elementUI图片墙上传
elementUI提供了照片墙上传的功能,我们直接拿来用。
以下是实现代码:
<template>
<div style="padding: 50px;">
<el-form class="form-wrapper padding" ref="addForm" :model="addForm" :rules="addRules" label-width="110px">
<el-form-item label="活动图片:" prop="photo">
<el-upload
:action="base"
multiple
accept="image/png, image/jpeg"
list-type="picture-card"
:before-upload="beforeUploadPicture"
:on-preview="handlePictureCardPreview"
:on-progress="uploadProgress"
:on-remove="handleRemove"
:on-success="uploadSuccess"
:on-error="uploadError"
:show-file-list="true">
<i class="el-icon-plus"></i>
</el-upload>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="addEnsure">保存</el-button>
</el-form-item>
</el-form>
<el-dialog class="preview-modal" :visible.sync="imgVisible" append-to-body>
<img width="100%" :src="dialogImageUrl" alt="photo">
</el-dialog>
</div>
</template>
<script type="text/ecmascript-6">
import base from 'api/env' // 配置了图片上传接口地址的js文件
export default {
data() {
return {
addForm: {
photo: '' // 活动图片
},
addRules: { // 表单验证规则
photo: [{required: true, message: '请上传活动图片', trigger: 'blur'}]
},
uploadComplete: true, // 图片上传完成状态
base: base.imgURL + 'upload/img',
imgVisible: false, // 上传图片预览
dialogImageUrl: '' // 图片预览地址
}
},
created() {
this.initForm();
},
methods: {
initForm() {
if(this.$refs.addForm){
this.$refs.addForm.resetFields();
}
},
// 上传图片前调用方法
beforeUploadPicture(file) {
if(file.size > 10*1024*1024){
this.$message.error("上传图片不能大于10M");
return false;
}
},
// 上传图片时调用
uploadProgress(event,file, fileList){
this.uploadComplete = false;
},
// 上传图片成功
uploadSuccess(res, file, fileList) {
this.uploadComplete = true;
this.fileChange(fileList);
},
// 上传图片出错
uploadError(err, file, fileList) {
this.$message.error("上传出错");
},
// 移除图片
handleRemove(file, fileList) {
this.fileChange(fileList);
},
// 设置photo值
fileChange(fileList) {
let temp_str = '';
if(fileList.length > 0){
for(let i=0; i<fileList.length; i++){
if(fileList[i].response){
if(fileList[i].response.code === 0){
if(i===0){
temp_str += fileList[i].response.data;
} else {
// 最终photo的格式是所有已上传的图片的url拼接的字符串(逗号隔开)
temp_str += ',' + fileList[i].response.data;
}
}
}
}
}
this.addForm.photo = temp_str;
},
// 图片预览
handlePictureCardPreview(file) {
this.dialogImageUrl = file.url;
this.imgVisible = true;
},
// 确认添加
addEnsure(){
if(!this.uploadComplete){
this.$message.error("图片正在上传,请稍等");
return;
}
this.$refs.addForm.validate((valid) => {
if(valid){
let params = {
photo: this.addForm.photo,
};
console.info(params);
// 调用接口... } else {
this.$message.error("请填写所有必填项");
}
});
}
}
}
</script>
效果图:
<template>
<div style="padding: 50px;">
<el-form class="form-wrapper padding" ref="editForm" :model="editForm" :rules="editRules" label-width="110px">
<el-form-item label="活动图片:" prop="photo">
<el-upload
:action="base"
multiple
accept="image/png, image/jpeg"
list-type="picture-card"
:on-preview="handlePictureCardPreview"
:on-remove="handleRemove"
:on-progress="uploadProgress"
:on-success="uploadSuccess"
:on-error="uploadError"
:file-list="editFiles"
:show-file-list="true">
<i class="el-icon-plus"></i>
