传统servelt项目之分页操作
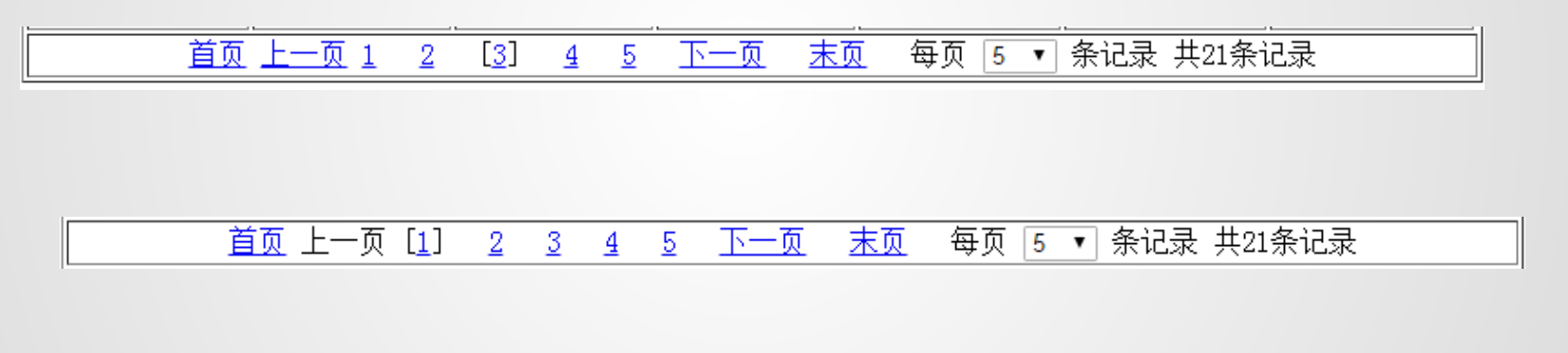
SELECT u_id,m_id as id,m_date as pubTime,m_body as body,m_image as image,m_tranum as tranum,
m_comnum as comnum,m_colnum as colnum,m_like as likeN,to_char(m_date,'yyyy-mm-dd hh24:mi:ss') as str_pubTime FROM
(
SELECT A.*, ROWNUM RN
FROM (SELECT * FROM weibo_tab where u_id=#{param1} and m_state = 0 order by m_date desc) A
WHERE ROWNUM <= #{param3}
)
WHERE RN >= #{param2}
package com.briup.common.util; import java.util.List; /**
* 分页的三个基本属性
* 1.每页几条记录size 可以有默认值5
* 2.当前页号 index 可以有默认值1
* 3.记录总数totalCount:不可能有默认值,需要查询数据库获取真正的记录总数
*
* 4.一共多少页 :totalPageCount=totalCount/size+1
* 5 30 31 32 33 34 35
* 5.上一页 index-1 当前页1,上一页1
* 6.下一页 index+1 当前页是最后一页 下一页:还是最后一页
*
* 扩展
* 分页Bean还可以放要查询的数据 protected List<T> list;
* 分页Bean还可以放页码列表 [1] 2 3 4 5 private int[] numbers;
*
* @author Administrator
*
* @param <T>
*/
public class PageBean<T> {
private int size = ;//每页显示记录 //
private int index = ;// 当前页号
private int totalCount = ;// 记录总数 ok private int totalPageCount = ;// 总页数 ok private int[] numbers;//展示页数集合 //ok
protected List<T> list;//要显示到页面的数据集 /**
* 得到开始记录
* @return
*/
public int getStartRow() { return (index - ) * size;
} /**
* 得到结束记录
* @return
*/
public int getEndRow() { return index * size;
} /**
* @return Returns the size.
*/
public int getSize() {
return size;
} /**
* @param size
* The size to set.
*/
public void setSize(int size) {
if (size > ) {
this.size = size;
}
}
/**
* @return Returns the currentPageNo.
*/
public int getIndex() {
if (totalPageCount == ) { return ;
} return index;
} /**
* @param currentPageNo
* The currentPageNo to set.
*/
public void setIndex(int index) {
if (index > ) {
this.index = index;
}
} /**
* @return Returns the totalCount.
*/
public int getTotalCount() {
return totalCount;
} /**
* @param totalCount
* The totalCount to set.
