利刃 MVVMLight 2:Model、View、ViewModel结构以及全局视图模型注入器的说明


using GalaSoft.MvvmLight;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks; namespace MVVMLightDemo.Model
{
public class WelcomeModel : ObservableObject
{
private String introduction;
/// <summary>
/// 欢迎词
/// </summary>
public String Introduction
{
get { return introduction; }
set { introduction = value; RaisePropertyChanged(()=>Introduction); }
}
}
}
using GalaSoft.MvvmLight;
using MVVMLightDemo.Model;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks; namespace MVVMLightDemo.ViewModel
{
public class WelcomeViewModel:ViewModelBase
{
/// <summary>
/// 构造函数
/// </summary>
public WelcomeViewModel()
{
Welcome = new WelcomeModel() { Introduction = "Hello World!" };
}
#region 属性 private WelcomeModel welcome;
/// <summary>
/// 欢迎词属性
/// </summary>
public WelcomeModel Welcome
{
get { return welcome; }
set { welcome = value; RaisePropertyChanged(()=>Welcome); }
}
#endregion
}
}
<Window x:Class="MVVMLightDemo.View.WelcomeView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WelcomeView" Height="" Width="">
<Grid>
<StackPanel VerticalAlignment="Center" HorizontalAlignment="Center" >
<TextBlock Text="{Binding Welcome.Introduction}" FontSize="" ></TextBlock>
</StackPanel>
</Grid>
</Window>
TextBlock 绑定了 Welcome.Introduction,所以应该显示Welcome对象下的Introduction属性。
这时候的ViewModel和View是没有任何关系的,所以我们在code-Behind的构造函数中写上如下代码:
using MVVMLightDemo.ViewModel;
using System.Windows; namespace MVVMLightDemo.View
{
/// <summary>
/// Interaction logic for WelcomeView.xaml
/// </summary>
public partial class WelcomeView : Window
{
public WelcomeView()
{
InitializeComponent();
this.DataContext = new WelcomeViewModel();
}
}
}
把 WelcomeViewModel 赋值给当前视图的数据上下文。所以可以在当前视图中使用ViewModel中所有的公开属性和命令。
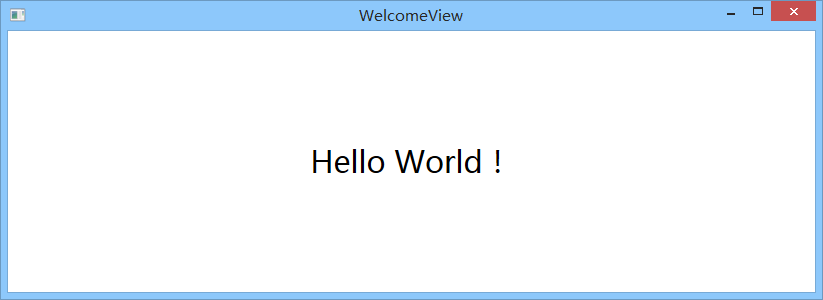
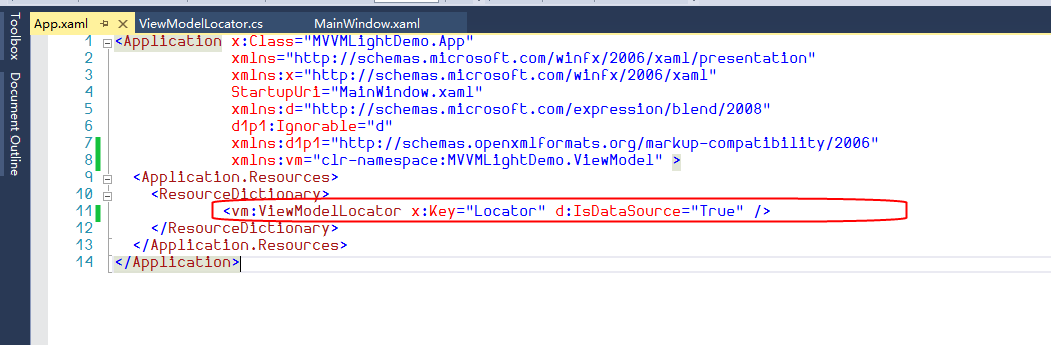
<Application x:Class="MVVMLightDemo.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
StartupUri="View/WelcomeView.xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
d1p1:Ignorable="d"
xmlns:d1p1="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:vm="clr-namespace:MVVMLightDemo.ViewModel" >
<Application.Resources>
<ResourceDictionary>
<vm:ViewModelLocator x:Key="Locator" d:IsDataSource="True" />
</ResourceDictionary>
</Application.Resources>
</Application>
所以每次App初始化的时候,就会去初始化ViewModelLocator类。
/*
In App.xaml:
<Application.Resources>
<vm:ViewModelLocator xmlns:vm="clr-namespace:MVVMLightDemo"
x:Key="Locator" />
</Application.Resources> In the View:
DataContext="{Binding Source={StaticResource Locator}, Path=ViewModelName}" You can also use Blend to do all this with the tool's support.
