poj 2632 Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 6655 | Accepted: 2886 |
Description
space with a diameter of 1 meter. Assume there are N robots, numbered from 1 through N. You will get to know the position and orientation of each robot, and all the instructions, which are carefully (and mindlessly) followed by the robots. Instructions are
processed in the order they come. No two robots move simultaneously; a robot always completes its move before the next one starts moving.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
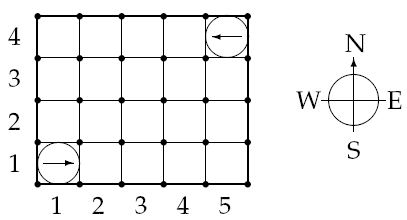
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
Output
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Only the first crash is to be reported.
Sample Input
4
5 4
2 2
1 1 E
5 4 W
1 F 7
2 F 7
5 4
2 4
1 1 E
5 4 W
1 F 3
2 F 1
1 L 1
1 F 3
5 4
2 2
1 1 E
5 4 W
1 L 96
1 F 2
5 4
2 3
1 1 E
5 4 W
1 F 4
1 L 1
1 F 20
Sample Output
Robot 1 crashes into the wall
Robot 1 crashes into robot 2
OK
Robot 1 crashes into robot 2
根据指令计算机器人走的方向和距离就好了,模拟题,只是注意旋转方向时候如果用0 1 2 3 代表4个方向,那么需要注意旋转方向不同会引起求出来的方向值是个负值,比如当前方向是1,假设正向是left,那么负向就是right,那么当right转动100次的时候,得到的方向就是-99,取余结果是-3,就会wa,避免的方法就是如果向反方向旋转的时候,机器人的方向值先加上一个很大的4的倍数的值,这样再减去指令要求的次数,就能保证是一个正数了
#include<stdio.h>
#include<string.h>
struct robot
{
int x, y;
int dir;
};
int dire[4][2] = { {0, 1}, {1, 0}, {0, -1}, {-1, 0}};
robot rob[110];
int map[110][110];
int getdir(char ch)
{
switch(ch)
{
case 'N':
return 0;
case 'E':
return 1;
case 'S':
return 2;
case 'W':
return 3;
}
}
int main()
{
int k;
scanf("%d", &k);
while(k--)
{
int A, B, n, m;
scanf("%d%d%d%d", &A, &B, &n, &m);
memset(rob, 0, sizeof(rob));
memset(map, 0, sizeof(map));
int i;
for(i = 1; i <= n; i++)
{
int a, b;
char ch;
scanf("%d%d %c", &a, &b, &ch);
rob[i].x = a;
rob[i].y = b;
rob[i].dir = getdir(ch);
map[a][b] = i;
}
bool flag = 1;
for(i = 0; i < m; i++)
{
int num, rep;
char act;
scanf("%d %c%d", &num, &act, &rep);
if(flag)
{
switch(act)
{
case 'L':
rob[num].dir += 400;
rob[num].dir -= rep;
rob[num].dir %= 4;
break;
case 'R':
rob[num].dir += rep;
rob[num].dir %= 4;
break;
case 'F':
map[rob[num].x][rob[num].y] = 0;
while(rep--)
{
rob[num].x = rob[num].x + dire[rob[num].dir][0];
rob[num].y = rob[num].y + dire[rob[num].dir][1];
if(rob[num].x < 1 || rob[num].x > A || rob[num].y < 1 || rob[num].y > B)
{
printf("Robot %d crashes into the wall\n", num);
flag = 0;
break;
}
else if(map[rob[num].x][rob[num].y])
{
printf("Robot %d crashes into robot %d\n", num, map[rob[num].x][rob[num].y]);
flag = 0;
break;
}
}
if(rep == -1)
{
map[rob[num].x][rob[num].y] = num; }
break;
}
}
}
if(flag)
{
printf("OK\n");
}
}
return 0;
}
poj 2632 Crashing Robots的更多相关文章
- 模拟 POJ 2632 Crashing Robots
题目地址:http://poj.org/problem?id=2632 /* 题意:几个机器人按照指示,逐个朝某个(指定)方向的直走,如果走过的路上有机器人则输出谁撞到:如果走出界了,输出谁出界 如果 ...
- POJ 2632 Crashing Robots (坑爹的模拟题)
Crashing Robots Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 6599 Accepted: 2854 D ...