</el-upload>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="editEnsure">保存</el-button>
</el-form-item>
</el-form>
<el-dialog class="preview-modal" :visible.sync="imgVisible" append-to-body>
<img width="100%" :src="dialogImageUrl" alt="photo">
</el-dialog>
</div>
</template>
<script type="text/ecmascript-6">
import base from 'api/env' // 配置了图片上传接口地址的js文件
export default {
data() {
return {
editForm: { // 编辑表单
photo: '' // 活动图片
},
editRules: { // 表单验证规则
photo: [{required: true, message: '请上传活动图片', trigger: 'blur'}]
},
editFiles: [],// 编辑时已上传图片初始化
uploadComplete: true,
base: base.imgURL + 'upload/img',
imgVisible: false, // 上传图片预览
dialogImageUrl: '' // 图片预览地址
}
},
created() {
this.initInfo();
},
methods: {
// 编辑
initInfo() {
this.editForm = {
id: 1,
photo: ''
};
// 这里photo应从服务器获取,存储的是数组,请按照相应格式获取图片url(这里直接给值)
let temp = [
{id: 123, photo: 'http://img4.imgtn.bdimg.com/it/u=2011641246,1136238184&fm=27&gp=0.jpg'},
{id: 124, photo: 'http://img2.imgtn.bdimg.com/it/u=302701032,2300144492&fm=27&gp=0.jpg'}
];
if(temp.length > 0){
for(let t=0; t<temp.length; t++){
//通过[{name: 'name', url: 'url地址'}]格式初始化照片墙
this.editFiles.push({name: 'name' + temp[t].id, url: temp[t].photo});
if(t===0){
this.editForm.photo += temp[t].photo
} else {
// 最终photo的格式是所有已上传的图片的url拼接的字符串(逗号隔开),根据实际需要修改格式
this.editForm.photo += ',' + temp[t].photo;
}
}
}
this.editVisible = true;
},
// 确认修改
editEnsure() {
if(!this.uploadComplete){
this.$message.error("图片正在上传,请稍等");
return;
}
console.info(this.editForm.photo);
// 调用接口...
},
// 上传图片前调用方法
beforeUploadPicture(file) {
if(file.size > 10*1024*1024){
this.$message.error("上传图片不能大于10M");
return false;
}
},
// 上传图片时调用
uploadProgress(event,file, fileList){
this.uploadComplete = false;
},
// 上传图片成功
uploadSuccess(res, file, fileList) {
this.uploadComplete = true;
this.fileChange(fileList);
},
// 上传图片出错
uploadError(err, file, fileList) {
this.$message.error("上传出错");
},
// 移除图片
handleRemove(file, fileList) {
this.fileChange(fileList);
},
// 设置photo值
fileChange(fileList) {
let temp_str = '';
if(fileList.length > 0){
for(let i=0; i<fileList.length; i++){
if(fileList[i].response){
if(fileList[i].response.code === 0){
if(i===0){
temp_str += fileList[i].response.data;
} else {
temp_str += ',' + fileList[i].response.data;
}
}
} else if(fileList[i].status && fileList[i].status === 'success'){
if(i===0){
temp_str += fileList[i].url;
} else {
temp_str += ',' + fileList[i].url;
}
}
}
}
this.editForm.photo = temp_str;
},
// 图片预览
handlePictureCardPreview(file) {
this.dialogImageUrl = file.url;
this.imgVisible = true;
}
}
}
</script>
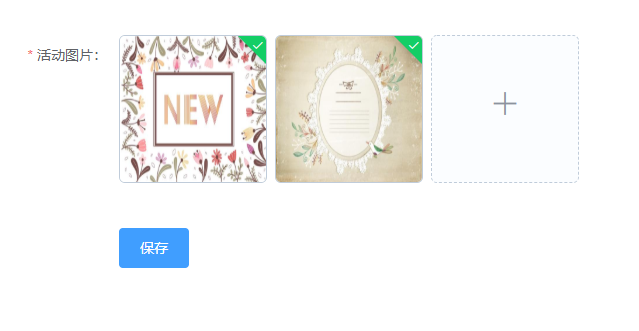
接下来就可以继续愉快地上传图片啦。
elementUI图片墙上传的更多相关文章
- vue+elementUI 图片上传问题
图片上传问题,获取后台的图片,并点击可以更换图片,并把图片存储到数据库中: (1)在编辑页面上,action指的图片上传的地址,header指请求头: (2)因为element-ui有自己上传的接口, ...
- elementUI 图片上传限制上传图片的宽高
文件上传,需当上传的文件类型为图片的时候,需要限制图片的宽高. 此处采用了new Promise异步加载的方式,等图片上传加载完成后, 页面代码: <el-form-item label=&qu ...
- 如何用elementui去实现图片上传和表单提交,用axios的post方法
下面是在vue搭建的脚手架项目中的组件component文件夹下面的upload.vue文件中的内容 <!--这个组件主要用来研究upload这个elementui的上传插件组件--> & ...
