[iOS微博项目 - 1.0] - 搭建基本框架
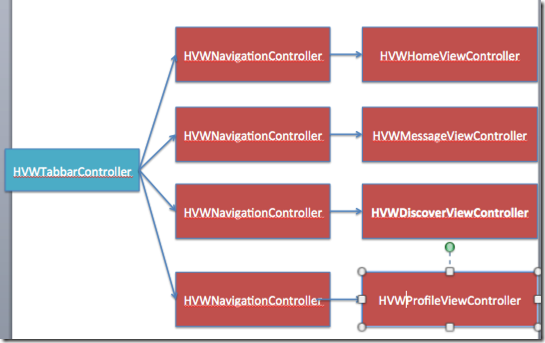
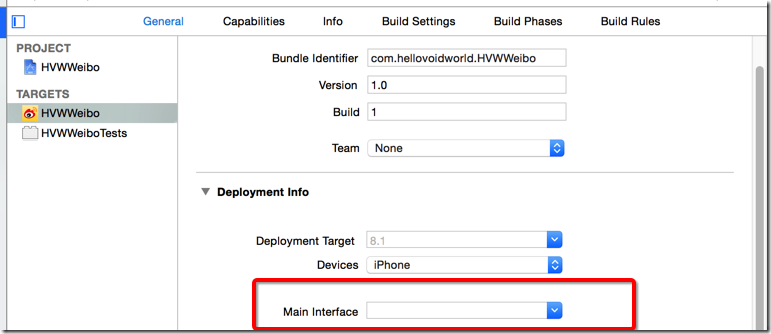
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch. // 启动后显示状态栏
UIApplication *app = [UIApplication sharedApplication];
app.statusBarHidden = NO; // 设置window
self.window = [[UIWindow alloc] init];
self.window.frame = [UIScreen mainScreen].bounds; [self.window makeKeyAndVisible]; return YES;
}
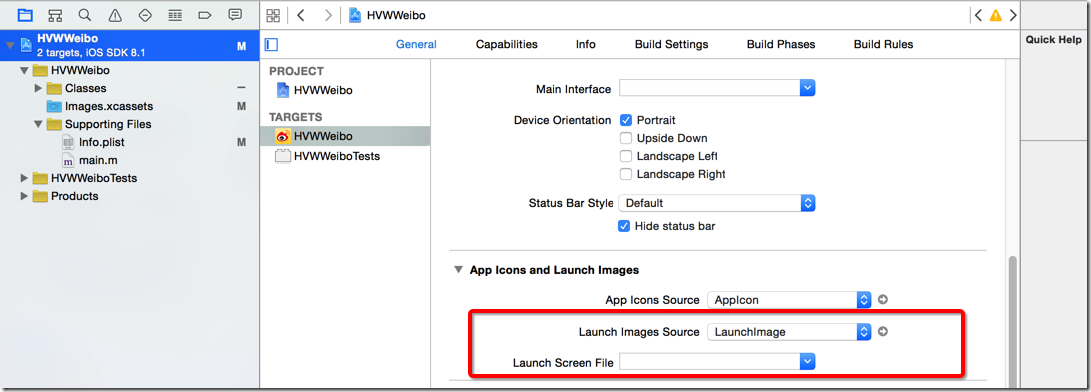
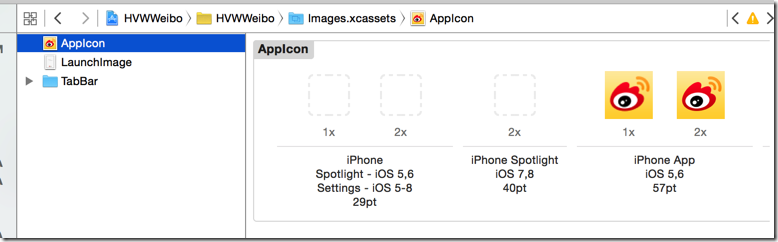
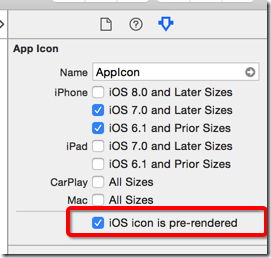
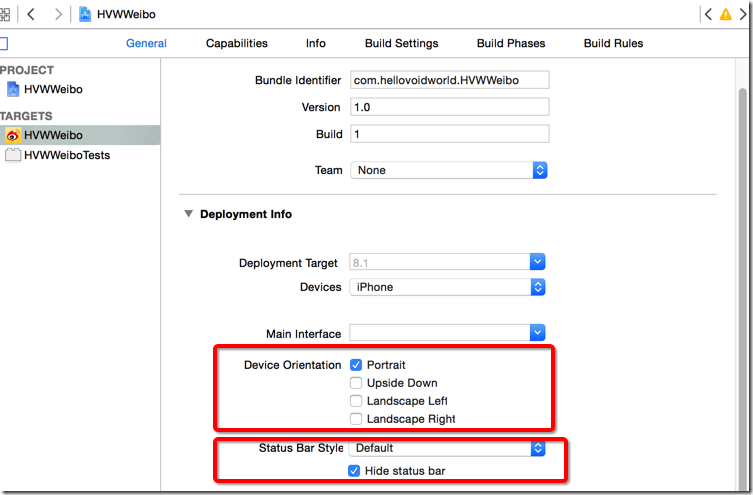

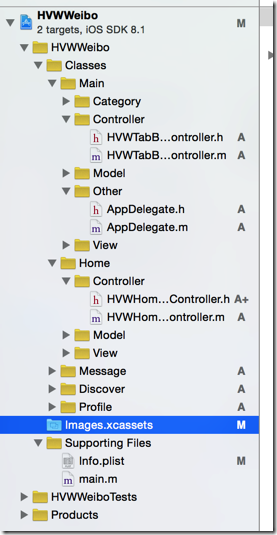
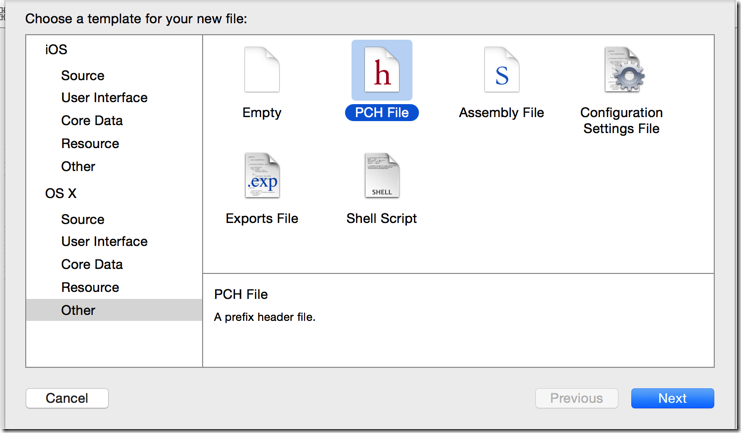
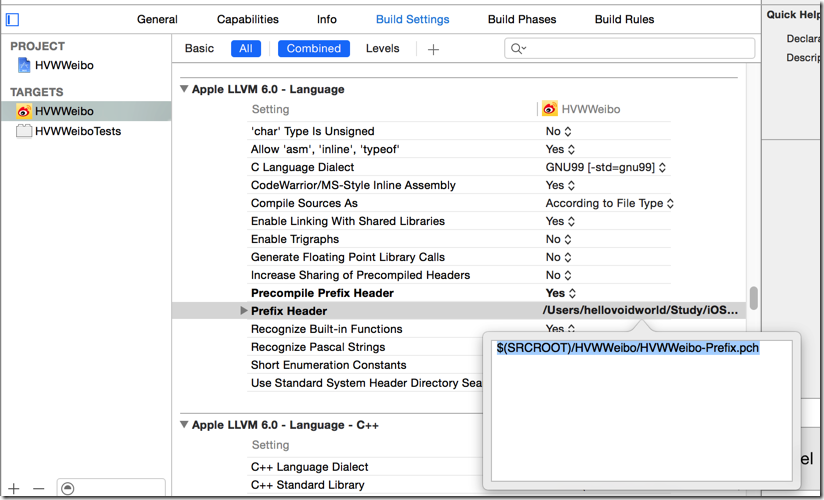
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch. // 启动后显示状态栏