*/
public void setTotalCount(int totalCount) {
if (totalCount >= ) {
this.totalCount = totalCount;
setTotalPageCountByRs();//根据总记录数计算总页�?
}
} public int getTotalPageCount() {
return this.totalPageCount;
} /**
* 根据总记录数计算总页�?
* 5
* 20 4
* 23 5
*/
private void setTotalPageCountByRs() {
if (this.size > && this.totalCount > && this.totalCount % this.size == ) {
this.totalPageCount = this.totalCount / this.size;
} else if (this.size > && this.totalCount > && this.totalCount % this.size > ) {
this.totalPageCount = (this.totalCount / this.size) + ;
} else {
this.totalPageCount = ;
}
setNumbers(totalPageCount);//获取展示页数集合
} public int[] getNumbers() {
return numbers;
} /**
* 设置显示页数集合
*
* 默认显示10个页码
* 41 42 43 44 [45 ] 46 47 48 49 50
*
*
* [1] 2 3 4 5 6 7 8 9 10
*
* 41 42 43 44 45 46 47 [48] 49 50
* @param totalPageCount
*/
public void setNumbers(int totalPageCount) {
if(totalPageCount>){
//!.当前数组的长度
int[] numbers = new int[totalPageCount>?:totalPageCount];//页面要显示的页数集合
int k =;
//
//1.数组长度<10 1 2 3 4 .... 7
//2.数组长度>=10
// 当前页<=6 1 2 3 4 10
// 当前页>=总页数-5 ......12 13 14 15
// 其他 5 6 7 8 9 当前页(10) 10 11 12 13
for(int i = ;i < totalPageCount;i++){
//保证当前页为集合的中�?
if((i>=index- (numbers.length/+) || i >= totalPageCount-numbers.length) && k<numbers.length){
numbers[k] = i+;
k++;
}else if(k>=numbers.length){
break;
}
} this.numbers = numbers;
} } public void setNumbers(int[] numbers) {
this.numbers = numbers;
} public List<T> getList() {
return list;
} public void setList(List<T> list) {
this.list = list;
} /*
public static int getTotalPageCount(int iTotalRecordCount, int iPageSize) {
if (iPageSize == 0) {
return 0;
} else {
return (iTotalRecordCount % iPageSize) == 0 ? (iTotalRecordCount / iPageSize) : (iTotalRecordCount / iPageSize) + 1;
}
}*/
}
pojo
Customer.java
package com.briup.bean; import java.io.Serializable; public class Customer implements Serializable{
private static final long serialVersionUID = -1415977267636644567L;
private Long id;
private String name;
private String password;
private String zip;
private String address;
private String telephone;
private String email;
public Customer() {
}
public Customer(Long id, String name, String password, String zip,
String address, String telephone, String email) {
super();
this.id = id;
this.name = name;
this.password = password;
this.zip = zip;
this.address = address;
this.telephone = telephone;
this.email = email;
}
public Customer(String name, String password, String zip,
String address, String telephone, String email) {
super(); this.name = name;
this.password = password;
this.zip = zip;
this.address = address;
this.telephone = telephone;
this.email = email;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getZip() {
return zip;
}
public void setZip(String zip) {
this.zip = zip;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getTelephone() {
return telephone;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
dao
package com.briup.dao; import java.util.List; import com.briup.bean.Customer;
import com.briup.bean.Student; public interface StudentDao {
/**
* ��ѯ����ѧ��
* @return
*/
public List<Customer> findAll();
/**
* ��ѯָ����Χ��ѧ��
* @param start
* @param end
* @return
*/
public List<Customer> findStu(int start, int size);
/**
* ��ѯ��¼����
* @return
*/
public int findCount();
/**
* ��ѯ��ϲ�ѯ�����ļ�¼����
* @param name
* @param minScore
* @return
*/
public int findCount(String name);
/**
* ��ѯ��ϲ�ѯ������ָ��ҳ���ѧ��
* @param start
* @param end
* @param name
* @param minScore
* @return
*/
public List<Customer> findStu(int start, int size, String name); }
dao.impl
package com.briup.dao.impl; import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List; import com.briup.bean.Customer;
import com.briup.bean.Student;
import com.briup.common.util.DBUtil;
import com.briup.dao.StudentDao; public class StudentDaoImpl implements StudentDao {
public List<Customer> findAll() {
Connection conn =DBUtil.getConnection();
Statement stmt =null;
ResultSet rs =null;
List<Customer> stuList = new ArrayList<Customer>();
try {
stmt =conn.createStatement();
rs = stmt.executeQuery("select * from student");
while(rs.next()){
Customer cus1 = new Customer();
cus1.setId(rs.getLong("customer_id"));
cus1.setAddress(rs.getString("address"));
cus1.setEmail(rs.getString("email"));
cus1.setName(rs.getString("name"));
cus1.setPassword(rs.getString("password"));
cus1.setTelephone(rs.getString("telephone"));
