题解报告:hdu 2196 Computer(树形dp)
Problem Description
Hint: the example input is corresponding to this graph. And from the graph, you can see that the computer 4 is farthest one from 1, so S1 = 3. Computer 4 and 5 are the farthest ones from 2, so S2 = 2. Computer 5 is the farthest one from 3, so S3 = 3. we also get S4 = 4, S5 = 4.
Input
Output
Sample Input
Sample Output
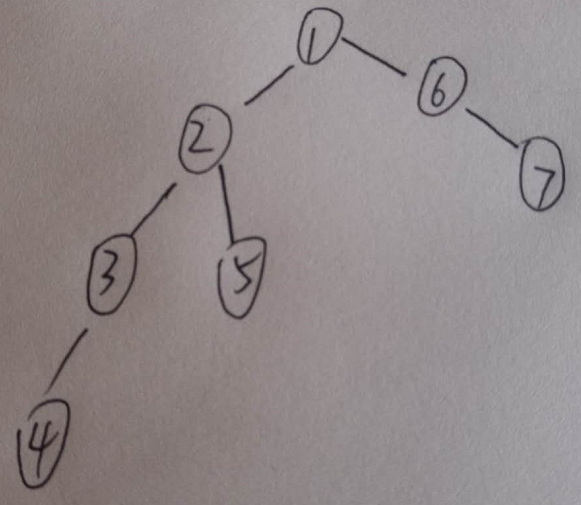
#include<bits/stdc++.h>
using namespace std;
const int maxn=;
struct node{int to,next,len;}edge[maxn<<];
int n,x,y,cnt,head[maxn],dp[maxn][],lgst[maxn];
void add_edge(int u,int v,int w){
edge[cnt].to=v;
edge[cnt].len=w;
edge[cnt].next=head[u];
head[u]=cnt++;
}
int dfs1(int u,int fa){
int Dmax=,Dsec=;
for(int i=head[u];~i;i=edge[i].next){
int v=edge[i].to;
if(v^fa){
int nowd=dfs1(v,u)+edge[i].len;
if(nowd>Dmax)lgst[u]=v,Dsec=Dmax,Dmax=nowd;//lgst[u]=v记录u的正向最长路径上的节点v
else if(nowd>Dsec)Dsec=nowd;
}
}
dp[u][]=Dmax,dp[u][]=Dsec;//记录每个节点的正向最长距离和正向次长距离
return Dmax;//返回正向最长距离
}
void dfs2(int u,int fa){
for(int i=head[u];~i;i=edge[i].next){
int v=edge[i].to;
if(v^fa){
if(v==lgst[u])dp[v][]=max(dp[u][],dp[u][])+edge[i].len;
else dp[v][]=max(dp[u][],dp[u][])+edge[i].len;
dfs2(v,u);
}
}
}
int main(){
while(~scanf("%d",&n)){
cnt=;memset(head,-,sizeof(head));
memset(dp,,sizeof(dp));
memset(lgst,,sizeof(lgst));
for(int i=;i<=n;++i){
scanf("%d%d",&x,&y);
add_edge(i,x,y);
add_edge(x,i,y);
}
dfs1(,-);
dfs2(,-);
for(int i=;i<=n;++i)
printf("%d\n",max(dp[i][],dp[i][]));
}
return ;
}
题解报告:hdu 2196 Computer(树形dp)的更多相关文章
- HDU 2196.Computer 树形dp 树的直径
Computer Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- HDU 2196 Computer 树形DP经典题
链接:http://acm.hdu.edu.cn/showproblem.php? pid=2196 题意:每一个电脑都用线连接到了还有一台电脑,连接用的线有一定的长度,最后把全部电脑连成了一棵树,问 ...
- HDU 2196 Computer 树形DP 经典题
给出一棵树,边有权值,求出离每一个节点最远的点的距离 树形DP,经典题 本来这道题是无根树,可以随意选择root, 但是根据输入数据的方式,选择root=1明显可以方便很多. 我们先把边权转化为点权, ...
- hdu 2196 Computer(树形DP)
Computer Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- hdu 2196 Computer 树形dp模板题
Computer Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total S ...
- hdu 2196 Computer(树形DP经典)
Computer Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
- HDU 2196 Computer (树dp)
题目链接:http://acm.split.hdu.edu.cn/showproblem.php?pid=2196 给你n个点,n-1条边,然后给你每条边的权值.输出每个点能对应其他点的最远距离是多少 ...
- HDU - 2196(树形DP)
题目: A school bought the first computer some time ago(so this computer's id is 1). During the recent ...
- hdu 2196【树形dp】
http://acm.hdu.edu.cn/showproblem.php?pid=2196 题意:找出树中每个节点到其它点的最远距离. 题解: 首先这是一棵树,对于节点v来说,它到达其它点的最远距离 ...
- HDU 2196 Compute --树形dp
Computer Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Su ...
随机推荐
- (转)CSS3全局实现所有元素的内边距和边框不增加
全局设置 border-box 很好,首先它符合直觉,其次它可以省去一次又一次的加加减减 它还有一个关键作用——让有边框的盒子正常使用百分比宽度.但是使用了 border-box 可能会与一些依赖默认 ...
- HDOJ_1000
#include int main() { int i, j; while(scanf("%d%d", &i, &j) == 2) printf("%d\ ...
- TiDB 整体架构 结合yarn zookeeper分析架构
TiDB 简介与整体架构| PingCAP https://www.pingcap.com/docs-cn/overview/ 真正金融级高可用 相比于传统主从 (M-S) 复制方案,基于 Raft ...
- BusyBox下ftpget的使用方法
在终端输入ftpget命令,可以得到以下帮助信息: BusyBox v1.17.4 (2010-12-22 10:59:18 CST) multi-call binary. Usage: ftpget ...
- ios常用到的第三方库
在iOS开发中不可避免的会用到一些第三方类库,它们提供了很多实用的功能,使我们的开发变得更有效率:同时,也可以从它们的源代码中学习到很多有用的东西. Reachability 检测网络连接 用来检查网 ...
- NOIP2016总结
Day1: T1:模拟: #include<iostream> #include<cstdio> #include<cstdlib> #include<cst ...
- async-await系列翻译(一)
本篇翻译的英文链接:https://docs.microsoft.com/en-us/dotnet/articles/standard/async-in-depth 使用.NET的基于任务的异步编程模 ...
- 「网络流24题」「LuoguP2774」方格取数问题(最大流 最小割
Description 在一个有 m*n 个方格的棋盘中,每个方格中有一个正整数.现要从方格中取数,使任意 2 个数所在方格没有公共边,且取出的数的总和最大.试设计一个满足要求的取数算法.对于给定的方 ...
- Watir: 当出现错误提示AutoItX3.dll 没有注册的时候,该怎么处理?
对于Ruby 1.8版本,以管理员身份运行命令行窗口,输入Regsvr32 AutoItX3.dll路径即可.对于1.9 版本,路径与1.8版本是不同的,我们可以进入Ruby安装目录下,搜索AutoI ...
- AES加密算法动画演示
波士顿大学的Howard Straubing做了这么一个动画来展示AES加密算法的演示,挺不错的. 点击这里看全屏