Power Network(网络流最大流 & dinic算法 + 优化)
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 24019 | Accepted: 12540 |
Description
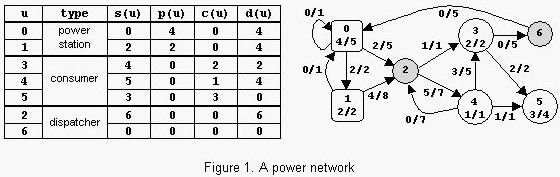
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20
7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7
(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5
(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15
6
Hint
Source
#include<iostream>
#include<stdio.h>
#include<string.h>
using namespace std;
#define MAXN 202
const int INT_MAX = 0x3f3f3f3f ;
int s, t;
int n, np, nc, m;
char str[];
int c[MAXN][MAXN];
int f[MAXN][MAXN];
int e[MAXN];
int h[MAXN];
void push(int u, int v)
{
int d = min(e[u], c[u][v] - f[u][v]);
f[u][v] += d;
f[v][u] = -f[u][v];
e[u] -= d;
e[v] += d;
}
bool relabel(int u)
{
int mh = INT_MAX;
for(int i=; i<n+; i++)
{
if(c[u][i] > f[u][i])
mh = min(mh, h[i]);
}
if(mh == INT_MAX)
return false; //残留网络中无从u出发的路
h[u] = mh + ;
for(int i=; i<n+; i++)
{
if(e[u] == ) //已无余流,不需再次push
break;
if(h[i] == mh && c[u][i] > f[u][i]) //push的条件
push(u, i);
}
return true;
}
void init_preflow()
{
memset(h, , sizeof(h));
memset(e, , sizeof(e));
h[s] = n+;
for(int i=; i<n+; i++)
{
if(c[s][i] == )
continue;
f[s][i] = c[s][i];
f[i][s] = -f[s][i];
e[i] = c[s][i];
e[s] -= c[s][i];
}
}
void push_relabel()
{
init_preflow();
bool flag = true; //表示是否还有relabel操作
while(flag)
{
flag = false;
for(int i=; i<n; i++)
if(e[i] > )
flag = flag || relabel(i);
}
}
int main()
{
while(scanf("%d%d%d%d", &n, &np, &nc, &m) != EOF)
{
s = n; t = n+;
memset(c, , sizeof(c));
memset(f, , sizeof(f));
while(m--)
{
scanf("%s", &str);
int u=, v=, z=;
sscanf(str, "(%d,%d)%d", &u, &v, &z);
c[u][v] = z;
}
for(int i=; i<np+nc; i++)
{
scanf("%s", &str);
int u=, z=;
sscanf(str, "(%d)%d", &u, &z);
if(i < np)
c[s][u] = z;
else if(i >= np && i < np + nc)
c[u][t] = z;
}
push_relabel();
printf("%d\n", e[t]);
}
}
dinic递归 110ms / 非递归 110 或 94 ms:http://hefeijack.iteye.com/blog/1885944
dinic 63ms/SAP 63 ms:http://www.cnblogs.com/kuangbin/archive/2012/09/11/2680908.html
dinic 63ms :http://blog.csdn.net/u011721440/article/details/38611197
初学者可以先去poj1273试试水=。=
这道题只要加一个 源点 和 汇点 , 就万事大吉了。
自己写了一遍,用dinic的递归形式,一开始tle,但就加了一句话,813msAC,
貌似是一种优化,但为啥不清楚。
#include<stdio.h>
#include<string.h>
#include<queue>
#include<algorithm>
using namespace std;
const int M = , inf = 0x3f3f3f3f ;
int m , n ;
int map[M][M] ;
int dis[M]; bool bfs ()
{
queue <int> q ;
while (!q.empty ())
q.pop () ;
memset (dis , 0xff , sizeof(dis)) ;
dis[] = ;
q.push () ;
while (!q.empty () ) {
int u = q.front () ;
q.pop () ;
for (int v = ; v <= n ; v++) {
if (map[u][v] && dis[v] == -) {
dis[v] = dis[u] + ;
q.push (v) ;
}
}
}
if (dis[n] > )
return true ;