cus1.setZip(rs.getString("zip"));
stuList.add(cus1);
} } catch (SQLException e) {
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, stmt, conn);
}
return stuList;
} public List<Customer> findStu(int start, int size) {
Connection conn =DBUtil.getConnection();
PreparedStatement pstmt =null;
ResultSet rs =null;
List <Customer> stuList = new ArrayList<Customer>();
try {
String sql = "select * from tbl_customer limit ?,?";
pstmt =conn.prepareStatement(sql);
pstmt.setInt(, start);
pstmt.setInt(, size);
rs = pstmt.executeQuery(); while(rs.next()){
/*private Long id;
private String name;
private String password;
private String zip;
private String address;
private String telephone;
private String email;*/
Customer cus1 = new Customer();
cus1.setId(rs.getLong("customer_id"));
cus1.setAddress(rs.getString("address"));
cus1.setEmail(rs.getString("email"));
cus1.setName(rs.getString("name"));
cus1.setPassword(rs.getString("password"));
cus1.setTelephone(rs.getString("telephone"));
cus1.setZip(rs.getString("zip")); stuList.add(cus1);
} } catch (SQLException e) {
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, pstmt, conn);
}
return stuList;
} public int findCount() {
Connection conn =DBUtil.getConnection();
Statement stmt =null;
ResultSet rs =null;
List <Student> stuList = new ArrayList<Student>();
int count = ;
try {
stmt =conn.createStatement();
rs = stmt.executeQuery("select count(*) from tbl_customer");
rs.next();
count = rs.getInt();
} catch (SQLException e) {
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, stmt, conn);
} return count;
} public int findCount(String name) {
Connection conn =DBUtil.getConnection();
Statement stmt =null; ResultSet rs =null;
List <Student> stuList = new ArrayList<Student>();
int count = ;
try {
StringBuilder sql = new StringBuilder("select count(*) from tbl_customer where 1=1 ");
if(name != null && !"".equals(name)){
sql.append(" and name like '%"+name+"%'");
} stmt =conn.createStatement();
rs = stmt.executeQuery(sql.toString());
rs.next();
count = rs.getInt();
System.out.println("StudentDaoImpl.findCount()"+count);
} catch (SQLException e) {
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, stmt, conn);
} return count;
} public List<Customer> findStu(int start, int size, String name
) {
Connection conn =DBUtil.getConnection();
Statement stmt =null;
ResultSet rs =null;
PreparedStatement pstmt=null;
List<Customer> stuList = new ArrayList<Customer>();
try {
stmt =conn.createStatement();
StringBuilder sql = new StringBuilder("select * from tbl_customer cus where 1=1 ");
if(name != null && !"".equals(name)){
sql.append(" and name like '%"+name+"%'");
} sql.append(" order by score desc"); String sql2 = "select * from tbl_customer limit ?,?"; pstmt = conn.prepareStatement(sql2);
pstmt.setInt(, start);
pstmt.setInt(, size);
rs = pstmt.executeQuery();
while(rs.next()){
Customer cus1 = new Customer();
cus1.setId(rs.getLong("customer_id"));
cus1.setAddress(rs.getString("address"));
cus1.setEmail(rs.getString("email"));
cus1.setName(rs.getString("name"));
cus1.setPassword(rs.getString("password"));
cus1.setTelephone(rs.getString("telephone"));
cus1.setZip(rs.getString("zip"));
stuList.add(cus1);
} } catch (SQLException e) {
e.printStackTrace();
}finally{
DBUtil.closeAll(rs, stmt, conn);
}
return stuList;
}
}
Service
package com.briup.service; import java.util.List; import com.briup.bean.Customer;
import com.briup.bean.Student;
import com.briup.common.util.PageBean; public interface StudentService { /**
* ��ѯ����ѧ��
* @return
*/
public List<Customer> findAll();
/**
* ��ɻ�ķ�ҳ�����������ѯ������
* @param pageBean
*/
public void findStu(PageBean<Customer> pageBean);
/**
* ��ɷ�ҳ���������ѯ������
* @param pageBean
*/
public void findStu(PageBean<Customer> pageBean, String name); }
Service.impl
package com.briup.service.impl; import java.util.List; import com.briup.bean.Customer;
import com.briup.bean.Student;
import com.briup.common.util.PageBean;
import com.briup.dao.StudentDao;
import com.briup.dao.impl.StudentDaoImpl;
import com.briup.service.StudentService; public class StudentServiceImpl implements StudentService { private StudentDao stuDao = new StudentDaoImpl(); public List<Customer> findAll() {
return this.stuDao.findAll();
} public void findStu(PageBean<Customer> pageBean) {
//��ѯ��ݿ���ȡ��¼����
//int totalCount = this.stuDao.findAll().size();//????
