1246 - Colorful Board
Time Limit: 2 second(s) | Memory Limit: 32 MB |
You are given a rectangular board. You are asked to draw M horizontal lines and N vertical lines in that board, so that the whole board will be divided into (M+1) x (N+1) cells. So, there will be M+1 rows each of which will exactly contain N+1 cells or columns. The yth cell of xth row can be called as cell(x, y). The distance between two cells is the summation of row difference and column difference of those two cells. So, the distance between cell(x1, y1) and cell(x2, y2) is
|x1 - x2| + |y1 - y2|
For example, the distance between cell (2, 3) and cell (3, 2) is |2 - 3| + |3 - 2| = 1 + 1 = 2.
After that you have to color every cell of the board. For that you are given K different colors. To make the board more beautiful you have to make sure that no two cells having the same color can have odd distance between them. For example, if you color cell (3, 5) with red, you cannot color cell (5, 8) with red, as the distance between them is 5, which is odd. Note that you can keep some color unused, but you can't keep some cell uncolored.
You have to determine how many ways to color the board using those K colors.
Input
Input starts with an integer T (≤ 20000), denoting the number of test cases.
Each case starts with a line containing three integers M, N, K (0 ≤ M, N ≤ 19, 1 ≤ K ≤ 50).
Output
For each case, print the case number and the number of ways you can color the board. The result can be large, so print the result modulo 1000000007.
Sample Input |
Output for Sample Input |
4 0 0 1 0 0 2 5 5 2 5 5 1 |
Case 1: 1 Case 2: 2 Case 3: 2 Case 4: 0 |
Time Limit: 2 second(s) | Memory Limit: 32 MB |
You are given a rectangular board. You are asked to draw M horizontal lines and N vertical lines in that board, so that the whole board will be divided into (M+1) x (N+1) cells. So, there will be M+1 rows each of which will exactly contain N+1 cells or columns. The yth cell of xth row can be called as cell(x, y). The distance between two cells is the summation of row difference and column difference of those two cells. So, the distance between cell(x1, y1) and cell(x2, y2) is
|x1 - x2| + |y1 - y2|
For example, the distance between cell (2, 3) and cell (3, 2) is |2 - 3| + |3 - 2| = 1 + 1 = 2.
