poj1573 Robot Motion
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 12507 | Accepted: 6070 |
Description
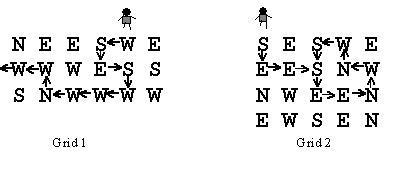
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
Input
the number of the column in which the robot enters from the north. The possible entry columns are numbered starting with one at the left. Then come the rows of the direction instructions. Each grid will have at least one and at most 10 rows and columns of
instructions. The lines of instructions contain only the characters N, S, E, or W with no blanks. The end of input is indicated by a row containing 0 0 0.
Output
once, and then the instructions on some number of locations repeatedly. The sample input below corresponds to the two grids above and illustrates the two forms of output. The word "step" is always immediately followed by "(s)" whether or not the number before
it is 1.
Sample Input
3 6 5
NEESWE
WWWESS
SNWWWW
4 5 1
SESWE
EESNW
NWEEN
EWSEN
0 0 0
Sample Output
10 step(s) to exit
3 step(s) before a loop of 8 step(s)
Source
简单的方位移动型模拟题,要用方位数组。移动模拟过程用搜索。
15777856
ksq2013 | 1573 | Accepted | 700K | 0MS | G++ | 1074B | 2016-07-21 22:16:18 |
#include<cstdio>
#include<cstring>
#include<iostream>
using namespace std;
const int mx[]={0,+1,0,-1};
const int my[]={+1,0,-1,0};//ESWN;
bool vis[11][11];
int n,m,s,mp[11][11],stp[11][11];
void dfs(int x,int y,int cnt)
{
if(x<1||x>n||y<1||y>m){
printf("%d step(s) to exit\n",cnt);
return;
}
if(vis[x][y]){
printf("%d step(s) before a loop of %d step(s)\n",stp[x][y],cnt-stp[x][y]);
return;
}
vis[x][y]=1;
stp[x][y]=cnt;
int tmp=mp[x][y];
dfs(x+mx[tmp],y+my[tmp],cnt+1);
}
int main()
{
while(scanf("%d%d%d",&n,&m,&s)&&s){
memset(mp,0,sizeof(mp));
memset(vis,0,sizeof(vis));
for(int x=1;x<=n;x++){
for(int y=1;y<=m;y++){
char ch;
cin>>ch;
switch(ch){
case 'E':mp[x][y]=0;break;
case 'S':mp[x][y]=1;break;
case 'W':mp[x][y]=2;break;
case 'N':mp[x][y]=3;break;
}
}
}
dfs(1,s,0);
}
return 0;
}
poj1573 Robot Motion的更多相关文章
- POJ1573——Robot Motion
Robot Motion Description A robot has been programmed to follow the instructions in its path. Instruc ...
- POJ-1573 Robot Motion模拟
题目链接: https://vjudge.net/problem/POJ-1573 题目大意: 有一个N*M的区域,机器人从第一行的第几列进入,该区域全部由'N' , 'S' , 'W' , 'E' ...
- poj1573 Robot Motion(DFS)
题目链接 http://poj.org/problem?id=1573 题意 一个机器人在给定的迷宫中行走,在迷宫中的特定位置只能按照特定的方向行走,有两种情况:①机器人按照方向序列走出迷宫,这时输出 ...
- POJ1573(Robot Motion)--简单模拟+简单dfs
题目在这里 题意 : 问你按照图中所给的提示走,多少步能走出来??? 其实只要根据这个提示走下去就行了.模拟每一步就OK,因为下一步的操作和上一步一样,所以简单dfs.如果出现loop状态,只要记忆每 ...
- POJ1573 Robot Motion(模拟)
题目链接. 分析: 很简单的一道题, #include <iostream> #include <cstring> #include <cstdio> #inclu ...
- 【POJ - 1573】Robot Motion
-->Robot Motion 直接中文 Descriptions: 样例1 样例2 有一个N*M的区域,机器人从第一行的第几列进入,该区域全部由'N' , 'S' , 'W' , 'E' ,走 ...
- Robot Motion(imitate)
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 11065 Accepted: 5378 Des ...
- 模拟 POJ 1573 Robot Motion
题目地址:http://poj.org/problem?id=1573 /* 题意:给定地图和起始位置,robot(上下左右)一步一步去走,问走出地图的步数 如果是死循环,输出走进死循环之前的步数和死 ...
- POJ 1573 Robot Motion(BFS)
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 12856 Accepted: 6240 Des ...
随机推荐
- js基本算法:冒泡排序,二分查找
知识扩充: 时间复杂度:算法的时间复杂度是一个函数,描述了算法的运行时间.时间复杂度越低,效率越高. 自我理解:一个算法,运行了几次时间复杂度就为多少,如运行了n次,则时间复杂度为O(n). 1.冒泡 ...
- Microsoft SharePoint Server 2013 Service Pack 1 (sp1)终于出来了!!!
Microsoft SharePoint Server 2013 Service Pack 1 终于出来了!以下是下载地址如下,大小1.25G. http://www.microsoft.com/zh ...
- Android - ADB 的使用
一.什么是ADB? 1.ADB全称Android Debug Bridge, 是android sdk里的一个工具,用这个工具可以直接操作管理android模拟器或者真实的andriod设备 2.AD ...
- IOS沙盒中的Documents、Library、tmp区别
1.Documents: 用户生成的文件.其他数据及其他程序不能重新创建的文件,iTunes备份和恢复的时候会包括此目录. 2.Library/Caches: 可以重新下载或者重新生成的数据,数据库缓 ...
- 优化MySchool数据库(事务、视图、索引)
事务.视图.索引: 事务:当生活逻辑中的“一个步骤”,需要使用多条SQL去完成时,必须使用事务来确保其“完整性“. 视图:简化数据库结构,方便你编写SQL语句(简化SQL语句的编写) 索引:提高“数据 ...
- 截取UIImage指定大小区域
截取UIImage指定大小区域 最近遇到这样的需求:从服务器获取到一张照片,只需要显示他的左半部分,或者中间部分等等.也就是截取UIImage指定大小区域. UIImage扩展 我的解决方案是对UII ...
- 转:能和LoadRunner匹敌的VS2010/2012Web负载测试
原文出处:http://www.cnblogs.com/aarond/archive/2013/04/18/performance.html VS自带的Web负载测试真的很大程度上能和专业的loadr ...
- jQuery Grid With ASP.Net MVC
jQuery Grid 能够在 ASP.NET MVC 中轻松地实现分页. 排序. 筛选以及 jQuery 插件网格中的 CRUD 操作. 具有以下特征: 时尚的表格数据呈现控件. JavaScrip ...
- 16、总经理要阅读的书籍 - IT软件人员书籍系列文章
总经理是公司的一个领导角色.他主要负责公司级别的比如规划,战略等等内容.有些公司的总经理,比如软件公司的总经理,往往是一个大的业务员,将更多的大型的软件项目投取过来,让公司能够有钱赚,让公司员工能够跟 ...
- SQL Server调优系列基础篇(常用运算符总结——三种物理连接方式剖析)
前言 上一篇我们介绍了如何查看查询计划,本篇将介绍在我们查看的查询计划时的分析技巧,以及几种我们常用的运算符优化技巧,同样侧重基础知识的掌握. 通过本篇可以了解我们平常所写的T-SQL语句,在SQL ...