使用 Microsoft.Extensions.DependencyInjection 进行依赖注入
没有
的日子,才是好日子~~~~~~~~~~
Using .NET Core 3.0 Dependency Injection and Service Provider with WPF
UPDATE: this article is based on a preview release of .NET Core 3.0. Please refer to Update on using HostBuilder, Dependency Injection and Service Provider with .NET Core 3.0 WPF applications for the latest one.
We all know that .NET Core provides built-in support for Dependency Injection. We typically use it in ASP.NET Core (starting form the ConfigureServices method in the Startup.cs file), but the feature isn’t limited to this framework. So, as .NET Core 3.0 supports also Windows Clients development, we can use it in our WPF and Windows Forms applications.
Let’s see how to do that, for example, in WPF using Visual Studio 2019. Suppose we want to create a service and we also have some application settings; we want to pass both of them to each window of our application via Dependency Injection.
First of all, we must add the required NuGet packages to the project. Right click on the Solution Explorer, select the Manage NuGet Packages command and add the following packages (be sure to select the Include prerelease check):
- Microsoft.Extensions.DependencyInjection
- Microsoft.Extensions.Options.ConfigurationExtensions
- Microsoft.Extensions.Configuration.Json
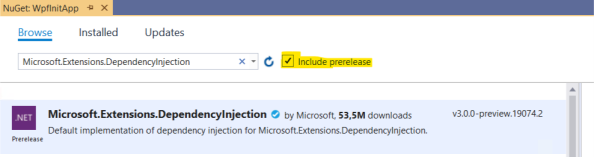
Adding Dependency Injection support to a .NET Core 3.0 WPF application
These packages are necessary to enable Dependency Injection support (the first one) and to store and retrieve application settings in the classic appsettings.json file (the other ones). They will automatically get all the required dependencies.
Then, let’s add a file named appsettings.json to the root folder of the project. Set its Build Action property to Content and Copy to Output Directory to Copy if newer:
1
2
3
4
5
6
7
|
{ "AppSettings" : { "StringSetting" : "Value" , "IntegerSetting" : 42, "BooleanSetting" : true } } |
All the prerequisites are met, so we can start writing our code. Let’s open the App.xaml file and remove the StartupUri property of the Application class. Then, we need to override the OnStartup method in App.xaml.cs:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
public partial class App : Application { public IServiceProvider ServiceProvider { get ; private set ; } public IConfiguration Configuration { get ; private set ; } protected override void OnStartup(StartupEventArgs e) { var builder = new ConfigurationBuilder() .SetBasePath(Directory.GetCurrentDirectory()) .AddJsonFile( "appsettings.json" , optional: false , reloadOnChange: true ); Configuration = builder.Build(); var serviceCollection = new ServiceCollection(); ConfigureServices(serviceCollection); ServiceProvider = serviceCollection.BuildServiceProvider(); var mainWindow = ServiceProvider.GetRequiredService<MainWindow>(); mainWindow.Show(); } private void ConfigureServices(IServiceCollection services) { // ... services.AddTransient( typeof (MainWindow)); } } |
In this method we create the Service Provider and configure the IoC container in a similar way of ASP.NET Core. We only need a bit of initialization. First of all, at line 9-13 we create an IConfiguration object that allows to read settings from the appsettings.json file (line 11). Then, we create an instance of a ServiceCollection class that will hold our services. Finally we call the ConfigureServices method (as we have in ASP.NET Core).
Within the latter (lines 24-29), we register all the services used by the application in the exact same way of ASP. NET Core. We’ll complete this method in a moment, but for now let’s notice that we register also the MainWindow class (line 28). This is important because, in this way, the window itself becomes part of the Dependency Injection chain. It means that, after calling this method, at line 20-21 we can get it from the ServiceProvider and then show it. But, more important, it means that we can pass to the MainWindow constructor all the dependencies it needs, as we do for ASP.NET Core Controllers.
Even if the actual services aren’t yet registered, we can run the application and see that everything works as expected.
Now it’s time to complicate the things a bit. First of all, let’s create an AppSettings.cs file to hold configuration settings. This file will map the settings that we write in appsettings.json:
1
2
3
4
5
6
7
8
|
public class AppSettings { public string StringSetting { get ; set ; } public int IntegerSetting { get ; set ; } public bool BooleanSetting { get ; set ; } } |
Then, create also a sample service with its interface:
1
2
3
4
5
6
7
8
9
|
public interface ISampleService { string GetCurrentDate(); } public class SampleService : ISampleService { public string GetCurrentDate() => DateTime.Now.ToLongDateString(); } |
Now we must register these services in the IoC Container, as usual:
1
2
3
4
5
6
7
8
9
|
private void ConfigureServices(IServiceCollection services) { services.Configure<AppSettings> (Configuration.GetSection(nameof(AppSettings))); services.AddScoped<ISampleService, SampleService>(); // ... } |
As said before, the MainWindow itself is in the IoC Container. So, when we get it from the Service Provider, it will automatically be injected with all required services, if any. So, we just need to modify its constructor:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
public partial class MainWindow : Window { private readonly ISampleService sampleService; private readonly AppSettings settings; public MainWindow(ISampleService sampleService, IOptions<AppSettings> settings) { InitializeComponent(); this .sampleService = sampleService; this .settings = settings.Value; } // ... } |
Running this code, we’ll obtain a result like the following:
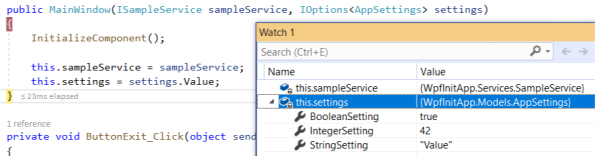
The .NET Core 3.0 WPF application with dependecies injected
原文地址:https://marcominerva.wordpress.com/2019/03/06/using-net-core-3-0-dependency-injection-and-service-provider-with-wpf/
参考:https://docs.microsoft.com/zh-cn/aspnet/core/fundamentals/dependency-injection?view=aspnetcore-3.0
使用 Microsoft.Extensions.DependencyInjection 进行依赖注入的更多相关文章
- Microsoft.Extensions.DependencyInjection中的Transient依赖注入关系,使用不当会造成内存泄漏
Microsoft.Extensions.DependencyInjection中(下面简称DI)的Transient依赖注入关系,表示每次DI获取一个全新的注入对象.但是使用Transient依赖注 ...