- 后台管理系统之“图片上传” --vue
图片上传(基于vue) 相信上传图片是所有系统必备的功能吧,工作中的第一个管理系统就在上传图片的功能上卡顿了一整天. 当时用的elementUI组件,但是由于样式和设计图样式差别较大再加上原生相较好理 ...
- 用Vue来实现图片上传多种方式
没有业务场景的功能都是耍流氓,那么我们先来模拟一个需要实现的业务场景.假设我们要做一个后台系统添加商品的页面,有一些商品名称.信息等字段,还有需要上传商品轮播图的需求. 我们就以Vue.Element ...
- vue之element-ui文件上传
vue之element-ui文件上传 文件上传需求 对于文件上传,实际项目中我们的需求一般分两种: 对于单个的文件上传,比如拖动上传个图片之类的,或者是文件. 和表单一起实现上传(这种情况一般都是 ...
- # quill-image-extend-module :实现vue-quill-editor图片上传,复制粘贴,拖拽
改造vue-quill-editor: 结合element-ui上传图片到服务器 quill-image-extend-module vue-quill-editor的增强模块, 功能: 提供图片上传 ...
- vue quill使用&quill 自定义图片上传&自定义mp4 更换标签
pluins 创建quill 目录 创建文件video.js import { Quill } from 'vue-quill-editor' // 源码中是import直接倒入,这里要用Quill. ...
- vue+axios+elementUI文件上传与下载
vue+axios+elementUI文件上传与下载 Simple_Learn 关注 0.5 2018.05.30 10:20 字数 209 阅读 15111评论 4喜欢 6 1.文件上传 这里主要 ...
随机推荐
- Flutter 页面布局 Stack层叠组件
Stack 表示堆的意思,我们可以用 Stack 或者 Stack 结合 Align 或者 Stack 结合 Positiond 来实现页面的定位布局 属性 说明 alignment 配置所有子元素的 ...
- openresty开发系列26--openresty中使用redis模块
openresty开发系列26--openresty中使用redis模块 在一些高并发的场景中,我们常常会用到缓存技术,现在我们常用的分布式缓存redis是最知名的, 操作redis,我们需要引入re ...
- SeetaFaceDetection识别人脸
SeetaFaceDetection识别人脸 #pragma warning(disable: 4819) #include <seeta/FaceEngine.h> #include & ...
- Spring cloud微服务安全实战-5-8实现基于session的SSO(认证服务器的session有效期)
认证服务器 session的有效期. 也就是认证服务器上的session的有效期 生成环境下,认证服务器一定是一个集群.集群.那么session一定是要在所有的服务器之间进行共享的.最简单的方式是用S ...
- ES6深入浅出-3 三个点运算 & 新版字符串-2. 新版字符串
这是以前的字符串..双引号,单引号.毫无区别 有时候在字符串里面写一些标签. 排版不好看 我就想回车一下.这样写虽然是好看.但是语法就报错了.es5的字符串不支持换行.我只想是想让它排版的好看一点. ...
- MyBatis-Spring项目
使用Spring IoC可以有效管理各类Java资源,达到即插即拔功能:通过AOP框架,数据库事务可以委托给Spring处理,消除很大一部分的事务代码,配合MyBatis的高灵活.可配置.可优化SQL ...
- bootstrap-table和bootstrap-switch
{% load staticfiles %} <!DOCTYPE html> <html lang="en"> <head> <meta ...
- 服务发现框架选型,Consul还是Zookeeper还是etcd
背景 本文并不介绍服务发现的基本原理.除了一致性算法之外,其他并没有太多高深的算法,网上的资料很容易让大家明白上面是服务发现. 想直接查看结论的同学,请直接跳到文末. 目前,市面上有非常多的服务发现工 ...
- MySQL 过滤复制+复制映射 配置方法
场景 node1 和 node2 为两台不同业务的MySQL服务器.业务方有个需求,需要将node1上的 employees库的departments .dept_manager 这2张表同步到 no ...
- python线程队列Queue-FIFO(35)
之前的文章中讲解很多关于线程间通信的知识,比如:线程互斥锁lock,线程事件event,线程条件变量condition 等等,这些都是在开发中经常使用的内容,而今天继续给大家讲解一个更重要的知识点 — ...