UVA - 699The Falling Leaves(递归先序二叉树)
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
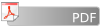
Each year, fall in the North Central region is accompanied by the brilliant colors of the leaves on the trees, followed quickly by the falling leaves accumulating under the trees. If the same thing happened to binary trees, how large would the piles of leaves become?
We assume each node in a binary tree "drops" a number of leaves equal to the integer value stored in that node. We also assume that these leaves drop vertically to the ground (thankfully, there's no wind to blow them around). Finally, we assume that the nodes are positioned horizontally in such a manner that the left and right children of a node are exactly one unit to the left and one unit to the right, respectively, of their parent. Consider the following tree:
The nodes containing 5 and 6 have the same horizontal position (with different vertical positions, of course). The node containing 7 is one unit to the left of those containing 5 and 6, and the node containing 3 is one unit to their right. When the "leaves" drop from these nodes, three piles are created: the leftmost one contains 7 leaves (from the leftmost node), the next contains 11 (from the nodes containing 5 and 6), and the rightmost pile contains 3. (While it is true that only leaf nodes in a tree would logically have leaves, we ignore that in this problem.)
Input
The input contains multiple test cases, each describing a single tree. A tree is specified by giving the value in the root node, followed by the description of the left subtree, and then the description of the right subtree. If a subtree is empty, the value -1 is supplied. Thus the tree shown above is specified as 5 7 -1 6 -1 -1 3 -1 -1. Each actual tree node contains a positive, non-zero value. The last test case is followed by a single -1 (which would otherwise represent an empty tree).
Output
For each test case, display the case number (they are numbered sequentially, starting with 1) on a line by itself. On the next line display the number of "leaves" in each pile, from left to right, with a single space separating each value. This display must start in column 1, and will not exceed the width of an 80-character line. Follow the output for each case by a blank line. This format is illustrated in the examples below.
Sample Input
5 7 -1 6 -1 -1 3 -1 -1
8 2 9 -1 -1 6 5 -1 -1 12 -1
-1 3 7 -1 -1 -1
-1
Sample Output
Case 1:
7 11 3 Case 2:
9 7 21 15
题解:给你一个先序二叉树,其中左子结点在父节点左一个单位,右节点在父节点右一个单位;让从左到右输出每个水平位置的权值和;本来用的从1开始root<<1建树,发现思路行不通;因为每个节点的root不同,所以考虑到从MAXN/2开始,-1,+1进行建树不就可以了么,每次如果不等于-1,就加上权值,然后找到左边最小的root,右边最大的root输出答案即可;
#include<cstdio>
#include<cstring>
#include<cmath>
#include<algorithm>
#include<queue>
using namespace std;
#define SI(x) scanf("%d",&x)
#define mem(x,y) memset(x,y,sizeof(x))
#define PI(x) printf("%d",x)
#define P_ printf(" ")
const int INF=0x3f3f3f3f;
typedef long long LL;
const int MAXN=100010;
int tree[MAXN];
int flot,cnt;
int l,r;
void solve(int root){
int x;
scanf("%d",&x);
if(!cnt&&x==-1){
flot=0;return;
}
cnt=1;
if(x!=-1){
l=min(l,root);
r=max(r,root);
tree[root]+=x;
solve(root-1);
solve(root+1);
} }
int main(){
int kase=0;
while(true){
flot=1;
memset(tree,0,sizeof(tree));
l=r=MAXN/2;
cnt=0;
solve(MAXN/2);
if(!flot)break;
printf("Case %d:\n",++kase);
for(int i=l;i<=r;i++){
if(i!=l)P_;printf("%d",tree[i]);
}
puts("\n");
}
return 0;
}
UVA - 699The Falling Leaves(递归先序二叉树)的更多相关文章
- Uva 699The Falling Leaves
0.唔.这道题 首先要明确根节点在哪儿 初始化成pos=maxn/2; 1.因为是先序的输入方法,所以这个建树的方法很重要 void build(int p) { int v; cin>> ...
- UVa 699 The Falling Leaves(递归建树)
UVa 699 The Falling Leaves(递归建树) 假设一棵二叉树也会落叶 而且叶子只会垂直下落 每个节点保存的值为那个节点上的叶子数 求所有叶子全部下落后 地面从左到右每 ...
