Python进阶之路---1.4python数据类型-数字
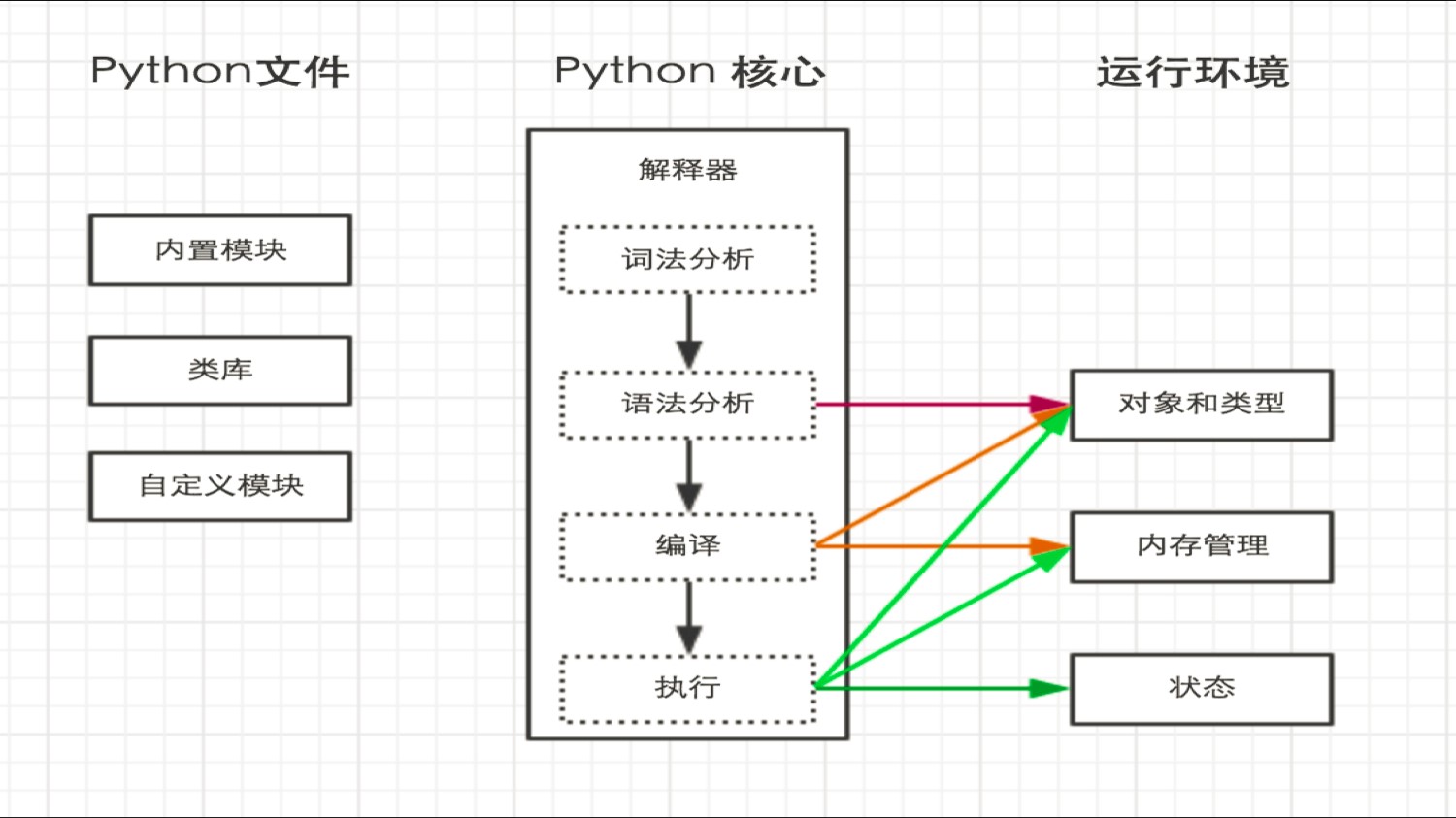
>>> ty =100
>>>print(ty)
100
5.数据类型-数字
在python中,变量就是变量,他没有类型,我们所说的“类型”是变量所指的内存中对象的类型。
python中有六个标准的数据类型:
Numbers (数字) String(字符串) List(列表) Tuple(元祖) sets(集合) Disctionaries(字典)
>>> a, b, c, d = 20, 5.5, True, 4+3j
>>> print(type(a), type(b), type(c), type(d))
<class 'int'> <class 'float'> <class 'bool'> <class 'complex'>
数值运算:
>>> 5 + 4 # 加法
9
>>> 4.3 - 2 # 减法
2.3
>>> 3 * 7 # 乘法
21
>>> 2 / 4 # 除法,得到一个浮点数
0.5
>>> 2 // 4 # 除法,得到一个整数,取商
0
>>> 17 % 3 # 取余
2
>>> 2 ** 5 # 乘方
32
数字常用的函数方法:
class int(object):
"""
int(x=0) -> integer
int(x, base=10) -> integer
将对象转换成字符类型
Convert a number or string to an integer, or return 0 if no arguments
are given. If x is a number, return x.__int__(). For floating point
numbers, this truncates towards zero. If x is not a number or if base is given, then x must be a string,
bytes, or bytearray instance representing an integer literal in the
given base. The literal can be preceded by '+' or '-' and be surrounded
by whitespace. The base defaults to 10. Valid bases are 0 and 2-36.
