iphone Dev 开发实例9:Create Grid Layout Using UICollectionView in iOS 6
In this tutorial, we will build a simple app to display a collection of recipe photos in grid layout. Here are what you’re going to learn:
- Introduction to UICollectionView
- How to Use UICollectionView to build a simple Grid-based layout
- Customizing the Collection Cell Background
Create Simple App with Grid Layout
To better understand how UICollectionView work, let’s get some hand-on experience and build a simple app. Open Xcode and create a new project using the “Single View application” template. Name the project as “RecipePhoto” with “Use Storyboard” and “Use Automatic Reference Count” enabled.
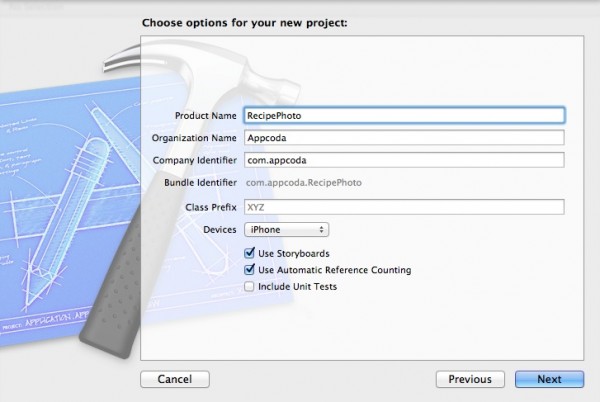
RecipePhoto – New Xcode Project
Designing the Collection View
Go to Storyboard and delete the default view controller. Instead, add a Collection View Controller from object library.

Add a Collection View Controller
In the “Size Inspector” of the Collection View, you can change various size-related attributes. Let’s alter the size of the cell and change it to 100 by 100 pixels.

Change the size of Collection View Cell
Next, select the Collection View Cell and set the identifier as “Cell” in the Attribute Inspector.
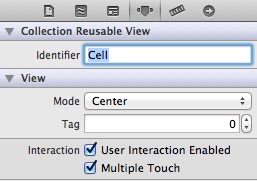
Cell Reuse Identifier
Then, add an Image View to the cell. Xcode automatically resizes the image view and make it fit into the cell. In the “Attribute Inspector”, set the tag value to 100 for later reference.

Add Image View to Cell
Coding the Collection View
In Project Navigator, right-click and select “New File”. Create a new class that is a subclass of UICollectionViewController and name it as RecipeCollectionViewController.
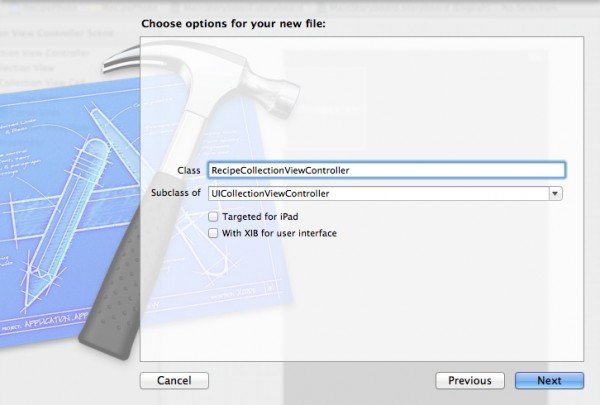
Create RecipeCollectionViewController
Go back to Storyboard and assign it as the custom class of the Collection View Controller.

