Flying to the Mars
Time Limit:1000MS Memory Limit:32768KB 64bit IO Format:%I64d & %I64u
Description
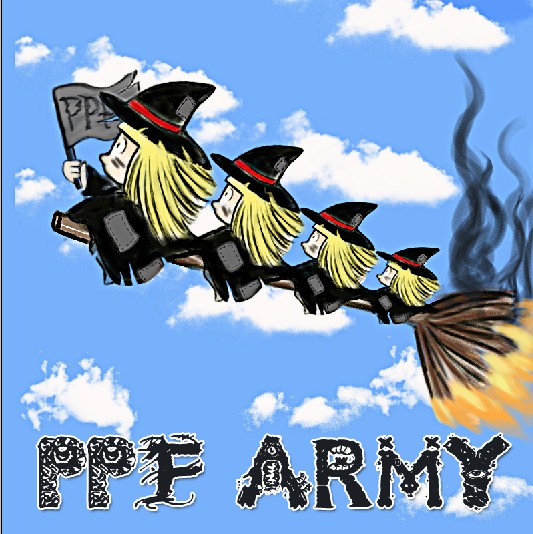
In the year 8888, the Earth is ruled by the PPF Empire . As the population growing , PPF needs to find more land for the newborns . Finally , PPF decides to attack Kscinow who ruling the Mars . Here the problem comes! How can the soldiers reach the Mars ? PPF convokes his soldiers and asks for their suggestions . “Rush … ” one soldier answers. “Shut up ! Do I have to remind you that there isn’t any road to the Mars from here!” PPF replies. “Fly !” another answers. PPF smiles :“Clever guy ! Although we haven’t got wings , I can buy some magic broomsticks from HARRY POTTER to help you .” Now , it’s time to learn to fly on a broomstick ! we assume that one soldier has one level number indicating his degree. The soldier who has a higher level could teach the lower , that is to say the former’s level > the latter’s . But the lower can’t teach the higher. One soldier can have only one teacher at most , certainly , having no teacher is also legal. Similarly one soldier can have only one student at most while having no student is also possible. Teacher can teach his student on the same broomstick .Certainly , all the soldier must have practiced on the broomstick before they fly to the Mars! Magic broomstick is expensive !So , can you help PPF to calculate the minimum number of the broomstick needed .
For example :
There are 5 soldiers (A B C D E)with level numbers : 2 4 5 6 4;
One method :
C could teach B; B could teach A; So , A B C are eligible to study on the same broomstick.
D could teach E;So D E are eligible to study on the same broomstick;
Using this method , we need 2 broomsticks.
Another method:
D could teach A; So A D are eligible to study on the same broomstick.
C could teach B; So B C are eligible to study on the same broomstick.
E with no teacher or student are eligible to study on one broomstick.
Using the method ,we need 3 broomsticks.
……
After checking up all possible method, we found that 2 is the minimum number of broomsticks needed.
Input
In a test case,the first line contains a single positive number N indicating the number of soldiers.(0<=N<=3000)
Next N lines :There is only one nonnegative integer on each line , indicating the level number for each soldier.( less than 30 digits);
Output
Sample Input
10
20
30
04
5
2
3
4
3
4
Sample Output
2
思路:统计输入的数的众数,小于众数的数是众数的学生,大于众数的数是众数的老师,故而众数就是答案。
输入的数不多于30digits,64位整数存放不下,32位更不行,但是很多人用32位或者64位做出来了,原因应该不是数据太弱,而是就算溢出也不影响众数的统计。以下程序用的是大数。另一程序用的是字符串哈希,让字符串映射一个整数。
AC Code:
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <map>
#include <string>
#include <cstdio>
#include <cmath>
#include <cstring> using namespace std; const int maxn = ;
struct Level
{
char l[];
int len;
}a[maxn];
int n; bool cmp(const Level& x, const Level& y)
{
if(x.len != y.len) return x.len < y.len;
for(int i = ; i >= - x.len; i--)
{
if(x.l[i] > y.l[i]) return false;
return true;
}
} int main()
{
char c;
while(scanf("%d%c", &n, &c) != EOF)
{
for(int i = ; i < n; i++)
{
memset(a[i].l, '', sizeof(a[i].l));
a[i].l[] = '\0';
a[i].len = ;
for(int j = ; scanf("%c", &c) && c != '\n';)
{
if(j == && c == '') continue;
a[i].l[j] = c;
a[i].len++;
j--;
}
}
sort(a, a + n, cmp);
int max = -;
for(int i = ; i < n; )
{
int j;
for(j = i + ; j < n; j++)
{
if(strcmp(a[i].l, a[j].l)) break;
}
if(max < j - i) max = j - i;
i = j;
}
printf("%d\n", max);
}
return ;
}
方法二:
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <map>
#include <string>
#include <cstdio>
#include <cmath>
#include <cstring> using namespace std; const int MOD = ;
const int MAXN = ;
const int LEN = ;
const int seed[] = {, };
int level[MAXN];
char s[LEN]; int Hash()
{
int res = ;
int i;
for(i = ; s[i] == ''; i++){}
for(; s[i] != '\0'; i++)
{
res += ((res * seed[s[i]&] + s[i]) % MOD);
}
return res;
} int main()
{
int n;
while(scanf("%d", &n) != EOF)
{
for(int i = ; i < n; i++)
{
scanf("%s", s);
level[i] = Hash();
}
sort(level, level + n);
int max = ;
for(int i = ; i < n;)
{
int j;
for(j = i + ; j < n && level[j] == level[i]; j++){}
if(max < j - i)
max = j - i;
i = j;
}
printf("%d\n", max);
}
return ;
}
Flying to the Mars的更多相关文章
- hdu---(1800)Flying to the Mars(trie树)
Flying to the Mars Time Limit: 5000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Other ...
