Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(五)
直接贴代码了:
NewsInfo 实体类:
public class NewsInfo
{
public int NewsInfoId { get; set; }
public string NewsInfoTitle { get; set; } public int? NewsTypeId { get; set; } public virtual NewsType NewsTypeInfo { get; set; }
}
NewsType 实体类:
public class NewsType
{
public int TypeId { get; set; } //[MaxLength(50)]
public string TypeName { get; set; } /// <summary>
/// 产品列表
/// </summary>
public virtual ICollection<NewsInfo> NewsInfoList { get; set; }
}
NewsInfoMap 实体映射类(利用 EF Fluent API 注册)
public class NewsInfoMap : EntityTypeConfiguration<NewsInfo>
{
public NewsInfoMap()
{
this.ToTable("NewsInfos"); // Primary Key
this.HasKey(t => t.NewsInfoId); // Properties
this.Property(t => t.NewsInfoId)
.HasDatabaseGeneratedOption(DatabaseGeneratedOption.Identity);
this.Property(t => t.NewsInfoTitle).HasColumnName("Title")
.IsRequired()
.HasMaxLength(); // Relationships
this.HasRequired(n => n.NewsTypeInfo)
.WithMany(t => t.NewsInfoList)
.HasForeignKey(t => t.NewsTypeId)
.WillCascadeOnDelete(false);
}
}
NewsTypeMap 实体映射类(利用 EF Fluent API 注册)
public class NewsTypeMap : EntityTypeConfiguration<NewsType>
{
public NewsTypeMap()
{
this.ToTable("NewsTypes"); // Primary Key
this.HasKey(t => t.TypeId); // Properties
this.Property(t => t.TypeId).HasColumnName("NewsTypeId")
.HasDatabaseGeneratedOption(DatabaseGeneratedOption.Identity);
this.Property(t => t.TypeName).HasColumnName("NewsTypeName")
.HasMaxLength();
}
}
NewContext
public class NewContext : DbContext
{
static NewContext()
{
Database.SetInitializer(new DropCreateDatabaseIfModelChanges<NewContext>()); // 架构改变,自动删除,并新建数据库
} public DbSet<NewsInfo> NewsInfos { get; set; }
public DbSet<NewsType> NewsTypes { get; set; } protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Configurations.Add(new NewsInfoMap());
modelBuilder.Configurations.Add(new NewsTypeMap());
}
}
实际测试:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data.Entity;
using System.ComponentModel.DataAnnotations;
using CodeFirstDemo.Extensions;
using System.Data.Entity.ModelConfiguration;
using System.ComponentModel.DataAnnotations.Schema;
using System.Reflection;
using static CodeFirstDemo.Extensions.MetaHelper; namespace CodeFirstDemo
{
class Program
{
static void Main(string[] args)
{
using (var db = new NewContext())
{
//TestInsertAndQuery(db);
TestGetDbContextMetaInfo(db); Console.ReadKey();
}
} static void TestInsertAndQuery(NewContext db)
{
Console.Write("Please Input News Type Title: ");
var name = Console.ReadLine(); var type_Model = new NewsType { TypeName = name };
db.NewsTypes.Add(type_Model);
db.SaveChanges(); Console.Write("Please Input Search Type Name : ");
var search_type = Console.ReadLine();
var query = from b in db.NewsTypes
where b.TypeName == search_type
select b; Console.WriteLine("Query Result:");