Base 0 means to interpret the base from the string as an integer literal.
>>> int('0b100', base=0)
4
"""
>>> a = ""
>>> type(a)
<class 'str'>
>>> b = int(a)
>>> type(b)
<class 'int'>
def bit_length(self): # real signature unknown; restored from __doc__
"""
int.bit_length() -> int Number of bits necessary to represent self in binary.
>>> bin(37)
'0b100101'
>>> (37).bit_length()
6
"""
return 0
def from_bytes(cls, bytes, byteorder, *args, **kwargs): # real signature unknown; NOTE: unreliably restored from __doc__
"""
int.from_bytes(bytes, byteorder, *, signed=False) -> int Return the integer represented by the given array of bytes. The bytes argument must be a bytes-like object (e.g. bytes or bytearray). The byteorder argument determines the byte order used to represent the
integer. If byteorder is 'big', the most significant byte is at the
beginning of the byte array. If byteorder is 'little', the most
significant byte is at the end of the byte array. To request the native
byte order of the host system, use `sys.byteorder' as the byte order value. The signed keyword-only argument indicates whether two's complement is
used to represent the integer.
"""
pass def to_bytes(self, length, byteorder, *args, **kwargs): # real signature unknown; NOTE: unreliably restored from __doc__
"""
int.to_bytes(length, byteorder, *, signed=False) -> bytes Return an array of bytes representing an integer. The integer is represented using length bytes. An OverflowError is
raised if the integer is not representable with the given number of
bytes. The byteorder argument determines the byte order used to represent the
integer. If byteorder is 'big', the most significant byte is at the
beginning of the byte array. If byteorder is 'little', the most
significant byte is at the end of the byte array. To request the native
byte order of the host system, use `sys.byteorder' as the byte order value. The signed keyword-only argument determines whether two's complement is
used to represent the integer. If signed is False and a negative integer
is given, an OverflowError is raised.
"""
pass
def __abs__(self, *args, **kwargs): # real signature unknown
""" abs(self) """
pass
用于获取数字的绝对值 age = -12
print(age)
b = age.__abs__()
print(b) output:
-12
12
def conjugate(self, *args, **kwargs): # real signature unknown
""" Returns self, the complex conjugate of any int. """
pass @classmethod # known case
def __add__(self, *args, **kwargs): # real signature unknown
""" Return self+value. """
pass 用户两个数字求和:
age = 12
print(age)
b = age.__add__(10)
print(b) output:
12
22
def __and__(self, *args, **kwargs): # real signature unknown
""" Return self&value. """
pass
用于进行两个数值的与运算
age = 12
print(age)
b = age.__and__(4)
print(b) output
12
4
用二进制与运算
00001100
00000100
结果
00000100
换算成10进制为
4
def __bool__(self, *args, **kwargs): # real signature unknown
""" self != 0 """
pass
布尔型判断
age = True
print(age)
b = age.__bool__()
print(b) output:
True
True
def __ceil__(self, *args, **kwargs): # real signature unknown
""" Ceiling of an Integral returns itself. """
pass
返回数字的上入整数,如果数值是小数,则返回的数值是整数加一
improt math
math.ceil(4.1) output:
5
def __divmod__(self, *args, **kwargs): # real signature unknown
