Buffered Channels and Worker Pools
原文链接:https://golangbot.com/buffered-channels-worker-pools/
buffered channels
- 带有缓冲区的channel 只有在缓冲区满之后 channel才会阻塞
WaitGroup
- 如果有多个 goroutine在后台执行 那么需要在主线程中 多次等待 可以有一个简单的方法 就是 通过WaitGroup 可以控制 Goroutines 直到它们都执行完成
例子
import (
"fmt"
"sync"
"time"
)
func process(i int, wg *sync.WaitGroup) {
fmt.Println("started Goroutine ", i)
time.Sleep(2 * time.Second)
fmt.Printf("Goroutine %d ended\n", i)
wg.Done()
}
func main() {
no := 3
var wg sync.WaitGroup
for i := 0; i < no; i++ {
wg.Add(1)
go process(i, &wg)
}
wg.Wait()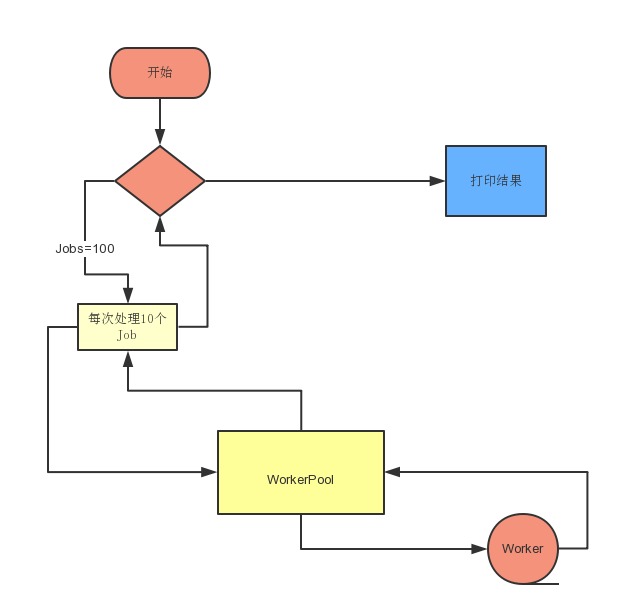
fmt.Println("All go routines finished executing")
}
Worker Pool Implementation
先贴一下个人理解的 程序执行的流程图
import (
"fmt"
"math/rand"
"sync"
"time"
)
type Job struct {
id int
randomno int
}
type Result struct {
job Job
sumofdigits int
}
var jobs = make(chan Job, 10)
var results = make(chan Result, 10)
func digits(number int) int {
sum := 0
no := number
for no != 0 {
digit := no % 10
sum += digit
no /= 10
}
time.Sleep(5 * time.Second)
return sum
}
func worker(wg *sync.WaitGroup) {
for job := range jobs {
output := Result{job, digits(job.randomno)}
results <- output
}
wg.Done()
}
func createWorkerPool(noOfWorkers int) {
var wg sync.WaitGroup
for i := 0; i < noOfWorkers; i++ {
wg.Add(1)
go worker(&wg)
}
wg.Wait()
close(results)
}
func allocate(noOfJobs int) {
for i := 0; i < noOfJobs; i++ {
randomno := rand.Intn(999)
job := Job{i, randomno}
jobs <- job
}
close(jobs)
}
func result(done chan bool) {
for result := range results {
fmt.Printf("Job id %d, input random no %d , sum of digits %d\n", result.job.id, result.job.randomno, result.sumofdigits)
}
done <- true
}
func main() {
startTime := time.Now()
noOfJobs := 100
go allocate(noOfJobs)
done := make(chan bool)
go result(done)
noOfWorkers := 10
createWorkerPool(noOfWorkers)
<-done
endTime := time.Now()
diff := endTime.Sub(startTime)
fmt.Println("total time taken ", diff.Seconds(), "seconds")
}
Buffered Channels and Worker Pools的更多相关文章
- 【Go入门教程7】并发(goroutine,channels,Buffered Channels,Range和Close,Select,超时,runtime goroutine)
有人把Go比作21世纪的C语言,第一是因为Go语言设计简单,第二,21世纪最重要的就是并行程序设计,而Go从语言层面就支持了并行. goroutine goroutine是Go并行设计的核心.goro ...
