thrift学习笔记
Thrift学习笔记
一:thrift介绍
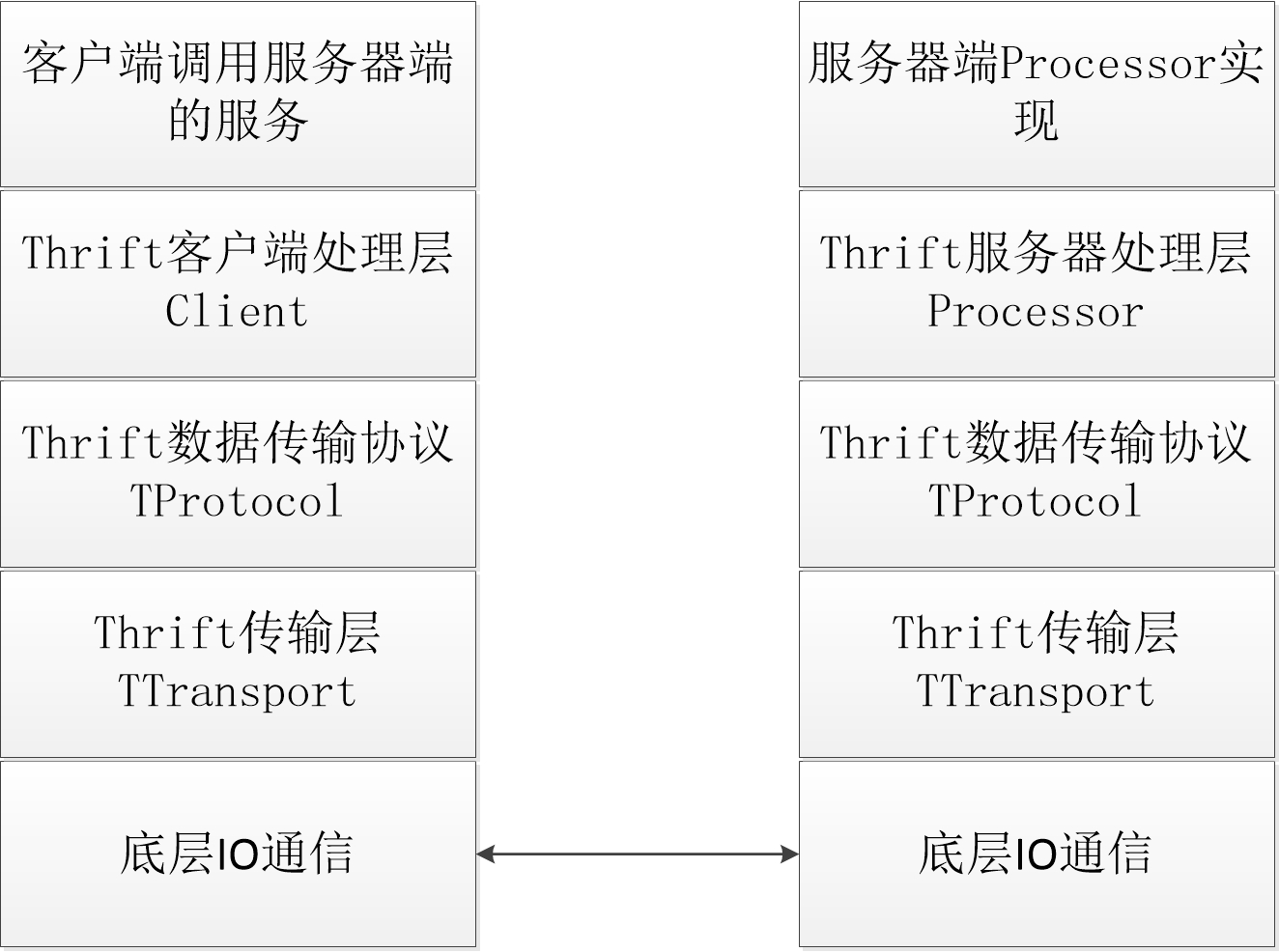
二 thrift数据传输协议
三 thrift传输层
四 thrift服务器端
五 thrift客户端
六 thrift开发步骤
1服务器端
2客户端
七 thrift数据类型
1基本类型
bool:布尔值,true 或 false
byte:8 位有符号整数
i16:16 位有符号整数
i32:32 位有符号整数
i64:64 位有符号整数
double:64 位浮点数
string:utf-8编码的字符串
2结构体类型
struct:定义公共的对象
enum: 枚举类型
3容器类型
list:对应 Java 的 ArrayList
set:对应 Java 的 HashSet
map:对应 Java 的 HashMap
4异常类型
exception:对应 Java 的 Exception
5服务类型
service:对应服务的类 提供接口
八 thrift例子
enum 类型
struct Student{
1: required i32 id
2: required string username
3: required string password
4: requried string number
5: optional double age
}
struct 类型
struct School{
1: required i32 id
2: required string name
3: required set<Student> students
4: required list<Student> rank
5: required map<string, string> number_name
}
service 类型
service ThriftMysqlService{
void addUser(1:Student user)
list<Student> queryAllUser()
Student queryOneUser(1:i32 id)
map<string, string> queryOneArticle(1:i32 id)
}
具体代码
thrift.thrift 定义数据类型和接口的文件
namespace java org.seava.thrift_example.thrift struct User{
1: required i32 userId
2: required string username
3: required string password
} service ThriftService{
void addUser(1:User user)
User queryUser(1:i32 id)
list<User> queryUserList()
map<string, string> queryUserNamePass()
map<i32, User> queryUserMap()
}
到apache的官网下载thrift.exe程序, 下载地址 http://thrift.apache.org/ ,下下来通过cmd命令窗口去运行如下命令
thrift -gen java xxx.thrift
接口实现 ThriftServiceImpl.java
package org.seava.thrift_example.thrift; import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map; public class ThriftServiceImpl implements ThriftService.Iface { public void addUser(User user) throws org.apache.thrift.TException{
System.out.println(user.userId + " " + user.username + " " + user.password);
} public User queryUser(int id) throws org.apache.thrift.TException{
System.out.println(id);
User user = new User();
user.userId = 100;
user.username = "FFF";
user.password = "NNN";
return user;
} public List<User> queryUserList() throws org.apache.thrift.TException{
User user = new User();
user.userId = 100;
user.username = "FFF";
user.password = "NNN";
User user2 = new User();
user2.userId = 102;
user2.username = "FFF2";
user2.password = "NNN2";
List<User> list = new ArrayList<User>();
list.add(user2);
list.add(user);
return list;
} public Map<String,String> queryUserNamePass() throws org.apache.thrift.TException{
User user = new User();
user.userId = 100;
user.username = "FFF";
user.password = "NNN";
Map<String, String> map = new HashMap<String, String>();
map.put("password", user.password);
map.put("useranme", user.username);
return map;
} public Map<Integer,User> queryUserMap() throws org.apache.thrift.TException{
User user = new User();
user.userId = 100;
user.username = "FFF";
user.password = "NNN";
User user2 = new User();
