Understanding the JavaScript Engine—— two phase
Understanding the JavaScript Engine — Part 1

I have been a Ruby on Rails developer for the last 2 years. I have used JavaScript both in its vanilla form and in some frameworks. However, I learned JavaScript as most new programmers do, by going through a course, without quite understanding how the JavaScript engine works.
Before diving deep into JavaScript, I decided to take some time off and understand its core working principles. I’ll be sharing what I’ve learned so far in this, and subsequent blog posts.
First, let me define some terms you’ll come across. I’ll add examples where necessary.
Syntax Parser
When you write code, a compiler converts your code into a set of instructions that the computer can understand. Part of the compiler is what is known as a syntax parser. The syntax parser goes through your code character by character, and determines if the syntax is valid or not.
Lexical Environment
In simple terms, a Lexical environment refers to where something sits physically in your code. Where something is written gives an idea of how the computer will interpret it and how it will interact with other variables and functions e.g
function hello() {
var greet = "Hello world"
}
In the function above, we can say that var greet
sits lexically in the function.
Execution Context
This is a wrapper that helps manage the code that is running. Looking at the gif below, you can see there’s an execution context stack and in it, we have a Global execution context. When functionA()
is called, it is added to the Stack meaning that functionA()
is currently being executed. The same goes for functionB()
.
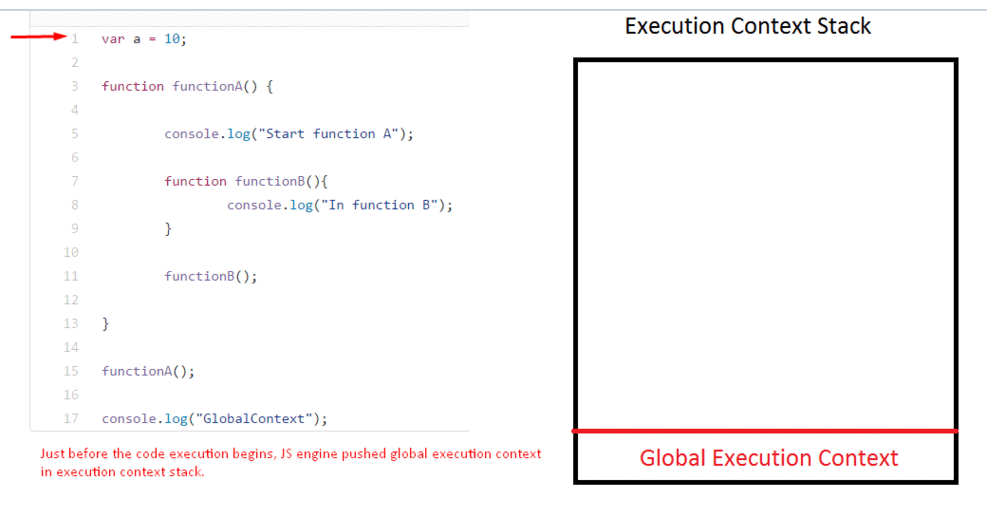
The execution context is created in two phases:
The first phase is called the creation phase. The global execution context creates two things for you, that you don’t have in your code; a global object(window)
and a special variable called this
. The window
object is a global object inside a browser. This object is different depending on whether you are using node or running JavaScript on the server. But there is always a global object when you’re running JavaScript. Take a look at the following image from a browser console:
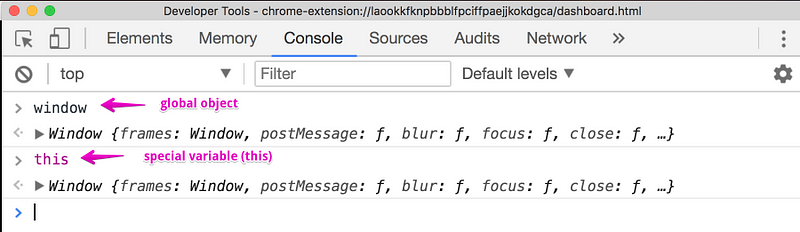
When you create a variable and function that is not inside a function, those variables and functions get attached to the global object.
var name = "Debby"; function greet() {
console.log("Hello", name)
}
If you run the above JavaScript code in the browser and you inspect the global object, you will see that the variable and the function were added to it.
