spring 5.x 系列第9篇 —— 整合mongodb (xml配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all
一、说明
1.1 项目结构说明
配置文件位于resources下,项目以单元测试的方式进行测试。
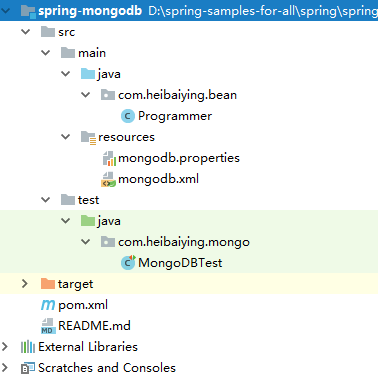
1.2 依赖说明
除了spring的基本依赖外,需要导入mongodb整合依赖包
<!--spring mongodb 整合依赖-->
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-mongodb</artifactId>
<version>2.1.3.RELEASE</version>
</dependency>
二、spring mongodb
2.1 新建配置文件
mongo.host=192.168.200.228
mongo.port=27017
# 数据库名称. 默认是'db'.
mongo.dbname=database
# 每个主机允许的连接数
mongo.connectionsPerHost=10
# 线程队列数,它和上面connectionsPerHost值相乘的结果就是线程队列最大值。如果连接线程排满了队列就会抛出异常
mongo.threadsAllowedToBlockForConnectionMultiplier=5
# 连接超时的毫秒 0是默认值且无限大。
mongo.connectTimeout=1000
# 最大等待连接的线程阻塞时间 默认是120000 ms (2 minutes).
mongo.maxWaitTime=1500
# 保持活动标志,控制是否有套接字保持活动超时 官方默认为true 且不建议禁用
mongo.socketKeepAlive=true
# 用于群集心跳的连接的套接字超时。
mongo.socketTimeout=1500
2.2 整合配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mongo="http://www.springframework.org/schema/data/mongo"
xsi:schemaLocation=
"http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/data/mongo http://www.springframework.org/schema/data/mongo/spring-mongo.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--扫描配置文件-->
<context:property-placeholder location="classpath:mongodb.properties"/>
<!--定义用于访问MongoDB的MongoClient实例-->
<mongo:mongo-client host="${mongo.host}" port="${mongo.port}">
<mongo:client-options
connections-per-host="${mongo.connectionsPerHost}"
threads-allowed-to-block-for-connection-multiplier="${mongo.threadsAllowedToBlockForConnectionMultiplier}"
connect-timeout="${mongo.connectTimeout}"
max-wait-time="${mongo.maxWaitTime}"
socket-keep-alive="${mongo.socketKeepAlive}"
socket-timeout="${mongo.socketTimeout}"
/>
</mongo:mongo-client>
<!--定义用于连接到数据库的连接工厂-->
<mongo:db-factory dbname="${mongo.dbname}" mongo-ref="mongoClient"/>
<!--实际操作mongodb的template,在代码中注入-->
<bean id="anotherMongoTemplate" class="org.springframework.data.mongodb.core.MongoTemplate">
<constructor-arg name="mongoDbFactory" ref="mongoDbFactory"/>
</bean>
</beans>
2.3 测试整合
/**
* @author : heibaiying
* @description : MongoDB 查询
*/
@RunWith(SpringRunner.class)
@ContextConfiguration(locations = "classpath:mongodb.xml")
public class MongoDBTest {
@Autowired
private MongoTemplate mongoTemplate;
@Test
public void insert() {
// 单条插入
mongoTemplate.insert(new Programmer("xiaoming", 12, 5000.21f, new Date()));
List<Programmer> programmers = new ArrayList<Programmer>();
// 批量插入
programmers.add(new Programmer("xiaohong", 21, 52200.21f, new Date()));
programmers.add(new Programmer("xiaolan", 34, 500.21f, new Date()));
mongoTemplate.insert(programmers, Programmer.class);
}
// 条件查询
@Test
public void select() {
Criteria criteria = new Criteria();
criteria.andOperator(where("name").is("xiaohong"), where("age").is(21));
Query query = new Query(criteria);
Programmer one = mongoTemplate.findOne(query, Programmer.class);
System.out.println(one);
}
// 更新数据
@Test
public void MUpdate() {
UpdateResult updateResult = mongoTemplate.updateMulti(query(where("name").is("xiaoming")), update("age", 35), Programmer.class);
System.out.println("更新记录数:" + updateResult.getModifiedCount());
}
// 删除指定数据
@Test
public void delete() {
DeleteResult result = mongoTemplate.remove(query(where("name").is("xiaolan")), Programmer.class);
System.out.println("影响记录数:" + result.getDeletedCount());
System.out.println("是否成功:" + result.wasAcknowledged());
}
}
附:源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all
spring 5.x 系列第9篇 —— 整合mongodb (xml配置方式)的更多相关文章
- spring 5.x 系列第10篇 —— 整合mongodb (代码配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构说明 配置文件位于com.heibaiying. ...