- 【Go入门教程9】并发(goroutine,channels,Buffered Channels,Range和Close,Select,超时,runtime goroutine)
有人把Go比作21世纪的C语言,第一是因为Go语言设计简单,第二,21世纪最重要的就是并行程序设计,而Go从语言层面就支持了并行. goroutine goroutine是Go并行设计的核心.goro ...
- A Tour of Go Buffered Channels
Channels can be buffered. Provide the buffer length as the second argument to make to initialize a b ...
- GO系列教程
1.介绍与安装 2.Hello World 3.变量 4. 类型 5.常量 6.函数(Function) 7.包 8.if-else 语句 9.循环 10.switch语句 11.数组和切片 12.可 ...
- goroutine与channels
goroutine(协程) 大家都知道java中的线程Thread,golang没有提供Thread的功能,但是提供了更轻量级的goroutine(协程),协程比线程更轻,创办一个协程很简单,只需要g ...
- 【转】 Anatomy of Channels in Go - Concurrency in Go
原文:https://medium.com/rungo/anatomy-of-channels-in-go-concurrency-in-go-1ec336086adb --------------- ...
- golang中channels的本质详解,经典!
原文:https://www.goinggo.net/2014/02/the-nature-of-channels-in-go.html The Nature Of Channels In Go 这篇 ...
- Why should I avoid blocking the Event Loop and the Worker Pool?
Don't Block the Event Loop (or the Worker Pool) | Node.js https://nodejs.org/en/docs/guides/dont-blo ...
- Go by Example
Go by Example Go is an open source programming language designed for building simple, fast, and reli ...
随机推荐
- jdbc查询
import java.util.ArrayList; import java.util.List; import org.springframework.jdbc.core.BeanProperty ...
- setTimeout的异步传输机制
setTimeout是异步的,在设置完setTimeout后,指定代码会在设定的时间后加入到任务队列,但并不是立即执行,js是单线程语言,所有的代码按顺序执行,即同步执行,同步执行的代码放在执行队列中 ...
- SSIS父子维度
1.数据仓库结构: 2.区域的AttributeHierarchyVisible设置为False 3.Parent ID的Usage设置为Parent 4.级别命名: 5.结果:
- 《java学习三》jvm性能优化-------调优
常见参数配置 -XX:+PrintGC 每次触发GC的时候打印相关日志 -XX:+UseSerialGC 串行回收 -XX:+PrintGCDetails 更详细的GC日志 -X ...
- kindeditor 修改上传图片的路径的方法
默认情况下kindeditor上传的图片在编辑器的根目录/attached/目录下.以日期建一个目录,然后保存文件.有些时候大概我们并不想这样.考虑到更新编辑器,或更换编辑器不太方便.比如我现在想把上 ...
- css3弹性伸缩和使用
columns 分栏 column的中文意思就是栏的意思,在html中,作用是分列,把一块内容相同比例均匀的分成一块一块的列,想报纸的内容似的,一篇文章在一张内容上分成好几栏那样显示,它的属性有 1 ...
- 【转】HTTPS系列干货(一):HTTPS 原理详解
HTTPS系列干货(一):HTTPS 原理详解 前言 HTTPS(全称:HyperText Transfer Protocol over Secure Socket Layer),其实 HTTPS 并 ...
- <Android 应用 之路> 聚合数据SDK
聚合数据介绍 聚合数据是一个为智能手机开发者,网站站长,移动设备开发人员及图商提供原始数据API服务的综合性云数据平台.包含手机聚合,网站聚合,LBS聚合三部分,其功能类似于Google APIS.[ ...
- .Net平台互操作技术:02. 技术介绍
上一篇文章简单介绍了.Net平台互操作技术的面临的主要问题,以及主要的解决方案.本文将重点介绍使用相对较多的P/Invoke技术的实现:C#通过P/Invoke调用Native C++ Dll技术.C ...
- Linux 系统挂载阿里云数据盘
适用系统:Linux(Redhat , CentOS,Debian,Ubuntu) * Linux的云服务器数据盘未做分区和格式化,可以根据以下步骤进行分区以及格式化操作. 下面的操作将会把数据盘划 ...