hdu 3853LOOPS (概率DP)
LOOPS
Time Limit: 15000/5000 MS (Java/Others) Memory Limit: 125536/65536 K (Java/Others) Total Submission(s): 2552 Accepted Submission(s): 1041
Homura wants to help her friend Madoka save the world. But because of the plot of the Boss Incubator, she is trapped in a labyrinth called LOOPS.
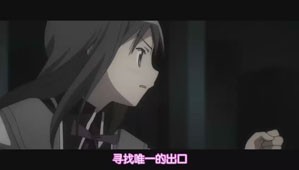
The following R lines, each contains C*3 real numbers, at 2 decimal places. Every three numbers make a group. The first, second and third number of the cth group of line r represent the probability of transportation to grid (r, c), grid (r, c+1), grid (r+1, c) of the portal in grid (r, c) respectively. Two groups of numbers are separated by 4 spaces.
It is ensured that the sum of three numbers in each group is 1, and the second numbers of the rightmost groups are 0 (as there are no grids on the right of them) while the third numbers of the downmost groups are 0 (as there are no grids below them).
You may ignore the last three numbers of the input data. They are printed just for looking neat.
The answer is ensured no greater than 1000000.
Terminal at EOF
0.50 0.50 0.00 1.00 0.00 0.00
- //#define LOCAL
- #include<stdio.h>
- #include<string.h>
- #include<stdlib.h>
- #define maxn 1001
- double dp[maxn][maxn];
- double map[maxn][maxn][];
- int main()
- {
- int rr,cc;
- #ifdef LOCAL
- freopen("test.in","r",stdin);
- #endif
- while(scanf("%d%d",&rr,&cc)!=EOF)
- {
- for(int i=;i<=rr;i++)
- for(int j=;j<=cc;j++)
- scanf("%lf%lf%lf",&map[i][j][],&map[i][j][],&map[i][j][]);
- memset(dp,,sizeof(dp));
- for(int i=rr;i>;i--)
- for(int j=cc;j>;j--){
- if(i==rr&&j==cc)
- continue; //(rr,cc)这个点是出口不需要在走了,停在原地
- if(map[i][j][]!=1.0) //如果没有到终点停下来的话,题目就会误解!
- {
- dp[i][j]=dp[i][j]*map[i][j][]+dp[i+][j]*map[i][j][]+dp[i][j+]*map[i][j][]+;
- dp[i][j]/=(1.0-map[i][j][]);
- }
- }
- printf("%.3lf\n",dp[][]);
- }
- return ;
- }
hdu 3853LOOPS (概率DP)的更多相关文章
- HDU 4599 概率DP
先推出F(n)的公式: 设dp[i]为已经投出连续i个相同的点数平均还要都多少次才能到达目标状态. 则有递推式dp[i] = 1/6*(1+dp[i+1]) + 5/6*(1+dp[1]).考虑当前这 ...
- HDU 5001 概率DP || 记忆化搜索
2014 ACM/ICPC Asia Regional Anshan Online 给N个点,M条边组成的图,每一步能够从一个点走到相邻任一点,概率同样,问D步后没走到过每一个点的概率 概率DP 測 ...
- hdu 3853 概率dp
题意:在一个R*C的迷宫里,一个人在最左上角,出口在右下角,在每个格子上,该人有几率向下,向右或者不动,求到出口的期望 现在对概率dp有了更清楚的认识了 设dp[i][j]表示(i,j)到(R,C)需 ...
- HDU 4815 概率dp,背包
Little Tiger vs. Deep Monkey Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65535/65535 K ( ...
- hdu 4050(概率dp)
算是挺简单的一道概率dp了,如果做了前面的聪聪于可可的话,这题不需要什么预处理,直接概率dp就行了... #include <stdio.h> #include <stdlib.h& ...
- HDU 4405 (概率DP)
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=4405 题目大意:飞行棋.如果格子不是飞行点,扔骰子前进.否则直接飞到目标点.每个格子是唯一的飞行起点 ...
- hdu 4336 概率dp + 状压
hdu 4336 小吃包装袋里面有随机赠送一些有趣的卡片,如今你想收集齐 N 张卡片.每张卡片在食品包装袋里出现的概率是p[i] ( Σp[i] <= 1 ), 问你收集全部卡片所需购买的食品数 ...
- hdu 4576(概率dp+滚动数组)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4576 思路:由于每次从某一位置到达另一位置的概率为0.5,因此我们用dp[i][j]表示第i次操作落在 ...
- hdu 5001 概率DP 图上的DP
http://acm.hdu.edu.cn/showproblem.php?pid=5001 当时一看是图上的就跪了 不敢写,也没退出来DP方程 感觉区域赛的题 一则有一个点难以想到 二则就是编码有 ...
随机推荐
- Datatable分页
using System; using System.Collections.Generic; using System.Web; using System.Data; /// <summary ...
- wooyunAPI
经常要爬去乌云的信息,但是每次都是硬爬,写完了发现乌云有提供API的,整理给大家: 1. WooYun Api是什么 通过WooYun开放的Api接口,其它网站或应用可以根据自己获取的权限调用WooY ...
- JAVA帮助文档全系列 JDK1.5 JDK1.6 JDK1.7 官方中英完整版下载
JAVA帮助文档全系列 JDK1.5 JDK1.6 JDK1.7 官方中英完整版下载JDK(Java Development Kit,Java开发包,Java开发工具)是一个写Java的applet和 ...
- CUBRID学习笔记 8 复制数据库
1 export database 类似sqlserver的分离数据库 cubrid unloaddb demodb分离后生成三个文件 demodb_objects, demodb_indexe ...
- 请求webservice接口的某方法数据
NSURL *url = [NSURL URLWithString:@"http://xxx.xxx.com/xxx/xxxxWS?wsdl"]; NSString *soapMs ...
- 虚拟机安装Centos64位Basic Service后 ifconfig查看无ip
vi /etc/sysconfig/network-scripts/ifcfg-eth0 将 ONBOOT="no" 改为 ONBOOT="yes" 保存后: ...
- vim配置php开发环境
1.ctags-用于代码间的跳转 安装 sudo apt-get install ctags 使用 1). 在某个目录下, 建立tags. ctags -R . --执行之后会在当前目录下生成一个ta ...
- hibernate(三)检索属性配置
检索即对象的获取:获取的时机和和方式:减少没必要的内存占用,尽量少的sql语句减少多余数据库的访问 一:类级别的检索:load() 和属性<class lazy=true> .无论 < ...
- ubuntu安装jdk-6u45-linux-x64.bin___ZC_20160423
for : Android4.4源码编译 环境 : ubuntu12.04_desktop_amd64 1. 1.1.jdk-6u45-linux-x64.bin 放置于 /home 1.2.命令&q ...
- mongoDB 修改器()
-----------------------------------2016-5-26 15:56:57-- source:[1],MongoDB更新操作符