Codeforces Round #843 (Div. 2) Problem C
C. Interesting Sequence
time limit per test
1 second
256 megabytes
input
standard input
standard output
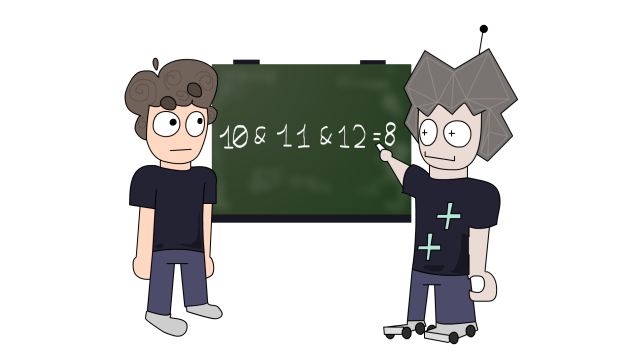
Each test contains multiple test cases. The first line contains the number of test cases t (1≤t≤2000). The description of the test cases follows.The only line of each test case contains two integers n, x (0≤n,x≤10^18).
For every test case, output the smallest possible value of mm such that equality holds.If the equality does not hold for any m, print −1 instead.We can show that if the required m exists, it does not exceed 5⋅10^18.
12
10
-1
24
1152921504606846976
In the first example, 10 & 11=10, but 10 & 11 & 12=8, so the answer is 12.
In the second example, 10=10, so the answer is 10.
In the third example, we can see that the required m does not exist, so we have to print −1.
思路:
我们可以
按位考虑。如果
- n 在这一位上是 0 , x 在这一位上是 0
- 选取任何的 m 都可行。
- n 在这一位上是 0 , x 在这一位上是 1
- 不可能实现。
- n 在这一位上是 1 , x 在这一位上是 0
- 必须等到某一个在这一位为 0 的数出现,才能满足要求。
- 设这个数最小为 k ,则可行域与 [k,+∞] 取交集。
- n 在这一位上是 1 , x 在这一位上是 1
- m 必须在某一个在这一位为 0 的数出现之前,才能满足要求。
- 设这个数最小为 k ,则可行域与 [n,k) 取交集。
最后,如果可行域不为空,输出最小元素。时间复杂度是 Θ(logmax(n,x))
代码:
1 #include<bits/stdc++.h>
2 #define N 70
3 using namespace std;
4 typedef long long ll;
5
6 void solve()
7 {
8 ll n,x;
9 scanf("%lld%lld",&n,&x);
10 bitset<64> bn(n),bx(x);
11 ll l=n,r=5e18;
12 for(int i=63;i>=0;i--)
13 {
14 if(bn[i]==0 && bx[i]==1)
15 {
16 puts("-1");
17 return;
18 }
19 if(bn[i]==0 && bx[i]==0) continue;
20 if(bn[i]==1 && bx[i]==0)
21 {
22 l=max(l,((n/(1ll<<i))+1)*(1ll<<i));
23 //二进制 1010 * 10 = 10100
24 //一个数乘 100...00 相当于左移相应的位数
25 //一个数整除 100...00 相当于把这个1右边的所有位数变成0
26 }
27 else{
28 r=min(r,((n/(1ll<<i))+1)*(1ll<<i)-1);
29 }
30 }
31
32 if(l<=r) printf("%lld\n",l);
33 else puts("-1");
34
35 return ;
36 }
37
38 int main()
39 {
40 int _;
41 cin>>_;
42 while(_--) solve();
43 return 0;
44 }
Noted by DanRan02
2023.1.11
Codeforces Round #843 (Div. 2) Problem C的更多相关文章
- Codeforces Round #716 (Div. 2), problem: (B) AND 0, Sum Big位运算思维
& -- 位运算之一,有0则0 原题链接 Problem - 1514B - Codeforces 题目 Example input 2 2 2 100000 20 output 4 2267 ...
- Codeforces Round #753 (Div. 3), problem: (D) Blue-Red Permutation
还是看大佬的题解吧 CFRound#753(Div.3)A-E(后面的今天明天之内补) - 知乎 (zhihu.com) 传送门 Problem - D - Codeforces 题意 n个数字,n ...
