problem-solving-with-algorithms-and-data-structure-usingpython(使用python解决算法和数据结构) -- 算法分析
1. 计算前n个整数的和
def sumOfN(n):
theSum = 0
for i in range(1,n+1):
theSum += i
return theSum
print(sumOfN(10))
解法一
def sumOfN(n):
return (n*(n+1))/2
print(sumOfN(10))
解法二
2. 乱序字符串检查
乱序字符串是指一个字符串只是另一个字符串的重新排列。
例如,'heart' 和 'earth' 就是乱序字符串。'python' 和 'typhon' 也是。
为了简单起见,我们假设所讨论的两个字符串具有相等的长度,并且他们由 26 个小写字母集合组成。
我们的目标是写一个布尔函数,它将两个字符串做参数并返回它们是不是乱序。
def anagramSolution1(s1,s2):
alist = list(s2)
pos1 = 0
stillok = True
while pos1 < len(s1) and stillok:
pos2 = 0
found = False
while pos2 < len(alist) and not found:
if s1[pos1] == alist[pos2]:
found = True
else:
pos2 += 1
if found:
alist[pos2] = None
else:
stillok = False
pos1 += 1
return stillok print(anagramSolution1('abcd', 'dcba'))
解法一:检查
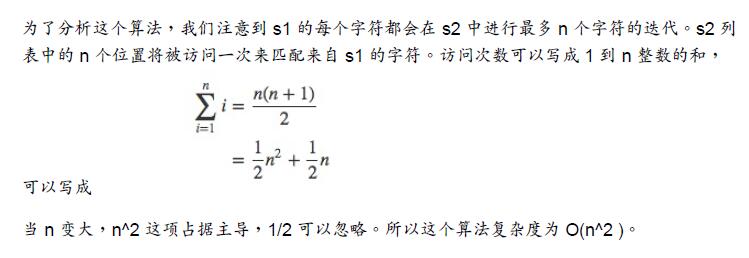
即使 s1,s2 不同,它们都是由完全相同的字符组成的。
所以,我们按照字母顺序从 a 到 z 排列每个字符串,如果两个字符串相同,那这两个字符串就是乱序字符串。 def anagramSolution1(s1,s2):
alist1 = list(s1)
alist2 = list(s2) alist1.sort()
alist2.sort() pos = 0
matches = True while pos < len(s1) and matches:
if alist1[pos] == alist2[pos]:
pos += 1
else:
matches = False
return matches print(anagramSolution1('abcde', 'edcba'))
解法二:排序和比较
首先你可能认为这个算法是 O(n),因为只有一个简单的迭代来比较排序后的 n 个字符。
但是,调用 Python 排序不是没有成本。正如我们将在后面的章节中看到的,排序通常是O(n^2) 或 O(nlogn)。
所以排序操作比迭代花费更多。最后该算法跟排序过程有同样的量级。
算法分析
利用两个乱序字符串具有相同数目的 a, b, c 等字符的事实。
我们首先计算的是每个字母出现的次数。
由于有 26 个可能的字符,我们就用 一个长度为 26 的列表,每个可能的字符占一个位置。
每次看到一个特定的字符,就增加该位置的计数器。
最后如果两个列表的计数器一样,则字符串为乱序字符串。 def anagramSolution1(s1,s2):
c1 = [0]*26
c2 = [0]*26 for i in range(len(s1)):
pos = ord(s1[i]) - ord('a') # ord 函数:返回对应的 ASCII 数值
c1[pos] += 1
for i in range(len(s2)):
pos = ord(s2[i]) - ord('a') # ord 函数:返回对应的 ASCII 数值
c2[pos] += 1
j = 0
stillok = True
while j < 26 and stillok:
if c1[j] == c2[j]:
j += 1
else:
stillok = False
return stillok print(anagramSolution1('apple', 'pleap'))
解法三:计数和比较
同样,这个方案有多个迭代,但是和第一个解法不一样,它不是嵌套的。
两个迭代都是 n, 第三个迭代,比较两个计数列表,需要 26 步,因为有 26 个字母。
一共T(n)=2n+26T(n)=2n+26,即 O(n),我们找到了一个线性量级的算法解决这个问题。
算法分析
3. 生成一个从0开始的n个数字的列表
def test1():
l = []
for i in range(1000):
l = l + [i]
test1
def test2():
l = []
for i in range(1000):
l.append(i)
test2
def test3():
l = [i for i in range(1000)]
test3
def test4():
l = list(range(1000))
test4
列表操作的效率
操作 | O()值 |
index[] | O(1) |
append | O(1) |
pop() | O(1) |
pop(i) | O(n) |
insert(i,item) | O(n) |
del operator | O(n) |
iteration | O(n) |
contains(in) | O(n) |
get slice[x:y] | O(k) |
del slice | O(n) |
set slice | O(n+k) |
reverse |
O(n) |
concatenate | O(k) |
sort | O(n*logn) |
multiply | O(n*k) |
字典操作的效率
操作 | O()值 |
copy | O(n) |
get item | O(1) |
set item | O(1) |
delete item | O(1) |
contains(in) | O(1) |
iteration | O(n) |
时间复杂性:https://wiki.python.org/moin/TimeComplexity
总结:
1. 算法分析是一种独立的测量算法的方法
2. 大O表示法允许根据问题的大小,通过其主要部分来对算法进行分类
problem-solving-with-algorithms-and-data-structure-usingpython(使用python解决算法和数据结构) -- 算法分析的更多相关文章
- 学习笔记之Problem Solving with Algorithms and Data Structures using Python
Problem Solving with Algorithms and Data Structures using Python — Problem Solving with Algorithms a ...