int totalCount = this.stuDao.findCount();
System.out.println("count="+totalCount);
//ʹ�ü�¼�������PageBean�е���������(totalCount,totalPageCount,numbers)���Ͳ�list����
pageBean.setTotalCount(totalCount); //����DAO���ȡָ��ҳ��ѧ����ݣ�������pageBean��list����
/*
*ÿҳsize = 5����¼
* �ڼ�ҳ ��ʼ��¼��>= �����¼��<= <
* 1 0 4 5
* 2 5 9 10
* 3 10 14 15
*
* index (index-1)*size index*size
*
*/
//int start = (pageBean.getIndex()-1)*pageBean.getSize();
//int end= pageBean.getIndex()*pageBean.getSize();
int start = pageBean.getStartRow();
int end = pageBean.getEndRow();
int size = pageBean.getSize();
List<Customer> list = this.stuDao.findStu(start,size);
pageBean.setList(list); } public void findStu(PageBean<Customer> pageBean, String name) {
//��ѯ��ݿ���ȡ��ϲ�ѯ�����ļ�¼����
int totalCount = this.stuDao.findCount(name);
System.out.println("count="+totalCount); //ʹ�ü�¼�������PageBean�е���������(totalCount,totalPageCount,numbers)���Ͳ�list����
pageBean.setTotalCount(totalCount); //����DAO���ȡָ��ҳ��ѧ����ݣ�������pageBean��list����
int start = pageBean.getStartRow();
int end = pageBean.getEndRow();
int size = pageBean.getSize();
List<Customer> list = this.stuDao.findStu(start,size,name);
pageBean.setList(list); } }
Servlet
package com.briup.web.servlet; import java.io.IOException; import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse; import com.briup.bean.Customer;
import com.briup.common.util.PageBean;
import com.briup.service.StudentService;
import com.briup.service.impl.StudentServiceImpl; public class ShowAllServlet extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("utf-8"); String sindex = request.getParameter("index"); // null ""
int index = ;
System.out.println(index);
try {
index = Integer.parseInt(sindex);//"5"
} catch (NumberFormatException e) {
e.printStackTrace();
} String ssize = request.getParameter("size"); // null ""
int size = ;
try {
size = Integer.parseInt(ssize);//"5"
} catch (NumberFormatException e) {
e.printStackTrace();
} String name = request.getParameter("name"); //
PageBean<Customer> pageBean = new PageBean<Customer>();
pageBean.setIndex(index);
pageBean.setSize(size);
StudentService stuService = new StudentServiceImpl();
//List<Student> stuList = stuBiz.findAll();
//stuService.findStu(pageBean);//����Ҫ����stuList����Ϊ����ҵ��㴦�?���е���ݶ���PageBean��
stuService.findStu(pageBean,name);
request.setAttribute("pageBean", pageBean);// !!!!!!!
