poj1039 Pipe【计算几何】
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions:11940 | Accepted: 3730 |
Description
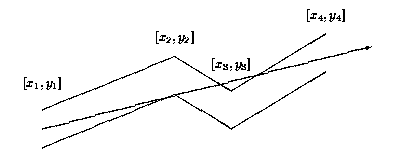
Each pipe component consists of many straight pipes connected tightly together. For the programming purposes, the company developed the description of each component as a sequence of points [x1; y1], [x2; y2], . . ., [xn; yn], where x1 < x2 < . . . xn . These are the upper points of the pipe contour. The bottom points of the pipe contour consist of points with y-coordinate decreased by 1. To each upper point [xi; yi] there is a corresponding bottom point [xi; (yi)-1] (see picture above). The company wants to find, for each pipe component, the point with maximal x-coordinate that the light will reach. The light is emitted by a segment source with endpoints [x1; (y1)-1] and [x1; y1] (endpoints are emitting light too). Assume that the light is not bent at the pipe bent points and the bent points do not stop the light beam.
Input
Output
Sample Input
4
0 1
2 2
4 1
6 4
6
0 1
2 -0.6
5 -4.45
7 -5.57
12 -10.8
17 -16.55
0
Sample Output
4.67
Through all the pipe.
Source
题意:
给n个点 构成两条平行的折线
问从管道口出发的光线最远能到达的横坐标
思路:
最远的光线一定是贴着管道的某两个端点走的
现在枚举这两个端点 判断其与后面折线的交点
刚开始没想到判断交点时 可以先判断line 和 line(up[k], down[k])
这样得到的k就是最小的不能达到的k
用这个k就可以拿来算line 和 line(up[k-1], up[k])以及line(down[k -1], down[k])的交点了
//#include <bits/stdc++.h>
#include<iostream>
#include<cmath>
#include<algorithm>
#include<stdio.h>
#include<cstring> using namespace std;
typedef long long int LL; #define zero(x) (((x) > 0? (x) : -(x)) < eps)
const double eps = 1e-;
int sgn(double x)
{
if(fabs(x) < eps) return ;
if(x < ) return -;
else return ;
} struct point{
double x, y;
point(){}
point(double _x, double _y)
{
x = _x;
y = _y;
}
point operator -(const point &b)const
{
return point(x - b.x, y - b.y);
}
double operator ^(const point &b)const
{
return x * b.y - y * b.x;
}
double operator *(const point &b)const
{
return x * b.x + y * b.y;
}
void input()
{
scanf("%lf%lf", &x, &y);
}
}; struct line{
point s, e;
line(){}
line(point _s, point _e)
{
s = _s;
e = _e;
}
//0表示直线重合,1表示平行,2相交
pair<int, point>operator &(const line &b)const
{
point res = s;
if(sgn((s - e) ^ (b.s - b.e)) == ){
if(sgn((s - b.e) ^ (b.s - b.e)) == ){
return make_pair(, res);
}
else return make_pair(, res);
}
double t = ((s - b.s) ^ (b.s - b.e)) / ((s - e) ^ (b.s - b.e));
res.x += (e.x - s.x) * t;
res.y += (e.y - s.y) * t;
return make_pair(, res);
}
}; //判断直线与线段相交
bool seg_inter_line(line l1, line l2)
{
return sgn((l2.s - l1.e) ^ (l1.s - l1.e)) * sgn((l2.e - l1.e) ^ (l1.s - l1.e)) <= ;
} int n;
point up[], down[];
int main()
{
while(scanf("%d", &n) != EOF && n != ){
for(int i = ; i < n; i++){
up[i].input();
down[i].x = up[i].x;
down[i].y = up[i].y - 1.0;
} bool flag = false;
double ans = -100000000.0;
int k;
for(int i = ; i < n; i++){
for(int j = i + ; j < n; j++){
for(k = ; k < n; k++){
if(seg_inter_line(line(up[i], down[j]), line(up[k], down[k])) == ){
break;
}
}
if(k >= n){
flag = true;
break;
}
if(k > max(i, j)){
if(seg_inter_line(line(up[i], down[j]), line(up[k - ], up[k]))){
pair<int, point>pr = line(up[i], down[j]) & line(up[k - ], up[k]);
point p = pr.second;
ans = max(ans, p.x);
}
if(seg_inter_line(line(up[i], down[j]), line(down[k - ], down[k]))){
pair<int, point>pr = line(up[i], down[j]) & line(down[k - ], down[k]);
point p = pr.second;
ans = max(ans, p.x);
}
} for(k = ; k < n; k++){
if(seg_inter_line(line(down[i], up[j]), line(up[k], down[k])) == ){
break;
}
}
if(k >= n){
flag = true;
break;
}
if(k > max(i, j)){
if(seg_inter_line(line(down[i], up[j]), line(up[k - ], up[k]))){
pair<int, point>pr = line(down[i], up[j]) & line(up[k - ], up[k]);
point p = pr.second;
ans = max(ans, p.x);
}
if(seg_inter_line(line(down[i], up[j]), line(down[k - ], down[k]))){
pair<int, point>pr = line(down[i], up[j]) & line(down[k - ], down[k]);
point p = pr.second;
ans = max(ans, p.x);
}
}
}
if(flag){
break;
}
}
//cout<<ans<<endl;
if(flag){
printf("Through all the pipe.\n");
}
else{
printf("%.2f\n", ans);
}
}
return ;
}
poj1039 Pipe【计算几何】的更多相关文章
- poj1039 Pipe(计算几何叉积求交点)
F - Pipe Time Limit:1000MS Memory Limit:10000KB 64bit IO Format:%I64d & %I64u Submit Sta ...