foreach (var item in query)
{
Console.WriteLine(item.TypeName);
}
Console.WriteLine("\n\n\n");
} static void TestGetDbContextMetaInfo(NewContext db)
{
Console.WriteLine("DbContext Meta: \n"); // 测试环境:Win10、SQL Server Express 2008、EntityFramework.6.1.0
// 经过实际测试,下面的所有代码,只有在第一个调用 db.GetTableName<NewsInfo>(); 才会比较慢,因为这
// 时候 EF 会从数据库中拿数据,后面的第二个(db.GetTableName<NewsType>()) 一直到结束都比较快了。可
// 能是 EF 已经缓存了 EDM 信息,所以就比较快了。 // Table Name
string newsTableName = db.GetTableName<NewsInfo>();
Console.WriteLine("NewsInfo TableName: " + newsTableName); // dbo.NewsInfos string NewTypeTableName = db.GetTableName<NewsType>();
Console.WriteLine("NewsType TableName: " + NewTypeTableName); //dbo.NewsTypes Console.WriteLine("\n"); // PK Name
var newsPKNameDic = db.GetTableKeyColumns<NewsInfo>(); // key=DbColumnName, Value= C# Property Info
Console.Write("newsPKName: ");
PrintStringPropertyInfoDic(newsPKNameDic); // ( NewsInfoId: NewsInfoId ) var newsTypePKNameDic = db.GetTableKeyColumns<NewsType>(); // key=DbColumnName, Value= C# Property Info
Console.Write("newsTypePKName: ");
PrintStringPropertyInfoDic(newsTypePKNameDic); // (NewsTypeId: TypeId ) Console.WriteLine("\n"); // all column name - property info
var newsAllColumnNamePropInfoDic = db.GetTableColumns<NewsInfo>(); // key=DbColumnName, Value = C# Property Info
Console.Write("news all column name - property info dictionary: ");
PrintStringPropertyInfoDic(newsAllColumnNamePropInfoDic); //( NewsInfoId: NewsInfoId ),( Title: NewsInfoTitle ),( NewsTypeId: NewsTypeId ) var newsTypeAllColumnNamePropInfoDic = db.GetTableColumns<NewsType>(); // key=DbColumnName, Value = C# Property Info
Console.Write("news type all column name - property info dictionary: ");
PrintStringPropertyInfoDic(newsTypeAllColumnNamePropInfoDic); //( NewsTypeId: TypeId ),( NewsTypeName: TypeName ) Console.WriteLine("\n"); // all column name - property name
var newsAllColumnNamePropNameDic = db.GetPropertyColumnNames<NewsInfo>(); // key= C# Property Name, Value= DbColumnName
Console.Write("news all column name - property name dictionary: ");
PrintPkNameDicCore(newsAllColumnNamePropNameDic, null); // ( NewsInfoId: NewsInfoId ),( NewsInfoTitle: Title ),( NewsTypeId: NewsTypeId ) var newsTypeAllColumnNamePropNameDic = db.GetPropertyColumnNames<NewsType>(); // key= C# Property Name, Value= DbColumnName
Console.Write("news type all column name - property name dictionary: ");
PrintPkNameDicCore(newsTypeAllColumnNamePropNameDic, null); // ( TypeId: NewsTypeId ),( TypeName: NewsTypeName ) Console.WriteLine("\n"); //经过测试,下面这 3 行代码发生了异常!
//List<EntityKey> newDependentList = db.GetDependentTypes(typeof(NewsInfo));
//Console.WriteLine("news dependent types: ");
//PrintEntityKeyList(newDependentList);
//Console.WriteLine(""); List<EntityKey> newsTypeDependentList = db.GetDependentTypes(typeof(NewsType));
