北大poj-1021
2D-Nim
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 4066 | Accepted: 1851 |
Description
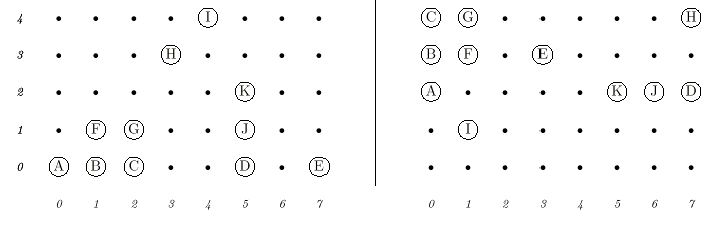
The player on move may remove (A), (B), (A, B), (A, B, C), or (B,F), etc., but may not remove (A, C), (D, E), (H, I) or (B, G).
For purposes of writing 2D-Nim-playing software, a certain
programmer wants to be able to tell whether or not a certain position
has ever been analyzed previously. Because of the rules of 2D-Nim, it
should be clear that the two boards above are essentially equivalent.
That is, if there is a winning strategy for the left board, the same one
must apply to the right board. The fact that the contiguous groups of
pieces appear in different places and orientations is clearly
irrelevant. All that matters is that the same clusters of pieces (a
cluster being a set of contiguous pieces that can be reached from each
other by a sequence of one-square vertical or horizontal moves) appear
in each. For example, the cluster of pieces (A, B, C, F, G) appears on
both boards, but it has been reflected (swapping left and right),
rotated, and moved. Your task is to determine whether two given board
states are equivalent in this sense or not.
Input
first line of the input file contains a single integer t (1 ≤ t ≤ 10),
the number of test cases, followed by the input data for each test case.
The first line of each test case consists of three integers W, H, and n
(1 ≤ W, H ≤ 100). W is the width, and H is the height of the grid in
terms of the number of grid points. n is the number of pieces on each
board. The second line of each test case contains a sequence of n pairs
of integers xi , yi, giving the coordinates of the pieces on the first
board (0 ≤ xi < W and 0 ≤ yi < H). The third line of the test case
describes the coordinates of the pieces on the second board in the same
format.
Output
program should produce a single line for each test case containing a
word YES or NO indicating whether the two boards are equivalent or not.
Sample Input
2
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 5 2 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 6 1 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
Sample Output
YES
NO
Source
#include <stdio.h>
#include <stdlib.h>
#include <string.h> typedef struct
{
int width;
int height;
int num;
int map[][][];
}Picture; typedef struct
{
int x;
int y;
int factor;
}Piont; Picture pic;
Piont points[][];
int factorCnt[][];
int connectCnt[][];
int connectMax[]; void Input()
{
int i, j, x, y = ;
memset(&pic, , sizeof(pic));
scanf("%d %d %d", &pic.width, &pic.height, &pic.num); for(j = ; j < ; j++)
{
for(i = ; i < pic.num; i++)
{
scanf("%d %d", &x, &y);
pic.map[j][y][x] = ;
points[j][i].x = x;
points[j][i].y = y;
}
}
/*
for(j = 0; j < 2; j++)
{
for(y = pic.height-1; y >= 0; y--)
{
for(x = 0; x < pic.width; x++)
{
printf("%d ", pic.map[j][y][x]);
}
printf("\n");
}
printf("------------------------\n");
}
*/
} void CalcFactor()
{
int i, j, x, y, factor;
memset(factorCnt, , sizeof(factorCnt));
for(j = ; j < ; j++)
{
for(i = ; i < pic.num; i++)
{
x = points[j][i].x;
y = points[j][i].y;
factor = (x > ) ? pic.map[j][y][x-] : ;
factor += (x < pic.width-) ? pic.map[j][y][x+] : ;
factor += (y > ) ? pic.map[j][y-][x] : ;
factor += (y < pic.height-) ? pic.map[j][y+][x] : ;
points[j][i].factor = factor;
factorCnt[j][factor]++;
}
}
/*
for(j = 0; j < 2; j++)
{
for(i = 0; i < pic.num; i++)
{
printf("%d ", points[j][i].factor);
}
printf("\n");
}
printf("------------------------\n");
*/
} void CheckResult()
{
int i, j = ;
for(i = ; i < ; i++)
{
if(factorCnt[][i] != factorCnt[][i]) break;
} if(connectMax[] != connectMax[])
{
printf("NO\n");
return;
} for(j = ; j < connectMax[]; j++)
{
if(connectCnt[][j] != connectCnt[][j]) break;
} if(i != || j != connectMax[])
{
printf("NO\n");
}
else
{
printf("YES\n");
}
} void CalcConnect()
{
int j, x, y, connect;
memset(connectMax, , sizeof(connectMax));
memset(connectCnt, , sizeof(connectCnt));
for(j = ; j < ; j++)
{
for(y = ; y < pic.height; y++)
{
for(x = ; x < pic.width; x++)
{
if(pic.map[j][y][x] != ) continue;
connect = ;
while(++x < pic.width && pic.map[j][y][x] == )
{
connect++;
}
connectCnt[j][connect]++;
if(connect > connectMax[j]) connectMax[j] = connect;
}
} for(x = ; x < pic.width; x++)
{
for(y = ; y < pic.height; y++)
{
if(pic.map[j][y][x] != ) continue;
connect = ;
while(++y < pic.height && pic.map[j][y][x] == )
{
connect++;
}
connectCnt[j][connect]++;
if(connect > connectMax[j]) connectMax[j] = connect;
}
}
}
} void Proc()
{
CalcFactor();
CalcConnect();
CheckResult();
} int main()
{
int num = ;
scanf("%d", &num);
while(num--)
{
Input();
Proc();
}
return ;
}
北大poj-1021的更多相关文章
- 北大POJ题库使用指南
原文地址:北大POJ题库使用指南 北大ACM题分类主流算法: 1.搜索 //回溯 2.DP(动态规划)//记忆化搜索 3.贪心 4.图论 //最短路径.最小生成树.网络流 5.数论 //组合数学(排列 ...