return false ;
} int find (int u , int low)
{
int a = ;
if (u == n)
return low ;
for (int v = ; v <= n ; v++) {
if (map[u][v] && dis[v] == dis[u] + && (a = find (v , min(map[u][v] , low)))) {
map[u][v] -= a ;
map[v][u] += a ;
return a ;
}
}
dis[u] = - ;//就这句
return ;
} int main ()
{
//freopen ("b.txt" , "r" , stdin) ;
int u , v , w ;
int ans ;
while (~ scanf ("%d%d" , &m , &n)) {
memset (map , , sizeof(map)) ;
while (m--) {
scanf ("%d%d%d" , &u , &v , &w) ;
map[u][v] += w ;
}
int maxn = ;
while (bfs()) {
if (ans = find( , inf))
maxn += ans ;
}
printf ("%d\n" , maxn) ;
}
return ;
}
用邻接表可以进一步优化(但上面那句话仍要加):因为用矩阵的话在find 和 bfs 时找点都要遍历一遍所有的点,但用邻接表的话可以很快找到。
110msAC:
#include<stdio.h>
#include<string.h>
#include<queue>
#include<algorithm>
using namespace std;
const int M = , inf = 0x3f3f3f3f ;
struct edge
{
int u , v , time_u ;
int w ;
}e[M * M * ];
int n , np , nc , m ;
int src , des ;
int cnt = ;
int dis[M] ;
char st[] ;
int head[M * M * ] ; void addedge (int u , int v , int w)
{
e[cnt].u = u ; e[cnt].v = v ; e[cnt].w = w ; e[cnt].time_u = head[u] ;
head[u] = cnt++ ;
e[cnt].v = u ; e[cnt].u = v ; e[cnt].w = ; e[cnt].time_u = head[v] ;
head[v] = cnt++ ;
}
bool bfs ()
{
queue <int> q ;
while (!q.empty ())
q.pop () ;
memset (dis , - , sizeof(dis)) ;
dis[src] = ;
q.push (src) ;
while (!q.empty ()) {
int u = q.front () ;
q.pop () ;
for (int i = head[u] ; i != - ; i = e[i].time_u) {
int v = e[i].v ;
if (dis[v] == - && e[i].w > ) {
dis[v] = dis[u] + ;
q.push (v) ;
}
}
}
if (dis[des] > )
return true ;
return false ;
} int find (int u , int low)
{
int a = ;
if (u == des)
return low ;
for (int i = head[u] ; i != - ; i = e[i].time_u) {
int v = e[i].v ;
if (e[i].w > && dis[v] == dis[u] + && (a = find(v , min (low , e[i].w)))) {
e[i].w -= a ;
e[i^].w += a ;
return a ;
}
}
dis[u] = - ;
return false ;
} int main ()
{
// freopen ("a.txt" , "r" , stdin) ;
int u , v , w ;
while (~ scanf ("%d%d%d%d" , &n , &np , &nc , &m)) {
src = n ;
des = n + ;
cnt = ;
memset (head , - , sizeof(head)) ;
while (m--) {
scanf ("%s" , st) ;
sscanf (st , "(%d,%d)%d" , &u , &v , &w) ;
addedge(u , v , w) ;
}
while (np--) {
scanf ("%s" , st) ;
sscanf (st , "(%d)%d" , &v , &w) ;
addedge (src , v , w) ;
}
while (nc--) {
scanf ("%s" , st) ;
sscanf (st , "(%d)%d" , &u , &w) ;
addedge (u , des , w) ;
} int ans = , res = ;
while (bfs()) {
while () {
if (ans = find(src , inf))
res += ans ;
else
break ;
}
}
printf ("%d\n" , res) ;
}
return ;
}
head[u]用来存放点u最新出现的时间 ,感觉和tarjan算法中的dfn[u]差不多;
time_u则保存上一次点u出现的时间 。
Power Network(网络流最大流 & dinic算法 + 优化)的更多相关文章
- POJ训练计划1459_Power Network(网络流最大流/Dinic)
解题报告 这题建模实在是好建.,,好贱.., 给前向星给跪了,纯dinic的前向星居然TLE,sad.,,回头看看优化,.. 矩阵跑过了.2A,sad,,, /******************** ...