After that you have to color every cell of the board. For that you are given K different colors. To make the board more beautiful you have to make sure that no two cells having the same color can have odd distance between them. For example, if you color cell (3, 5) with red, you cannot color cell (5, 8) with red, as the distance between them is 5, which is odd. Note that you can keep some color unused, but you can't keep some cell uncolored.
You have to determine how many ways to color the board using those K colors.
Input
Input starts with an integer T (≤ 20000), denoting the number of test cases.
Each case starts with a line containing three integers M, N, K (0 ≤ M, N ≤ 19, 1 ≤ K ≤ 50).
Output
For each case, print the case number and the number of ways you can color the board. The result can be large, so print the result modulo 1000000007.
Sample Input |
Output for Sample Input |
4 0 0 1 0 0 2 5 5 2 5 5 1 |
Case 1: 1 Case 2: 2 Case 3: 2 Case 4: 0 |
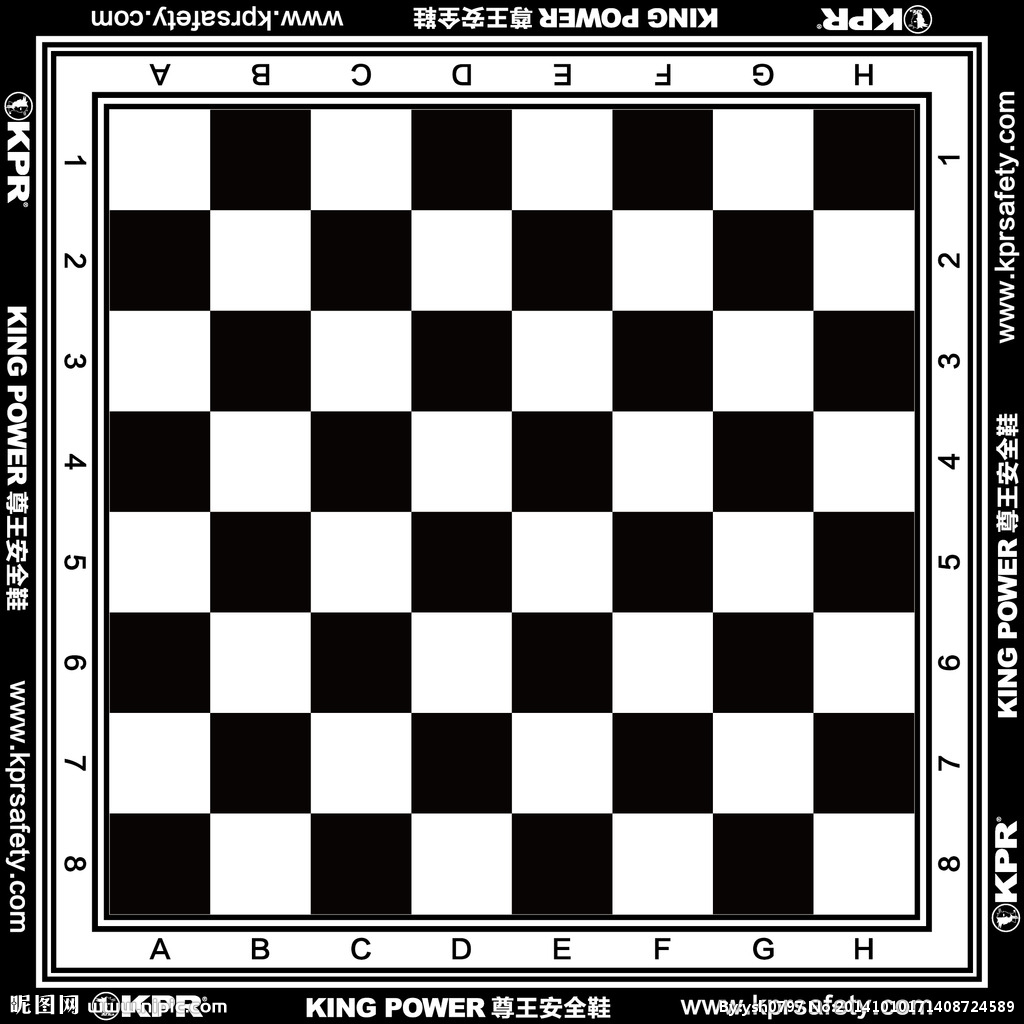
1 #include<stdio.h>
2 #include<algorithm>
3 #include<string.h>
4 #include<iostream>
5 using namespace std;
6 typedef long long LL;
7 const LL N= 1000000007;
8 LL yan[1005][1005];
9 LL STL[1005][1005];
10 LL pp[1005];
11 LL quick(LL n,LL m);
12 int main(void)
13 {
14 int i,j,k;
15 scanf("%d",&k);
16 int s;
17 yan[0][0]=1;
18 for(i=1; i<=1000; i++)
19 {
20 for(j=0; j<=i; j++)
21 {
22 if(j==0||i==j)
23 yan[i][j]=1;
24 else
25 {
26 yan[i][j]=(yan[i-1][j]+yan[i-1][j-1])%N;
27 }
28 }
29 }
30 pp[0]=1;
31 for(i=1;i<=1000;i++)
32 pp[i]=(pp[i-1]*i)%N;
33 memset(STL,0,sizeof(STL));
34 STL[0][0]=1;
35 STL[1][0]=0;
36 STL[1][1]=1;
37 for(i=2; i<=1000; i++)
38 {
39 for(j=1; j<=i; j++)
40 {
41 if(j==1||i==j)
42 STL[i][j]=1;
43 else
44 {
45 STL[i][j]=((STL[i-1][j]*j)%N+STL[i-1][j-1])%N;
46 }
47 }
48 }
49 for(s=1; s<=k; s++)
50 {
51 int x1,x2,x3,x4;
52 scanf("%d %d %d",&x1,&x2,&x3);
53 x1+=1;
54 x2+=1;
55 LL sum=(x1*x2);
56 LL he=(sum+1)/2;
57 LL cnt=0;
58 for(i=1; i<=min((LL)x3,he); i++)
59 {
60 LL x=x3-i;
61 LL kk=quick(x,sum-he);
62 LL ak=((STL[he][i]*yan[x3][i]%N)*kk)%N;
63 cnt=(cnt+ak*pp[i]%N)%N;
64 }
65 printf("Case %d: ",s);
66 printf("%lld\n",cnt);
67 }
68 return 0;
69 }
70
71 LL quick(LL n,LL m)
72 {
73 LL ans=1;n%=N;
74 while(m)
75 {
76 if(m&1)
77 ans=(ans*n)%N;
78 n=(n*n)%N;
79 m/=2;
80 }
81 return ans;
82 }
1246 - Colorful Board的更多相关文章
- LightOJ - 1246 Colorful Board(DP+组合数)
http://lightoj.com/volume_showproblem.php?problem=1246 题意 有个(M+1)*(N+1)的棋盘,用k种颜色给它涂色,要求曼哈顿距离为奇数的格子之间 ...