Assign RecipeCollectionViewController as the custom class
As said, the UICollectionView operates in a very similar way to UITableView. To populate data in UITableView, we have to implement two methods defined in the UITableViewDataSource protocol. Much like the UITableView, the UICollectionViewDataSource protocol defines data source methods for providing the data of the collection view. At least, you have to implement the collectionView:numberOfItemsInSection: and collectionView:cellForItemAtIndexPath: methods.
Let’s move on to code the RecipeCollectionViewController class. First, download this image pack, unzip it and add all the images into the Xcode project.
In RecipeCollectionViewController.m, declare an array for the image files:
1
2 3 |
And initialize it in viewDidLoad method:
1
2 3 4 5 6 7 8 |
- (void)viewDidLoad
{ [super viewDidLoad]; // Initialize recipe image array recipeImages = [NSArray arrayWithObjects:@"angry_birds_cake.jpg", @"creme_brelee.jpg", @"egg_benedict.jpg", @"full_breakfast.jpg", @"green_tea.jpg", @"ham_and_cheese_panini.jpg", @"ham_and_egg_sandwich.jpg", @"hamburger.jpg", @"instant_noodle_with_egg.jpg", @"japanese_noodle_with_pork.jpg", @"mushroom_risotto.jpg", @"noodle_with_bbq_pork.jpg", @"starbucks_coffee.jpg", @"thai_shrimp_cake.jpg", @"vegetable_curry.jpg", @"white_chocolate_donut.jpg", nil]; } |
Next, implement the two mandatory methods of UICollectionViewDataSource protocol:
1
2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section {
return recipeImages.count; } - (UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath{ |
The collectionView:numberOfItemsInSection: method returns the number of recipe images. The cellForItemAtIndexPath: method provides the data for the collection view cells. We first define a cell identifier and then request the collectionView to dequeue a reusable cell using that reuse identifier. In iOS 6, you no longer need to create the cell manually. The dequeueReusableCellWithReuseIdentifier: method will automatically create a cell or return you with a cell from re-use queue. At last, we get the image view by using the tag value and assign it with a recipe image.
Now compile and run the app. You should have a grid-based photo app.
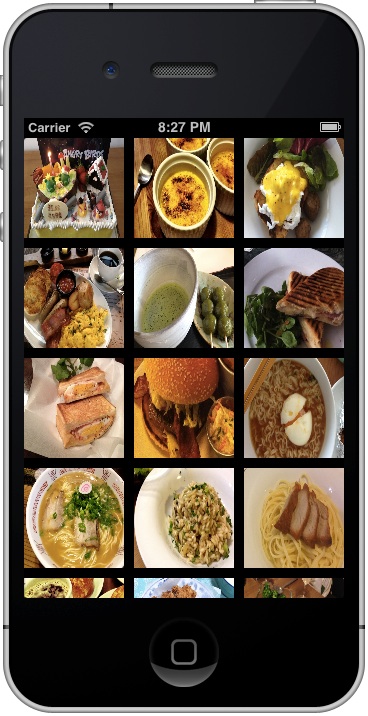
RecipePhoto App with Grid-based Layout
Customizing the Collection Cell Background
Isn’t UICollectionView great? With a few lines of code, you can create a grid-based photo app. But what if you want to add a picture frame to the photos? Like other UI elements, the design of the collection view cell lets developers customize the background easily. The UICollectionViewCell is actually comprised of three different views including background, selected background and content view. It’s best to illustrate the cell views using a picture:
- Background View – background view of the cell
- Selected Background View – the background view when the cell is selected. When user selects the cell, this selected background view will be layered above the background view.
- Content View – obviously, it’s the cell content.
We have already used the content view to display the recipe photo. We’ll make use of the background view to show the picture frame. In the image pack you downloaded earlier, it includes a file named “pic_frame.png”, which is the picture frame. The size of the frame is 100 by 100 pixel. In order to frame the recipe photo, we’ll first resize the image view of cell and re-arrange its position.
Go to Storyboard and select the image view. Set X to 5 and Y to 8. The width and height should be changed to 90 and 72 pixels respectively.