- hdu 1800 Flying to the Mars
Flying to the Mars 题意:找出题给的最少的递增序列(严格递增)的个数,其中序列中每个数字不多于30位:序列长度不长于3000: input: 4 (n) 10 20 30 04 ou ...
- (贪心 map) Flying to the Mars hdu1800
Flying to the Mars Time Limit: 5000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Other ...
- 杭电 1800 Flying to the Mars(贪心)
http://acm.hdu.edu.cn/showproblem.php?pid=1800 Flying to the Mars Time Limit: 5000/1000 MS (Java/Oth ...
- HDOJ.1800 Flying to the Mars(贪心+map)
Flying to the Mars 点我挑战题目 题意分析 有n个人,每个人都有一定的等级,高等级的人可以教低等级的人骑扫帚,并且他们可以共用一个扫帚,问至少需要几个扫帚. 这道题与最少拦截系统有异 ...
- HDU 1800——Flying to the Mars——————【字符串哈希】
Flying to the Mars Time Limit: 5000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Other ...
- HDU1800 Flying to the Mars 【贪心】
Flying to the Mars Time Limit: 5000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Other ...
- --hdu 1800 Flying to the Mars(贪心)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1800 Ac code: #include<stdio.h> #include<std ...
- hdu 1800 Flying to the Mars(简单模拟,string,字符串)
题目 又来了string的基本用法 //less than 30 digits //等级长度甚至是超过了int64,所以要用字符串来模拟,然后注意去掉前导零 //最多重复的个数就是答案 //关于str ...
随机推荐
- 软工实践Beta冲刺答辩
福大软工 · 第十二次作业 - Beta答辩总结 组长本次博客作业链接 项目宣传视频链接 本组成员 1 . 队长:白晨曦 031602101 2 . 队员:蔡子阳 031602102 3 . 队员:陈 ...
- Alpha事后诸葛会议
[设想和目标] Q1:我们的软件要解决什么问题?是否定义得很清楚?是否对典型用户和典型场景有清晰的描述? "小葵日记"是为了解决18-30岁年轻用户在记录生活时希望得到一美体验友好 ...
- HDU 1277 Nested Dolls
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=1677 题意: 玩俄罗斯套娃,问最后至少还剩几个. 题解: 这题可以和拦截导弹做对比,因为这里是二维的 ...
- Spring Boot(八)集成Spring Cache 和 Redis
在Spring Boot中添加spring-boot-starter-data-redis依赖: <dependency> <groupId>org.springframewo ...
- OSG学习:使用OSG中预定义的几何体
常用的内嵌几何体包括: osg::Box //正方体 osg::Capsule //太空舱 osg::Cone //椎体 osg::Cylinder //柱体 osg::HeightField //高 ...
- ViewController 视图控制器的常用方法
ViewController 视图控制器 ,是控制界面的控制器,通俗的来说,就是管理我们界面的大boss,视图控制器里面包含了视图,下面举个例子,让视图在两个视图上调转. 定义一个视图控制器: MyV ...
- php 多维数组排序
PHP中array_multisort可以用来一次对多个数组进行排序,或者根据某一维或多维对多维数组进行排序. 关联(string)键名保持不变,但数字键名会被重新索引. 输入数组被当成一个表的列并以 ...
- IDEA中Git的更新/提交/还原方法
记录一下在IDEA上怎样将写的代码提交到GitHub远程库: 下面这个图是基本的提交代码的顺序: 1. 将代码Add到stage暂存区本地修改了代码后,需先将代码add到暂存区,最后才能真正提价到gi ...
- 【vue】vue组件的自定义事件
父组件: <template> <div> <my-child abcClick="sayHello"></my-child> &l ...
- 常用的一些sql
--根据某一列中包括的逗号将一行数据变多行 select a,c from (with test as (select 'abc' a,'1,2,3' c from dual e) select a, ...