POJ 2352 stars (树状数组入门经典!!!)
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 54352 | Accepted: 23386 |
Description
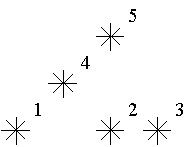
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level 0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
Output
Sample Input
5
1 1
5 1
7 1
3 3
5 5
Sample Output
1
2
1
1
0
Hint
给你n给点的star的坐标,star左下角star的个数是star的等级(包括左下边界,即x相同或者y相同
最好输出等级1到等级n的star个数
所以这题可以用树状数组写
所以统计某个等级的个数就是统计x前面比它小的星星的数量
但是有需要注意的地方
树状数组是不能统计0的
所以每个x都要++
这也也不会影响统计的结果
#include<queue>
#include<set>
#include<cstdio>
#include <iostream>
#include<algorithm>
#include<cstring>
#include<cmath>
using namespace std;
#define max_v 320010
int a[max_v];
int c[max_v];
//C数组C[i] = a[i – 2^k + 1] + … + a[i] ,k为二进制下某尾0的个数。
int lowbit(int x)//返回2的k次方的值
{
return x&(-x);
}
void update(int x,int d)//更新树状数组
{
while(x<max_v)
{
c[x]=c[x]+d;
x=x+lowbit(x);
}
}
int getsum(int x)//就是把C数组全部加起来
{
int res=;
while(x>)
{
res=res+c[x];
x=x-lowbit(x);
}
return res; }
int main()
{
int n,x,y;
while(~scanf("%d",&n))
{
memset(c,,sizeof(c));
memset(a,,sizeof(a));
for(int i=;i<n;i++)
{
scanf("%d %d",&x,&y);//下标可能从0开始,所以要x+1
a[getsum(x+)]++;//求出其左下角star个数
update(x+,);//更新树状数组
}
for(int i=;i<n;i++)
{
printf("%d\n",a[i]);
}
}
return ;
}
/*
题目意思:
给你n给点的star的坐标,star左下角star的个数是star的等级(包括左下边界,即x相同或者y相同
最好输出等级1到等级n的star个数 分析:树状树主要用来解决区间问题
所以这题可以用树状数组写 一开始给出的数据是已经按照y升序排序好了的,如果y相同则按照x升序排序
所以统计某个等级的个数就是统计x前面比它小的星星的数量
但是有需要注意的地方
树状数组是不能统计0的
所以每个x都要++
这也也不会影响统计的结果 */
POJ 2352 stars (树状数组入门经典!!!)的更多相关文章
- POJ 2352 【树状数组】
题意: 给了很多星星的坐标,星星的特征值是不比他自己本身高而且不在它右边的星星数. 给定的输入数据是按照y升序排序的,y相同的情况下按照x排列,x和y都是介于0和32000之间的整数.每个坐标最多有一 ...
- hdu 1541/poj 2352:Stars(树状数组,经典题)
Stars Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submi ...
- POJ 2352 && HDU 1541 Stars (树状数组)
一開始想,总感觉是DP,但是最后什么都没想到.还暴力的交了一发. 然后開始写线段树,结果超时.感觉自己线段树的写法有问题.改天再写.先把树状数组的写法贴出来吧. ~~~~~~~~~~~~~~~~~~~ ...
- 树状数组入门 hdu1541 Stars
树状数组 树状数组(Binary Indexed Tree(B.I.T), Fenwick Tree)是一个查询和修改复杂度都为log(n)的数据结构.主要用于查询任意两位之间的所有元素之和,但是每次 ...
- POJ 2299 Ultra-QuickSort 求逆序数 (归并或者数状数组)此题为树状数组入门题!!!
Ultra-QuickSort Time Limit: 7000MS Memory Limit: 65536K Total Submissions: 70674 Accepted: 26538 ...
- POJ-2352 Stars 树状数组
Stars Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 39186 Accepted: 17027 Description A ...
- Stars(树状数组或线段树)
Stars Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 37323 Accepted: 16278 Description A ...
- poj 2229 Ultra-QuickSort(树状数组求逆序数)
题目链接:http://poj.org/problem?id=2299 题目大意:给定n个数,要求这些数构成的逆序对的个数. 可以采用归并排序,也可以使用树状数组 可以把数一个个插入到树状数组中, 每 ...
- Stars(树状数组)
http://acm.hdu.edu.cn/showproblem.php?pid=1541 Stars Time Limit: 2000/1000 MS (Java/Others) Memor ...
随机推荐
- HDU-2046 骨牌铺方格【递推】
http://acm.hdu.edu.cn/showproblem.php?pid=2046 和前面的一样,a[i] = a[i-1] + a[i-2] #include<iostream> ...
- MySql的InnoDB存储引擎--索引
索引分类: 1.聚集索引:索引顺序与物理顺序一致. MySql 的 InnoDB 中,主键索引就是聚集索引.好处是,进行搜索的时候,因为索引和物理顺序一致,所以找数据的时候更快. 2.非聚集索引:索引 ...
- react框架 Dva & Umi
概念 // http://localhost:3000/ //models import IndexPage from './routes/IndexPage'; import Products fr ...
- 使用装饰器减少try ...finally的重复使用
@util.try_except_bskgk def added_user_handle(cur, search_time): added_user_sql = """ ...
- JS cookie 设置 查看 删除
JScookie 常用的3个预设函数(库) <!DOCTYPE HTML> <html> <head> <meta charset="utf-8&q ...
- redhat系统下三种主要的软件包安装方法
1. 通过RPM软件包来安装 RPM(Redhat Package Management)标准的软件包,只需简单地输入命令“rpm -ivh filename.rpm”即可: 如果需要对已经安装的RP ...
- MooseFS安装部署
环境信息 Master服务器 dev04 chunkserver服务器 dev02.dev03.dev04 metalogger服务器 dev03 mount客户端 dev01.dev02 安装前下载 ...
- javascript使用web proxy来实现ajax cross-domain通信
在现代浏览器中,都强加了对javacript代码的访问限制,比如一个页面的js无法向非同源的url实现ajax请求,获得数据.在这时,是浏览器端会报错: No 'Access-Control-Allo ...
- Java中执行.exe文件
public static void main(String args[]){ try { String command ="notepad"; // 笔记本 Process ch ...
- 用适配器模式处理复杂的UITableView中cell的业务逻辑
用适配器模式处理复杂的UITableView中cell的业务逻辑 适配器是用来隔离数据源对cell布局影响而使用的,cell只接受适配器的数据,而不会与外部数据源进行交互. 源码: ModelCell ...