request.setAttribute("name", name); // 3com.bjsxt request.getRequestDispatcher("/jsp/showAll.jsp").forward(request,
response);
} public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
} }
jsp
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%>
<%@taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> <%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>"> <title>查询并显示所有学生信息</title> <meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
<script type="text/javascript">
function changeIndex(index){
location.href="stu?index="+index;
} function changeSize(size){
//alert(size);
location.href= "stu?size="+size;
} function change2(index,size){
//location.href="servlet/ShowAllServlet?index="+index+"&size="+size;
document.forms[].action="stu?index="+index+"&size="+size;
document.forms[].submit();
} function change(index,size){
document.forms[].index.value = index;
//document.forms[0].size.value = size;
document.getElementById("size").value = size;
document.forms[].submit();
} </script>
</head> <body>
<hr>
<form action="stu" method="post" id="form1">
<table align="center">
<tr>
<td>姓名</td>
<td>
<input type="text" name="name" value="${name }">
<input type="hidden" name="index" value="">
<input type="hidden" id="size" name="size">
</td> <td><input type="submit" value="提交"></td>
</tr>
</table>
</form>
<hr> <!-- 显示所有学生 /stumanager/ -->
<table align="center" border="" width="60%">
<tr>
<th>学生 编号</th>
<th>学生姓名</th>
<th>学生年龄</th>
<th>学生成绩</th>
<th>vs.index</th>
<th>更新操作</th>
<th>删除操作</th>
</tr>
<c:forEach items="${pageBean.list}" var="stu" varStatus="vs">
<tr> <td>${stu.id }</td>
<td>${stu.name }</td>
<td>${stu.password}</td>
<td>${stu.zip }</td>
<td>${stu.address }</td>
<td>${stu.telephone }</td>
<td>${stu.email }</td>
<td>${vs.index }</td>
<td><a href="/stumanager/servlet/StudentServlet?operate=preupdate&sid=${stu.id}">更新</a></td>
<td><a href="/stumanager/servlet/StudentServlet?operate=delete&sid=${stu.id}">删除</a></td>
</tr>
</c:forEach>
<tr>
<td colspan="" align="center">
<a href="javascript:change(1,${pageBean.size })">首页</a>
<c:if test="${pageBean.index !=1 }">
<a href="javascript:change(${pageBean.index-1 },${pageBean.size })">上一页 </a>
</c:if>
<c:if test="${pageBean.index ==1 }">
上一页
</c:if>
<c:forEach items="${pageBean.numbers }" var="num">
<c:if test="${num ==pageBean.index }">
[<a href="javascript:change(${num },${pageBean.size })">${num }</a>]
</c:if>
<c:if test="${num != pageBean.index }">
<a href="javascript:change(${num },${pageBean.size })">${num }</a>
</c:if>
</c:forEach>
<c:if test="${pageBean.index != pageBean.totalPageCount }">
<a href="javascript:change(${pageBean.index+1 },${pageBean.size })">下一页</a>
</c:if>
<c:if test="${pageBean.index == pageBean.totalPageCount }">
下一页
</c:if>
<a href="javascript:change(${pageBean.totalPageCount },${pageBean.size })">末页</a>
每页
<select onchange="change(${pageBean.index},this.value)">
<c:forEach begin="" end="" step="" var="i">
<c:if test="${i==pageBean.size }">
<option value="${i }" selected="selected">${i }</option>
</c:if>
<c:if test="${i!=pageBean.size }">
<option value="${i }">${i }</option>
</c:if>
</c:forEach>
</select>
条记录
直接跳到第
<select onchange="change(this.value,${pageBean.size})">
<c:forEach begin="" end="${ pageBean.totalPageCount }" var="num">
<c:if test="${num == pageBean.index }">
<option value="${num }" selected="selected">${num }</option>
</c:if>
<c:if test="${num != pageBean.index }">
<option value="${num }">${num }</option>
</c:if>
</c:forEach>
</select>
页 共${pageBean.totalCount }条记录
</td>
</tr>
</table> </body>
</html>
分页细节分析见github。
传统servelt项目之分页操作的更多相关文章
- asp.net动态网站repeater控件使用及分页操作介绍
asp.net动态网站repeater控件使用及分页操作介绍 1.简单介绍 Repeater 控件是一个容器控件,可用于从网页的任何可用数据中创建自定义列表.Repeater 控件没有自己内置的呈现功 ...
- AngularJS进阶(二十六)实现分页操作
JS实现分页操作 前言 项目开发过程中,进行查询操作时有可能会检索出大量的满足条件的查询结果.在一页中显示全部查询结果会降低用户的体验感,故需要实现分页显示效果.受前面"JS实现时间选择插件 ...
