集训第四周(高效算法设计)F题 (二分+贪心)
Description
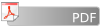
A set of n<tex2html_verbatim_mark> 1-dimensional items have to be packed in identical bins. All bins have exactly the same length l<tex2html_verbatim_mark> and each item i<tex2html_verbatim_mark> has length lil<tex2html_verbatim_mark> . We look for a minimal number of bins q<tex2html_verbatim_mark> such that
- each bin contains at most 2 items,
- each item is packed in one of the q<tex2html_verbatim_mark> bins,
- the sum of the lengths of the items packed in a bin does not exceed l<tex2html_verbatim_mark> .
You are requested, given the integer values n<tex2html_verbatim_mark> , l<tex2html_verbatim_mark> , l1<tex2html_verbatim_mark> , ..., ln<tex2html_verbatim_mark> , to compute the optimal number of bins q<tex2html_verbatim_mark> .
Input
The input begins with a single positive integer on a line by itself indicating the number of the cases following, each of them as described below. This line is followed by a blank line, and there is also a blank line between two consecutive inputs.
The first line of the input file contains the number of items n<tex2html_verbatim_mark>(1n
105)<tex2html_verbatim_mark> . The second line contains one integer that corresponds to the bin length l
10000<tex2html_verbatim_mark> . We then have n<tex2html_verbatim_mark> lines containing one integer value that represents the length of the items.
Output
For each test case, the output must follow the description below. The outputs of two consecutive cases will be separated by a blank line.
For each input file, your program has to write the minimal number of bins required to pack all items.
Sample Input
1 10
80
70
15
30
35
10
80
20
35
10
30
Sample Output
6
Note: The sample instance and an optimal solution is shown in the figure below. Items are numbered from 1 to 10 according to the input order.
#include"iostream"
#include"algorithm"
using namespace std; const int maxn=100000+10; int n,l;
int a[maxn]; void Init()
{
cin>>n>>l;
for(int i=0;i<n;i++) cin>>a[i];
} void Work()
{
sort(a,a+n);
int j=0,ans=0;
for(int i=n-1;i>=0;i--)
{
if(i<j) break;
ans++;
while(a[i]+a[j]<=l&&i>j)
{
j++;
break;
}
}
cout<<ans<<endl;
} int main()
{
int T,t;
cin>>T;
t=T;
while(T--)
{
if(t!=T+1) cout<<endl;
Init();
Work();
}
return 0;
}
集训第四周(高效算法设计)F题 (二分+贪心)的更多相关文章
- 集训第四周(高效算法设计)I题 (贪心)
Description Shaass has n books. He wants to make a bookshelf for all his books. He wants the bookshe ...
- 集训第四周(高效算法设计)E题 (区间覆盖问题)
UVA10382 :http://acm.hust.edu.cn/vjudge/problem/viewProblem.action?id=21419 只能说这道题和D题是一模一样的,不过要进行转化, ...
- 集训第四周(高效算法设计)D题 (区间覆盖问题)
原题 UVA10020 :http://acm.hust.edu.cn/vjudge/problem/viewProblem.action?id=19688 经典的贪心问题,区间上贪心当然是右区间越 ...
- 集训第四周(高效算法设计)A题 Ultra-QuickSort
原题poj 2299:http://poj.org/problem?id=2299 题意,给你一个数组,去统计它们的逆序数,由于题目中说道数组最长可达五十万,那么O(n^2)的排序算法就不要再想了,归 ...
- 集训第四周(高效算法设计)P题 (构造题)
Description There are N<tex2html_verbatim_mark> marbles, which are labeled 1, 2,..., N<te ...
- 集训第四周(高效算法设计)O题 (构造题)
A permutation on the integers from 1 to n is, simply put, a particular rearrangement of these intege ...
- 集训第四周(高效算法设计)N题 (二分查找优化题)
原题:poj3061 题意:给你一个数s,再给出一个数组,要求你从中选出m个连续的数,m越小越好,且这m个数之和不小于s 这是一个二分查找优化题,那么区间是什么呢?当然是从1到数组长度了.比如数组长度 ...
- 集训第四周(高效算法设计)M题 (扫描法)
原题:UVA11078 题意:给你一个数组,设a[],求一个m=a[i]-a[j],m越大越好,而且i必须小于j 怎么求?排序?要求i小于j呢.枚举?只能说超时无上限.所以遍历一遍数组,设第一个被减数 ...
- 集训第四周(高效算法设计)L题 (背包贪心)
Description John Doe is a famous DJ and, therefore, has the problem of optimizing the placement of ...
随机推荐
- ES6的新方法实现数组去重
ES6里新添加了两个很好用的东西,set和Array.from. set是一种新的数据结构,它可以接收一个数组或者是类数组对象,自动去重其中的重复项目. 在这我们可以看见,重复的项目已经被去掉了,包括 ...
- 操作JavaScript的Alert弹框
@Testpublic void testHandleAlert(){ WebElement button =driver.findElement(By.xpath("input" ...
- HBase Region Assign流程详解
Hbase是kv存储,但是逻辑上我们可以把存储在hbase上的kv数据当成表,rowkey可以认为是表的主键.为了便于分布式操作,hbase会把表横向切分成一块一块的数据,而每块就是一个Region. ...
- 5 月编程语言排行榜:Java第一,R跌出Top20
我们都知道,最近,TIOBE 发布了 5 月份编程语言排行榜.其中,前三名依然健稳不变,他们分别是 Java.C.C++,第四则为: Python ,第五则为 VB .NET. 下面两张图,我们可以看 ...
- U9249 【模板】BSGS
题目描述 给定a,b,p,求最小的非负整数x 满足a^x≡b(mod p) 若无解 请输出“orz” 输入输出格式 输入格式: 三个整数,分别为a,b,p 输出格式: 满足条件的非负整数x 输入输出样 ...
- python 使用 Pyscript 调试 报错
UnicodeEncodeError: 'ascii' codec can't encode characters in position 13-16: ordinal not in range(12 ...
- 简洁大方的wordpress主题,不容错过的主题,附带主题源码下载
cu主题是由疯狂的大叔设计,界面简洁大方是它最大的特点之一. 手残君也比较喜爱这款主题,在使用的过程中,根据手残君的个人习惯,对其进行了优化. 标题优化 标题居中显示 增加标题div背景色 标题div ...
- android v7包的关联
最近在使用到侧滑栏的时候,使用到了v7包下的actionbar,结果折腾了好久才折腾好,其实很简单的,操作步骤如下: 1. 在eclipse中导入v7包的工程 2. 在自己的工程中打开properti ...
- Petri网的工具
需要寻找 Petri 网的工具的朋友可以在 http://www.informatik.uni-hamburg.de/TGI/PetriNets/tools/complete_db.html 里面找一 ...
- Node.js——req、res对象
requset对象类型<http.IncomingMessage>,继承stream.Readable类 requset对象: req.headers req.rawHeaders req ...