- poj 2632 Crashing Robots(模拟)
链接:poj 2632 题意:在n*m的房间有num个机器,它们的坐标和方向已知,现给定一些指令及机器k运行的次数, L代表机器方向向左旋转90°,R代表机器方向向右旋转90°,F表示前进,每次前进一 ...
- POJ 2632 Crashing Robots(较为繁琐的模拟)
题目链接:http://poj.org/problem?id=2632 题目大意:题意简单,N个机器人在一个A*B的网格上运动,告诉你机器人的起始位置和对它的具体操作,输出结果: 1.Robot i ...
- POJ 2632 Crashing Robots 模拟 难度:0
http://poj.org/problem?id=2632 #include<cstdio> #include <cstring> #include <algorith ...
- poj 2632 Crashing Robots 模拟
题目链接: http://poj.org/problem?id=2632 题目描述: 有一个B*A的厂库,分布了n个机器人,机器人编号1~n.我们知道刚开始时全部机器人的位置和朝向,我们可以按顺序操控 ...
- POJ 2632 Crashing Robots (模拟 坐标调整)(fflush导致RE)
题目链接:http://poj.org/problem?id=2632 先话说昨天顺利1Y之后,直到今天下午才再出题 TAT,真是刷题计划深似海,从此AC是路人- - 本来2632是道略微恶心点的模拟 ...
- Poj OpenJudge 百练 2632 Crashing Robots
1.Link: http://poj.org/problem?id=2632 http://bailian.openjudge.cn/practice/2632/ 2.Content: Crashin ...
- poj 2632 Crashing Robots_模拟
做的差点想吐,调来调去,编译器都犯毛病,wlgq,幸好1a. 题意:给你机器人怎走的路线,碰撞就输出 #include <cstdlib> #include <iostream> ...
随机推荐
- 数据库连接工具类 数据库连接工具类——仅仅获得连接对象 ConnDB.java
package com.util; import java.sql.Connection; import java.sql.DriverManager; /** * 数据库连接工具类——仅仅获得连接对 ...
- oracle11g服务项及其启动顺序
oracle安装完成后共七个服务,含义分别为: 1. Oracle ORCL VSS Writer Service:Oracle卷映射拷贝写入服务,VSS(Volume Shadow Copy Ser ...
- windows 下svn 创建分支 合并分支 冲突
我用的系统是win7+Subversion 1.7.4.服务器搭建就略过了,我也是从网上找的,基本上就是几个命令吧!我用的CentOs6.5 .网上找了几个命令搭建很快,基本上是: 1.# sudo ...
- SSL使用windows证书库中证书实现双向认证
前一段时间对OpenSSL库中的SSL通讯稍微琢磨了一下,在百度文库中找了个示例程序,然后在机器上跑,哇塞,运行成功!那时那个惊喜啊,SSL蛮简单的嘛.前几天,老板要我整一个SSL通讯,要使用wind ...
- Nmap命令的29个实用范例
Nmap即网络映射器对Linux系统/网络管理员来说是一个开源且非常通用的工具.Nmap用于在远程机器上探测网络,执行安全扫描,网络审计和搜寻开放端口.它会扫描远程在线主机,该主机的操作系统,包过滤器 ...
- Tortoise SVN Clean up失败的解决方法
step1: 到 sqlite官网 (http://www.sqlite.org/download.html) 下载 sqlite3.exe (找到 Precompiled Binaries for ...
- HAML学习
来源:http://ningandjiao.iteye.com/blog/1772845 一个技术能够风靡,一定是有它的原因的,在熟悉之前,我们没有资格去对它做任何的判断. Haml 是一种简洁优美的 ...
- (C#) 发布程序,包含某些配置文件或数据文件。
在VS2012里面,右击需要发布的Project,选择“Properties“, 在弹出的窗口里面点选”Publish“, 再点击”Application Files“, 将默认的Publish St ...
- Redis 内存使用优化与存储
Redis 常用数据类型 Redis 最为常用的数据类型主要有以下五种: • String • Hash • List • Set • Sorted set 在具体描述这几种数据类型之前,我们先通过一 ...
- HDU 1532 (Dinic算法)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1532 题目大意: 就是由于下大雨的时候约翰的农场就会被雨水给淹没,无奈下约翰不得不修建水沟,而且是网络 ...