- LightOJ - 1246 - Colorful Board(DP)
链接: https://vjudge.net/problem/LightOJ-1246 题意: You are given a rectangular board. You are asked to ...
- AC日记——丑数 codevs 1246
1246 丑数 USACO 时间限制: 1 s 空间限制: 128000 KB 题目等级 : 钻石 Diamond 题解 查看运行结果 题目描述 Description 对于一给定的素 ...
- [LeetCode] Battleships in a Board 平板上的战船
Given an 2D board, count how many different battleships are in it. The battleships are represented w ...
- UP Board 串口使用心得
前言 原创文章,转载引用务必注明链接. 本文使用Markdown写成,为获得更好的阅读体验和正常的图片.链接,请访问我的博客: http://www.cnblogs.com/sjqlwy/p/up_s ...
- UP Board 网络设置一本通
前言 原创文章,转载引用务必注明链接,水平有限,欢迎指正. 本文环境:ubilinux 3.0 on UP Board 本文使用Markdown写成,为获得更好的阅读体验和正常的图片.链接,请访问我的 ...
- UP Board USB无线网卡一贴通
前言 原创文章,转载引用务必注明链接,水平有限,欢迎指正. 本文环境:ubilinux 3.0 kernel 4.4.0 本文使用Markdown写成,为获得更好的阅读体验和正常的图片.链接,请访问我 ...
- 在UP Board 上搭建M——L服务器
前言 原创文章,转载引用务必注明链接,水平有限,欢迎指正. 本文环境:ubilinux 3.0 on UP Board 初识免流 所谓免流,就是免除手机访问网络产生的流量费用.其原理在乌云网上有过报道 ...
- UP Board 妄图启动ubilinux失败
前言 原创文章,转载引用务必注明链接. 经历了上次的上电开机失败,我们终于发现需要手动为UP板安装系统,因为没有显示器的Headless模式时,使用Linux比较方便,另外熟悉Debian系的,所以选 ...
随机推荐
- EPOLL原理详解(图文并茂)
文章核心思想是: 要清晰明白EPOLL为什么性能好. 本文会从网卡接收数据的流程讲起,串联起CPU中断.操作系统进程调度等知识:再一步步分析阻塞接收数据.select到epoll的进化过程:最后探究e ...
- java四则运算规则
java四则运算规则 1.基本规则 运算符:进行特定操作的符号.例如:+ 表达式:用运算符连起来的式子叫做表达式.例如:20 + 5.又例如:a + b 四则运算: 加:+ 减:- 乘:* 除:/ 取 ...
- pow()是如何实现的?
如1.5 ** 2.5,如何计算?似乎是这样的: 1. cmath calculates pow(a,b) by performing exp(b * log(a)). stackoverflow 2 ...
- Mysql索引数据结构详解(1)
慢查询解决:使用索引 索引是帮助Mysql高效获取数据的排好序的数据结构 常见的存储数据结构: 二叉树 二叉树不适合单边增长的数据 红黑树(又称二叉平衡树) 红黑树会自动平衡父节点两边的 ...
- Scala(二)【基本使用】
一.变量和数据类型 1.变量 语法:val / var 变量名:变量类型 = 值 val name:String = "zhangsan" 注意 1.val定义的变量想到于java ...
- 【Netty】最透彻的Netty原理架构解析
这可能是目前最透彻的Netty原理架构解析 本文基于 Netty 4.1 展开介绍相关理论模型,使用场景,基本组件.整体架构,知其然且知其所以然,希望给大家在实际开发实践.学习开源项目方面提供参考. ...
- Oracle中的instr函数
最近修改某个条件,由原来输入一个数据修改为可以输入多个,如图所示: 在实现时用到了regexp_substr函数进行分割连接起来的数据,查询时还用到了instr函数进行判断,但出现了问题,当子库存输入 ...
- Dubbo多注册中心
一.创建提供者08-provider-registers (1) 创建工程 直接复制05-provider-group工程,并命名为08-provider-registers (2) 修改配置文件 二 ...
- lucene索引的增、删、改
package com.hope.lucene;import org.apache.lucene.document.Document;import org.apache.lucene.document ...
- 二进制转换为ip地址
#include <stdio.h> #include<math.h> int power(int b)//定义幂函数 { int i = 2, j = 1; if (b == ...