uva 11374 最短路+记录路径 dijkstra最短路模板
UVA - 11374
Time Limit:1000MS | Memory Limit:Unknown | 64bit IO Format:%lld & %llu |
[Submit] [Go Back] [ id=22966" style="color:rgb(106,57,6); text-decoration:none">Status
Description
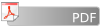
ProblemD: Airport Express |
In a small city called Iokh, a train service, Airport-Express, takes residents to the airport more quickly than other transports. There are two types of trains in Airport-Express, theEconomy-Xpress and theCommercial-Xpress.
They travel at different speeds, take different routes and have different costs.
Jason is going to the airport to meet his friend. He wants to take the Commercial-Xpress which is supposed to be faster, but he doesn't have enough money. Luckily he has a ticket for the Commercial-Xpress which can take him one station forward. If he used
the ticket wisely, he might end up saving a lot of time. However, choosing the best time to use the ticket is not easy for him.
Jason now seeks your help. The routes of the two types of trains are given. Please write a program to find the best route to the destination. The program should also tell when the ticket should be used.
Input
The input consists of several test cases. Consecutive cases are separated by a blank line.
The first line of each case contains 3 integers, namely N,S andE (2 ≤N ≤ 500, 1 ≤S,E ≤N),
which represent the number of stations, the starting point and where the airport is located respectively.
There is an integer M (1 ≤ M ≤ 1000) representing the number of connections between the stations of the Economy-Xpress. The nextM lines give the information of the routes of the
Economy-Xpress. Each consists of three integersX, Y and Z (X,Y ≤N, 1 ≤Z ≤
100). This meansX andY are connected and it takesZ minutes to travel between these two stations.
The next line is another integer K (1 ≤ K ≤ 1000) representing the number of connections between the stations of the Commercial-Xpress. The nextK lines contain the information
of the Commercial-Xpress in the same format as that of the Economy-Xpress.
All connections are bi-directional. You may assume that there is exactly one optimal route to the airport. There might be cases where you MUST use your ticket in order to reach the airport.
Output
For each case, you should first list the number of stations which Jason would visit in order. On the next line, output "TicketNot Used" if you decided NOT to use the ticket; otherwise, state the station where Jason should get on the train
of Commercial-Xpress. Finally, print thetotal time for the journey on the last line. Consecutive sets of output must be separated by a blank line.
Sample Input
4 1 4
4
1 2 2
1 3 3
2 4 4
3 4 5
1
2 4 3
Sample Output
1 2 4
2
5
Problemsetter: Raymond Chun
Originally appeared in CXPC, Feb. 2004
http://acm.hust.edu.cn/vjudge/problem/viewProblem.action?id=22966
由于仅仅能做一次商业线。我们能够枚举商业线T(a,b),则总时间为f(a)+T(a,b)+g(b);f和g用两次dijkstra来计算,以S为起点的dijkstra和以E为起点的dijkstra;
#include <iostream>
#include <cstdio>
#include <vector>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std;
