Fire!(BFS)
Description
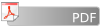
Problem B: Fire!
Joe works in a maze. Unfortunately, portions of the maze have caught on fire, and the owner of the maze neglected to create a fire escape plan. Help Joe escape the maze.
Given Joe's location in the maze and which squares of the maze are on fire, you must determine whether Joe can exit the maze before the fire reaches him, and how fast he can do it.
Joe and the fire each move one square per minute, vertically or horizontally (not diagonally). The fire spreads all four directions from each square that is on fire. Joe may exit the maze from any square that borders the edge of the maze. Neither Joe nor the fire may enter a square that is occupied by a wall.
Input Specification
The first line of input contains a single integer, the number of test cases to follow. The first line of each test case contains the two integers R and C, separated by spaces, with 1 <= R, C <= 1000. The following R lines of the test case each contain one row of the maze. Each of these lines contains exactly C characters, and each of these characters is one of:
- #, a wall
- ., a passable square
- J, Joe's initial position in the maze, which is a passable square
- F, a square that is on fire
There will be exactly one J in each test case.
#include <iostream>
#include <algorithm>
#include <queue>
#include <vector>
#include <utility>
#include <cstdio>
#include <cstring> using namespace std; struct Pos
{
int y, x, step;
Pos(int a, int b, int c) {y = a; x = b; step = c;}
};
int r, c, fi, fj, ji, jj;
char m[][];
queue<Pos> que;
int fx[], fy[], top, tail; const int d[][] = {{, }, {, -}, {, }, {-, }}; bool Judge(int i, int j)
{
return i > - && j > - && i < r && j < c && m[i][j] == '.';
} bool Judge2(int i, int j)
{
return i > - && j > - && i < r && j < c && m[i][j] != '#' && m[i][j] != 'F';
} bool isExit(int i, int j)
{
return i == || j == || i == r - || j == c - ;
} void fire_spread()
{
int y, x, yy, xx, end = tail;
for(; top < end; top++){
y = fy[top];
x = fx[top];
for(int i = ; i < ; i++){
yy = y + d[i][];
xx = x + d[i][];
if(Judge2(yy, xx)){
m[yy][xx] = 'F';
fx[tail] = xx;
fy[tail] = yy;
tail++;
}
}
}
} int main()
{
int t;
scanf("%d", &t);
while(t--){
bool ok = false;
top = tail = ;
while(!que.empty()) que.pop();
scanf("%d %d", &r, &c);
for(int i = ; i < r; i++){
scanf("%s", m[i]);
for(int j = ; m[i][j] != '\0'; j++){
if(m[i][j] == 'J') que.push(Pos(i, j, ));
else if(m[i][j] == 'F') fy[tail] = i, fx[tail] = j, tail++;
}
}
int pre = ;
fire_spread();
while(!que.empty()){
Pos cur = que.front();
que.pop();
if(isExit(cur.y, cur.x)){
pre = cur.step + ;
ok = true;
break;
}
if(cur.step > pre) {
fire_spread();
pre++;
}
for(int i = ; i < ; i++){
int ii = cur.y + d[i][];
int jj = cur.x + d[i][];
if(Judge(ii, jj)) {
m[ii][jj] = 'P';
que.push(Pos(ii, jj, cur.step + ));
}
}
}
if(!ok) puts("IMPOSSIBLE");
else printf("%d\n", pre);
}
return ;
}
Fire!(BFS)的更多相关文章
- UVA 11624 Fire! (bfs)
算法指南白书 分别求一次人和火到达各个点的最短时间 #include<cstdio> #include<cstring> #include<queue> #incl ...
- UVA - 11624 Fire! bfs 地图与人一步一步先后搜/搜一次打表好了再搜一次
UVA - 11624 题意:joe在一个迷宫里,迷宫的一些部分着火了,火势会向周围四个方向蔓延,joe可以向四个方向移动.火与人的速度都是1格/1秒,问j能否逃出迷宫,若能输出最小时间. 题解:先考 ...
- UVA11624 Fire! —— BFS
题目链接:https://vjudge.net/problem/UVA-11624 题解: 坑点:“portions of the maze havecaught on fire”, 表明了起火点不唯 ...