// newsTypeDependentList 里面的信息如下:
// type= C#实体类的名称,比如:NewsInfo。
// Keys = 一个或外键字段对应的 C# Property 信息。比如:Nullable<int> NewsTypeId
Console.WriteLine("news type dependent types: ");
PrintEntityKeyList(newsTypeDependentList); // ( NewsInfo: { type=Nullable`1, name=NewsTypeId } ) Console.WriteLine("\n"); //
var newsPKColumnIdentityDic = db.GetComputedColumnNames<NewsInfo>();
Console.Write("news primaryKey column name - identity dictionary: ");
PrintPkNameDicCore(newsPKColumnIdentityDic, null); // ( NewsInfoId: True ) var newsTypePKColumnIdentityDic = db.GetComputedColumnNames<NewsType>();
Console.Write("news type primaryKey column name - identity dictionary: ");
PrintPkNameDicCore(newsTypePKColumnIdentityDic, null); // ( NewsTypeId: True ) Console.WriteLine("\n"); //
string newsInfoTitleColumnName = db.GetColumnName<NewsInfo>("NewsInfoTitle");
Console.Write("newsInfo title column name: " + newsInfoTitleColumnName); // Title
Console.WriteLine(""); string typeNameColumnName = db.GetColumnName<NewsType>("TypeName");
Console.Write("NewsType typeName column name: " + typeNameColumnName); // NewsTypeName
Console.WriteLine(""); Console.WriteLine("\n"); } static void PrintStringPropertyInfoDic(Dictionary<string, PropertyInfo> infoDic)
{
PrintPkNameDicCore<PropertyInfo>(infoDic, t => t.Name);
} static void PrintPkNameDicCore<T>(Dictionary<string, T> infoDic, Func<T, object> printCoreFunc)
{
int i = ;
foreach (var item in infoDic)
{
if (i > )
{
Console.Write(",");
}
Console.Write("( {0}: {1} )", item.Key, printCoreFunc == null ? item.Value : printCoreFunc(item.Value));
i++;
}
Console.WriteLine("");
} static void PrintEntityKeyList(IEnumerable<EntityKey> entityKeyList)
{
int i = ;
foreach (var item in entityKeyList)
{
if (i > )
{
Console.Write(",");
}
string keyTextInfo = string.Join(",", item.Keys.Select(c => string.Format("type={0}, name={1} ", c.PropertyType.Name, c.Name)));
Console.Write("( {0}: {{ {1} }} )", item.Type.Name, keyTextInfo);
i++;
}
Console.WriteLine("");
}
} }
运行效果图
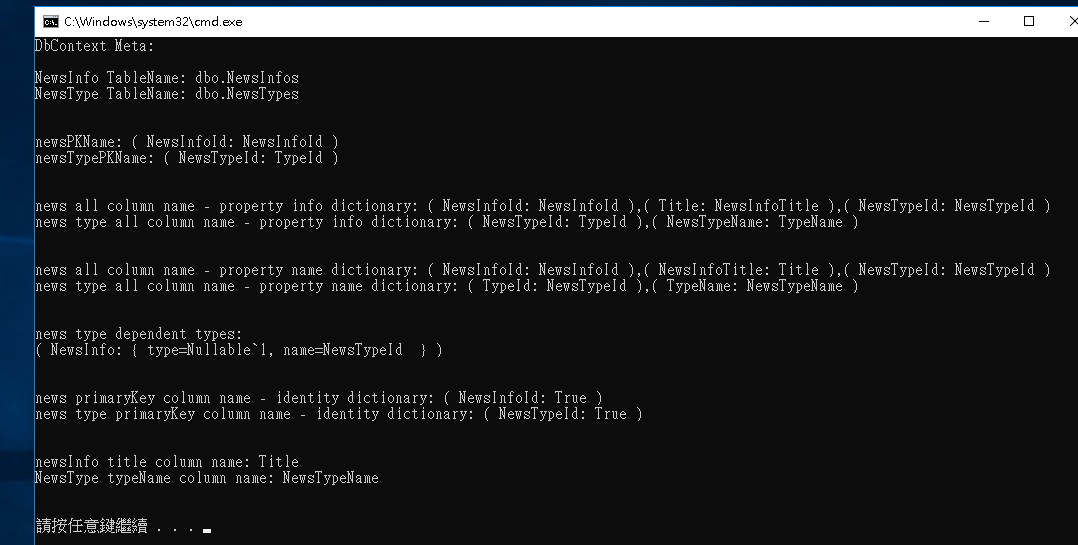
谢谢浏览!
Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(五)的更多相关文章
- Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(一)
1. 案例1 - 类型和表之间的EF代码优先映射 从EF6.1开始,有一种更简单的方法可以做到这一点.有关 详细信息,请参阅我的新EF6.1类型和表格之间的映射. 直接贴代码了 从EF6.1开始,有一 ...
- Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(四)
经过上一篇,里面有测试代码,循环60万次,耗时14秒.本次我们增加缓存来优化它. DbContextExtensions.cs using System; using System.Collectio ...
- Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(三)
接着上一篇,我们继续来优化. 直接贴代码了: LambdaHelper.cs using System; using System.Collections.Generic; using System. ...
- Entity Framework 6 中如何获取 EntityTypeConfiguration 的 Edm 信息?(二)
接着上一篇 直接贴代码了: using System; using System.Collections.Generic; using System.Data.Entity; using System ...