user2.userId = 102;
user2.username = "FFF2";
user2.password = "NNN2";
Map<Integer, User> map = new HashMap<Integer, User>();
map.put(user.userId, user);
map.put(user2.userId, user2);
return map;
} }
服务器 Server.java
package org.seava.thrift_example.thrift; import org.apache.thrift.TProcessor;
import org.apache.thrift.protocol.TBinaryProtocol;
import org.apache.thrift.protocol.TCompactProtocol;
import org.apache.thrift.server.THsHaServer;
import org.apache.thrift.server.TNonblockingServer;
import org.apache.thrift.server.TServer;
import org.apache.thrift.server.TSimpleServer;
import org.apache.thrift.server.TThreadPoolServer;
import org.apache.thrift.transport.TFramedTransport;
import org.apache.thrift.transport.TNonblockingServerSocket;
import org.apache.thrift.transport.TNonblockingServerTransport;
import org.apache.thrift.transport.TServerSocket;
import org.apache.thrift.transport.TTransportException; public class Server { public static int port = 8090; /**
* 简单服务器类型 阻塞单线程
* 步骤
* 创建TProcessor
* 创建TServerTransport
* 创建TProtocol
* 创建TServer
* 启动Server
*/
public static void startSimpleServer(){
//创建processor
TProcessor tprocessor = new ThriftService.Processor<ThriftService.Iface>(new ThriftServiceImpl());
try {
//创建transport 阻塞通信
TServerSocket serverTransport = new TServerSocket(port);
//创建protocol
TBinaryProtocol.Factory protocol = new TBinaryProtocol.Factory();
//将processor transport protocol设入到服务器server中
TServer.Args args = new TServer.Args(serverTransport);
args.processor(tprocessor);
args.protocolFactory(protocol);
//定义服务器类型 设定参数
TServer server = new TSimpleServer(args);
//开启服务
server.serve();
} catch (TTransportException e) {
e.printStackTrace();
}
} /**
* 多线程服务器 阻塞多线程
*/
public static void startThreadPoolServer(){
//创建processor
TProcessor tprocessor = new ThriftService.Processor<ThriftService.Iface>(new ThriftServiceImpl());
try{
//创建transport 阻塞通信
TServerSocket serverTransport = new TServerSocket(port);
//创建protocol 数据传输协议
TBinaryProtocol.Factory protocol = new TBinaryProtocol.Factory();
TThreadPoolServer.Args args = new TThreadPoolServer.Args(serverTransport);
args.processor(tprocessor);
args.protocolFactory(protocol);
//创建服务器类型 多线程
TServer server = new TThreadPoolServer(args);
//开启服务
server.serve();
}catch(Exception e){
e.printStackTrace();
}
} /**
* 非阻塞I/O
*/
public static void startTNonblockingServer(){
//创建processor
TProcessor tprocessor = new ThriftService.Processor<ThriftService.Iface>(new ThriftServiceImpl());
try{
//创建transport 非阻塞 nonblocking
TNonblockingServerTransport serverTransport = new TNonblockingServerSocket(port);
//创建protocol 数据传输协议
TCompactProtocol.Factory protocol = new TCompactProtocol.Factory();
//创建transport 数据传输方式 非阻塞需要用这种方式传输
TFramedTransport.Factory transport = new TFramedTransport.Factory();