UIApplication *app = [UIApplication sharedApplication];
app.statusBarHidden = NO; // 设置window
self.window = [[UIWindow alloc] init];
self.window.frame = [UIScreen mainScreen].bounds; // 创建根控制器
HVWTabBarViewController *tabVC = [[HVWTabBarViewController alloc] init];
self.window.rootViewController = tabVC; [self.window makeKeyAndVisible]; return YES;
}
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view. // 添加子控制器
// 首页
HVWHomeViewController *homeVC = [[HVWHomeViewController alloc] init];
homeVC.view.backgroundColor = [UIColor redColor];
homeVC.title = @"首页";
[self addChildViewController:homeVC]; // 消息
HVWMessageViewController *messageVC = [[HVWMessageViewController alloc] init];
messageVC.view.backgroundColor = [UIColor blueColor];
messageVC.title = @"消息";
[self addChildViewController:messageVC]; // 发现
HVWDiscoverViewController *discoverVC = [[HVWDiscoverViewController alloc] init];
discoverVC.view.backgroundColor = [UIColor yellowColor];
discoverVC.title = @"发现";
[self addChildViewController:discoverVC]; // 我
HVWProfileViewController *profileVC = [[HVWProfileViewController alloc] init];
profileVC.view.backgroundColor = [UIColor greenColor];
profileVC.title = @"我";
[self addChildViewController:profileVC];
}
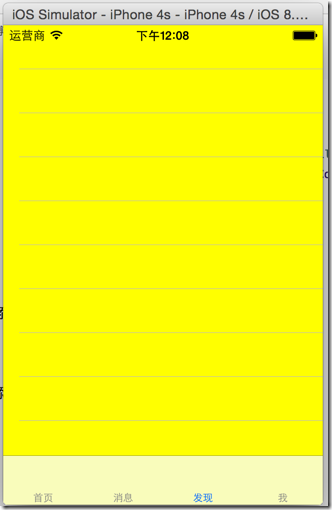
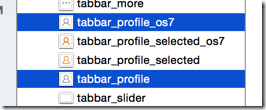
#ifndef HVWWeibo_HVWWeibo_Prefix_pch
#define HVWWeibo_HVWWeibo_Prefix_pch // Include any system framework and library headers here that should be included in all compilation units.