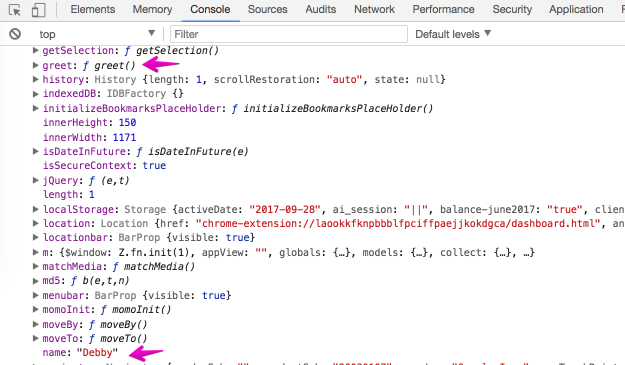
During the creation phase, the syntax parser recognizes where you have defined variables and functions. It therefore sets up memory space for the variables and functions. It’s not actually moving code to the top of the page. What this means is that before your code begins to be executed line by line, the JavaScript engine has already set aside memory space for the variables and functions that you’ve written. This is what is called Hoisting.
The next phase is the execution phase, where assignments are made. When the JavaScript engine sets up memory space for variables, it doesn’t know which values will be stored in them. Therefore, it puts a placeholder called undefined
. That placeholder means; I don’t know what this value is yet. All variables in JavaScript are initially set to undefined
while functions sit in memory in their entirety. This is why it’s possible to declare a variable without assigning it and the JavaScript engine will not throw any error.
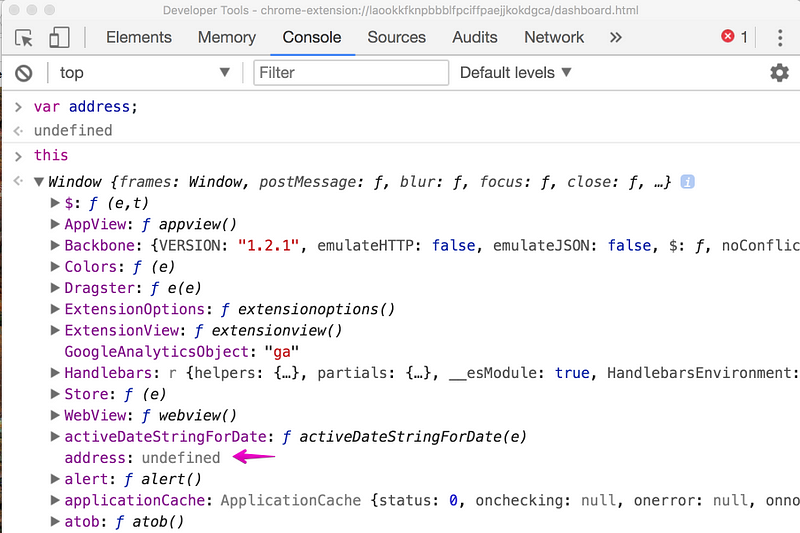
This is just a brief introduction to how the JavaScript engine executes the code you write. To know more, you can use the resources below:
JavaScript: Understanding the Weird Parts by Anthony Alicea on Udemy.Execution context, Scope chain and JavaScript internals by Rupesh Mishra.
Understanding the JavaScript Engine—— two phase的更多相关文章
- 「2014-3-13」Javascript Engine, Java VM, Python interpreter, PyPy – a glance
提要: url anchor (ajax) => javascript engine (1~4 articles) => java VM vs. python interpreter =& ...
- Javascript Engine, Java VM, Python interpreter, PyPy – a glance
提要: url anchor (ajax) => javascript engine (1~4 articles) => java VM vs. python interpreter =& ...