TNonblockingServer.Args args = new TNonblockingServer.Args(serverTransport);
args.processor(tprocessor);
args.transportFactory(transport);
args.protocolFactory(protocol);
//创建服务器 类型是非阻塞
TServer server = new TNonblockingServer(args);
//开启服务
server.serve();
}catch(Exception e){
e.printStackTrace();
}
} /**
* 半同步半异步的非阻塞I/O
*/
public static void startTHsHaServer(){
//创建processor
TProcessor tprocessor = new ThriftService.Processor<ThriftService.Iface>(new ThriftServiceImpl());
try{
//创建transport 非阻塞
TNonblockingServerTransport serverTransport = new TNonblockingServerSocket(port);
//非阻塞需要的传输方式
TFramedTransport.Factory transport = new TFramedTransport.Factory();
//数据传输协议
TCompactProtocol.Factory protocol = new TCompactProtocol.Factory();
//创建半同步半异步服务
THsHaServer.Args args = new THsHaServer.Args(serverTransport);
args.processor(tprocessor);
args.transportFactory(transport);
args.protocolFactory(protocol);
//创建 服务类型
TServer server = new THsHaServer(args);
//开启服务
server.serve();
}catch(Exception e){
e.printStackTrace();
}
} public static void main(String args[]){
//开启简单服务器
// Server.startSimpleServer();
//开启多线程服务器
// Server.startThreadPoolServer();
// Server.startTNonblockingServer();
// Server.startTHsHaServer();
Server.startTNonblockingServer();
}
}
Server.java实现了简单服务器(阻塞单线程) 阻塞多线程 非阻塞 半同步半异步非阻塞
注意: 非阻塞时传输层需要选择TFramedTransport
客户端 Client.java
package org.seava.thrift_example.thrift; import java.util.List;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit; import org.apache.thrift.async.TAsyncClientManager;
import org.apache.thrift.protocol.TBinaryProtocol;
import org.apache.thrift.protocol.TCompactProtocol;
import org.apache.thrift.protocol.TProtocol;
import org.apache.thrift.protocol.TProtocolFactory;
import org.apache.thrift.transport.TFramedTransport;
import org.apache.thrift.transport.TNonblockingSocket;
import org.apache.thrift.transport.TNonblockingTransport;
import org.apache.thrift.transport.TSocket;
import org.apache.thrift.transport.TTransport; public class Client implements Runnable { public static String ip = "localhost";
public static int port = 8090;
public static int time_out = 30000; /**
* 客户端设置
* 创建Transport
* 创建TProtocol
* 基于TTransport和TProtocol创建Client
* 调用Client的相应方法
*/
public static void startSimpleClient(){
TTransport transport = null;
try{
//创建Transport
transport = new TSocket(ip, port, time_out);
//创建TProtocol
TProtocol protocol = new TBinaryProtocol(transport);
//基于TTransport和TProtocol创建Client
ThriftService.Client client = new ThriftService.Client(protocol);
transport.open();
//调用client方法
List<User> list = client.queryUserList();
for(User user : list){
System.out.println(user.userId + " " + user.username + " " + user.password);
}
Map<String, String> map = client.queryUserNamePass();
System.out.println(map);
User user = client.queryUser(10);
