
else printf("I don't know.\n");
}
}
printf("\n");
}
return 0;
}
UVA - 12232 Exclusive-OR (并查集扩展偏离向量)的更多相关文章
- UVA 12232 Exclusive-OR(并查集+思想)
题意:给你n个数,接着三种操作: I p v :告诉你 Xp = v I p q v :告诉你 Xp ^ Xq = v Q k p1 p2 … pk:问你k个数连续异或的结果 注意前两类操作可能会出现 ...
- POJ 1182 (经典食物链 /并查集扩展)
(參考他人资料) 向量偏移--由"食物链"引发的总结 http://poj.org/problem?id=1182这道食物链题目是并查集的变型.非常久曾经做的一次是水过的,这次 ...
- UVA 11987 - Almost Union-Find(并查集)
UVA 11987 - Almost Union-Find 题目链接 题意:给定一些集合,操作1是合并集合,操作2是把集合中一个元素移动到还有一个集合,操作3输出集合的个数和总和 思路:并查集,关键在 ...
- POJ 2492 并查集扩展(判断同性恋问题)
G - A Bug's Life Time Limit:10000MS Memory Limit:65536KB 64bit IO Format:%I64d & %I64u S ...
- UVA - 1197 (简单并查集计数)
Severe acute respiratory syndrome (SARS), an atypical pneumonia of unknown aetiology, was recognized ...
- UVA 10158 War(并查集)
//思路详见课本 P 214 页 思路:直接用并查集,set [ k ] 存 k 的朋友所在集合的代表元素,set [ k + n ] 存 k 的敌人 所在集合的代表元素. #include< ...
- UVA - 11987 Almost Union-Find 并查集的删除
Almost Union-Find I hope you know the beautiful Union-Find structure. In this problem, you're to imp ...
- uva 6910 - Cutting Tree 并查集的删边操作,逆序
https://icpcarchive.ecs.baylor.edu/index.php?option=com_onlinejudge&Itemid=8&page=show_probl ...
- UVA - 208 Firetruck(并查集+dfs)
题目: 给出一个结点d和一个无向图中所有的边,按字典序输出这个无向图中所有从1到d的路径. 思路: 1.看到紫书上的提示,如果不预先判断结点1是否能直接到达结点d,上来就直接dfs搜索的话会超时,于是 ...
随机推荐
- [置顶] ※数据结构※→☆线性表结构(queue)☆============循环队列 顺序存储结构(queue circular sequence)(十)
循环队列 为充分利用向量空间,克服"假溢出"现象的方法是:将向量空间想象为一个首尾相接的圆环,并称这种向量为循环向量.存储在其中的队列称为循环队列(Circular Queue). ...
- 在防火墙的例外中注册程序(Windows7和XP),改写注册表
在写程序的时候,经常遇到被防火墙拦截的情况,尤其是一些网络程序,不管是对外访问还是外部连接,都会被拦截. 在大多情况下,Windows会静默拦截外部对内的连接访问,而内部对外的访问会提示用户信息. 现 ...
- hash应用以及vector的使用简介:POJ 3349 Snowflake Snow Snowflakes
今天学的hash.说实话还没怎么搞懂,明天有时间把知识点总结写了,今天就小小的写个结题报告吧! 题意: 在n (n<100000)个雪花中判断是否存在两片完全相同的雪花,每片雪花有6个角,每个角 ...
- linux 软连接 硬连接
1.Linux链接概念 Linux链接分两种,一种被称为硬链接(Hard Link),另一种被称为符号链接(Symbolic Link).默认情况下,ln命令产生硬链接. [硬连接] 硬连接指通过索引 ...
- Opencv各个版本的万能头文件
每次下载opencv的新版本时,都需要重新写头文件,更改链接库配置,很麻烦有木有?下面这个头文件是我在别人的代码中淘出来的,很不错,与大家分享~(具体作者忘记了,不好意思啊) 作者很巧妙地利用Open ...
- junit测试时,出现java.lang.IllegalStateException: Failed to load ApplicationContext
课程设计要求进行junit测试,我是在已经做好的ssh项目上做的测试,测试类代码如下 package com.zhang.web.services; import static org.junit.A ...
- <Win32_17>集音频和视频播放功能于一身的简易播放器
前段时间,在学习中科院杨老师的教学视频时,他说了一句话: "我很反对百八十行的教学程序,要来就来一个完整的程序" 对此,我很是赞同.所谓真刀真枪的做了,你才会发现其中的奥秘——然而 ...
- 算法起步之Dijkstra算法
原文:算法起步之Dijkstra算法 友情提示:转载请注明出处[作者 idlear 博客:http://blog.csdn.net/idlear/article/details/19687579 ...
- 浅谈Swift语法
Apple 在2014年6月的WWDC公布了一款新型的开发语言,很多美国程序猿的价值观貌似和我们非常大的不同,在公布的时候我们能够听到,场下的欢呼声是接连不断的.假设换作我们,特别是像有Objecti ...
- MFC中模态对话框和非模态对话框的差别
在MFC中有模态对话框和非模态对话框,那这两种有什么差别呢. 又都是用于什么场合呢. 首先,要弄清楚2种对话框是怎样创建的. 然后要弄清楚2种对话框有什么差别,可能从表面上看,模态会堵塞主对话框.可原 ...