// You will also need to set the Prefix Header build setting of one or more of your targets to reference this file. // 判别是否iOS7或以上版本系统
#define iOS7 ([UIDevice currentDevice].systemVersion.doubleValue >= 7.0) #endif
#import "UIImage+Extension.h" @implementation UIImage (Extension) + (UIImage *) imageWithNamed:(NSString *) imageName {
UIImage *image = nil; // 如果是iOS7或以上版本
if (iOS7) {
image = [UIImage imageNamed:[NSString stringWithFormat:@"%@_os7", imageName]];
} // 如果是iOS6
if (nil == image) {
image = [UIImage imageNamed:imageName];
} return image;
} @end
#import "HVWTabBarViewController.h"
#import "HVWHomeViewController.h"
#import "HVWMessageViewController.h"
#import "HVWDiscoverViewController.h"
#import "HVWProfileViewController.h"
#import "UIImage+Extension.h" @interface HVWTabBarViewController () @end @implementation HVWTabBarViewController - (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view. // 添加子控制器
// 首页
HVWHomeViewController *homeVC = [[HVWHomeViewController alloc] init];
[self addChildViewController:homeVC WithTitle:@"首页" image:@"tabbar_home" seletectedImage:@"tabbar_home_selected"]; // 消息
HVWMessageViewController *messageVC = [[HVWMessageViewController alloc] init];
[self addChildViewController:messageVC WithTitle:@"消息" image:@"tabbar_message_center" seletectedImage:@"tabbar_message_center_selected"]; // 发现
HVWDiscoverViewController *discoverVC = [[HVWDiscoverViewController alloc] init];
[self addChildViewController:discoverVC WithTitle:@"发现" image:@"tabbar_discover" seletectedImage:@"tabbar_discover_selected"]; // 我
HVWProfileViewController *profileVC = [[HVWProfileViewController alloc] init];
[self addChildViewController:profileVC WithTitle:@"我" image:@"tabbar_profile" seletectedImage:@"tabbar_profile_selected"]; } /** 添加tab子控制器 */
- (void) addChildViewController:(UIViewController *) viewController WithTitle:(NSString *) title image:(NSString *) imageName seletectedImage:(NSString *) selectedImageName { // 设置随机背景色
viewController.view.backgroundColor = [UIColor colorWithRed:arc4random_uniform()/255.0 green:arc4random_uniform()/255.0 blue:arc4random_uniform()/255.0 alpha:1.0]; // 设置标题
viewController.title = title;
// 设置图标
viewController.tabBarItem.image = [UIImage imageWithNamed:imageName]; // 被选中时图标
UIImage *selectedImage = [UIImage imageWithNamed:selectedImageName];
// 如果是iOS7,不要渲染被选中的tab图标(iOS7中会自动渲染成为蓝色)
if (iOS7) {
selectedImage = [selectedImage imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
}
viewController.tabBarItem.selectedImage = selectedImage; // 添加子控制器
[self addChildViewController:viewController];
} @end
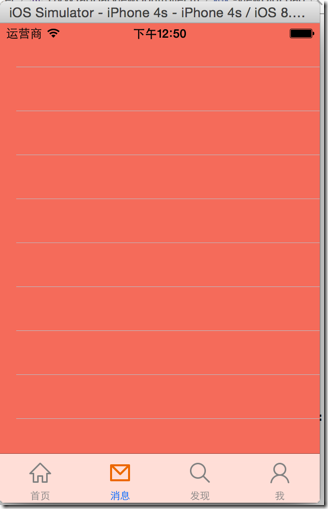
/** 添加tab子控制器 */
- (void) addChildViewController:(UIViewController *) viewController WithTitle:(NSString *) title image:(NSString *) imageName seletectedImage:(NSString *) selectedImageName { // 设置随机背景色
viewController.view.backgroundColor = [UIColor colorWithRed:arc4random_uniform()/255.0 green:arc4random_uniform()/255.0 blue:arc4random_uniform()/255.0 alpha:1.0]; // 设置标题,直接设置title可以同时设置tabBarItem和navigationItem的title
// viewController.tabBarItem.title = title;
// viewController.navigationItem.title = title;
viewController.title = title; // 设置图标
viewController.tabBarItem.image = [UIImage imageWithNamed:imageName]; // 被选中时图标
UIImage *selectedImage = [UIImage imageWithNamed:selectedImageName];
// 如果是iOS7,不要渲染被选中的tab图标(iOS7中会自动渲染成为蓝色)
if (iOS7) {
selectedImage = [selectedImage imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
}
viewController.tabBarItem.selectedImage = selectedImage; // 添加子控制器
UINavigationController *nav = [[UINavigationController alloc] initWithRootViewController:viewController];
[self addChildViewController:nav];
}
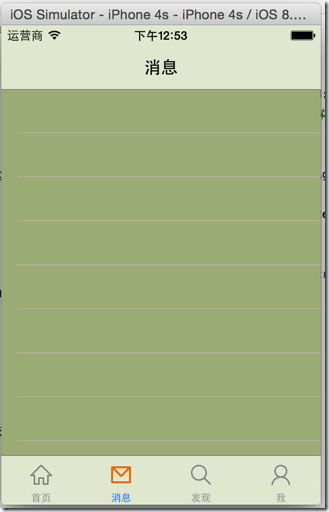

#import "HVWNavigationViewController.h" @interface HVWNavigationViewController () @end @implementation HVWNavigationViewController - (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
} - (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
} /** 重写push方法 */
- (void)pushViewController:(UIViewController *)viewController animated:(BOOL)animated {
// 如果不是根控制器,隐藏TabBar
if (self.viewControllers.count > ) {
// 注意这里不是self(navigationController),是push出来的ViewContoller隐藏TabBar
viewController.hidesBottomBarWhenPushed = YES;
} // 最后一定要调用父类的方法
[super pushViewController:viewController animated:animated];
} @end
// HVWWeibo-Prefix.pch
#ifndef HVWWeibo_HVWWeibo_Prefix_pch
#define HVWWeibo_HVWWeibo_Prefix_pch // Include any system framework and library headers here that should be included in all compilation units.
