KoaHub平台基于Node.js开发的Koa router路由插件代码信息详情
koa-router
Router middleware for koa. Provides RESTful resource routing.
Router middleware for koa
- Express-style routing using app.get, app.put, app.post, etc.
- Named URL parameters.
- Named routes with URL generation.
- Responds to OPTIONS requests with allowed methods.
- Support for 405 Method Not Allowed and 501 Not Implemented.
- Multiple route middleware.
- Multiple routers.
- Nestable routers.
- ES7 async/await support (koa-router 7.x).
npm install koa-router
- koa-router
- Router
- new Router([opts])
- instance
- .get|put|post|patch|delete ⇒ Router
- .param(param, middleware) ⇒ Router
- .use([path], middleware, [...]) ⇒ Router
- .routes ⇒ function
- .allowedMethods([options]) ⇒ function
- .redirect(source, destination, code) ⇒ Router
- .route(name) ⇒ Layer | false
- .url(name, params) ⇒ String | Error
- static
- .url(path, params) ⇒ String
- Router
Param
|
Type
|
Description
|
[opts] | Object | |
[opts.prefix] | String | prefix router paths |
var app = require('koa')(); var router = require('koa-router')(); router.get('/', function *(next) {...}); app .use(router.routes()) .use(router.allowedMethods());
router.get|put|post|patch|delete ⇒ Router
router .get('/', function *(next) { this.body = 'Hello World!'; }) .post('/users', function *(next) { // ... }) .put('/users/:id', function *(next) { // ... }) .del('/users/:id', function *(next) { // ... });
router.get('user', '/users/:id', function *(next) { // ... }); router.url('user', 3); // => "/users/3"
router.get( '/users/:id', function *(next) { this.user = yield User.findOne(this.params.id); yield next; }, function *(next) { console.log(this.user); // => { id: 17, name: "Alex" } } );
var forums = new Router(); var posts = new Router(); posts.get('/', function *(next) {...}); posts.get('/:pid', function *(next) {...}); forums.use('/forums/:fid/posts', posts.routes(), posts.allowedMethods()); // responds to "/forums/123/posts" and "/forums/123/posts/123" app.use(forums.routes());
var router = new Router({ prefix: '/users' }); router.get('/', ...); // responds to "/users" router.get('/:id', ...); // responds to "/users/:id"
router.get('/:category/:title', function *(next) { console.log(this.params); // => { category: 'programming', title: 'how-to-node' } });
Param
|
Type
|
Description
|
path | String | |
[middleware] | function | route middleware(s) |
callback | function | route callback |
router.use([path], middleware, [...]) ⇒ Router
Param
|
Type
|
[path] | String |
middleware | function |
[...] | function |
router.use(session(), authorize()); // use middleware only with given path router.use('/users', userAuth()); app.use(router.routes());
router.prefix(prefix) ⇒ Router
Param
|
Type
|
prefix | String |
router.prefix('/things/:thing_id')
router.allowedMethods([options]) ⇒ function
Param
|
Type
|
Description
|
[options] | Object | |
[options.throw] | Boolean | throw error instead of setting status and header |
[options.notImplemented] | Function | throw the returned value in place of the default NotImplemented error |
[options.methodNotAllowed] | Function | throw the returned value in place of the default MethodNotAllowed error |
var app = koa(); var router = router(); app.use(router.routes()); app.use(router.allowedMethods());
var app = koa(); var router = router(); var Boom = require('boom'); app.use(router.routes()); app.use(router.allowedMethods({ throw: true, notImplemented: () => new Boom.notImplemented(), methodNotAllowed: () => new Boom.methodNotAllowed() }));
router.redirect(source, destination, code) ⇒ Router
router.redirect('/login', 'sign-in');
router.all('/login', function *() { this.redirect('/sign-in'); this.status = 301; });
Param
|
Type
|
Description
|
source | String | URL or route name. |
destination | String | URL or route name. |
code | Number | HTTP status code (default: 301). |
router.route(name) ⇒ Layer | false
Param
|
Type
|
name | String |
router.url(name, params) ⇒ String | Error
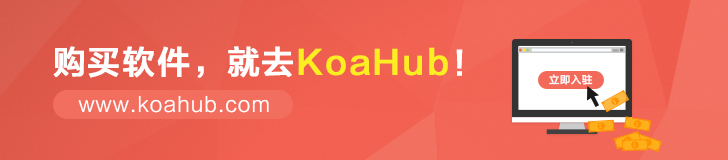
KoaHub平台基于Node.js开发的Koa router路由插件代码信息详情的更多相关文章
- KoaHub平台基于Node.js开发的Koa 连接支付宝插件代码信息详情
KoaHub平台基于Node.js开发的Koa 链接支付宝插件代码信息详情 easy-alipay alipay payment & notification APIs easy-alipay ...