- [Algorithms] Tree Data Structure in JavaScript
In a tree, nodes have a single parent node and may have many children nodes. They never have more th ...
- CDOJ 483 Data Structure Problem DFS
Data Structure Problem Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://acm.uestc.edu.cn/#/proble ...
- [LeetCode] Add and Search Word - Data structure design 添加和查找单词-数据结构设计
Design a data structure that supports the following two operations: void addWord(word) bool search(w ...
- hdu-5929 Basic Data Structure(双端队列+模拟)
题目链接: Basic Data Structure Time Limit: 7000/3500 MS (Java/Others) Memory Limit: 65536/65536 K (Ja ...
- HDU 5929 Basic Data Structure 模拟
Basic Data Structure Time Limit: 7000/3500 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Oth ...
- hdu 4217 Data Structure? 树状数组求第K小
Data Structure? Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others) ...
- (*medium)LeetCode 211.Add and Search Word - Data structure design
Design a data structure that supports the following two operations: void addWord(word) bool search(w ...
- Add and Search Word - Data structure design
https://leetcode.com/problems/add-and-search-word-data-structure-design/ Design a data structure tha ...
- 211. Add and Search Word - Data structure design
题目: Design a data structure that supports the following two operations: void addWord(word) bool sear ...
随机推荐
- Storm一致性事物
Storm是一个分布式的流处理系统,利用anchor和ack机制保证所有的tuple都被处理成功.如果tuple出错,则可以被重传,但是如何保证出错的tuple只被处理一次呢?换句话说Storm如何保 ...
- Jmeter服务器监控 serveragent如何使用
安装jmeter插件Plugins Managerjmeter-plugins.org推出了全新的Plugins Manager,对于其提供的插件进行了集中的管理,我们只需要安装这个管理插件,即可以在 ...
- 把本地git仓库的项目上传到远程仓库
之前在学校实验室服务器上建了一个git远程仓库,存放我写的express项目代码.后来由于出去实习,就无法访问那个远程仓库了,因为它在校园网内. 还好我的笔记本中有这个项目完整的本地仓库,于是我就试着 ...
- 转的很好的前端html 内容
HTML 初识 web服务本质 import socket def main(): sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s ...
- iOS(Swift)-Runtime之关于页面跳转的捷径【Runtime获取当前ViewController,很常用】
写在前面 在我们操作页面跳转时,如果当前的类不是UIViewcontroller(下面用VC表示),你会不会写一个代理,或者block给VC传递信息,然后在VC里面进行 ///假如targetVc是将 ...
- MySQL多实例(二)
一.多实例MySQL数据库原理 1.1:原理图 1.2:多实例原理(什么是多实例) 简单来说MySQL多实例就是在一台服务器上同时开启多个不用的服务端口(如:3306.3307),同时运行多个MySQ ...
- J05-Java IO流总结五 《 BufferedInputStream和BufferedOutputStream 》
1. 概念简介 BufferedInputStream和BufferedOutputStream是带缓冲区的字节输入输出处理流.它们本身并不具有IO流的读取与写入功能,只是在别的流(节点流或其他处理流 ...
- linux 手动释放buff/cache
为了解决buff/cache占用过多的问题执行以下命令即可 syncecho 1 > /proc/sys/vm/drop_cachesecho 2 > /proc/sys/vm/drop_ ...
- SVN的安装和使用手册
下载`TortoiseSVN 官网下载址:https://www.visualsvn.com/visualsvn/download/tortoisesvn/ 下载完成后是这样的 安装TortoiseS ...
- 2018春招-今日头条笔试题-第二题(python)
题目描述:2018春招-今日头条笔试题5题(后附大佬答案-c++版) 解题思路: 利用深度优先搜索 #-*- coding:utf-8 -*- class DFS: ''' num:用于存储最后执行次 ...