// You will also need to set the Prefix Header build setting of one or more of your targets to reference this file. #ifdef __OBJC__
#import <UIKit/UIKit.h>
#import <Foundation/Foundation.h>
#import "UIImage+Extension.h"
#endif // 测试用log
#ifdef DEBUG
#define HVWLog(...) NSLog(__VA_ARGS__)
#else
#define HVWLog(...)
#endif // 判别是否iOS7或以上版本系统
#define iOS7 ([UIDevice currentDevice].systemVersion.doubleValue >= 7.0) // 随机颜色
#define RandomColor [UIColor colorWithRed:arc4random_uniform(256)/255.0 green:arc4random_uniform(256)/255.0 blue:arc4random_uniform(256)/255.0 alpha:1.0] #endif
/** 寻找朋友按钮事件 */
- (void) searchFriend {
HVWLog(@"searchFriend");
}

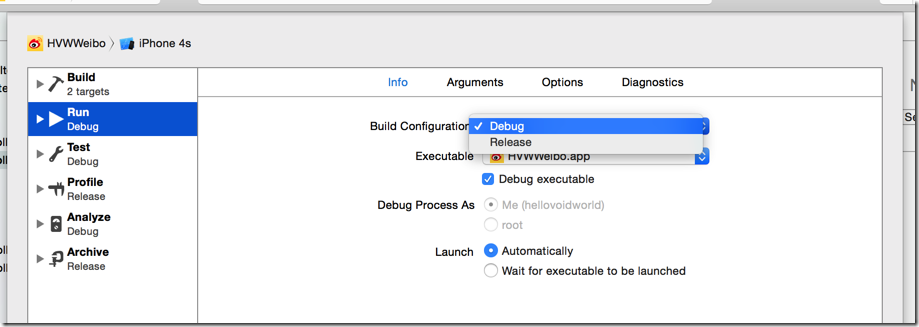
//
// UIBarButtonItem+Extension.m
// HVWWeibo
//
// Created by hellovoidworld on 15/1/31.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "UIBarButtonItem+Extension.h" @implementation UIBarButtonItem (Extension) + (instancetype) itemWithImage:(NSString *) imageName hightlightedImage:(NSString *) highlightedImageName target:(id)target selector:(SEL)selector {
UIBarButtonItem *item = [[self alloc] init]; // 创建按钮
UIButton *button = [UIButton buttonWithType:UIButtonTypeCustom];
UIImage *image = [UIImage imageNamed:imageName];
[button setImage:image forState:UIControlStateNormal];
[button setImage:[UIImage imageNamed:highlightedImageName] forState:UIControlStateHighlighted]; // 一定要设置frame,才能显示
button.frame = CGRectMake(, , image.size.width, image.size.height); // 设置事件
[button addTarget:target action:selector forControlEvents:UIControlEventTouchUpInside]; item.customView = button;
return item;
} @end
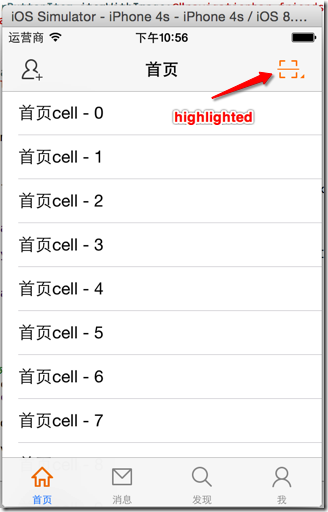
// HVWHomeViewController.m
- (void)viewDidLoad {
[super viewDidLoad]; // 添加导航控制器按钮
// 左边按钮