System.out.println(user.userId + " " + user.username + " " + user.password);
Map<Integer, User> map_u = client.queryUserMap();
System.out.println(map_u);
User uu = new User();
uu.userId = 1111;
uu.username = "mmbbmmbb";
uu.password = "ppbbppbb";
client.addUser(uu);
}catch(Exception e){
e.printStackTrace();
}
} /**
* 调用阻塞服务器的客户端
*/
public static void startNonblockingClient(){
TTransport transport = null;
try{
transport = new TFramedTransport(new TSocket(ip, port));
TCompactProtocol protocol = new TCompactProtocol(transport);
ThriftService.Client client = new ThriftService.Client(protocol);
transport.open();
//调用client方法
List<User> list = client.queryUserList();
for(User user : list){
System.out.println(user.userId + " " + user.username + " " + user.password);
}
Map<String, String> map = client.queryUserNamePass();
System.out.println(map);
User user = client.queryUser(10);
System.out.println(user.userId + " " + user.username + " " + user.password);
Map<Integer, User> map_u = client.queryUserMap();
System.out.println(map_u);
User uu = new User();
uu.userId = 1111;
uu.username = "mmbbmmbb";
uu.password = "ppbbppbb";
client.addUser(uu);
}catch(Exception e){
e.printStackTrace();
}
} public static void startAsynClient(){
try{
TAsyncClientManager clientManager = new TAsyncClientManager();
TNonblockingTransport transport = new TNonblockingSocket(ip, port, time_out);
TProtocolFactory tprotocol = new TCompactProtocol.Factory();
ThriftService.AsyncClient asyncClient = new ThriftService.AsyncClient(tprotocol, clientManager, transport);
System.out.println("Client start ...");
CountDownLatch latch = new CountDownLatch(1);
AsynCallback callBack = new AsynCallback(latch);
System.out.println("call method queryUser start ...");
asyncClient.queryUser(100, callBack);
System.out.println("call method queryUser end");
boolean wait = latch.await(30, TimeUnit.SECONDS);
System.out.println("latch.await =:" + wait);
}catch(Exception e){
e.printStackTrace();
}
} public void run(){
Client.startSimpleClient();
} public static void main(String args[]){
//调用简单服务器
// Client.startSimpleClient();
/*Client c1 = new Client();
Client c2 = new Client(); new Thread(c1).start();
new Thread(c2).start();*/ // Client.startNonblockingClient();
// Client.startNonblockingClient();
Client.startAsynClient();
}
}
客户端实现了 阻塞单线程 和 异步客户端
具体代码在github上:https://github.com/WaterHsu/thrift-example.git
thrift学习笔记的更多相关文章
- Thrift学习笔记—IDL基本类型
thrift 采用IDL(Interface Definition Language)来定义通用的服务接口,并通过生成不同的语言代理实现来达到跨语言.平台的功能.在thrift的IDL中可以定义以下一 ...
- 学习笔记:The Log(我所读过的最好的一篇分布式技术文章)
前言 这是一篇学习笔记. 学习的材料来自Jay Kreps的一篇讲Log的博文. 原文很长,但是我坚持看完了,收获颇多,也深深为Jay哥的技术能力.架构能力和对于分布式系统的理解之深刻所折服.同时也因 ...
- springmvc学习笔记--Interceptor机制和实践
前言: Spring的AOP理念, 以及j2ee中责任链(过滤器链)的设计模式, 确实深入人心, 处处可以看到它的身影. 这次借项目空闲, 来总结一下SpringMVC的Interceptor机制, ...