- POJ1039 Pipe
嘟嘟嘟 大致题意:按顺序给出\(n\)个拐点表示一个管道,注意这些点是管道的上端点,下端点是对应的\((x_i, y_i - 1)\).从管道口射进一束光,问能达到最远的位置的横坐标.若穿过管道,输出 ...
- POJ 1039:Pipe 计算几何
Pipe Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 9773 Accepted: 2984 Description ...
- 杭电ACM分类
杭电ACM分类: 1001 整数求和 水题1002 C语言实验题——两个数比较 水题1003 1.2.3.4.5... 简单题1004 渊子赛马 排序+贪心的方法归并1005 Hero In Maze ...
- 转载:hdu 题目分类 (侵删)
转载:from http://blog.csdn.net/qq_28236309/article/details/47818349 基础题:1000.1001.1004.1005.1008.1012. ...
- hdoj Pipe&&南阳oj管道问题&&poj1039(计算几何问题...枚举)
Pipe Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submis ...
- Pipe(点积叉积的应用POJ1039)
Pipe Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 9723 Accepted: 2964 Description ...
- 【计算几何初步-代码好看了点线段相交】【HDU2150】Pipe
题目没什么 只是线段相交稍微写的好看了点 Pipe Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Jav ...
- POJ - 1039 Pipe(计算几何)
http://poj.org/problem?id=1039 题意 有一宽度为1的折线管道,上面顶点为(xi,yi),所对应的下面顶点为(xi,yi-1),假设管道都是不透明的,不反射的,光线从左边入 ...
随机推荐
- linux -- Ubuntu Server 安装图形界面
1.连接网络,你一定要确保网络通畅,如果你和我一样使用Wireless,那先找根网线插上,因为下面的安装都要通过网络下载组件的. 2.进入图形界面的命令是startX,敲击后会有安装xinit的提示. ...
- 最简单的基于FFMPEG+SDL的音频播放器 ver2 (採用SDL2.0)
===================================================== 最简单的基于FFmpeg的音频播放器系列文章列表: <最简单的基于FFMPEG+SDL ...
- 页面的checkbox框的全选与反选
if (typeof jQuery == 'undefined') { alert("请先导入jQuery");} else { jQuery.extend({ ...
- 【Java面试题】18 java中数组有没有length()方法?string没有lenght()方法?下面这条语句一共创建了多少个对象:String s="a"+"b"+"c"+"d";
数组没有length()这个方法,有length的属性.String有有length()这个方法. int a[]; a.length;//返回a的长度 String s; s.length();// ...
- win10系统下cmd输入一下安装的软件命令提示拒绝访问解决办法
问题:win10系统安装了nvm,cmd命令提示不是内部或外部命令 解决:卸载nvm,重新安装,再一次输入nvm,发现正常显示: 问题:win10安装了nvm之后,安装node成功,输入node命令, ...
- xpath的数据和节点类型以及XPath中节点匹配的基本方法
XPath数据类型 XPath可分为四种数据类型: 节点集(node-set) 节点集是通过路径匹配返回的符合条件的一组节点的集合.其它类型的数据不能转换为节点集. 布尔值(boolean) ...
- PHP-006
Q:应用程序执行时的路径 "D:\*******\protected\runtime" 是无效的. 请确定它是一个可被 Web server process 写入资料的目录. A: ...
- php 获取图片base64编码格式数据
$image_file = '1.jpg'; $image_info = getimagesize($image_file); $base64_image_content = "data:{ ...
- UE4 Run On Server与Run on owning client
- 第二篇:一个经典的比喻( 关于TCP连接API )
前言 编程是对现实世界的模拟,网络通信自然也是对现实世界通信的模拟.可以将网络通信中使用的各种API和对现实世界中的各种通信设备进行通讯的操作进行对比以加深理解. 对比 socket() 函数 vs ...