self.navigationItem.leftBarButtonItem = [HVWBarButtonItem itemWithImage:@"navigationbar_friendsearch" hightlightedImage:@"navigationbar_friendsearch_highlighted" target:self selector:@selector(searchFriend)]; // 右边按钮
self.navigationItem.rightBarButtonItem = [HVWBarButtonItem itemWithImage:@"navigationbar_pop" hightlightedImage:@"navigationbar_pop_highlighted" target:self selector:@selector(pop)];
} /** 左边导航栏按钮事件 */
- (void) searchFriend {
HVWLog(@"searchFriend");
} /** 右边导航栏按钮事件 */
- (void) pop {
HVWLog(@"pop");
}
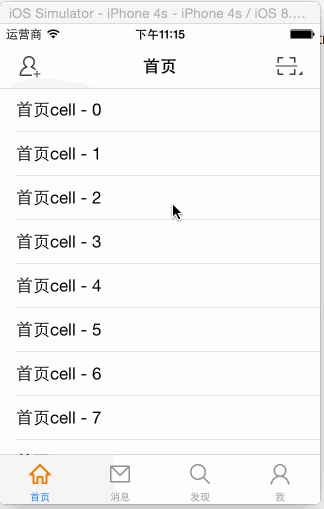
// HVWNavigationViewController.m
/** 重写push方法 */
- (void)pushViewController:(UIViewController *)viewController animated:(BOOL)animated {
// 如果不是根控制器,隐藏TabBar
if (self.viewControllers.count > ) {
// 注意这里不是self(navigationController),是push出来的ViewContoller隐藏TabBar
viewController.hidesBottomBarWhenPushed = YES; // 加上“返回上一层”按钮和“直接回到根控制器”按钮
viewController.navigationItem.leftBarButtonItem = [UIBarButtonItem itemWithImage:@"navigationbar_back" hightlightedImage:@"navigationbar_back_highlighted" target:self selector:@selector(back)]; viewController.navigationItem.rightBarButtonItem = [UIBarButtonItem itemWithImage:@"navigationbar_more" hightlightedImage:@"navigationbar_more_highlighted" target:self selector:@selector(more)];
} // 最后一定要调用父类的方法
[super pushViewController:viewController animated:animated];
} /** 返回上一层 */
- (void) back {
[self popViewControllerAnimated:YES];
} /** 返回根控制器 */
- (void) more {
[self popToRootViewControllerAnimated:YES];
}
[iOS微博项目 - 1.0] - 搭建基本框架的更多相关文章
- [iOS微博项目 - 3.0] - 手动刷新微博
github: https://github.com/hellovoidworld/HVWWeibo A.下拉刷新微博 1.需求 在“首页”界面,下拉到一定距离的时候刷新微博数据 刷新数据的时候使 ...
- [iOS微博项目 - 2.0] - OAuth授权3步
A.概念 OAUTH协议为用户资源的授权提供了一个安全的.开放而又简易的标准.与以往的授权方式不同之处是OAUTH的授权不会使第三方触及到用户的帐号信息(如用户名与密码),即第三方无需使用用 ...
- [iOS微博项目 - 4.0] - 自定义微博cell
github: https://github.com/hellovoidworld/HVWWeibo A.自定义微博cell基本结构 1.需求 创建自定义cell的雏形 cell包含:内容.工具条 内 ...
