JavaScript之深入理解【函数】
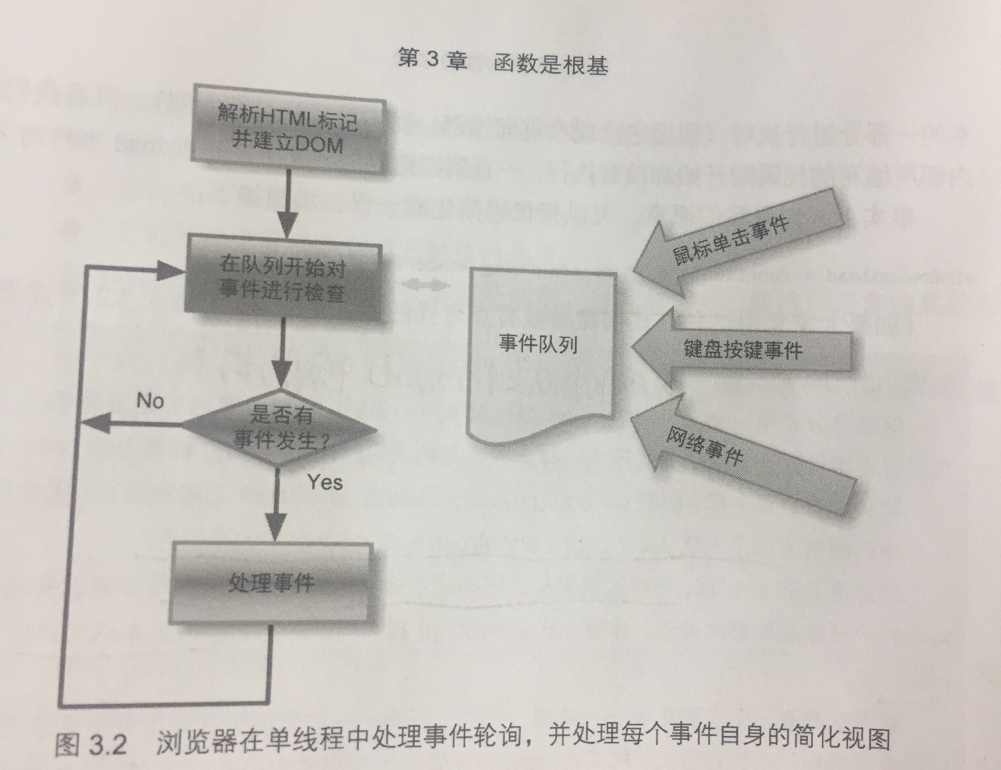
- function useless(callback){
- return callback();
- }
- var text = 'Johnny';
- console.log(useless(function(){ return text; }) == text);//true
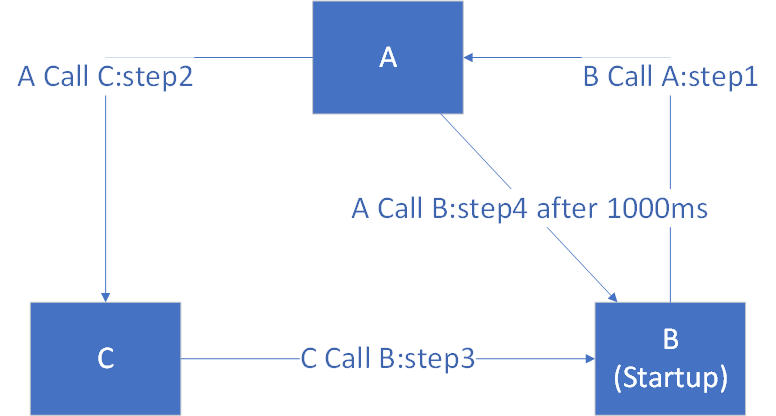
- var count = 1;
- var MAX_COUNT = 2;
- function A(messageA,fn1,arg1){
- console.log("A:",messageA);
- C("A call C");
- fn1(arg1);
- setTimeout(B("A call B once again after 1000ms"), 1000);//A再次回调B,二者形成了双向沟(调)通(用)
- }
- function B(messageB,fn2){ //启动入口:B(" startup from B");
- console.log("B:",messageB);
- if(count < MAX_COUNT){
- count++;
- A("B call A",function(msg){
- console.log(msg);
- },"B call A's others arg/function");
- }
- }
- function C(messageC,fn3){
- console.log("C:",messageC);
- B("C call B");
- }
test/output:
- B("Startup from B");
- B: Startup from B
- A: B call A
- C: A call C
- B: C call B
- B call A's others arg/function
- B: A call B once again after 1000ms
4.函数声明
格式:function [名称可选]([arg1,arg2,...,argN]) { ... }
[]表示:可选,非必须
5.作用域和函数
意义:当我们声明一个函数时,不仅要关注该函数可用的作用域,还要关注该函数自身所创建的作用域,以及函数内部的声明是如何影响这些作用域的。
特征:
1.在JavaScript中,作用域是由function进行声明的,而不是代码块【()、{}等】。
比如:if(...) { } //并不能像其他语言一样形成作用域
2.变量声明的作用域开始于:声明的地方;结束于所在函数的结尾,与代码嵌套无关。
3.机制提升:命令函数的作用域是指声明该函数的整个函数范围,与代码嵌套无关。
4.对于作用域声明,全局上下文就像一个包含页面所有代码的超大型函数。
- function outer(){
- assert(typeof outer == 'function',"1 outer() is in scope"); //true
- assert(typeof inner == 'function',"2 inner() is in scope"); //true
- assert(typeof a == 'number',"3 a is in scope");
- assert(typeof b == 'number',"4 b is in scope");
- assert(typeof c == 'number',"5 c is in scope");
- var a = 1;
- function inner(){
- assert(typeof inner == 'function',"6 inner() is in scope"); //true
- assert(typeof a == 'number',"7 a is in scope"); //true