- KoaHub平台基于Node.js开发的Koa JWT认证插件代码信息详情
koa-jwt Koa JWT authentication middleware. koa-jwt Koa middleware that validates JSON Web Tokens and ...
- KoaHub平台基于Node.js开发的Koa EJS渲染插件代码信息详情
koa-ejs ejs render middleware for koa koa-ejs Koa ejs view render middleware. support all feature of ...
- KoaHub平台基于Node.js开发的Koa的skip插件代码详情
koahub-skip koahub skip middleware koahub skip Conditionally skip a middleware when a condition is m ...
- KoaHub平台基于Node.js开发的Koa的简单包装到请求库的类似接口
co-request co-request promisify wrapper for request co-request Simple wrapper to the request library ...
- KoaHub平台基于Node.js开发的Koa的调试实用程序
debug small debugging utility debug tiny node.js debugging utility modelled after node core's debugg ...
- KoaHub平台基于Node.js开发的Koa的连接MongoDB插件代码详情
koa-mongo MongoDB middleware for koa, support connection pool. koa-mongo koa-mongo is a mongodb midd ...
- KoaHub平台基于Node.js开发的Koa的rewrite and index support插件代码详情
koa-static-server Static file serving middleware for koa with directory, rewrite and index support k ...
- KoaHub平台基于Node.js开发的Koa的get/set session插件代码详情
koa-session2 Middleware for Koa2 to get/set session use with custom stores such as Redis or mongodb ...
随机推荐
- C#版--简单工厂模式
为什么要用设计模式? 1.设计模式是前人根据经验总结出来的,使用设计模式,就相当于是站在了前人的肩膀上. 2.设计模式使程序易读.熟悉设计模式的人应该能够很容易读懂运用设计模式编写的程序. 3.设计模 ...
- 小兔JS教程(五) 简单易懂的JSON入门
上一节的参考答案: http://xiaotublog.com/demo.html?path=homework/04/index2 本节重点来介绍一下JSON,JSON(JavaScript Obje ...
- Linux上使用shell脚本查看内存情况(超实用)
#!/bin/bashexport chknum=1 #shell搅拌存放目录(输出日志文件执行后也存于该目录)echo 3 > /wls/wls81/shellsyncwhile [ $chk ...
- KB奇遇记(9):艰难的上线
经历了非常多的磨难,系统也“如约“在2017年01月01日勉强上线了.尽管我认为它还不到上线的程度,条件不具备,但上头的指令下来和计划便是在这一天.整个上线过程从2016年3月8号开始到上线日,扣除中 ...
- 用Spark学习矩阵分解推荐算法
在矩阵分解在协同过滤推荐算法中的应用中,我们对矩阵分解在推荐算法中的应用原理做了总结,这里我们就从实践的角度来用Spark学习矩阵分解推荐算法. 1. Spark推荐算法概述 在Spark MLlib ...
- 《JAVASCRIPT高级程序设计》第二章
把javascript应用在网页中,需要涉及web的核心语言-html:如何让javascript既能与html共存,又不影响页面的显示效果,经过长时间的讨论.试错,最终的决定是为web增加统一的脚本 ...
- java原装代码完成pdf在线预览和pdf打印及下载
这是我在工作中,遇到这样需求,完成需求后,总结的成果,就当做是工作笔记,以免日后忘记,当然,能帮助到别人是最好的啦! 下面进入正题: 前提准备: 1. 项目中至少需要引入的jar包,注意版本: a) ...
- jquery在Asp.net下实现ajax
前台代码: <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="AjaxTest ...
- 认识 getAttribute() setAttribute()
getAttribute()方法不属于document对象,所以不能通过document对象调用,它只能通过元素节点对象调用 var paras = document.getElementsByTag ...
- Java文件中为什么只能有一个public修饰的类, 并且类名还必须与文件名相同
当编写一个java源代码文件时,此文件通常被称为编译单元(有时也被称为转译单元).每个编译单元都必须有一个后缀名.java,而在编译单元内则可以有一个public类,该类的名称必须与文件的名称相同(包 ...