See http://www.galasoft.ch/mvvm
*/ using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Ioc;
using Microsoft.Practices.ServiceLocation; namespace MVVMLightDemo.ViewModel
{
/// <summary>
/// This class contains static references to all the view models in the
/// application and provides an entry point for the bindings.
/// </summary>
public class ViewModelLocator
{
/// <summary>
/// Initializes a new instance of the ViewModelLocator class.
/// </summary>
public ViewModelLocator()
{
ServiceLocator.SetLocatorProvider(() => SimpleIoc.Default); #region Code Example
////if (ViewModelBase.IsInDesignModeStatic)
////{
//// // Create design time view services and models
//// SimpleIoc.Default.Register<IDataService, DesignDataService>();
////}
////else
////{
//// // Create run time view services and models
//// SimpleIoc.Default.Register<IDataService, DataService>();
////}
#endregion SimpleIoc.Default.Register<MainViewModel>();
} #region 实例化
public MainViewModel Main
{
get
{
return ServiceLocator.Current.GetInstance<MainViewModel>();
}
} #endregion public static void Cleanup()
{
// TODO Clear the ViewModels
}
}
}
/*
In App.xaml:
<Application.Resources>
<vm:ViewModelLocator xmlns:vm="clr-namespace:MVVMLightDemo"
x:Key="Locator" />
</Application.Resources> In the View:
DataContext="{Binding Source={StaticResource Locator}, Path=ViewModelName}" You can also use Blend to do all this with the tool's support.
See http://www.galasoft.ch/mvvm
*/ using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Ioc;
using Microsoft.Practices.ServiceLocation; namespace MVVMLightDemo.ViewModel
{
/// <summary>
/// This class contains static references to all the view models in the
/// application and provides an entry point for the bindings.
/// </summary>
public class ViewModelLocator
{
/// <summary>
/// Initializes a new instance of the ViewModelLocator class.
/// </summary>
public ViewModelLocator()
{
ServiceLocator.SetLocatorProvider(() => SimpleIoc.Default); #region Code Example
////if (ViewModelBase.IsInDesignModeStatic)
////{
//// // Create design time view services and models
//// SimpleIoc.Default.Register<IDataService, DesignDataService>();
////}
////else
////{
//// // Create run time view services and models
//// SimpleIoc.Default.Register<IDataService, DataService>();
////}
#endregion SimpleIoc.Default.Register<MainViewModel>();
SimpleIoc.Default.Register<WelcomeViewModel>();
} #region 实例化
public MainViewModel Main
{
get
{
return ServiceLocator.Current.GetInstance<MainViewModel>();
}
} public WelcomeViewModel Welcome
{
get
{
return ServiceLocator.Current.GetInstance<WelcomeViewModel>();
}
} #endregion public static void Cleanup()
{
// TODO Clear the ViewModels
}
}
}
public WelcomeView()
{
InitializeComponent();
this.DataContext = new WelcomeViewModel();
}
中的 this.DataContext = new WelcomeViewModel(); 可以去掉了,直接在WelcomeView中这样写:


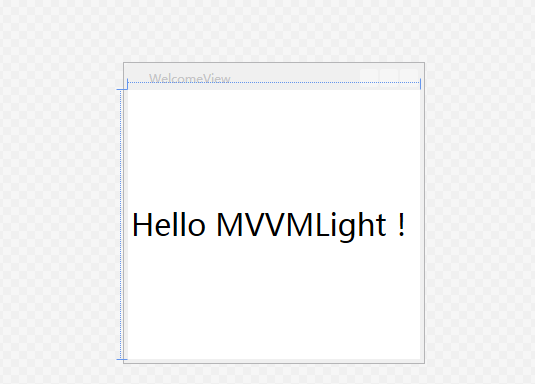
利刃 MVVMLight 2:Model、View、ViewModel结构以及全局视图模型注入器的说明的更多相关文章
- PyQt学习随笔:Model/View架构中多个视图之间选择数据项同步
我们知道多个视图之间通过使用相同的model就可以实现数据的共享(具体请参考< PyQt学习随笔:ListView控件的视图和数据模型分离案例>),除了数据的共享之外,多个视图之间还可以同 ...