- assert(typeof b == 'number',"8 b is in scope"); //true
- assert(typeof c == 'number',"9 c is in scope"); //true
- }
- var b = 2;
- if(a == 1){
- assert(typeof a == 'number',"10 a is in scope"); //true
- assert(typeof b == 'number',"11 b is in scope"); //true
- assert(typeof c == 'number',"12 c is in scope");
- var c= 3;
- }
- assert(typeof a == 'number',"13 a is in scope"); //true
- assert(typeof b == 'number',"14 b is in scope"); //true
- assert(typeof c == 'number',"15 c is in scope"); //true
- inner();
- }
- outer();
- assert(typeof outer == 'function',"16 outer() is in scope"); //true
- assert(typeof inner == 'function',"17 inner() is in scope");
- assert(typeof a == 'number',"18 a is in scope");
- assert(typeof b == 'number',"19 b is in scope");
- assert(typeof c == 'number',"20 c is in scope");
output:
- 1 outer() is in scope
- 2 inner() is in scope
- 3 a is in scope
- 4 b is in scope
- 5 c is in scope
- 10 a is in scope
- 11 b is in scope
- 12 c is in scope
- 13 a is in scope
- 14 b is in scope
- 15 c is in scope
- 6 inner() is in scope
- 7 a is in scope
- 8 b is in scope
- 9 c is in scope
- 16 outer() is in scope
- 17 inner() is in scope
- 18 a is in scope
- 19 b is in scope
- 20 c is in scope
s
JavaScript之深入理解【函数】的更多相关文章
- 理解和使用 JavaScript 中的回调函数
理解和使用 JavaScript 中的回调函数 标签: 回调函数指针js 2014-11-25 01:20 11506人阅读 评论(4) 收藏 举报 分类: JavaScript(4) 目录( ...
- [转]理解与使用Javascript中的回调函数
在Javascript中,函数是第一类对象,这意味着函数可以像对象一样按照第一类管理被使用.既然函数实际上是对象:它们能被“存储”在变量中,能作为函数参数被传递,能在函数中被创建,能从函数中返回. 因 ...
- 【JavaScript】理解与使用Javascript中的回调函数
在Javascript中,函数是第一类对象,这意味着函数可以像对象一样按照第一类管理被使用.既然函数实际上是对象:它们能被“存储”在变量中,能作为函数参数被传递,能在函数中被创建,能从函数中返回. 因 ...
- JavaScript callback function 回调函数的理解
来源于:http://mao.li/javascript/javascript-callback-function/ 看到segmentfault上的这个问题 JavaScript 回调函数怎么理解, ...