- 浅析Entity Framework Core中的并发处理
前言 Entity Framework Core 2.0更新也已经有一段时间了,园子里也有不少的文章.. 本文主要是浅析一下Entity Framework Core的并发处理方式. 1.常见的并发处 ...
- 在Entity Framework 7中进行数据迁移
(此文章同时发表在本人微信公众号“dotNET每日精华文章”,欢迎右边二维码来关注.) 题记:虽然EF7重新设计了Entity Framework,不过也还是能够支持数据迁移的. Entity Fra ...
- [Programming Entity Framework] 第3章 查询实体数据模型(EDM)(一)
http://www.cnblogs.com/sansi/archive/2012/10/18/2729337.html Programming Entity Framework 第二版翻译索引 你可 ...
- 如何处理Entity Framework / Entity Framework Core中的DbUpdateConcurrencyException异常(转载)
1. Concurrency的作用 场景有个修改用户的页面功能,我们有一条数据User, ID是1的这个User的年龄是20, 性别是female(数据库中的原始数据)正确的该User的年龄是25, ...
- Entity Framework添加记录时获取自增ID值
与Entity Framework相伴的日子痛并快乐着.今天和大家分享一下一个快乐,两个痛苦. 先说快乐的吧.Entity Framework在将数据插入数据库时,如果主键字段是自增标识列,会将该自增 ...
随机推荐
- Linux 内存释放
简介 linux 内存释放通过如下命令,将cache与buff根据环境进行释放操作,避免重启释放内存. 操作 1.将内存中buff数据保存磁盘 sync 2.清理cache与buff缓存 echo 3 ...
- 【LOJ#6682】梦中的数论(min_25筛)
[LOJ#6682]梦中的数论(min_25筛) 题面 LOJ 题解 注意题意是\(j|i\)并且\((j+k)|i\), 不难发现\(j\)和\((j+k)\)可以任意取\(i\)的任意因数,且\( ...
- 转 SSD论文解读
版权声明:本文为博主原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接和本声明. 本文链接:https://blog.csdn.net/u010167269/article/det ...
- Python爬取酷狗飙升榜前十首(100)首,写入CSV文件
酷狗飙升榜,写入CSV文件 爬取酷狗音乐飙升榜的前十首歌名.歌手.时间,是一个很好的爬取网页内容的例子,对爬虫不熟悉的读者可以根据这个例子熟悉爬虫是如何爬取网页内容的. 需要用到的库:requests ...
- vscode 设置代码格式化缩进为2个空格
打开文件——>首选——>设置 输入搜索 tabsize 按照下图设置即可,然后打开 注意:如果不将Detect Indentation 勾选取消 以前用tab创建的忘记依然为4个空格
- 被公司的垃圾XG人事系统吓尿了
OA要尝试设置单点登录,拿现有的HR系统尝试,结果不知道HR系统的加密方式和验证地址,于是乎找HR厂商——厦门XG软件实施人员.结果那个技术人员支支吾吾不肯给我,搞得非常的烦. 真奇怪了,不开源的软件 ...
- WindowServer优化
Windows Server 2016 禁止自动更新 1. 打开cmd,输入sconfig,出现如下图: 2. 输入5回车,在输入m回车,完成关闭自动更新.
- Asp.Net Core 开发之旅之NLog日志
NLog已是日志库的一员大佬,使用也简单方便,本文介绍的环境是居于.NET CORE 3.0 1.安装 Install-Package NLog.Web.AspNetCore 2.创建配置文件 在we ...
- [Go] 利用channel形成管道沟通循环内外
这个要解决的问题是,比如如果有一个大循环,取自一个大的文件,要进行逻辑处理,那么这个逻辑的代码要放在循环每一行的循环体里面,这样有可能会出现一个for循环的逻辑嵌套,一层又一层,类似俄罗斯套娃.如果放 ...
- Lnmp架构部署动态网站环境.2019-7-3-1.3
Php安装 一.安装准备 1.Php依赖包 [root@Lnmp tools]# yum install -y zlib libxml libjpeg freetype libpng gd curl ...