- 网络流最大流——dinic算法
前言 网络流问题是一个很深奥的问题,对应也有许多很优秀的算法.但是本文只会讲述dinic算法 最近写了好多网络流的题目,想想看还是写一篇来总结一下网络流和dinic算法以免以后自己忘了... 网络流问 ...
- [讲解]网络流最大流dinic算法
网络流最大流算法dinic ps:本文章不适合萌新,我写这个主要是为了复习一些细节,概念介绍比较模糊,建议多刷题去理解 例题:codevs草地排水,方格取数 [抒情一下] 虽然老师说这个多半不考,但是 ...
- POJ 1459 Power Network(网络流 最大流 多起点,多汇点)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 22987 Accepted: 12039 D ...
- POJ1459 Power Network 网络流 最大流
原文链接http://www.cnblogs.com/zhouzhendong/p/8326021.html 题目传送门 - POJ1459 题意概括 多组数据. 对于每一组数据,首先一个数n,表示有 ...
- 网络流——最大流Dinic算法
前言 突然发现到了新的一年什么东西好像就都不会了凉凉 算法步骤 建残量网络图 在残量网络图上跑增广路 重复1直到没有增广路(注意一个残量网络图要尽量把价值都用完,不然会浪费建图的时间) 代码实现 #i ...
- 网络流之最大流Dinic算法模版
/* 网络流之最大流Dinic算法模版 */ #include <cstring> #include <cstdio> #include <queue> using ...
- hdu-3572 Task Schedule---最大流判断满流+dinic算法
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=3572 题目大意: 给N个任务,M台机器.每个任务有最早才能开始做的时间S,deadline E,和持 ...
- 网络流(最大流-Dinic算法)
摘自https://www.cnblogs.com/SYCstudio/p/7260613.html 网络流定义 在图论中,网络流(Network flow)是指在一个每条边都有容量(Capacity ...
随机推荐
- Android--保持加速度传感器在屏幕关闭后运行
由于写论文需要,需要用手机加速度采集数据,关于android加速度传感器的介绍网上一抓一大把,但大多都是大同小异,跟官网文档差不多.自己写了个取加速度传感器的APK,发现数据有点不对劲,原理屏幕一关后 ...
- Asp.Net的两种开发方式
来源:http://www.zhidao91.com/asp-net/ 在经过对.Net平台深入的学习以后,我发现很多语言开发动态网站时,它的后台逻辑都差不多是相同的,今天在这里我给大家来聊聊在.Ne ...
- [30分钟]MSSQL快速入门教程
1.什么是SQL语句 sql语言:结构化的查询语言.(Structured Query Language),是关系数据库管理系统的标准语言. 它是一种解释语言:写一句执行一句,不需要整体编译执行.语法 ...
- Android 中R文件丢失问题解决方案
Project → clean 项目上右键→android Tools→ fix project 检查xml文件中有无命名错误,特别是@+id写成@id的[特别是这条,注意看控制台打印的xml错误]
- 自己保留:data provider
<system.data> <DbProviderFactories > <add name="MySQL Data Provider" ...
- 深入理解jQuery中$.get、$.post、$.getJSON和$.ajax的用法
当我们用javascript写ajax程序写得很“开心”的时候,突然有人告诉你有一种东西叫jquery,它会告诉你不直接和HttpRequest是多么的快乐,同时你再也不需要再烦恼纠结的ajax乱码问 ...
- tableviewCell的xib中collectionView签协议
- 团队项目作业第二项:利用NABCD模型进行竞争性需求分析
项目需求分析与建议--NABCD模型(王鲁跃负责) N (Need 需求) 对于现在的学生来说,我们认为打字是很重要的.不管在什么方面都需要进行电脑打字,例如文员.QQ.MSN.制作,论文等等,都需要 ...
- 小菜鸟学 Spring-Dependency injection(二)
注入方式一:set注入 <bean id="exampleBean" class="examples.ExampleBean"> <!-- s ...
- hdu4333 扩展KMP
慢慢研究可以发现,可以用扩展kmp来求.由于扩展kmp的next[]只有一部分,当前位子前面那部分和母串的后部分,所以可以将字符串复制接在后面一次. 先求如果next[]>0&& ...