- poj 1021矩阵平移装换后是否为同一个矩阵
2D-Nim Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 3081 Accepted: 1398 Descriptio ...
- POJ 1021 2D-Nim
Description The 2D-Nim board game is played on a grid, with pieces on the grid points. On each move, ...
- POJ 1021 人品题
报告见代码.. #include <iostream> #include <cstdio> #include <cstring> #include <algo ...
- 【Java】深深跪了,OJ题目Java与C运行效率对比(附带清华北大OJ内存计算的对比)
看了园友的评论之后,我也好奇清橙OJ是怎么计算内存占用的.重新测试的情况附在原文后边. -------------------------------------- 这是切割线 ----------- ...
- POJ 1861 Network (Kruskal算法+输出的最小生成树里最长的边==最后加入生成树的边权 *【模板】)
Network Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 14021 Accepted: 5484 Specia ...
- 各大OJ
北大POJ 杭电HDU 浙大ZOj 蓝桥杯 PAT
- leetcode学习笔记--开篇
1 LeetCode是什么? LeetCode是一个在线的编程测试平台,国内也有类似的Online Judge平台.程序开发人员可以通过在线刷题,提高对于算法和数据结构的理解能力,夯实自己的编程基础. ...
- OJ题目JAVA与C运行效率对比
[JAVA]深深跪了,OJ题目JAVA与C运行效率对比(附带清华北大OJ内存计算的对比) 看了园友的评论之后,我也好奇清橙OJ是怎么计算内存占用的.重新测试的情况附在原文后边. ----------- ...
- C++ 指针常见用法小结
1. 概论 2.指针基础 3. 指针进阶 4. 一维数组的定义与初始化 5. 指针和数组 6. 指针运算 7. 多维数组和指针 8. 指针形参 9. 数组形参 10. 返回指针和数组 11. 结语 ...
随机推荐
- [名词解释 ] transparent
1.材质,效果透明. 2.思想透明,容易获取(思维简单,单纯) 3.后台静默(of a process or interface) functioning without the user being ...
- prometheus告警插件-alertmanager
prometheus本身不支持告警功能,主要通过插件alertmanage来实现告警.AlertManager用于接收Prometheus发送的告警并对于告警进行一系列的处理后发送给指定的用户. pr ...
- 阿里云 RDS for MySQL支持什么引擎
问题:我们的服务器是买的是阿里云,mysql版本5.011 ,本地和服务器配置一样,在本地可以安装discuzX3.4,但是在服务器上却报错了,如下图: 找了半天,才知道阿里云RDS 支持的mysql ...
- 配置Spring MVC - 2019
未完 软件环境:Eclipse-EE 1. 创建Maven Project 2. pom.xml - [更新日期19/03/31] <dependencies> <dependenc ...
- x变成y的最少操作次数(层次遍历)
输入x,y,x为源数字,y为目标值.输出x变成y的最少操作次数. x每次可以执行三种操作:-1 . +1 . x2: 如 x=5,y=8:5-1=4,4x2=8;所以输出结果为2(次操作). 可以发现 ...
- JavaScript前端面试题总结
1.em和rem 像素(px):用于元素的边框或定位. em/rem:用于做响应式页面,em相对于父元素,rem相对于根元素. rem 单位翻译为像素值是由 html 元素的字体大小决定的. 此字体大 ...
- Android proguard混淆签名打包出现"android proguard failed to export application"解决方案
刚刚接触安卓,不是很熟悉.发现之前可以正常打包的项目出现添加混淆再进行打包签名的APK之后提示"android proguard failed to export application&q ...
- ckeditor自定义插件--一键给所有的图片添加链接
ckeditor自定义插件在网上查了查,感觉还是比较好用的,写了一个一键给编辑器中的所有图片添加链接. 在ckeditor目录下的plugins下建以插件为名的文件夹,在里边建plugin.js文件, ...
- oracle中“ORA-00060: 等待资源时检测到死锁” 或存储过程编译卡死 解决方法
之前在调试存储过程时,出现卡死情况,无法插入数据 解决方法 1.查看那些表被锁住: --1.查看那些表被锁住--- select b.owner,b.object_name,a.session_id, ...
- js for in循环遍历对象,获取key:value值
var testObj = { 'a':'111', 'b':'222', 'c':'333', 'd':'444'}for(var i in testObj){ console.log(i); // ...