- Codeforces Round #243 (Div. 2) Problem B - Sereja and Mirroring 解读
http://codeforces.com/contest/426/problem/B 对称标题的意思大概是.应当指出的,当线数为奇数时,答案是线路本身的数 #include<iostream& ...
- Codeforces Round #439 (Div. 2) Problem E (Codeforces 869E) - 暴力 - 随机化 - 二维树状数组 - 差分
Adieu l'ami. Koyomi is helping Oshino, an acquaintance of his, to take care of an open space around ...
- Codeforces Round #439 (Div. 2) Problem C (Codeforces 869C) - 组合数学
— This is not playing but duty as allies of justice, Nii-chan! — Not allies but justice itself, Onii ...
- Codeforces Round #439 (Div. 2) Problem B (Codeforces 869B)
Even if the world is full of counterfeits, I still regard it as wonderful. Pile up herbs and incense ...
- Codeforces Round #439 (Div. 2) Problem A (Codeforces 869A) - 暴力
Rock... Paper! After Karen have found the deterministic winning (losing?) strategy for rock-paper-sc ...
- Codeforces Round #427 (Div. 2) Problem D Palindromic characteristics (Codeforces 835D) - 记忆化搜索
Palindromic characteristics of string s with length |s| is a sequence of |s| integers, where k-th nu ...
- Codeforces Round #427 (Div. 2) Problem C Star sky (Codeforces 835C) - 前缀和
The Cartesian coordinate system is set in the sky. There you can see n stars, the i-th has coordinat ...
- Codeforces Round #427 (Div. 2) Problem A Key races (Codeforces 835 A)
Two boys decided to compete in text typing on the site "Key races". During the competition ...
随机推荐
- Java枚举类的学习
package java1; /** * @author 高槐玉 * #Description: * 枚举类的使用 * 1,枚举类的理解:类的对象只有有限个,确定的.我们称此类为枚举类 * 2.当需要 ...
- 【笔记】Win7连接公司内网无法打开网页
win7尝试使用VPN连接公司内网发现无法打开单点登录网页 DNS解析没问题 服务器也能ping通 查了半天才发现是MTU的问题 win7的默认值1400 公司的华为设备设置的比这个值小 要根据具体的 ...
- Vue案例——todolist
最近在学习vue,实现todolist案例,实现效果如下: 该案例分为四个部分:header为输入框,body为列表,item是列表中的条目,footer为最下方的统计. 实现步骤: ①创建项目 vu ...
- 力扣51. N 皇后(回溯法)
按照国际象棋的规则,皇后可以攻击与之处在同一行或同一列或同一斜线上的棋子. n 皇后问题 研究的是如何将 n 个皇后放置在 n×n 的棋盘上,并且使皇后彼此之间不能相互攻击. 给你一个整数 n ,返回 ...
- 一、MySQL 函数
1.MySQL 字符串函数 函数 描述 实例 结果展示 说明 REPLACE(s,s1,s2) 将字符串s2代替字符串s中的字符串s1 SELECT REPLACE(ccc.contract_no,& ...
- Ubuntu22 vim配置
插件管理器 vim-plug # 下载插件管理器 sh -c 'curl -fLo ~/.vim/autoload/plug.vim --create-dirs \ https://raw.githu ...
- ABAP链接FTP把txt文件数据获取到内表
啥都不说,直接上代码 ******* 如果无法链接FTP,可能需要往表SAPFTP_SERVERS加入IP地址和端口(21)即可 DATA:p_host TYPE char64 VALUE 'IP', ...
- tp项目部署到宝塔,运行nginx时无法访问首页之外的页面
http://www.upwqy.com/details/254.html tp项目 部署到宝塔里面 运行环境nginx 直接访问首页是可以访问的.但是请求其他的接口的时候 报404 . 需要把下面这 ...
- Kubernetes Service发布
一.定义Service 1-1.首先创建一个Deployment 类型nginx #定义Deployment类型nginx yaml文件 apiVersion: apps/v1 kind: Deplo ...
- P1982 [NOIP2013 普及组] 小朋友的数字 题解
目录 简单版 题目 code 本题 code 简单版 先要会做这道题 题目 P1115 最大子段和https://www.luogu.com.cn/problem/P1115 这道题其实是动态规划,d ...