- Model Binding is not working. MVC3 视图模型绑定不成功
问题出现在POST方法中,当我要将数据提交到后台的时候,绑定的变量值为null 原因是视图中的名称跟Controller中的视图的名称不一致造成的. 假如你视图中的Model采用的是Html.Labe ...
- 利刃 MVVMLight
已经很久没有写系列文章了,上一次是2012年写的HTLM5系列,想想我们应该是较早一批使用HTML5做项目的人. 相比我当时动不动100+的粉丝增长和两天3000+的阅读量,MVVM Light只能算 ...
- Qt Model/View(模型/视图)结构(无师自通)
Model/View(模型/视图)结构是 Qt 中用界面组件显示与编辑数据的一种结构,视图(View)是显示和编辑数据的界面组件,模型(Model)是视图与原始数据之间的接口. GUI 应用程序的一个 ...
- Qt Model/View(官方翻译,图文并茂)
http://doc.trolltech.com/main-snapshot/model-view-programming.html 介绍 Qt 4推出了一组新的item view类,它们使用mode ...
- (转)Qt Model/View 学习笔记 (一)——Qt Model/View模式简介
Qt Model/View模式简介 Qt 4推出了一组新的item view类,它们使用model/view结构来管理数据与表示层的关系.这种结构带来的 功能上的分离给了开发人员更大的弹性来定制数据项 ...
- qt model/view 架构基础介绍之QListWidget
# -*- coding: utf-8 -*- # python:2.x __author__ = 'Administrator' from PyQt4.QtGui import * from Py ...
- Qt 学习之路 2(41):model/view 架构
Qt 学习之路 2(41):model/view 架构 豆子 2013年1月23日 Qt 学习之路 2 50条评论 有时,我们的系统需要显示大量数据,比如从数据库中读取数据,以自己的方式显示在自己的应 ...
- 第十五章、Model/View架构中Item Views部件的父类
老猿Python博文目录 老猿Python博客地址 引言:本章早就写好了,其简版<第15.18节 PyQt(Python+Qt)入门学习:Model/View架构中视图Item Views父类详 ...
随机推荐
- UI控件库
UI控件库分享:DWZ(j-UI).LigerUI.Linb DWZ(j-UI): 在线演示地址:http://demo.dwzjs.com 在线文档:http://demo.dwzjs.com/do ...
- Mybatis之动态构建SQL语句
今天一个新同事问我,我知道如何利用XML的方式来构建动态SQL,可是Mybatis是否能够利用注解完成动态SQL的构建呢?!!答案是肯定的,MyBatis 提供了注解,@InsertProvider, ...
- openwrt_git_pull命令提示merger冲突时如何解决?
直接贴代码 tf@ubuntu:~/projects/openwrt1407$ git pull Updating 331ecb0..d12dc6e error: Your local changes ...
- Best JavaScript Tools for Developers
JavaScript solves multiple purposes; it helps you to create interactive websites, web applications, ...
- 【剑指offer】的功率值
标题叙述性说明: 实现函数double Power(double base, int exponent),求base的exponent次方.不得使用库函数.同一时候不须要考虑大数问题. 分析描写叙述: ...
- 代码阅读软件kscope源码安装指导
安装 kscope-1.6.2 1. ./configure --without-arts --prefix=/soft/kscope-1.6.2 (I customize the installi ...
- 如何解决 Django中出现的 [Errno 13] Permission denied问题
环境:linux 如果你使用了Apache部署了Django项目,在上传文件时可能会出现 “[Errno 13] Permission denied:某目录”的错误. 这是因为apache没有权限在该 ...
- AngularJS的工作原理
AngularJS的工作原理 个人觉得,要很好的理解AngularJS的运行机制,才能尽可能避免掉到坑里面去.在这篇文章中,我将根据网上的资料和自己的理解对AngularJS的在启动后,每一步都做了些 ...
- Day4:T3搜索 T4数学题排列组合
T3:搜索 很出名的题吧,费解的开关 同T2一样也是一题很考思考的 附上题解再解释吧: 对于每个状态,算法只需要枚举第一行改变哪些灯的状态,只要第一行的状态固定了,接下来的状态改变方法都是唯一的:每一 ...
- poj3083走玉米地问题
走玉米地迷宫,一般有两种简单策略,遇到岔路总是优先沿着自己的左手方向,或者右手方向走.给一个迷宫,给出这两种策略的步数,再给出最短路径的长度. ######### #.#.#.#.# S....... ...