poj-1021--2D-Nim--点阵图同构
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 4136 | Accepted: 1882 |
Description
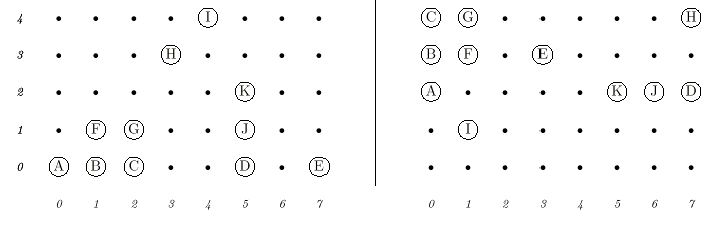
The player on move may remove (A), (B), (A, B), (A, B, C), or (B,F), etc., but may not remove (A, C), (D, E), (H, I) or (B, G).
For purposes of writing 2D-Nim-playing software, a certain programmer wants to be able to tell whether or not a certain position has ever been analyzed previously. Because of the rules of 2D-Nim, it should be clear that the two boards above are essentially equivalent. That is, if there is a winning strategy for the left board, the same one must apply to the right board. The fact that the contiguous groups of pieces appear in different places and orientations is clearly irrelevant. All that matters is that the same clusters of pieces (a cluster being a set of contiguous pieces that can be reached from each other by a sequence of one-square vertical or horizontal moves) appear in each. For example, the cluster of pieces (A, B, C, F, G) appears on both boards, but it has been reflected (swapping left and right), rotated, and moved. Your task is to determine whether two given board states are equivalent in this sense or not.
Input
Output
Sample Input
2
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 5 2 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 6 1 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
Sample Output
YES
NO
总结
就是连通性和图的同构判断。
分析
找出属于同一组的点很简单,DFS就可以搞定。图的同构可以用图的Hash来判断。这个不是我想出来的,是网上看来的:
∑i,]distance(pi,pj)∑i,]distance(pi,pj)
即同一组中所有点的距离加起来,这个数值做为这个图的哈希值。
/*
author:tonygsw
data:2018.8.7
account:zj1228
link:http://poj.org/problem?id=1020
*/
#define ll long long
#define IO ios::sync_with_stdio(false) #include<map>
#include<queue>
#include<math.h>
#include<vector>
#include<stdio.h>
#include<string.h>
#include<iostream>
#include<algorithm>
using namespace std;
class Node{
public:
int x,y;
};
Node node[];int hash1[],hash2[];
int matri[][];bool vis[][];
int w,h,num,len,len1,len2;int to[][]={,,-,,,,,-};
void init()
{
memset(matri,,sizeof(matri));
memset(vis,,sizeof(vis));
len1=len2=;
}
bool judge(int x,int y)
{
if(x>=&&x<w&&y>=&&y<h&&matri[x][y]&&(!vis[x][y]))
return true;
return false;
}
int dis(Node a,Node b)
{
return pow(a.x-b.x,)+pow(a.y-b.y,);
}
void bfs(int x,int y,int ju)
{
Node beg,nex;len=;
queue<Node>way;
beg.x=x,beg.y=y;
way.push(beg);
vis[x][y]=;
node[len++]=beg;
while(!way.empty())
{
beg=way.front();
way.pop();
for(int i=;i<;i++)
{
nex.x=beg.x+to[i][];
nex.y=beg.y+to[i][];
if(judge(nex.x,nex.y))
{
way.push(nex);
vis[nex.x][nex.y]=;
node[len++]=nex;
}
}
}
for(int i=;i<len;i++)
for(int j=;j<len;j++)
{
if(ju)hash1[len1++]=dis(node[i],node[j]);
else hash2[len2++]=dis(node[i],node[j]);
}
}
bool ans()
{
if(len1!=len2)return false;
else
{
sort(hash1,hash1+len1);
sort(hash2,hash2+len2);
for(int i=;i<len1;i++)
{
if(hash1[i]!=hash2[i])return false;
}
return true;
}
}
int main()
{
int t;
scanf("%d",&t);int x,y;
while(t--)
{
init();
scanf("%d%d%d",&w,&h,&num);
for(int i=;i<num;i++)
{
scanf("%d%d",&x,&y);
matri[x][y]=;
}
for(int i=;i<w;i++)
for(int j=;j<h;j++)
if(matri[i][j]&&(!vis[i][j]))
bfs(i,j,);
memset(matri,,sizeof(matri));
memset(vis,,sizeof(vis));
for(int i=;i<num;i++)
{
scanf("%d%d",&x,&y);
matri[x][y]=;
}
for(int i=;i<w;i++)
for(int j=;j<h;j++)
if(matri[i][j]&&(!vis[i][j]))
bfs(i,j,); if(ans())cout<<"YES"<<endl;
else cout<<"NO"<<endl;
}
return ;
}
/*
2
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 5 2 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
8 5 11
0 0 1 0 2 0 5 0 7 0 1 1 2 1 5 1 3 3 6 1 4 4
0 4 0 3 0 2 1 1 1 4 1 3 3 3 5 2 6 2 7 2 7 4
*/
poj-1021--2D-Nim--点阵图同构的更多相关文章
- poj 1021矩阵平移装换后是否为同一个矩阵
2D-Nim Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 3081 Accepted: 1398 Descriptio ...