""" Return divmod(self, value). """
pass
数字相除,将商和余数返回一个数组,相当于 a//b ,返回(商,余数)
age = 12
print(age)
b = age.__divmod__(5)
print(b)
print(type(b)) outuput:
12
(2, 2)
<class 'tuple'>
def __eq__(self, *args, **kwargs): # real signature unknown
""" Return self==value. """
pass
用于判断数值是否相等,等价于 a == b
age = 12
print(age)
b = age.__eq__(5)
print(b) output:
12
False
def __float__(self, *args, **kwargs): # real signature unknown
""" float(self) """
pass
用于判断数值是否是,浮点型,即小数型
age = 4.2
print(age)
b = age.__float__()
print(type(b)) output:
4.2
<class 'float'>
def __floordiv__(self, *args, **kwargs): # real signature unknown
""" Return self//value. """
pass
用于数字相除取其商,例如, 4//3 返回 1
age = 4
print(age)
b = age.__floordiv__(3)
print(b) output:
4
1
def __floor__(self, *args, **kwargs): # real signature unknown
""" Flooring an Integral returns itself. """
pass
def __format__(self, *args, **kwargs): # real signature unknown
pass
def __getattribute__(self, *args, **kwargs): # real signature unknown
""" Return getattr(self, name). """
pass
def __getnewargs__(self, *args, **kwargs): # real signature unknown
pass
def __ge__(self, *args, **kwargs): # real signature unknown
""" Return self>=value. """
pass
数字大小判断, self > value
age = 4
print(age)
b = age.__ge__(2)
print(b)
print(type(b)) output:
4
True
<class 'bool'>
def __gt__(self, *args, **kwargs): # real signature unknown
""" Return self>value. """
pass
大于等于,self >= value
age = 4
print(age)
b = age.__gt__(2)
print(b)
print(type(b)) output:
4
True
<class 'bool'>
def __index__(self, *args, **kwargs): # real signature unknown
""" Return self converted to an integer, if self is suitable for use as an index into a list. """
pass
用于切片,数字无意义
def __init__(self, x, base=10): # known special case of int.__init__
""" 构造方法,执行 x = 123 或 x = int(10) 时,自动调用,暂时忽略 """
"""
int(x=0) -> int or long
int(x, base=10) -> int or long Convert a number or string to an integer, or return 0 if no arguments
are given. If x is floating point, the conversion truncates towards zero.
If x is outside the integer range, the function returns a long instead. If x is not a number or if base is given, then x must be a string or
Unicode object representing an integer literal in the given base. The
literal can be preceded by '+' or '-' and be surrounded by whitespace.
The base defaults to 10. Valid bases are 0 and 2-36. Base 0 means to
interpret the base from the string as an integer literal.
>>> int('0b100', base=0)
4
# (copied from class doc)
"""
pass
def __int__(self):
""" 转换为整数 """
""" x.__int__() <==> int(x) """
pass
def __invert__(self):
""" x.__invert__() <==> ~x """
pass
def __le__(self, *args, **kwargs): # real signature unknown
""" Return self<=value. """
pass
小于等于
def __lshift__(self, *args, **kwargs): # real signature unknown
""" Return self<<value. """
pass
def __lt__(self, *args, **kwargs): # real signature unknown
""" Return self<value. """
pass 小于
def __mod__(self, *args, **kwargs): # real signature unknown
""" Return self%value. """
pass
取余数
def __mul__(self, *args, **kwargs): # real signature unknown
""" Return self*value. """
pass
乘积
def __ne__(self, *args, **kwargs): # real signature unknown
""" Return self!=value. """