- DotNetCore跨平台~一起聊聊Microsoft.Extensions.DependencyInjection
写这篇文章的心情:激动 Microsoft.Extensions.DependencyInjection在github上同样是开源的,它在dotnetcore里被广泛的使用,比起之前的autofac, ...
- 解析 Microsoft.Extensions.DependencyInjection 2.x 版本实现
项目使用了 Microsoft.Extensions.DependencyInjection 2.x 版本,遇到第2次请求时非常高的内存占用情况,于是作了调查,本文对 3.0 版本仍然适用. 先说结论 ...
- 使用诊断工具观察 Microsoft.Extensions.DependencyInjection 2.x 版本的内存占用
目录 准备工作 大量接口与实现类的生成 elasticsearch+kibana+apm asp.net core 应用 请求与快照 Kibana 上的请求记录 请求耗时的分析 请求内存的分析 第2次 ...
- Microsoft.Extensions.DependencyInjection 之三:展开测试
目录 前文回顾 IServiceCallSite CallSiteFactory ServiceProviderEngine CompiledServiceProviderEngine Dynamic ...
- Microsoft.Extensions.DependencyInjection 之三:反射可以一战(附源代码)
目录 前文回顾 IServiceCallSite CallSiteFactory ServiceProviderEngine CompiledServiceProviderEngine Dynamic ...
- Microsoft.Extensions.DependencyInjection 之二:使用诊断工具观察内存占用
目录 准备工作 大量接口与实现类的生成 elasticsearch+kibana+apm asp.net core 应用 请求与快照 Kibana 上的请求记录 请求耗时的分析 请求内存的分析 第2次 ...
- Microsoft.Extensions.DependencyInjection 之一:解析实现
[TOC] 前言 项目使用了 Microsoft.Extensions.DependencyInjection 2.x 版本,遇到第2次请求时非常高的内存占用情况,于是作了调查,本文对 3.0 版本仍 ...
- MvvmLight + Microsoft.Extensions.DependencyInjection + WpfApp(.NetCore3.1)
git clone MvvmLight失败,破网络, 就没有直接修改源码的方式来使用了 Nuget安装MvvmLightLibsStd10 使用GalaSoft.MvvmLight.Command命名 ...
随机推荐
- Kubernetes学习之基础概念
本文章目录 kubernetes特性 kubernetes集群架构与组件 一.kubernetes集群架构 二.集群组件 三.ubernetes集群术语 深入理解Pod对象 一.Pod容器分类 基础容 ...
- PostgreSQL 基本数据类型及常用SQL 函数操作
数据类型 名字 别名 描述 bigint int8 有符号的8字节整数 bigserial serial8 自动增长的8字节整数 bit [ (n) ] 定长位串 bit varying [ (n ...
- docker的小技巧记录(如果使用了更多会继续添加)
docker小技巧 复制本地sql脚本到docker容器mysql中进行使用 # 找到容器 docker ps # 复制文件 cp ./xxx.sql container-id:/tmp/ # 进入容 ...
- Intel重大漏洞之Meltdown和Spectre
史上最大漏洞危机:影响所有 iPhone.Android.PC 设备,修复困难重重 近日,英特尔的日子可并不好过. 作为全球知名芯片制造商,任何有关英特尔芯片漏洞的问题都会导致全球上百万设备遭受牵连. ...
- iView学习笔记(二):Table行编辑操作
1.前端准备工作 首先新建一个项目,然后引入iView插件,配置好router npm安装iView npm install iview --save cnpm install iview --sav ...
- AI人工智能-Python实现前后端人机聊天对话
[前言] AI 在人工智能进展的如火如荼的今天,我们如果不尝试去接触新鲜事物,马上就要被世界淘汰啦~ 本文拟使用Python开发语言实现类似于WIndows平台的“小娜”,或者是IOS下的“Siri” ...
- ECHO命令输出空行的11种方法和效率
标题: 批处理技术内幕:ECHO命令作者: Demon链接: http://demon.tw/reverse/cmd-internal-echo.html版权: 本博客的所有文章,都遵守“署名-非商业 ...
- javascript学习3、数据类型、数据类型转换、运算符
数据类型包括:基本数据类型和引用数据类型 基本数据类型指的是简单的数据段,引用数据类型指的是有多个值构成的对象. 当我们把变量赋值给一个变量时,解析器首先要确认的就是这个值是基本类型值还是引用类型值 ...
- 【时空大数据】Access 到 Postgres 数据迁移遇到的ODBC坑----驱动程序和应用程序之间的体系结构不匹配
1.安装Postgres10 2.安装Postgis插件 3.创建数据库 4.执行postgis脚本插件:参考https://www.cnblogs.com/defineconst/p/1064853 ...
- 运维常用shell脚本之日志清理
1.创建一个日志清理脚本 #/bin/bash for i in `find /root/.pm2/logs -name "*.log"` do cat /dev/null > ...