- spring 5.x 系列第13篇 —— 整合RabbitMQ (xml配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构说明 本用例关于rabbitmq的整合提供简单消 ...
- spring 5.x 系列第11篇 —— 整合memcached (xml配置方式)
文章目录 一.说明 1.1 XMemcached客户端说明 1.2 项目结构说明 1.3 依赖说明 二.spring 整合 memcached 2.1 单机配置 2.2 集群配置 2.3 存储基本类型 ...
- spring 5.x 系列第17篇 —— 整合websocket (xml配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构说明 项目模拟一个简单的群聊功能,为区分不同的聊 ...
- spring 5.x 系列第15篇 —— 整合dubbo (xml配置方式)
文章目录 一. 项目结构说明 二.项目依赖 三.公共模块(dubbo-common) 四. 服务提供者(dubbo-provider) 4.1 productService是服务的提供者( 商品数据用 ...
- spring 5.x 系列第1篇 —— springmvc基础 (xml配置方式)
文章目录 一.搭建hello spring工程 1.1 项目搭建 1.2 相关配置讲解 二.配置自定义拦截器 三.全局异常处理 四.参数绑定 4.1 参数绑定 4.2 关于日期格式转换的三种方法 五. ...
- spring 5.x 系列第14篇 —— 整合RabbitMQ (代码配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构说明 本用例关于rabbitmq的整合提供简单消 ...
- spring 5.x 系列第12篇 —— 整合memcached (代码配置方式)
文章目录 一.说明 1.1 XMemcached客户端说明 1.2 项目结构说明 1.3 依赖说明 二.spring 整合 memcached 2.1 单机配置 2.2 集群配置 2.3 存储基本类型 ...
- spring 5.x 系列第18篇 —— 整合websocket (代码配置方式)
源码Gitub地址:https://github.com/heibaiying/spring-samples-for-all 一.说明 1.1 项目结构说明 项目模拟一个简单的群聊功能,为区分不同的聊 ...
随机推荐
- Mysql主从复制,读写分离(mysql-proxy)
Mysql主从复制,读写分离(mysql-proxy) 下面介绍MySQL主从复制,读写分离,双主结构完整构建过程,不涉及过多理论,只有实验和配置的过程. Mysql主从复制(转载请注明出处,博文地址 ...
- STL algorithm算法lexicographical_compare(30)
lexicographical_compare原型: std::lexicographical_compare default (1) template <class InputIterator ...
- 白平衡自己主动(AWB)算法---2,颜色计算
本文说明了白平衡算法估计当前场景的色温过程. 色温计算的原理并不复杂,但要做到,还是一道,认真做好每一步,这需要大量的测试,和算法一直完好. 关于该过程首先简要: 1, 取的图像数据,并划分MxN块, ...
- libev整体设计
转自:http://m.blog.csdn.NET/blog/weiqubo/16355653 libev是Marc Lehmann用C写的高性能事件循环库.通过libev,可以灵活地把各种事件组织管 ...
- Eucalyptus企业云计算(建立能够和Amazon EC2兼容的云)
Eucalyptus是与一个在加利福尼亚大学的研究性项目,创建了一个使企业能够使用它们内部IT资源(包括服务器.存储系统.网络设备)的开源界面,来建立能够和Amazon EC2兼容的云. “Eucal ...
- MySQL 执行原生sql
public class MySqlHelper { private YourContext _context; public MySqlHelper(YourContext context) { _ ...
- WPF 自定义范围分组
<Window x:Class="ViewExam.MainWindow" xmlns="http://schemas.microsoft.com/w ...
- WPF数据模板和控件模板
WPF中有控件模板和数据模板,控件模板可以让我们自定义控件的外观,而数据模板定义了数据的显示方式,也就是数据对象的可视结构,但是这里有一个问题需要考虑,数据是如何显示出来的?虽然数据模板定义了数 ...
- IOS开发之把 Array 和 Dictionaries 序列化成 JSON 对象
1 前言通过 NSJSONSerialization 这个类的 dataWithJSONObject:options:error:方法来实现,Array 和 dictionary 序列化成 JSON ...
- 芯片超Intel,盈利比肩Apple,三星成科技界"全民公敌"
原标题:芯片超英特尔,盈利比肩苹果:三星现在是科技界“全民公敌”了 当人们津津乐道于三星的手机业务或者是电视业务时,它已静悄悄的拿下了芯片行业的第一,并且凭借着在芯片上的巨大获利让它的老对手们眼红 ...