const int MAXN = 505;
const int INF = 0x3f3f3f3f;
struct Edge {
int from, to, dist;
};
struct HeapNode {
int d, u;
bool operator< (const HeapNode rhs) const {
return d > rhs.d;
}
};
struct Dijkstra {
int n, m; // 点数和边数
vector<Edge> edges; //边列表
vector<int> G[MAXN]; // 每一个点出发的边编号(0開始)
bool done[MAXN]; // 是否已标记
int d[MAXN]; //s 到各个点的距离
int p[MAXN]; //最短路中上一个点,也能够是上一条边
void init(int n) {
this->n = n;
for (int i = 0; i < n; i++)
G[i].clear();
edges.clear();
}
void AddEdge(int from, int to, int dist) {
edges.push_back((Edge){from, to, dist});
m = edges.size();
G[from].push_back(m-1);
}
void dijkstra(int s) {
priority_queue<HeapNode> Q;
for (int i = 0; i < n; i++)
d[i] = INF;
d[s] = 0;
memset(done, 0, sizeof(done));
Q.push((HeapNode){0, s});
while (!Q.empty()) {
HeapNode x = Q.top();
Q.pop();
int u = x.u;
if (done[u])
continue;
done[u] = true;
for (int i = 0; i < G[u].size(); i++) {
Edge &e = edges[G[u][i]];
if (d[e.to] > d[u] + e.dist) {
d[e.to] = d[u] + e.dist;
p[e.to] = e.from;
Q.push((HeapNode){d[e.to], e.to});
}
}
}
}
void getPath(vector<int> &path, int s, int e) {
int cur = e;
while (1) {
path.push_back(cur);
if (cur == s)
return ;
cur = p[cur];
}
}
};
int n, m, k, s, e;
int x, y, z;
vector<int> path;
int main() {
int first = 1;
while (scanf("%d%d%d", &n, &s, &e) != EOF) {
if (first)
first = 0;
else printf("\n");
s--, e--;
Dijkstra ans[2];
ans[0].init(n);
ans[1].init(n);
scanf("%d", &m);
while (m--) {
scanf("%d%d%d", &x, &y, &z);
x--, y--;
ans[0].AddEdge(x, y, z);
ans[0].AddEdge(y, x, z);
ans[1].AddEdge(x, y, z);
ans[1].AddEdge(y, x, z);
}
ans[0].dijkstra(s);
ans[1].dijkstra(e);
scanf("%d", &k);
path.clear();
int Min = ans[0].d[e];
int flagx = -1, flagy = -1;
while (k--) {
scanf("%d%d%d", &x, &y, &z);
x--, y--;
if (Min > ans[0].d[x] + z + ans[1].d[y]) {
Min = ans[0].d[x] + z + ans[1].d[y];
flagx = x, flagy = y;
}
if (Min > ans[1].d[x] + z + ans[0].d[y]) {
Min = ans[1].d[x] + z + ans[0].d[y];
flagx = y, flagy = x;
}
}
if (flagx == -1) //推断是否须要坐商业线
{
ans[0].getPath(path, s, e);
reverse(path.begin(), path.end());
for (int i = 0; i < path.size()-1; i++)
printf("%d ", path[i]+1);
printf("%d\n", path[path.size()-1]+1);
printf("Ticket Not Used\n");
printf("%d\n", Min);
}
else {
ans[0].getPath(path, s, flagx);
reverse(path.begin(), path.end());
ans[1].getPath(path, e, flagy);
for (int i = 0; i < path.size()-1; i++)
printf("%d ", path[i]+1);
printf("%d\n", path[path.size()-1]+1);
printf("%d\n", flagx+1);
printf("%d\n", Min);
}
}
return 0;
}
uva 11374 最短路+记录路径 dijkstra最短路模板的更多相关文章
- HDOJ 5294 Tricks Device 最短路(记录路径)+最小割
最短路记录路径,同一时候求出最短的路径上最少要有多少条边, 然后用在最短路上的边又一次构图后求最小割. Tricks Device Time Limit: 2000/1000 MS (Java/Oth ...
- UVA 624(01背包记录路径)
https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem& ...
- E - Coin Change UVA - 674 &&(一些记录路径的方法)
这一道题并不难,我们只需要将dp数组先清空,再给dp[0]=1,之后就按照完全背包的模板写 主要是我们要证明着一种方法不会出现把(1+3+4)(1+4+3)当作两种方法,这一点如果自己写过背包的那个表 ...
- hdu 4871 树的分治+最短路记录路径
/* 题意:给你一些节点和一些边,求最短路径树上是k个节点的最长的路径数. 解:1.求出最短路径树--spfa加记录 2.树上进行操作--树的分治,分别处理子树进行补集等运算 */ #include& ...