- Georgia and Bob POJ - 1704 阶梯Nim
$ \color{#0066ff}{ 题目描述 }$ Georgia and Bob decide to play a self-invented game. They draw a row of g ...
- POJ 1704 Staircase Nim 阶梯博弈
#include<stdio.h> #include<string.h> #include<algorithm> using namespace std; int ...
- POJ 1021 2D-Nim
Description The 2D-Nim board game is played on a grid, with pieces on the grid points. On each move, ...
- POJ 1021 人品题
报告见代码.. #include <iostream> #include <cstdio> #include <cstring> #include <algo ...
- 一位学长的ACM总结(感触颇深)
发信人: fennec (fennec), 信区: Algorithm 标 题: acm 总结 by fennec 发信站: 吉林大学牡丹园站 (Wed Dec 8 16:27:55 2004) AC ...
- 狗狗40题~ (Volume C)
A - Triangles 记忆化搜索呗.搜索以某三角形为顶的最大面积,注意边界情况. #include <stdio.h> #include <cstring> #inclu ...
- Topographic ICA as a Model of Natural Image Statistics(作为自然图像统计模型的拓扑独立成分分析)
其实topographic independent component analysis 早在1999年由ICA的发明人等人就提出了,所以不算是个新技术,ICA是在1982年首先在一个神经生理学的背景 ...
- 【POJ】【2068】Nim
博弈论/DP 这是Nim?这不是巴什博奕的变形吗…… 我也不会捉啊,不过一看最多只有20个人,每人最多拿16个石子,总共只有8196-1个石子,范围好像挺小的,嗯目测暴力可做. so,记忆化搜索直接水 ...
- 【POJ】【2975】Nim
博弈论 我哭……思路错误WA了6次?(好像还有手抖点错……) 本题是要求Nim游戏的第一步必胜策略有几种. 一开始我想:先全部异或起来得到ans,从每个比ans大的堆里取走ans个即可,答案如此累计… ...
随机推荐
- SPOJ NICEBTRE - Nice Binary Trees(树 先序遍历)
传送门 Description Binary trees can sometimes be very difficult to work with. Fortunately, there is a c ...
- tf.nn.conv2d卷积函数之图片轮廓提取
一.tensorflow中二维卷积函数的参数含义:def conv2d(input, filter, strides, padding, use_cudnn_on_gpu=True, data_for ...
- [Linux] 006 命令格式与目录处理命令
1. 命令格式 命令 [-选项] [参数] 如,ls -la /etc 说明 个别命令使用不遵循此格式 当有多个选项时,可以写在一起 简化选项与完整选项 如,-a 为简化选项,--all 为完整选项 ...
- MyEclipse下Junit报错"The input type of the launch configuration"
MyEclipse下Junit运行测试用例的时候报错: "The input type of the launch configuration does not exist" 原因 ...
- Java 不被看好前景堪忧?可能是想多了!
Java技术栈 www.javastack.cn 优秀的Java技术公众号 来源:代码湾 Java发行二十多年来,尤其是在战胜C和C++成为最受程序员喜欢的编程语言之后,一直都是开发者的宠儿. 虽然斯 ...
- 修改ps工具栏字体大小
修改ps工具栏字体大小 先改电脑分辨率或者改首选项--界面---文字,退出后,重新打开,但你会发现问题还是没解决,我们接着往下 找到文件夹安装目录下的photoshops.exe启动文件(查找方法 ...
- Java JNA (三)—— 结构体使用及简单示例
JNA简介 JNA全称Java Native Access,是一个建立在经典的JNI技术之上的Java开源框架(https://github.com/twall/jna).JNA提供一组Java工具类 ...
- elasticsearch 深入 —— 全文检索
全文搜索 我们已经介绍了搜索结构化数据的简单应用示例,现在来探寻 全文搜索(full-text search) :怎样在全文字段中搜索到最相关的文档. 全文搜索两个最重要的方面是: 相关性(Relev ...
- 利用delegate来解决类之间相互引用问题(引用死锁)
类之间相互引用--类A中需要调用类B中的方法,同时,类B中又要调用类A中的方法.(也被称为引用死锁,通常会出现编译错误). 解决方法是,在类A中引用类B,并使类A成为类B的delegate,这样在类A ...
- springboot2.0+websocket集成【群发消息+单对单】(二)
https://blog.csdn.net/qq_21019419/article/details/82804921 版权声明:本文为博主原创文章,遵循 CC 4.0 by-sa 版权协议,转载请附上 ...