- 学习笔记:The Log(我所读过的最好的一篇分布式技术文章)
前言 这是一篇学习笔记. 学习的材料来自Jay Kreps的一篇讲Log的博文. 原文非常长.可是我坚持看完了,收获颇多,也深深为Jay哥的技术能力.架构能力和对于分布式系统的理解之深刻所折服.同一时 ...
- Dubbo -- 系统学习 笔记 -- 依赖
Dubbo -- 系统学习 笔记 -- 目录 依赖 必需依赖 缺省依赖 可选依赖 依赖 必需依赖 JDK1.5+ 理论上Dubbo可以只依赖JDK,不依赖于任何三方库运行,只需配置使用JDK相关实现策 ...
- spring cloud 学习(二)关于 Eureka 的学习笔记
关于 Eureka 的学习笔记 个人博客地址 : https://zggdczfr.cn/ ,欢迎光临~ 前言 Eureka是Netflix开发的服务发现组件,本身是一个基于REST的服务.Sprin ...
- SparkSQL学习笔记
概述 冠状病毒来临,宅在家中给国家做贡献之际,写一篇随笔记录SparkSQL的学习笔记,目的有二,一是记录整理之前的知识作为备忘录,二是分享技术,大家共同进步,有问题也希望大家不吝赐教.总体而言,大数 ...
- js学习笔记:webpack基础入门(一)
之前听说过webpack,今天想正式的接触一下,先跟着webpack的官方用户指南走: 在这里有: 如何安装webpack 如何使用webpack 如何使用loader 如何使用webpack的开发者 ...
- PHP-自定义模板-学习笔记
1. 开始 这几天,看了李炎恢老师的<PHP第二季度视频>中的“章节7:创建TPL自定义模板”,做一个学习笔记,通过绘制架构图.UML类图和思维导图,来对加深理解. 2. 整体架构图 ...
随机推荐
- java多线程学习笔记——详细
一.线程类 1.新建状态(New):新创建了一个线程对象. 2.就绪状态(Runnable):线程对象创建后,其他线程调用了该对象的start()方法.该状态的线程位于可运行线程池中, ...
- 七中滤波方法测试matlab实现
http://blog.163.com/xiaheng0804@126/blog/static/1205282120132129471816/ 创建两个混合信号,便于更好测试滤波器效果.同时用七中滤波 ...
- 软件测试技术(三)——使用因果图法进行的UI测试
目标程序 较上次增加两个相同的输入框 使用方法介绍 因果图法 在Introduction to Software Testing by Paul一书中,将软件测试的覆盖标准划分为四类,logical ...
- JavaScript相关图书推荐
JavaScript语言精粹(修订版) 作 者 Douglas Crockford(道格拉斯·克罗克福德) 著:赵泽欣 等 译 出 版 社 电子工业出版社 出版时间 2012-09-01 版 ...
- bzoj 2599 [IOI2011]Race (点分治)
[题意] 问树中长为k的路径中包含边数最少的路径所包含的边数. [思路] 统计经过根的路径.假设当前枚举到根的第S个子树,若x属于S子树,则有: ans<-dep[x]+min{ dep[y] ...
- 大连网络赛 1006 Football Games
//大连网络赛 1006 // 吐槽:数据比较水.下面代码可以AC // 但是正解好像是:排序后,前i项的和大于等于i*(i-1) #include <bits/stdc++.h> usi ...
- 发布 asp.net网站 到本地IIS
http://blog.csdn.net/jiben2qingshan/article/details/9249139 概述 网站是由一个个页面组成的,是万维网具体的变现形式,关于万维网,网页的方面的 ...
- data audit on hadoop fs
最近项目中遇到了存储在HDFS上的数据格式不对,是由于数据中带有\r\n的字符,程序处理的时候没有考虑到这些情况.历史数据大概有一年的时间,需要把错误的数据或者重复的数据给删除了,保留正确的数据,项目 ...
- 【Hadoop代码笔记】Hadoop作业提交之Child启动reduce任务
一.概要描述 在上篇博文描述了TaskTracker启动一个独立的java进程来执行Map任务.接上上篇文章,TaskRunner线程执行中,会构造一个java –D** Child address ...
- Linux 命令之last命令详解
last:命令解释show listing of last logged in users 指令所在路径:/usr/bin/last 命令输出字段介绍: 第一列:用户名 第二列:终端位置.pts/0 ...