- UVA 11374 Halum (差分约束系统,最短路)
题意:给定一个带权有向图,每次你可以选择一个结点v 和整数d ,把所有以v为终点的边权值减少d,把所有以v为起点的边权值增加d,最后要让所有的边权值为正,且尽量大.若无解,输出结果.若可无限大,输出结 ...
- HDU 2544 - 最短路 - [堆优化dijkstra][最短路模板题]
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=2544 Time Limit: 5000/1000 MS (Java/Others) Memory Li ...
- PAT甲题题解-1030. Travel Plan (30)-最短路+输出路径
模板题最短路+输出路径如果最短路不唯一,输出cost最小的 #include <iostream> #include <cstdio> #include <algorit ...
- 训练指南 UVA - 11374(最短路Dijkstra + 记录路径 + 模板)
layout: post title: 训练指南 UVA - 11374(最短路Dijkstra + 记录路径 + 模板) author: "luowentaoaa" catalo ...
- UVA 11374 Airport Express 机场快线(单源最短路,dijkstra,变形)
题意: 给一幅图,要从s点要到e点,图中有两种无向边分别在两个集合中,第一个集合是可以无限次使用的,第二个集合中的边只能挑1条.问如何使距离最短?输出路径,用了第二个集合中的哪条边,最短距离. 思路: ...
随机推荐
- Java技术——多态的实现原理
.方法表与方法调用 如有类定义 Person, Girl, Boy class Person { public String toString(){ return "I'm a person ...
- day18-socket 编程
1.Socket是网络上的使用的交互信息得方法,也叫套接字 用于描述IP地址和端口,是一个通信链的句柄,应用程序通常通过"套接字"向网络发出请求或者应答网络请求. 通讯原理 Soc ...
- 使用 Button 类在 XNA 中创建图形按钮(九)
平方已经开发了一些 Windows Phone 上的一些游戏,算不上什么技术大牛.在这里分享一下经验,仅为了和各位朋友交流经验.平方会逐步将自己编写的类上传到托管项目中,没有什么好名字,就叫 WPXN ...
- Leetcode23--->Merge K sorted Lists(合并k个排序的单链表)
题目: 合并k个排序将k个已排序的链表合并为一个排好序的链表,并分析其时间复杂度 . 解题思路: 类似于归并排序的思想,lists中存放的是多个单链表,将lists的头和尾两个链表合并,放在头,头向后 ...
- [python学习篇][廖雪峰][4]函数--reduce
reduce的用法.reduce把一个函数作用在一个序列[x1, x2, x3...]上,这个函数必须接收两个参数,reduce把结果继续和序列的下一个元素做累积计算,其效果就是: reduce(f, ...
- xtrabackup: error: last checkpoint LSN (3409281307) is larger than last copied LSN (3409274368). #2
1.错误发生场景:使用2.4.1版本的xtrabackup工具进行全备,备份日志中报出此错误2.知识要点:MySQL中,redo 日志写进程会在三种条件下被触发从log buffer中写日志到redo ...
- mq类----2
手动应答方式 使用get my_consumer.php 消费者 生产者和上一篇 一样 <?php /** * Created by PhpStorm. * User: brady * Dat ...
- LaTeX:毕设模版设计问题1 各级标题编号设为黑体
我用'titlesec'宏包设计各级标题格式指定字体为黑体,结果标题编号仍为Times New Roman .
- BZOJ 3626 [LNOI2014]LCA ——树链剖分
思路转化很巧妙. 首先把询问做差分. 然后发现加入一个点就把路径上的点都+1,询问的时候直接询问到根的路径和. 这样和原问题是等价的,然后树链剖分+线段树就可以做了. #include <map ...
- Red is good(bzoj 1419)
Description 桌面上有R张红牌和B张黑牌,随机打乱顺序后放在桌面上,开始一张一张地翻牌,翻到红牌得到1美元,黑牌则付出1美元.可以随时停止翻牌,在最优策略下平均能得到多少钱. Input 一 ...