- 理解javascript中的回调函数(callback)【转】
在JavaScrip中,function是内置的类对象,也就是说它是一种类型的对象,可以和其它String.Array.Number.Object类的对象一样用于内置对象的管理.因为function实 ...
- 深入理解JavaScript执行上下文、函数堆栈、提升的概念
本文内容主要转载自以下两位作者的文章,如有侵权请联系我删除: https://feclub.cn/post/content/ec_ecs_hosting http://blog.csdn.net/hi ...
- 理解与使用Javascript中的回调函数 -2
在javascript中回调函数非常重要,它们几乎无处不在.像其他更加传统的编程语言都有回调函数概念,但是非常奇怪的是,完完整整谈论回调函数的在线教程比较少,倒是有一堆关于call()和apply() ...
- 理解与使用Javascript中的回调函数
在Javascript中,函数是第一类对象,这意味着函数可以像对象一样按照第一类管理被使用.既然函数实际上是对象:它们能被“存储”在变量中,能作为函数参数被传递,能在函数中被创建,能从函数中返回. 因 ...
- JavaScript大杂烩2 - 理解JavaScript的函数
JavaScript中的字面量 书接上回,我们已经知道在JavaScript中存在轻量级的string,number,boolean与重量级的String,Number,Boolean,而且也知道了之 ...
- 《JavaScript高级程序设计》读书笔记(三)基本概念第六小节理解函数
内容---语法---数据类型---流程控制语句 上一小节---理解函数 本小节 函数--使用function关键字声明,后跟一组参数以及函数体 function functionName(arg0, ...
随机推荐
- java拦截器(interceptor)
1.声明式 (1)注解,使用Aspect的@Aspect (2)实现HandlerInterceptor /** * 拦截请求 * * @author Administrator * */ @Comp ...
- CQOI2019(十二省联考)游记
CQOI2019(十二省联考)游记 Day -? 自从联赛爆炸,\(THUWC\)爆炸,\(WC\)爆炸(就没有不爆炸的)之后我已经无所畏惧... 听说是考\(4.5 h\)吗? Day -1 \(Z ...
- windows 和linux 路径解析的区别
windows下使用的是“\”作为分隔符,而linux则反其道而行之使用"/"作为分隔符.所以在windows 环境中获取路径常见 C:\windows\system 的形式,而l ...
- leetcode 54. Spiral Matrix 、59. Spiral Matrix II
54题是把二维数组安卓螺旋的顺序进行打印,59题是把1到n平方的数字按照螺旋的顺序进行放置 54. Spiral Matrix start表示的是每次一圈的开始,每次开始其实就是从(0,0).(1,1 ...
- 在Winform开发框架中对附件文件进行集中归档处理
在我们Winform开发中,往往需要涉及到附件的统一管理,因此我倾向于把它们独立出来作为一个附件管理模块,这样各个模块都可以使用这个附件管理模块,更好的实现模块重用的目的.在涉及附件管理的场景中,一个 ...
- nginx简单的命令
nginx -s reload|reopen|stop|quit #重新加载配置|重启|停止|退出 nginx nginx -t #测试配置是否有语法错误 nginx [-?hvVtq] [-s si ...
- vue 修改数据界面没有及时更新nextTick
使用场景:有些时候,我们使用vue修改了一些数据,但是页面上的DOM还没有更新,这个时候我们就需要使用到nextTick. vm.$nextTick( [callback] ) 说明: 将回调延迟到下 ...
- Promise async-await 异步解决方案
1.简介: async和await在干什么,async用于申明一个function是异步的,而await可以认为是async wait的简写,等待一个异步方法执行完成. 2.基本语法 在C ...
- ERP常见问题总结处理
1. ERP系统的重量录入组件设计不合理易导致录入错误 如下图所示: 修正方法: 1. 更正数据 使用SQL语句更正数据,已经生产完成,作为单据重新录入比较麻烦 UPDATE e_wms_materi ...
- 4月22日MySQL学习
前面学习的知识基本都是概念知识没有什么代码,然后还有图形界面来辅助学习. 今天学习了MySQL的存储引擎,最常用的两种 MYISAM:不支持事务,也不支持外键,但是访问速度快. INNODB:支持事务 ...