【Algorithm】基数排序
一. 算法描述
- 分配,先从个位开始,根据位值(0-9)分别放到0~9号桶中(比如53,个位为3,则放入3号桶中)
- 收集,再将放置在0~9号桶中的数据按顺序放到数组中
- 重复(1)(2)过程,从个位到最高位(比如32位无符号整形最大数4294967296,最高位10位)

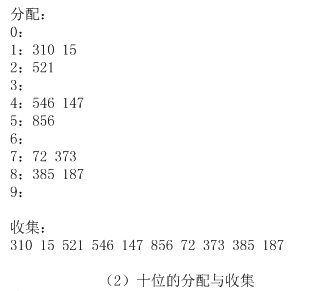

二. 算法实现
#include<stdio.h>
#define MAX 20
#define SHOWPASS
#define BASE 10 // 打印数组
void print(int *a, int n) {
int i;
for (i = ; i < n; i++) {
printf("%d\t", a[i]);
}
} // 基数排序
void radixsort(int *a, int n) {
int i, b[MAX], m = a[], exp = ; // 得到数组的最大值
for (i = ; i < n; i++) {
if (a[i] > m) {
m = a[i];
}
} while (m / exp > ) {
int bucket[BASE] = { }; for (i = ; i < n; i++) {
bucket[(a[i] / exp) % BASE]++;
} for (i = ; i < BASE; i++) {
bucket[i] += bucket[i - ];
} for (i = n - ; i >= ; i--) {
b[--bucket[(a[i] / exp) % BASE]] = a[i];
} for (i = ; i < n; i++) {
a[i] = b[i];
} exp *= BASE; #ifdef SHOWPASS
printf("\nPASS : ");
print(a, n);
#endif
}
} int main() {
int arr[MAX];
int i, n;
printf("请输入总元素数目 (n <= %d) : ", MAX);
scanf("%d", &n);
n = n < MAX ? n : MAX; printf("请输入 %d 个元素 : \n", n);
for (i = ; i < n; i++) {
scanf("%d", &arr[i]);
} printf("\n排序前 : ");
print(&arr[], n); radixsort(&arr[], n); printf("\n排序之后 : ");
print(&arr[], n);
printf("\n"); return ;
}
三. 算法分析
- 平均时间复杂度:O(dn)(d即表示整形的最高位数)
- 空间复杂度:O(rn) (r表示0~9,用于存储临时的序列)
- 稳定性:稳定
参考资料
[1] http://blog.csdn.net/cjf_iceking/article/details/7943609
[2] http://zh.wikipedia.org/wiki/%E5%9F%BA%E6%95%B0%E6%8E%92%E5%BA%8F
【Algorithm】基数排序的更多相关文章
- 【HNOI 2018】寻宝游戏
Problem Description 某大学每年都会有一次 \(Mystery\ Hunt\) 的活动,玩家需要根据设置的线索解谜,找到宝藏的位置,前一年获胜的队伍可以获得这一年出题的机会. 作为新 ...
- Algorithm in Practice - Sorting and Searching
Algorithm in Practice Author: Zhong-Liang Xiang Date: Aug. 1st, 2017 不完整, 部分排序和查询算法, 需添加. Prerequisi ...
- BZOJ.5285.[AHOI/HNOI2018]寻宝游戏(思路 按位计算 基数排序..)
BZOJ LOJ 洛谷 话说vae去年的专辑就叫寻宝游戏诶 只有我去搜Mystery Hunt和infinite corridor了吗... 同样按位考虑,假设\(m=1\). 我们要在一堆\(01\ ...
- [Algorithm] Warm-up puzzles
闲下来后,需要讲最近涉及到的算法全部整理一下,有个indice,方便记忆宫殿的查找 MIT的算法课,地球上最好:https://ocw.mit.edu/courses/electrical-engin ...
- 小白初识 - 基数排序(RadixSort)
基数排序算是桶排序和计数排序的衍生吧,因为基数排序里面会用到这两种其中一种. 基数排序针对的待排序元素是要有高低位之分的,比如单词adobe,activiti,activiti就高于adobe,这个是 ...
- 基数排序——尚未补完的坑QAQ
基数排序复杂度是(n+b)logn/logb 我们找一个基数 每次处理一部分位 从低位到高位处理 t是出现次数 s是这个桶管辖的起点 然后就可以写了 不过我这里是指针版的 有点难看 #include& ...
- 洛谷【P1177】【模板】基数排序
题目传送门:https://www.luogu.org/problemnew/show/P1177 我对计数排序的理解:https://www.cnblogs.com/AKMer/p/9649032. ...
- POJ 2388 基数排序
这题可以直接nth_element过去 比如这样子 //By SiriusRen #include <cstdio> #include <algorithm> using na ...
- [Algorithm] 面试题之犄角旮旯 第贰章
闲下来后,需要讲最近涉及到的算法全部整理一下,有个indice,方便记忆宫殿的查找 MIT的算法课,地球上最好: Design and Analysis of Algorithms 本篇需要重新整理, ...
随机推荐
- 运行代码时报linker command failed with exit code 1 错误
一个c语言项目,在.h文件中原来只有一些方法的声明,后来我加入了一些变量声明后,编译的时候报错: 运行代码时报linker command failed with exit code 1 错误 怎么回 ...
- [Canvas]RPG游戏雏形 (地图加载,英雄出现并移动)
源码请点此下载并用浏览器打开index.html观看 图例: 代码: <!DOCTYPE html> <html lang="utf-8"> <met ...
- Android 模仿QQ空间风格的 UI
本文内容 环境 演示模仿QQ空间风格的UI 虽然这个 UI 跟现在的QQ空间有点差别,但是也能学到很多东西. 下载 Demo 环境 Windows 7 64 位 Eclipse ADT V22.6.2 ...
- yii源码三 -- db
<AR> CActiveRecord:path:/framework/db/ar/CActiveRecord.phpoverview:is the base class for class ...
- 如何设置Apache中的最大连接数
Apache的主要工作模式有两种:prefork和worker 一.两种模式 prefork模式(缺省模式) prefork是Unix平台上的默认(缺省)MPM,使用多个子进程,每个子进程只有一个线程 ...
- VMware用于Site Recovery Manager 5的vSphere Replication功能一览
http://www.searchstorage.com.cn/showcontent_54838.htm 参考:深度解析SRM 5.0和vSphere Replication http://wenk ...
- vsphere 5.1 性能最佳实践。
1.关于CPU负载.extop显示的结果 如果CPU load average>=1,说明主机过载了. 如果PCPU used%在80%左右说明良好,90%以上就临近过载了. VM赋予过多的vC ...
- 1004: 不明飞行物(ufo)
#include <iostream> #include <iomanip> #include <cstdlib> #include <string> ...
- WIP 004 - Quote/Policy Search
Please create the search form Auto complete for first name and last name Related tables System_LOBs ...
- …… are only available on JDK 1.5 and higher 错误
"C:\Program Files\Java\jdk1.8.0_73\bin\java" -ea -Didea.test.cyclic.buffer.size=1048576 &q ...