- 手动从0搭建ABP框架-ABP官方完整解决方案和手动搭建简化解决方案实践
本文主要讲解了如何把ABP官方的在线生成解决方案运行起来,并说明了解决方案中项目间的依赖关系.然后手动实践了如何从0搭建了一个简化的解决方案.ABP官方的在线生成解决方案源码下载参考[3],手动搭 ...
- [iOS微博项目 - 2.6] - 获取微博数据
github: https://github.com/hellovoidworld/HVWWeibo A.新浪获取微博API 1.读取微博API 2.“statuses/home_time ...
- [iOS微博项目 - 3.2] - 发送微博
github: https://github.com/hellovoidworld/HVWWeibo A.使用微博API发送微博 1.需求 学习发送微博API 发送文字微博 发送带有图片的微博 ...
- [iOS微博项目 - 3.1] - 发微博界面
github: https://github.com/hellovoidworld/HVWWeibo A.发微博界面:自定义UITextView 1.需求 用UITextView做一个编写微博的输 ...
- [iOS微博项目 - 1.7] - 版本新特性
A.版本新特性 1.需求 第一次使用新版本的时候,不直接进入app,而是展示新特性界面 github: https://github.com/hellovoidworld/HVWWeibo ...
- [iOS微博项目 - 1.1] - 设置导航栏主题(统一样式)
A.导航栏两侧文字按钮 1.需求: 所有导航栏两侧的文字式按钮统一样式 普通样式:橙色 高亮样式:红色 不可用样式:亮灰 阴影:不使用 字体大小:15 github: https://github ...
随机推荐
- java_十进制数转换为二进制,八进制,十六进制数的算法
java_十进制数转换为二进制,八进制,十六进制数的算法 java Ê®½øÖÆÊýת»»Îª¶þ½øÖÆ,°Ë½øÖÆ,Ê®Áù½øÖÆÊýµÄË㕨 using System; using S ...
- makefile的常用规则
一.前言 这篇文章介绍在LINUX下进行C语言编程所需要的基础知识.在这篇文章当中,我们将会学到以下内容: 源程序编译 Makefile的编写 程序库的链接 程序的调试 头文件和系统求助 二.正文 1 ...
- Codeforces Round #242 (Div. 2) C. Magic Formulas (位异或性质 找规律)
题目 比赛的时候找出规律了,但是找的有点慢了,写代码的时候出了问题,也没交对,还掉分了.... 还是先总结一下位移或的性质吧: 1. 交换律 a ^ b = b ^ a 2. 结合律 (a^b) ^ ...
- 宏os_file_read_func
# define os_file_read(file, buf, offset, offset_high, n) \ os_file_read_func(file, buf, offset, offs ...
- 由于管理员设置的策略,该磁盘处于脱机状态-Win 2008 R2
问题截图: 做了个小说网站www.114369.cn,使用的是云主机,系统是Win 2008 R2,进入服务器后发现磁盘有问题 只有c盘,没有d盘,提示:由于管理员设置的策略,该磁盘处于脱机状态 解决 ...
- OK335xS PMIC(TPS65910A3A1RSL) reset
/*********************************************************************** * OK335xS PMIC(TPS65910A3A1 ...
- Java [Leetcode 326]Power of Three
题目描述: Given an integer, write a function to determine if it is a power of three. Follow up:Could you ...
- 01day1
最大音量 动态规划 题意:给出一个初始值和一个变化序列 c,在第 i 步可以加上或减去 c[i],求 n 步之后能达到的最大值.有一个限定值 maxlevel,在变化过程中值不能超过 maxlevel ...
- border-radius 在安卓手机竟然不完美支持
如果给图片加了width:50px;height:50px;border-radius:25px;-webkit-border-radius:25px;border:3px solid #fff; 在 ...
- MySQL5.6 replication architecture --原图来自姜承尧