poj2676 (dfs+回溯)
Time Limit: 2000MS | Memory Limit: 65536K | |||
Total Submissions: 24108 | Accepted: 11259 | Special Judge |
Description
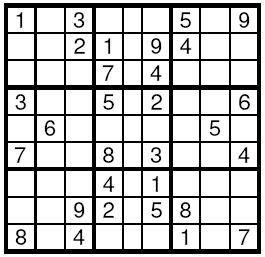
Input
Output
Sample Input
1
103000509
002109400
000704000
300502006
060000050
700803004
000401000
009205800
804000107
Sample Output
143628579
572139468
986754231
391542786
468917352
725863914
237481695
619275843
854396127
Source
#include<iostream>
#include<cstdio>
#include<cstring>
#include<string>
#include<cstdlib>
#include<cmath>
#include<algorithm>
#include<vector>
#include<queue>
#include<stack>
#define Max(a,b) ((a)>(b)?(a):(b))
#define Min(a,b) ((a)<(b)?(a):(b))
#define Swap(a,b,t) t=a,a=b,b=t
#define Mem0(x) memset(x,0,sizeof(x))
#define Mem1(x) memset(x,-1,sizeof(x))
#define MemX(x) memset(x,0x3f,sizeof(x));
using namespace std;
typedef long long ll;
const int inf=0x3f3f3f;
const double eps=1e-12;
const int MAX=15;
int map[MAX][MAX];
char temp[MAX];
bool row[MAX][MAX],line[MAX][MAX],rl[MAX][MAX],flag=false;
void dfs(int x,int y)
{
if (x==10){
flag=true;
return ;
}
if (map[x][y]){
if (y==9)
dfs(x+1,1);
else
dfs(x,y+1);
if (flag)
return ;
}
else{
int k=3*((x-1)/3)+(y-1)/3+1;
for (int i=1;i<10;i++){
if (!row[x][i]&&!line[y][i]&&!rl[k][i]){
map[x][y]=i;
row[x][i]=line[y][i]=rl[k][i]=true;
if (y==9)
dfs(x+1,1);
else
dfs(x,y+1);
if(flag)
return ;
map[x][y]=0;
row[x][i]=line[y][i]=rl[k][i]=false;
}
}
}
}
int main()
{
int t;
cin>>t;
while (t--){
flag=false;
memset(map,0,sizeof(map));
memset(row,false,sizeof(row));
memset(line,false,sizeof(line));
memset(rl,false,sizeof(rl));
for (int i=1;i<10;i++){
cin>>temp+1;
for (int j=1;j<10;j++){
map[i][j]=temp[j]-'0';
if (map[i][j]){
int k=3*((i-1)/3)+(j-1)/3+1;
row[i][map[i][j]]=line[j][map[i][j]]=rl[k][map[i][j]]=true;
}
}
}
dfs(1,1);
for (int i=1;i<10;i++){
for (int j=1;j<10;j++){
printf("%d",map[i][j]);
}
printf("\n");
}
}
return 0;
}
poj2676 (dfs+回溯)的更多相关文章
- 素数环(dfs+回溯)
题目描述: 输入正整数n,把整数1,2...n组成一个环,使得相邻两个数和为素数.输出时从整数1开始逆时针排列并且不能重复: 例样输入: 6 例样输出: 1 4 3 2 5 6 1 6 5 2 3 4 ...
- NOJ 1074 Hey Judge(DFS回溯)
Problem 1074: Hey Judge Time Limits: 1000 MS Memory Limits: 65536 KB 64-bit interger IO format: ...
- HDU 1016 Prime Ring Problem(经典DFS+回溯)
Prime Ring Problem Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- HDU 2181 哈密顿绕行世界问题(经典DFS+回溯)
哈密顿绕行世界问题 Time Limit: 3000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total ...
- HDU1016 Prime Ring Problem(DFS回溯)
Prime Ring Problem Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Other ...
- uva 193 Graph Coloring(图染色 dfs回溯)
Description You are to write a program that tries to find an optimal coloring for a given graph. Col ...
- P1074 靶形数独 dfs回溯法
题目描述 小城和小华都是热爱数学的好学生,最近,他们不约而同地迷上了数独游戏,好胜的他们想用数独来一比高低.但普通的数独对他们来说都过于简单了,于是他们向 Z 博士请教,Z 博士拿出了他最近发明的“靶 ...
- 剪格子---(dfs回溯)
如图p1.jpg所示,3 x 3 的格子中填写了一些整数. 我们沿着图中的红色线剪开,得到两个部分,每个部分的数字和都是60. 本题的要求就是请你编程判定:对给定的m x n 的格子中的整数,是否可以 ...
- 蓝桥杯 算法提高 8皇后·改 -- DFS 回溯
算法提高 8皇后·改 时间限制:1.0s 内存限制:256.0MB 问题描述 规则同8皇后问题,但是棋盘上每格都有一个数字,要求八皇后所在格子数字之和最大. 输入格式 一个8*8 ...
随机推荐
- su
参数选项:-,-l,--login 切换用户的同时,将用户的家目录.系统环境变量等重新按切换后的用户初始化.-c 向shell传递单个命令,仅希望在某个用户下执行命令,而不用直接切换到该用户下来操作. ...
- 智能指针之auto_ptr和scoped_ptr
部分参考地址https://blog.csdn.net/yanglingwell/article/details/56011576 auto_ptr是c++标准库里的智能指针,但是具有以下几个明显的缺 ...
- dba_tables、all_tables、user_tables
本文摘抄自:http://blog.csdn.net/daxiang12092205/article/details/42921063 dba_tables : 系统里所有的表的信息,需要DBA权限才 ...
- (一)自定义ViewGroup绘制出菜单
从网上学习了hyman大神的卫星菜单实现,自己特意亲自又写了一编代码,对自定义ViewGroup的理解又深入了一点.我坚信只有自己写出来的知识才会有更加好的的掌握.因此也在自己的博客中将这个卫星菜单的 ...
- chromedriver链接
http://npm.taobao.org/mirrors/chromedriver/
- BZOJ2194:快速傅立叶之二(FFT)
Description 请计算C[k]=sigma(a[i]*b[i-k]) 其中 k < = i < n ,并且有 n < = 10 ^ 5. a,b中的元素均为小于等于100的非 ...
- 解决hash冲突方法
转自:https://www.cnblogs.com/wuchaodzxx/p/7396599.html 目录 开放定址法 线性探测再散列 二次探测再散列 伪随机探测再散列 再哈希法 链地址法 建立公 ...
- [19/04/12-星期五] 多线程_任务定时调度(Timer、Timetask和QUARTZ)
一.Timer和Timetask 通过Timer和Timetask,我们可以实现定时启动某个线程. java.util.Timer 在这种实现方式中,Timer类作用是类似闹钟的功能,也就是定时或者每 ...
- 使用@AspectJ注解开发Spring AOP
一.实体类: Role public class Role { private int id; private String roleName; private String note; @Overr ...
- GetSystemMetrics()函数的用法 转
转自 http://www.cnblogs.com/lidabo/archive/2012/07/10/2584725.html 可以用GetSystemMetrics函数可以获取系统分辨率,但这只是 ...