- 配置一个简单的传统SSM项目
背景 我们知道,从2002年开始,Spring一直在飞速的发展,如今已经成为了在Java EE开发中的标准,早期的项目都是传统的Spring-SpringMVC-Mybatis项目,打成一个war包丢 ...
- Spring Boot学习笔记:传统maven项目与采用spring boot项目区别
项目结构区别 传统的maven构建的项目结构如下: 用maven构建的采用springboot项目结构如下: 二者结构一致,区别如下:传统项目如果需要打成war包,需要在WEB-INF目录结构配置we ...
- 011PHP文件处理——文件处理 文件内容分页操作类
<?php /** * 文件内容分页操作类: */ //访问地址:http://basicphp.com/006file/011.php?&page=1 class StrPage { ...
- MySQL中的分页操作结合python
mysql中的分页操作结合python --分页: --方式1: ;-- 读取十行 , --从第十行读取 往后再读十行 --方式2: offset ; --从第二十行开始读取10行 -- 结合pyth ...
- vue中的分页操作
首先,先看分页的代码: 这里还需要进行操作: 1.分页操作主要传递俩个数据,total和pagenum,一个显示当前页面共有多少条数据,一个是翻页后的操作,看列表的数据能不能跟着改变,在进页面发送请求 ...
- 携程 Apollo 配置中心传统 .NET 项目集成实践
官方文档存在的问题 可能由于 Apollo 配置中心的客户端源码一直处于更新中,导致其相关文档有些跟不上节奏,部分文档写的不规范,很容易给做对接的新手朋友造成误导. 比如,我在参考如下两个文档使用传统 ...
- Vue-CLI 项目中相关操作
0830总结 Vue-CLI 项目中相关操作 一.前台路由的基本工作流程 目录结构 |vue-proj | |src | | |components | | | |Nav.vue | | |views ...
随机推荐
- 预定义的 $_GET 变量用于收集来自 method="get" 的表单中的值
PHP $_GET 变量 在 PHP 中,预定义的 $_GET 变量用于收集来自 method="get" 的表单中的值. $_GET 变量 预定义的 $_GET 变量用于收集来自 ...
- PHP easter_days() 函数
------------恢复内容开始------------ 实例 输出不同年份的复活节与 3 月 21 日之间的天数: <?phpecho "Easter Day is " ...
- PHP jdmonthname() 函数
------------恢复内容开始------------ 实例 返回 1998 年 1 月 13 日这天的格利高里历法的月份简写字符串: <?php$jd=gregoriantojd(1,1 ...
- PHP time_sleep_until() 函数
实例 延迟执行当前脚本直到 10 秒: <?php// wake up ten seconds from nowtime_sleep_until(time()+10);?>高佣联盟 www ...
- 2020牛客暑假多校训练营 第二场 H Happy Triangle set 线段树 分类讨论
LINK:Happy Triangle 这道题很容易. 容易想到 a+b<x a<x<b x<a<b 其中等于的情况在第一个和第三个之中判一下即可. 前面两个容易想到se ...
- Vue Router详细教程
1.什么是路由 1.1路由简介 说起路由你想起了什么?路由是一个网络工程里面的术语. 路由(routing)就是通过互联的网络把信息从源地址传输到目的地址的活动. --- 维基百科 额,啥玩意? 没听 ...
- odoo自定义模块项目结构,odoo自定义模块点安装不成功解决办法
如图所示:在odoo源码的根目录中创建自己的项目文件(project) 在odoo.conf配置文件中的addons_path路径中加入自己项目的文件夹路径,推荐使用绝对路径 addons_path ...
- OpenCL Kernel设计优化
使用Intel® FPGA SDK for OpenCL™ 离线编译器,不需要调整kernel代码便可以将其最佳的适应于固定的硬件设备,而是离线编译器会根据kernel的要求自适应调整硬件的结构. 通 ...
- 十分钟搭建自己的私有NuGet服务器-BaGet
目录 前言 开始 搭建BaGet 上传程序包 在vs中使用 其他 最后 前言 NuGet是用于微软.NET(包括 .NET Core)开发平台的软件包管理器.NuGet能够令你在项目中添加.移除和更新 ...
- XCTF-WEB-高手进阶区(1-4)笔记
1:baby_web 题目描述:想想初始页面是哪个 通过Dirsearch软件扫描发现Index.php被藏起来了,访问他便会指向1.php 于是通过Burp修改Get为index.php,然后放入R ...