- UVA 11624 Fire! BFS搜索
题意:就是问你能不能在火烧到你之前,走出一个矩形区域,如果有,求出最短的时间 分析:两遍BFS,然后比较边界 #include<cstdio> #include<algorithm& ...
- UVA 11624 Fire! bfs 难度:0
http://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem&p ...
- ACM: FZU 2150 Fire Game - DFS+BFS+枝剪 或者 纯BFS+枝剪
FZU 2150 Fire Game Time Limit:1000MS Memory Limit:32768KB 64bit IO Format:%I64d & %I64u ...
- UVa 11624 Fire!(BFS)
Fire! Time Limit: 5000MS Memory Limit: 262144KB 64bit IO Format: %lld & %llu Description Joe ...
- foj 2150 Fire Game(bfs暴力)
Problem Description Fat brother and Maze are playing a kind of special (hentai) game on an N*M ...
- fzu 2150 Fire Game 【身手BFS】
称号:fzupid=2150"> 2150 Fire Game :给出一个m*n的图,'#'表示草坪,' . '表示空地,然后能够选择在随意的两个草坪格子点火.火每 1 s会向周围四个 ...
随机推荐
- 编码剖析Spring管理bean的原理
project目录 MyClassPathXMLApplicationContext读取xml,以及实例化bean. 因为是一开始实例化配置文件所有bean,所以需要构造器完成这些工作. packag ...
- 有助于提高你的 Web 开发技能的7个模式库
正如语言风格指南一样,模式库有两个主要用途.首先,是它们提供了一组编码或设计标准,Web 开发团队可以在整个网站中应用,有助于保持一致的编码实践和外观:其次,对于要学习网页设计最佳实践来说是宝贵的财富 ...
- atitit.js 各版本 and 新特性跟浏览器支持报告
atitit.js 各版本 and 新特性跟浏览器支持报告 一个完整的JavaScript实现是由以下3个不同部分组成的 •核心(ECMAScript)--JavaScript的核心ECMAScrip ...
- 使用Alcatraz来管理Xcode插件
Alcatraz 是一个帮你管理 Xcode 插件.模版以及颜色配置的工具.它可以直接集成到 Xcode 的图形界面中,让你感觉就像在使用 Xcode 自带的功能一样. 安装和删除 使用如下的命令行来 ...
- 单元测试之NSNull 检测
本文主要讲 单元测试之NSNull 检测,在现实开发中,我们最烦的往往就是服务端返回的数据中隐藏着NSNull的数据,一般我们的做法是通过[data isKindOfClass:[NSNull cla ...
- C#:Oracle数据库带参PLSQL语句的正确性验证
在有Oracle数据库C#项目中,有一个这样的需求:在界面上配置了带参数的PLSQL语句,但是要通过程序验证其正确性,那么该如何实现?这就是本文要探讨的内容. 一:通过OracleCommand对象的 ...
- 一些新的web性能优化技术
1.IconFont:图标字体,这是近年来新流行的一种以字体代替图片的技术.它可以适应任何分辨率而不会出现图片模糊问题,与图片相比它具有更小的容量,更高的灵活性(像字体一样可以设置图标大小.颜色.透明 ...
- QT on Android开发
1.安装QT 2.安装JDK 配置如下系统环境变量: JAVA_HOME D:\Java\jdk Path %JAVA_HOME%\bin;%JAVA_HOME%\jre\bin CLASSPATH ...
- ASP.NET MVC中实现多个按钮提交的几种方法
有时候会遇到这种情况:在一个表单上需要多个按钮来完成不同的功能,比如一个简单的审批功能. 如果是用webform那不需要讨论,但asp.net mvc中一个表单只能提交到一个Action处理,相对比较 ...
- 新版PHP 7效能實測:Drupal 7能快70%,碎形計算大勝Ruby和Python
PHP 7才剛在12月3日正式釋出,網頁開發框架Zend公司立刻發表了一份PHP新舊版效能大車拼報告,除了PHP 7和PHP 5.6之外,也把HHVM 3.7版納入一起比較. Zend公司選擇了幾套知 ...