Hdu 2475-Box LCT,动态树
Box
Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 2813 Accepted Submission(s): 821
Jack can perform the “MOVE x y” operation to the boxes: take out box x; if y = 0, put it on the ground; Otherwise, put it inside box y. All the boxes inside box x remain the same. It is possible that an operation is illegal, that is, if box y is contained (directly or indirectly) by box x, or if y is equal to x.
In the following picture, box 2 and 4 are directly inside box 6, box 3 is directly inside box 4, box 5 is directly inside box 1, box 1 and 6 are on the ground.

The picture below shows the state after Jack performs “MOVE 4 1”:
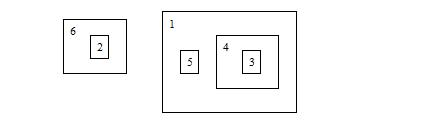
Then he performs “MOVE 3 0”, the state becomes:

During a sequence of MOVE operations, Jack wants to know the root box of a specified box. The root box of box x is defined as the most outside box which contains box x. In the last picture, the root box of box 5 is box 1, and box 3’s root box is itself.
For each test case, the first line has an integer N (1 <= N <= 50000), representing the number of boxes.
Next line has N integers: a1, a2, a3, ... , aN (0 <= ai <= N), describing the initial state of the boxes. If ai is 0, box i is on the ground, it is not contained by any box; Otherwise, box i is directly inside box ai. It is guaranteed that the input state is always correct (No loop exists).
Next line has an integer M (1 <= M <= 100000), representing the number of MOVE operations and queries.
On the next M lines, each line contains a MOVE operation or a query:
1. MOVE x y, 1 <= x <= N, 0 <= y <= N, which is described above. If an operation is illegal, just ignore it.
2. QUERY x, 1 <= x <= N, output the root box of box x.
0 1
5
QUERY 1
QUERY 2
MOVE 2 0
MOVE 1 2
QUERY 1
6
0 6 4 6 1 0
4
MOVE 4 1
QUERY 3
MOVE 1 4
QUERY 1
1
2
1
1
#include<bits/stdc++.h>
using namespace std;
#define MAXN 50010
struct node
{
int left,right;
}tree[MAXN];
int father[MAXN],rev[MAXN],Stack[MAXN],cc[MAXN];
int read()
{
int s=,fh=;char ch=getchar();
while(ch<''||ch>''){if(ch=='-')fh=-;ch=getchar();}
while(ch>=''&&ch<=''){s=s*+(ch-'');ch=getchar();}
return s*fh;
}
int isroot(int x)
{
return tree[father[x]].left!=x&&tree[father[x]].right!=x;
}
void pushdown(int x)
{
int l=tree[x].left,r=tree[x].right;
if(rev[x]!=)
{
rev[x]^=;rev[l]^=;rev[r]^=;
swap(tree[x].left,tree[x].right);
}
}
void rotate(int x)
{
int y=father[x],z=father[y];
if(!isroot(y))
{
if(tree[z].left==y)tree[z].left=x;
else tree[z].right=x;
}
if(tree[y].left==x)
{
father[x]=z;father[y]=x;tree[y].left=tree[x].right;tree[x].right=y;father[tree[y].left]=y;
}
else
{
father[x]=z;father[y]=x;tree[y].right=tree[x].left;tree[x].left=y;father[tree[y].right]=y;
}
}
void splay(int x)
{
int top=,y,z,i;Stack[++top]=x;
for(i=x;!isroot(i);i=father[i])Stack[++top]=father[i];
for(i=top;i>=;i--)pushdown(Stack[i]);
while(!isroot(x))
{
y=father[x];z=father[y];
if(!isroot(y))
{
if((tree[y].left==x)^(tree[z].left==y))rotate(x);
else rotate(y);
}
rotate(x);
}
}
void access(int x)
{
int last=;
while(x!=)
{
splay(x);
tree[x].right=last;
last=x;x=father[x];
}
}
void makeroot(int x)
{
access(x);splay(x);rev[x]^=;
}
void link(int u,int v)
{
makeroot(u);/*access(u);*/father[u]=v;splay(u);//////////////
}
void cut(int u,int v)
{
/*makeroot(u);*//*access(u);*/access(u);splay(u);father[tree[u].left]=;tree[u].left=;
}
int findroot(int x)
{
access(x);splay(x);
while(tree[x].left!=)x=tree[x].left;
return x;
}
int Findroot(int u,int v)
{
/*access(v);splay(v);*/access(v);splay(u);
while(tree[u].right!=)u=tree[u].right;
if(u==v)return ;
return ;
}
int main()
{
int n,i,m,k,x,y,a,tt=;
char fh[];
while(scanf("%d",&n)!=EOF)
{
if(tt)puts("");
tt++;
for(i=;i<=n;i++)
{
tree[i].left=tree[i].right=father[i]=rev[i]=cc[i]=;
}
for(i=;i<=n;i++)
{
a=read();
if(a!=)
{
link(i,a);
//cc[i]=a;
}
}
m=read();
for(i=;i<=m;i++)
{
scanf("\n%s",fh);
if(fh[]=='Q')
{
k=read();
printf("%d\n",findroot(k));
}
else
{
x=read();y=read();
if(y==)cut(x,y);
else if(x!=y)
{
if(Findroot(x,y)==)continue;
cut(x,y);link(x,y);
/*access(y);splay(x);
int t=x;
while(tree[t].right!=0)t=tree[t].right;
if(t!=y){cut(x,y);link(x,y);}*/
}
/*if(x!=0&&y!=0)
{
if(Findroot(y,x)==1)continue;
}
if(cc[x]!=0)
{
cut(x,cc[x]);
//access(x);access(cc[x]);splay(cc[x]);father[tree[cc[x]].left]=0;tree[cc[x]].left=0;splay(cc[x]);//father[x]=tree[cc[x]].left=0;
}
cc[x]=y;
if(cc[x]!=0)
{
//link(x,cc[x]);
access(x);father[x]=cc[x];//splay(x);
}*/
}
}
//printf("\n");
}
return ;
}
Hdu 2475-Box LCT,动态树的更多相关文章
- HDU 2475 BOX 动态树 Link-Cut Tree
Box Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) [Problem De ...
