python's object model
[python's object model]
1、object.__init__(self[, ...])
如果subclass没有实现__init__,那么python类在实例化的时候,显然会调用到父ClassObject的__init__,所以在subclass没实现__init__的时候,对象可以正常实现继承特性。
如果subclass实现了__init__,但是没有调用super的__init__,则父类实例中的变量在子类实例中不会存在,因为没有执行父ClassObject的__init__,所以无法正常实现继承特性。
If a base class has an __init__() method, the derived class’s __init__() method, if any, must explicitly call it to ensure proper initialization of the base class part of the instance; for example: BaseClass.__init__(self, [args...])
需使用super来调用继承链上下一个__init__。
2、class.__mro__, class.mro(),MethodResolutionOrder
class.mro()是C3算法的实现,class.__mro__包含C3算法的結果。
从python2.3起C3算法用于检测多重继承中是否存在环,即A->B,B->A的情况,若存在则runtime错误。显然在C3算法的保证下,python2.3起多重继承序并不是深度优先,也不是广度优先。而是一种深度优先的拓扑排序。
C3算法参考:http://blog.sina.com.cn/s/blog_45ac0d0a01018488.html
3、super()函数按照C3算法生成的mro来选择后继类,即当前类后面的类对象中寻找。
super参考:http://www.cnblogs.com/lovemo1314/archive/2011/05/03/2035005.html
4. getattr(object, name[, default])
Return the value of the named attribute of object. name must be a string. If the string is the name of one of the object’s attributes, the result is the value of that attribute. For example, getattr(x, 'foobar') is equivalent to x.foobar. If the named attribute does not exist, default is returned if provided, otherwise AttributeError is raised.
5、hasattr(object, name)
The arguments are an object and a string. The result is True if the string is the name of one of the object’s attributes, False if not. (This is implemented by calling getattr(object, name) and seeing whether it raises an exception or not.)
6、None
None是一个特殊的常量。None和False不同。None不是0。None不是空字符串。None和任何其他的数据类型比较永远返回False。None有自己的数据类型NoneType。你可以将None复制给任何变量,但是你不能创建其他NoneType对象。
参考:http://eriol.iteye.com/blog/1319295
7、object.__call__(self[, args...])
Called when the instance is “called” as a function; if this method is defined, x(arg1, arg2, ...) is a shorthand for x.__call__(arg1, arg2, ...).
Class instances are callable only when the class has a __call__() method; x(arguments) is a shorthand for x.__call__(arguments).
8、所有的instance.__class__指向自己的Class Type,所有class.__class__指向type对象。
测试程序: 输出为:
可见所有class.__class__指向都一样
9、type(object) & type(name, bases, dict)
With one argument, return the type of an object. The return value is a type object. The isinstance() built-in function is recommended for testing the type of an object.
With three arguments, return a new type object. This is essentially a dynamic form of the class statement. The name string is the class name and becomes the __name__ attribute; the bases tuple itemizes the base classes and becomes the __bases__ attribute; and the dict dictionary is the namespace containing definitions for class body and becomes the __dict__ attribute.
10、type & object, type、object是python对象模型的两大基石,
1)type(object)函数是根据 instace.__class__.来判断类型,该对象的__name__即为类名
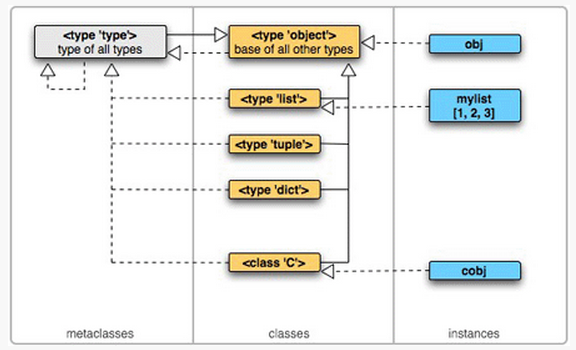
1)使用def class: body 定义的方法,均保存在 ClassType的 __dict__中,当instance要调用时,是则ClassType的__dict__中找出来的。
2)方法(函数)只有三种:
1))function:如果在ClassType的__dict__中,通过instance调用时,为绑定方法。如果在instance的__dict__中,通过instance调用,则为非绑定方法。
2))classmethod:绑定了cls
3))staticmethod:永远不会绑定
3)可以给ClassType、instance动态添加方法、属性,但object()生成的instance无法添加。
python's object model的更多相关文章
- Selenium的PO模式(Page Object Model)|(Selenium Webdriver For Python)
研究Selenium + python 自动化测试有近两个月了,不能说非常熟练,起码对selenium自动化的执行有了深入的认识. 从最初无结构的代码,到类的使用,方法封装,从原始函数 ...