- Browser Render Engine & Javascript Engine
Browser Render Engine Programming Language Open Source Javascript Engine Comparation for CSS Compati ...
- JavaScript Engine 可视化
JavaScript Engine 可视化 图解 JavaScript Engine JavaScript 可视化 (7 部曲) ️ JavaScript Visualized: Event Loop
- Understanding Delegated JavaScript Events
While I ended up using a CSS-only implementation for this pen, I started by writing it mostly using ...
- v8 javascript engine
https://code.google.com/p/v8-wiki/wiki/BuildingWithGYP vs2013git v8 http://github.com/v8/v8-git-mirr ...
- Attacking JavaScript Engines: A case study of JavaScriptCore and CVE-2016-4622(转)
转:http://phrack.org/papers/attacking_javascript_engines.html Title : Attacking JavaScript Engines: A ...
- Bring JavaScript to your Java enterprise with Vert.x
转自:https://opensource.com/article/18/4/benefits-javascript-vertx If you are a Java programmer, chanc ...
- JavaScript Interview Questions: Event Delegation and This
David Posin helps you land that next programming position by understanding important JavaScript fund ...
随机推荐
- STL容器 -- Bitset
核心内容:Bitset 是 STL 中的二进制容器, 存放的时 bit 位元素, 每一位只占一个 bit 位, 取值 0 或者 1, 可以像整形元素一样按位与或非, 并且大大优化了时间和空间复杂度. ...
- 借助jxl将Excel中的数据注入到Bean中
前言 使用了Hibernate的项目中需要导入多张表的数据,但是我又不想写多次取出Excle数据放到Bean里的代码,于是写了个ExcleUtils来帮助我做这件事. 基本思路 技术上,首先肯定是要借 ...
- BZOJ3262陌上花开(三维偏序问题(CDQ分治+树状数组))+CDQ分治基本思想
emmmm我能怎么说呢 CDQ分治显然我没法写一篇完整的优秀的博客,因为我自己还不是很明白... 因为这玩意的思想实在是太短了: fateice如是说道: 如果说对于一道题目的离线操作,假设有n个操作 ...
- /etc/default/useradd配置文件详解
/etc/default/useradd文件内容如下: [xf@xuexi ~]$ cat /etc/default/useradd # useradd defaults file GROUP=100 ...
- ssvm和console 模板机 连接不上管理节点
说明: cloudstack 版本http://www.shapeblue.com/packages/ 并不是官方的 systemvm64template-4.6.0-vmware.ova 官 ...
- Opencv学习笔记1:安装opencv和VS2015并进行环境配置
用了Opencv一段时间了,简单记录一下opencv在vs2015下的配置. 第一部分:OpenCV3.2.0的下载 OpenCV官方下载地址: https://opencv.org/releases ...
- 51nod1515 明辨是非 并查集 + set
一开始想的时候,好像两个并查集就可以做......然后突然懂了什么.... 相同的并查集没有问题,不同的就不能并查集了,暴力的来个set就行了..... 合并的时候启发式合并即可做到$O(n \log ...
- [APIO2015]巴厘岛的雕塑 --- 贪心 + 枚举
[APIO2015]巴厘岛的雕塑 题目描述 印尼巴厘岛的公路上有许多的雕塑,我们来关注它的一条主干道. 在这条主干道上一共有\(N\)座雕塑,为方便起见,我们把这些雕塑从 1 到\(N\)连续地进行 ...
- [NOIP2017]列队(线段树/裂点splay)
考虑n=1的做法,就是支持: 1.在线删一个数 2.在结尾加一个数 3.查询序列的第y个数 用线段树记录区间内被删元素的个数,可以通过线段树上二分快速得解,对于新增的数,用vector记录即可. 对于 ...
- 背包的第k优解[动态规划]
From easthong ☆背包的第k优解 描述 Description DD 和好朋友们要去爬山啦!他们一共有 K 个人,每个人都会背一个包.这些包的容量是 ...