pass
不等于
def __or__(self, *args, **kwargs): # real signature unknown
""" Return self|value. """
pass
或
def __pos__(self, *args, **kwargs): # real signature unknown
""" +self """
pass
def __pow__(self, *args, **kwargs): # real signature unknown
""" Return pow(self, value, mod). """
pass 幂
def __radd__(self, *args, **kwargs): # real signature unknown
""" Return value+self. """
pass
+
def __repr__(self):
"""转化为解释器可读取的形式 """
""" x.__repr__() <==> repr(x) """
pass def __str__(self):
"""转换为人阅读的形式,如果没有适于人阅读的解释形式的话,则返回解释器课阅读的形式"""
""" x.__str__() <==> str(x) """
pass
def __trunc__(self, *args, **kwargs):
""" 返回数值被截取为整形的值,在整形中无意义 """
pass
数值类型:
整型(int)-通常被称为是整型或整数,是正或负整数,不带小数点。
长整型(long integers)-无限大小的整数,整数最后是一个大写或者小写的L
浮点型(floadting point real values)-浮点型由整数部分与小数部分组成,也可以使用科学计数法表示
复数(complex numbers)-复数的虚部以字母J或j结尾。如2+3i
int | long | float | complex |
---|---|---|---|
10 | 51924361L | 0.0 | 3.14j |
100 | -0x19323L | 15.20 | 45.j |
-786 | 0122L | -21.9 | 9.322e-36j |
080 | 0xDEFABCECBDAECBFBAEl | 32.3+e18 | .876j |
-0490 | 535633629843L | -90. | -.6545+0J |
-0x260 | -052318172735L | -32.54e100 | 3e+26J |
0x69 | -4721885298529L | 70.2-E12 | 4.53e-7j |
)3ZJKN.png)
- 长整型也可以使用小写"L",但是还是建议您使用大写"L",避免与数字"1"混淆。Python使用"L"来显示长整型。
- Python还支持复数,复数由实数部分和虚数部分构成,可以用a + bj,或者complex(a,b)表示, 复数的实部a和虚部b都是浮点型
Python数学函数:
函数 | 返回值 ( 描述 ) |
---|---|
abs(x) | 返回数字的绝对值,如abs(-10) 返回 10 |
ceil(x) | 返回数字的上入整数,如math.ceil(4.1) 返回 5 |
cmp(x, y) | 如果 x < y 返回 -1, 如果 x == y 返回 0, 如果 x > y 返回 1 |
exp(x) | 返回e的x次幂(ex),如math.exp(1) 返回2.718281828459045 |
fabs(x) | 返回数字的绝对值,如math.fabs(-10) 返回10.0 |
floor(x) | 返回数字的下舍整数,如math.floor(4.9)返回 4 |
log(x) | 如math.log(math.e)返回1.0,math.log(100,10)返回2.0 |
log10(x) | 返回以10为基数的x的对数,如math.log10(100)返回 2.0 |
max(x1, x2,...) | 返回给定参数的最大值,参数可以为序列。 |
min(x1, x2,...) | 返回给定参数的最小值,参数可以为序列。 |
modf(x) | 返回x的整数部分与小数部分,两部分的数值符号与x相同,整数部分以浮点型表示。 |
pow(x, y) | x**y 运算后的值。 |
round(x [,n]) | 返回浮点数x的四舍五入值,如给出n值,则代表舍入到小数点后的位数。 |
sqrt(x) | 返回数字x的平方根,数字可以为负数,返回类型为实数,如math.sqrt(4)返回 2+0j |
python随机函数:
随机数可以用于数学,游戏,安全等领域中,还经常被嵌入到算法中,用以提高算法效率,并提高程序的安全性。
Python包含以下常用随机数函数:
函数 | 描述 |
---|---|
choice(seq) | 从序列的元素中随机挑选一个元素,比如random.choice(range(10)),从0到9中随机挑选一个整数。 |
randrange ([start,] stop [,step]) | 从指定范围内,按指定基数递增的集合中获取一个随机数,基数缺省值为1 |
random() | 随机生成下一个实数,它在[0,1)范围内。 |
seed([x]) | 改变随机数生成器的种子seed。如果你不了解其原理,你不必特别去设定seed,Python会帮你选择seed。 |
shuffle(lst) | 将序列的所有元素随机排序 |
uniform(x, y) | 随机生成下一个实数,它在[x,y]范围内。 |
Python三角函数:
函数 | 描述 | |
---|---|---|
acos(x) | 返回x的反余弦弧度值。 | |
asin(x) | 返回x的反正弦弧度值。 | |
atan(x) | 返回x的反正切弧度值。 | |
atan2(y, x) | 返回给定的 X 及 Y 坐标值的反正切值。 | |
cos(x) | 返回x的弧度的余弦值。 | |
hypot(x, y) | 返回欧几里德范数 sqrt(x*x + y*y)。 | |
sin(x) | 返回的x弧度的正弦值。 | |
tan(x) | 返回x弧度的正切值。 | |
degrees(x) | 将弧度转换为角度,如degrees(math.pi/2) , 返回90.0 | |
radians(x) | 将角度转换为弧度 |
Python数学常量:
常量 | 描述 |
---|---|
pi | 数学常量 pi(圆周率,一般以π来表示) |
e | 数学常量 e,e即自然常数(自然常数)。 |
注意:
- 1、Python可以同时为多个变量赋值,如a, b = 1, 2。
- 2、一个变量可以通过赋值指向不同类型的对象。
- 3、数值的除法(/)总是返回一个浮点数,要获取整数使用//操作符。
- 4、在混合计算时,Python会把整型转换成为浮点数。
Python进阶之路---1.4python数据类型-数字的更多相关文章
- Python进阶之路---1.5python数据类型-字符串
字符串 *:first-child { margin-top: 0 !important; } body>*:last-child { margin-bottom: 0 !important; ...