Resize image view to fit into the photo frame
In the cellForItemAtIndexPath: method of RecipeCollectionViewController.m, add the following line of code:
1
|
cell.backgroundView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"photo-frame.png"]];
|
We first load the photo frame image and set it as the cell background. Now compile and run the app again. Your app should look like this:

iphone Dev 开发实例9:Create Grid Layout Using UICollectionView in iOS 6的更多相关文章
- iphone Dev 开发实例10:How To Add a Slide-out Sidebar Menu in Your Apps
Creating the Xcode Project With a basic idea about what we’ll build, let’s move on. You can create t ...
- iphone Dev 开发实例8: Parsing an RSS Feed Using NSXMLParser
From : http://useyourloaf.com/blog/2010/10/16/parsing-an-rss-feed-using-nsxmlparser.html Structure o ...
- iphone dev 入门实例6:How To Use UIScrollView to Scroll and Zoom and Page
http://www.raywenderlich.com/10518/how-to-use-uiscrollview-to-scroll-and-zoom-content Getting Starte ...
- iphone dev 入门实例5:Get the User Location & Address in iPhone App
Create the Project and Design the Interface First, create a new Xcode project using the Single View ...
- iphone dev 入门实例4:CoreData入门
The iPhone Core Data Example Application The application developed in this chapter will take the for ...
- iphone dev 入门实例1:Use Storyboards to Build Table View
http://www.appcoda.com/use-storyboards-to-build-navigation-controller-and-table-view/ Creating Navig ...
- iphone dev 入门实例7:How to Add Splash Screen in Your iOS App
http://www.appcoda.com/how-to-add-splash-screen-in-your-ios-app/ What’s Splash Screen? For those who ...
- iphone dev 入门实例2:Pass Data Between View Controllers using segue
Assigning View Controller Class In the first tutorial, we simply create a view controller that serve ...
- iphone dev 入门实例3:Delete a Row from UITableView
How To Delete a Row from UITableView I hope you have a better understanding about Model-View-Control ...
随机推荐
- leetcode 40 Combination Sum II --- java
Given a collection of candidate numbers (C) and a target number (T), find all unique combinations in ...
- 重启Tomcat的脚本
说明:一台服务器上跑了8个Tomcat case的方式: #!/bin/bash #reboot tomcat!!! #Author:fansik echo -e "\033[1;42;31 ...
- mysql5.6启动占用内存很大的解决方法
vps的内存为512M,安装好nginx,php等启动起来,mysql死活启动不起来看了日志只看到对应pid被结束了,后跟踪看发现是内存不足被killed; 调整my.cnf 参数,重新配置(系统默认 ...
- codeForce-19D Points (点更新+离散化)
题目大意:在二维坐标系的x正半轴,y正半轴和第一象限内,有三种操作: 1.add x,y (添加点<x,y>): 2.remove x,y(移除点<x,y>): 3.find ...
- STM32时钟系统
一.在STM32中,有五个时钟源,为HSI.HSE.LSI.LSE.PLL. ①HSI是高速内部时钟,RC振荡器,频率为8MHz. ②HSE是高速外部时钟,可接石英/陶瓷谐振器,或者接外部时钟源,频率 ...
- 关于正则表达式处理textarea里的换行
将textarea里的内容存入数据库时,会自动将回车换行符过滤成空格,也会将多个空格转换成一个空格,即:将\n等换成 “ ”存入数据库 因此为了将内容从数据库中按照原来格式读出写入到html 就必须 ...
- js获取客户端操作系统
function detectOS() { var sUserAgent = navigator.userAgent; var isWin = (navigator.platform == " ...
- JavaScript验证
<script type="text/javascript"> /*密码*/ function password() { var pas ...
- linux包之gdb之gdb命令与core文件产生
gdb-7.2-64.el6_5.2.x86_64/usr/bin/gcore/usr/bin/gdb/usr/bin/gdb-add-index/usr/bin/gdbtui/usr/bin/gst ...
- 【转】windows7的桌面右键菜单的“新建”子菜单,在注册表哪个位置,如何在“新建"里面添加一个新项
点击桌面,就会弹出菜单,然后在“新建”中就又弹出可以新建的子菜单栏.office与txt 的新建都是在这里面的.我想做的事情是:在右键菜单的“新建” 中添加一个“TQ文本”的新建项,然后点击它之后,桌 ...