- hdu 2475 BOX (splay)
版权声明:本文为博主原创文章,未经博主允许不得转载. hdu 2475 Splay树是一种神奇的东西... 题意: 有一些箱子,要么放在地上,要么放在某个箱子里面 . 现在有两种操作: (1) MOV ...
- LCT 动态树 模板
洛谷:P3690 [模板]Link Cut Tree (动态树) /*诸多细节,不注意就会调死去! 见注释.*/ #include<cstdio> #include<iostream ...
- [HNOI2010]弹飞绵羊 (平衡树,LCT动态树)
题面 题解 因为每个点都只能向后跳到一个唯一的点,但可能不止一个点能跳到后面的某个相同的点, 所以我们把它抽象成一个森林.(思考:为什么是森林而不是树?) 子节点可以跳到父节点,根节点再跳就跳飞了. ...
- Fzu Problem 2082 过路费 LCT,动态树
题目:http://acm.fzu.edu.cn/problem.php?pid=2082 Problem 2082 过路费 Accept: 528 Submit: 1654Time Limit ...
- Hdu 3966-Aragorn's Story LCT,动态树
题目:http://acm.hdu.edu.cn/showproblem.php?pid=3966 Aragorn's Story Time Limit: 10000/3000 MS (Java/Ot ...
- HDU 5002 Tree(动态树LCT)(2014 ACM/ICPC Asia Regional Anshan Online)
Problem Description You are given a tree with N nodes which are numbered by integers 1..N. Each node ...
- Hdu 4010-Query on The Trees LCT,动态树
Query on The Trees Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65768/65768 K (Java/Othe ...
- HDU 2475 Box 树型转线型 + 伸展树
树型转线型.第一次听说这个概念. . . , 可是曾经已经接触过了,如LCA的预处理部分和树链剖分等.可是没想到还能这么用,三者虽说有不同可是大体思想还是非常相近的,学习了. 推荐博客http://b ...
随机推荐
- js跨浏览器事件对象、事件处理程序
项目中有时候会不用jquery这么好用的框架,需要自己封装一些事件对象和事件处理程序,像封装AJAX那样:这里面考虑最多的还是浏览器的兼容问题,原生js封装如下:var EventUtil={ //节 ...
- 深入了解shell
接触linux很久了,但一直没有总线,老是尝鲜,什么都想学,但好多没多没有记住,特的总结了一些基本的东西,查了很多资料,不完善的方面我会慢慢的更新…… 操作系统与外部最主要的接口就叫做shell. ...
- 浅析MySQL中exists与in的使用 (写的非常好)
转自http://sunxiaqw.blog.163.com/blog/static/990654382013430105130443/ exists对外表用loop逐条查询,每次查询都会查看exis ...
- js EasyUI前台 价格=数量*单价联动的实现
废话,不多说,,效果图如下:
- Java 中判断char 是否为空格 和空
//判断是否char是否为空import java.util.*; public class test{ public static void main(String[] args){ String ...
- tar 解压缩
解压 tar –xvf file.tar //解压 tar包 tar -xzvf file.tar.gz //解压tar.gz tar -xjvf file.tar.bz2 //解压 tar.bz ...
- oracle简单两个操作
sqlplus sys/密码 as sysdba ALTER USER 账号 IDENTIFIED BY 新密码; select * from (select rownum 别名 ,表名.* fro ...
- Linux 消息队列编程
消息队列.信号量以及共享内存被称作 XSI IPC,它们均来自system V的IPC功能,因此具有许多共性. 键和标识符: 内核中的每一种IPC结构(比如信号量.消息队列.共享内存)都用一个非负整数 ...
- thinkphp使用问题
下面总结一些,我在使用中遇到的问题,以后遇到了再补充 一.<a>标签的跳转问题 问题:我在控制器Home/Index/index里面使用了Public里面的index.html模板,ind ...
- Django 入门
Django 入门 Django是一个开放源代码的Web应用框架,由Python写成.采用了MVC的软件设计模型,即模型M,视图V和控制器C.它最初是被开发来用于管理劳伦斯出版集团旗下的一些以新闻内容 ...