- Page Object Model (Selenium, Python)
时间 2015-06-15 00:11:56 Qxf2 blog 原文 http://qxf2.com/blog/page-object-model-selenium-python/ 主题 Sel ...
- Selenium的PO模式(Page Object Model)[python版]
Page Object Model 简称POM 普通的测试用例代码: .... #测试用例 def test_login_mail(self): driver = self.driver driv ...
- Python+Selenium框架设计--- Page Object Model
POM(Page Object Model):页面对象模型,POM是一种最近几年非常流行的自动化测试模型,或者思想,POM不是一个框架,就是一个解决问题的思想.采用POM的目的,是为了解决前端中UI变 ...
- Nova Conductor 与 Versioned Object Model 机制
目录 文章目录 目录 Nova Conductor 数据库访问代理机制 Versioned Object Model 机制 Nova Conductor Conductor 服务作为 Nova 核心部 ...
- 在C#开发中如何使用Client Object Model客户端代码获得SharePoint 网站、列表的权限情况
自从人类学会了使用火,烤制的方式替代了人类的消化系统部分功能,从此人类的消化系统更加简单,加速了人脑的进化:自从SharePoint 2010开始有了Client Side Object Model ...
- 解决在使用client object model的时候报“object does not belong to a list”错误
在查看别人代码的时候,发现了个有意思的问题,使用client object model将一个文件check in 我使用的是如下语句获取file Microsoft.SharePoint.Client ...
- SharePoint Client Object Model API 介绍以及工作原理解析
CSOM和ServerAPI 的对比 SharePoint从2010开始引入了Client Object Model的API(后文中用CSOM来代替),从名字来看,我们可以简单的看出,该API是面向客 ...
- BOM (Browser Object Model) 浏览器对象模型
l对象的角色,因此所有在全局作用域中声明的变量/函数都会变成window对象的属性和方法; // PS:尝试访问未声明的变量会抛出错误,但是通过查询window对象,可以知道某个可能未声明的对象是否存 ...
随机推荐
- vue.js 源代码学习笔记 ----- decoder
/* @flow */ let decoder export function decode (html: string): string { decoder = decoder || documen ...
- c++的关联容器入门(map and set)
目录 std::map std::set C++的关联容器主要是两大类map和set 我们知道谈到C++容器时,我们会说到 顺序容器(Sequence containers),关联容器(Associa ...
- ubuntu16.04安装Nvidia显卡驱动、CUDA8.0和cudNN V6
Nvidia显卡驱动安装 在ubuntu搜索框输入 软件更新,打开 "软件和更新" 对话框,在 附加驱动里选择系统检测到的Nvidia驱动,应用更改,重启系统: 安装完成之后查看G ...
- BZOJ5091: [Lydsy1711月赛]摘苹果(简单概率)
5091: [Lydsy1711月赛]摘苹果 Time Limit: 1 Sec Memory Limit: 256 MBSubmit: 214 Solved: 163[Submit][Statu ...
- 博客网站-Hexo+GitHub+Netlify
Hexo+GitHub+Netlify一站式搭建属于自己的博客网站 https://www.cnblogs.com/kerbside/p/10130606.html https://hhongwen. ...
- AlertDialog中使用ListView绑定数据
在实际工作过程中,单单使用AlertDialog的单选功能不一定能满足我们的需求,需要绑定数据到 listview 1. 自定义Layout LayoutInflater factory = Layo ...
- java代码块执行顺序
父类 public class Father { public Father() { System.out.println("父类构造PUBLIC father"); } stat ...
- 升级到SQL Server 2012/2014时一些需要考虑的事项
1. 使用Upgrade Adviser评估升级前需要解决的事情. https://msdn.microsoft.com/zh-cn/library/ms144256(v=sql.110).aspx ...
- MacOS 如何截屏
在Mac OS X下有很强大的截屏功能,它不仅仅是对屏幕的全屏COPY,而是包括很多细节在里面,就从这点来看,已经比过所有版本的Windows了. 下面我来向大家详细介绍一下: 对全屏的截图我们可以通 ...
- SCSI学习笔记
1. sudo sg_inq --len=36 /dev/sda 使用INQUIRY来查询scsi设备信息