- python精进之路1---基础数据类型
python精进之路1---基本数据类型 python的基本数据类型如上图,重点需要掌握字符串.列表和字典. 一.int.float类型 int主要是用于整数类型计算,float主要用于小数. int ...
- Python进阶之路---1.2python版本差异
Python2.*与python3.*版本差异 作为一个初学者,我们应该如何选择python的版本进行学习呢,这两个版本有什么区别呢,接下来让我们简单了解一下,以便我们后续的学习. Python版本差 ...
- python进阶之路之文件处理
Python之文件处理 *:first-child { margin-top: 0 !important; } body>*:last-child { margin-bottom: 0 !imp ...
- python进阶之路4.2---装饰器
*:first-child { margin-top: 0 !important; } body>*:last-child { margin-bottom: 0 !important; } /* ...
- Python进阶之路---1.3python环境搭建
python环境安装 windows python环境安装 下载安装包 https://www.python.org/downloads/ 安装并指定安装目录 C:\python2 ...
- Python进阶之路---1.1python简介
Python简介 Python简介 Python (发音:[ 'paiθ(ə)n; (US) 'paiθɔn ]n.蟒蛇,巨蛇 ),是一种面向对象的解释 ...
- python全栈开发笔记---基本数据类型--数字型魔法
数字 int a1 =123 a2=456 int 讲字符串转换为数字 a = " #字符串 b = int(a) #将字符串转换成整形 b = b + 1000 #只有整形的时候才可以进 ...
- python进阶之路4.1---生成器与迭代器
*:first-child { margin-top: 0 !important; } body>*:last-child { margin-bottom: 0 !important; } /* ...
随机推荐
- myFocus焦点图插件
注意 1.焦点图初始化ID和图片最外层ID保持一致 2.图片列表外面必须包裹一个div,且id必须为pic http://demo.jb51.net/js/myfocus/tutorials.html ...
- C# TextBox控件 显示大量数据
串口通信:在使用TextBox空间显示数据时,因为要显示大量的接收到的数据,当数据量大且快速(串口1ms发送一条数据)时,使用+=的方式仍然会造成界面的卡顿(已使用多线程处理),但使用AppendTe ...
- (五)JS学习笔记 - Sizzle选择器
Sizzle词法解析 sizzle对于分组过滤处理都用正则,其中都有一个特点,就是都是元字符^开头,限制匹配的初始,所以tokenize也是从左边开始一层一层的剥离. •可能会应用到正则如下: // ...
- 字符串编码---hash函数的应用
之前就听说过有个叫做hash表的东西,这段时间在上信息论与编码,也接触了一些关于编码的概念,直到今天做百度之星的初赛的d题时,才第一次开始学并用hash 一开始我用的是mutimap和mutiset, ...
- uva 10212 - The Last Non-zero Digit.
#include <cstdio> #define ll long long const ll MOD = 1e9; int main() { ll N, M; while(scanf(& ...
- $().text() 和 $().html()
1:性能 stackflow:http://stackoverflow.com/questions/1910794/what-is-the-difference-between-jquery-text ...
- PHP中取出字符串中的空格 逗号
preg_replace("/\s| |,|,/","",$_str) PHP中取出字符串中的空格 逗号 (包括中文状态下)
- [vim]插件NerdTree
NerdTree 这是插件的作用就是在vim中增加一个资源管理器 使用 利用设置的快捷键 C-e 可以快速打开或者关闭文件树 然后在文件树中可以快速搜索到你的文件, o打开当当前, t打开一个新的ta ...
- 指针与数组、大小端之 printf("%x,%x,%x\n",*(a+1),ptr1[-1],*ptr2);
在X86系统下,以下程序输出的值为多少? #include <stdio.h> #include <stdlib.h> int main(void) { ]={,,,,}; ) ...
- TF.Learn
TF.Learn 手写文字识别 转载请注明作者:梦里风林Google Machine Learning Recipes 7官方中文博客 - 视频地址Github工程地址 https://githu ...