- UVA 1525 Falling Leaves
题目链接:https://vjudge.net/problem/UVA-1525 题目链接:https://vjudge.net/problem/POJ-1577 题目大意 略. 分析 建树,然后先序 ...
- UVA.699 The Falling Leaves (二叉树 思维题)
UVA.699 The Falling Leaves (二叉树 思维题) 题意分析 理解题意花了好半天,其实就是求建完树后再一条竖线上的所有节点的权值之和,如果按照普通的建树然后在计算的方法,是不方便 ...
- UVA 699 The Falling Leaves (二叉树水题)
本文纯属原创.转载请注明出处,谢谢. http://blog.csdn.net/zip_fan. Description Each year, fall in the North Central re ...
- java创建二叉树并实现非递归中序遍历二叉树
java创建二叉树并递归遍历二叉树前面已有讲解:http://www.cnblogs.com/lixiaolun/p/4658659.html. 在此基础上添加了非递归中序遍历二叉树: 二叉树类的代码 ...
- 【LeetCode-面试算法经典-Java实现】【145-Binary Tree Postorder Traversal(二叉树非递归后序遍历)】
[145-Binary Tree Postorder Traversal(二叉树非递归后序遍历)] [LeetCode-面试算法经典-Java实现][全部题目文件夹索引] 原题 Given a bin ...
- UVa 699 The Falling Leaves (树水题)
Each year, fall in the North Central region is accompanied by the brilliant colors of the leaves on ...
- UVa699 The Falling Leaves
// UVa699 The Falling Leaves // 题意:给一棵二叉树,每个节点都有一个水平位置:左儿子在它左边1个单位,右儿子在右边1个单位.从左向右输出每个水平位置的所有结点的权值 ...
随机推荐
- You have not agreed to the Xcode license.
You have not agreed to the Xcode license. Before running the installer again please agree to the lic ...
- Flink资料(1)-- Flink基础概念(Basic Concept)
Flink基础概念 本文描述Flink的基础概念,翻译自https://ci.apache.org/projects/flink/flink-docs-release-1.0/concepts/con ...
- 分享一个自用的 Inno Setup 软件打包脚本
此脚本支持打包mysql.安装mysql服务.安装windows服务.操作ini文件.操作注册表.高效压缩文件等功能,基本能满足常用的软件打包需求. ;定义各种常量 #define MyAppName ...
- The Water Problem(排序)
The Water Problem Time Limit: 1500/1000 MS (Java/Others) Memory Limit: 131072/131072 K (Java/Othe ...
- poj 1094 Sorting It All Out(图论)
http://poj.org/problem?id=1094 这一题,看了个大牛的解题报告,思路变得非常的清晰: 1,先利用floyd_warshall算法求出图的传递闭包 2,再判断是不是存在唯一的 ...
- android基础5——使用资源
Android会基于当前的硬件.设备和语言配置来为某个资源标识符选择最合适的值. 1.在代码中使用资源 使用静态类R来访问资源.R类是基于外部资源而生的类,并且是在项目编译的时候创建的.R的每一个子类 ...
- Codeforces Round #277.5 (Div. 2)-D
题意:求该死的菱形数目.直接枚举两端的点.平均意义每一个点连接20条边,用邻接表暴力计算中间节点数目,那么中间节点任选两个与两端可组成的菱形数目有r*(r-1)/2. 代码: #include< ...
- 关于响应式、媒体查询和media的关系 、流媒体布局flex 和em rem像素的使用 我有一些废话要讲.....
一.什么是响应式 随着移动端越来遇火 网站的布局成为一个热议的话题 有的人喜欢用手机浏览网站.有的人喜欢用paid浏览网站.有人喜欢用电脑浏览网站 那么问题来了 我们怎么样才能使用一套css样式 完成 ...
- 给定数组A,大小为n,现给定数X,判断A中是否存在两数之和等于X
题目:给定数组A,大小为n,现给定数X,判断A中是否存在两数之和等于X 思路一: 1,先采用归并排序对这个数组排序, 2,然后寻找相邻<k,i>的两数之和sum,找到恰好sum>x的 ...
- Visusl Studio常用快捷键
Visusl Studio常用快捷键 快捷键 功能 ctrl+k,c 注释选中行 ctrl+k,u 取消对选中行的注释 CTRL + SHIFT + B:生成解决方案 CTRL + ...