Sudoku POJ - 3076
Time Limit: 10000MS | Memory Limit: 65536K | |
Total Submissions: 5769 | Accepted: 2684 |
Description
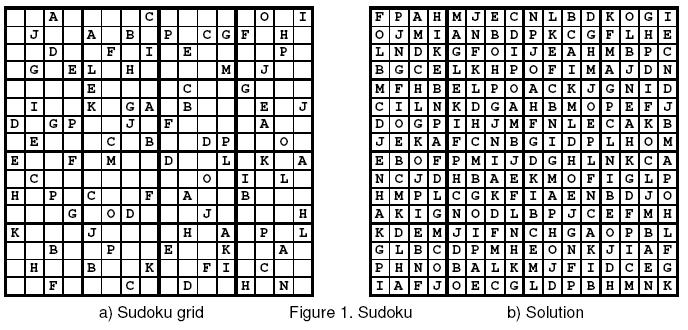
Write a Sudoku playing program that reads data sets from a text file.
Input
Output
Sample Input
--A----C-----O-I
-J--A-B-P-CGF-H-
--D--F-I-E----P-
-G-EL-H----M-J--
----E----C--G---
-I--K-GA-B---E-J
D-GP--J-F----A--
-E---C-B--DP--O-
E--F-M--D--L-K-A
-C--------O-I-L-
H-P-C--F-A--B---
---G-OD---J----H
K---J----H-A-P-L
--B--P--E--K--A-
-H--B--K--FI-C--
--F---C--D--H-N-
Sample Output
FPAHMJECNLBDKOGI
OJMIANBDPKCGFLHE
LNDKGFOIJEAHMBPC
BGCELKHPOFIMAJDN
MFHBELPOACKJGNID
CILNKDGAHBMOPEFJ
DOGPIHJMFNLECAKB
JEKAFCNBGIDPLHOM
EBOFPMIJDGHLNKCA
NCJDHBAEKMOFIGLP
HMPLCGKFIAENBDJO
AKIGNODLBPJCEFMH
KDEMJIFNCHGAOPBL
GLBCDPMHEONKJIAF
PHNOBALKMJFIDCEG
IAFJOECGLDPBHMNK
Source
这个就是2676变了一下形
直接上代码了
#include <iostream>
#include <cstdio>
#include <sstream>
#include <cstring>
#include <map>
#include <cctype>
#include <set>
#include <vector>
#include <stack>
#include <queue>
#include <algorithm>
#include <cmath>
#include <bitset>
#define rap(i, a, n) for(int i=a; i<=n; i++)
#define rep(i, a, n) for(int i=a; i<n; i++)
#define lap(i, a, n) for(int i=n; i>=a; i--)
#define lep(i, a, n) for(int i=n; i>a; i--)
#define rd(a) scanf("%d", &a)
#define rlld(a) scanf("%lld", &a)
#define rc(a) scanf("%c", &a)
#define rs(a) scanf("%s", a)
#define rb(a) scanf("%lf", &a)
#define rf(a) scanf("%f", &a)
#define pd(a) printf("%d\n", a)
#define plld(a) printf("%lld\n", a)
#define pc(a) printf("%c\n", a)
#define ps(a) printf("%s\n", a)
#define MOD 2018
#define LL long long
#define ULL unsigned long long
#define Pair pair<int, int>
#define mem(a, b) memset(a, b, sizeof(a))
#define _ ios_base::sync_with_stdio(0),cin.tie(0)
//freopen("1.txt", "r", stdin);
using namespace std;
const int maxn = , INF = 0x7fffffff;
int S[maxn], head[maxn], vis[maxn];
int U[maxn], D[maxn], L[maxn], R[maxn];
int C[maxn], X[maxn];
int n, m, ans, ret, ans1; void init()
{
for(int i = ; i <= m; i++)
D[i] = i, U[i] = i, R[i] = i + , L[i] = i - ;
L[] = m, R[m] = ;
mem(S, ), mem(head, -);
ans = m + ;
} void delc(int c)
{
L[R[c]] = L[c], R[L[c]] = R[c];
for(int i = D[c]; i != c; i = D[i])
for(int j = R[i]; j != i; j = R[j])
U[D[j]] = U[j], D[U[j]] = D[j], S[C[j]]--; } void resc(int c)
{
for(int i = U[c]; i != c; i = U[i])
for(int j = L[i]; j != i; j = L[j])
U[D[j]] = j, D[U[j]] = j, S[C[j]]++;
L[R[c]] = c, R[L[c]] = c;
} void add(int r, int c)
{
ans++, S[c]++, C[ans] = c, X[ans] = r;
D[ans] = D[c];
U[ans] = c;
U[D[c]] = ans;
D[c] = ans;
if(head[r] < ) head[r] = L[ans] = R[ans] = ans;
else L[ans] = head[r], R[ans] = R[head[r]],L[R[head[r]]] = ans, R[head[r]] = ans;
} bool dfs(int sh)
{
if(!R[])
{
sort(vis, vis + * );
int cnt = ;
for(int i = ; i < ; i++)
{
for(int j = ; j < ; j++)
{
int num = vis[cnt++]; num=num - (i * + j) * ;
printf("%c", 'A' + num - );
// cout << 111 << endl; }
printf("\n");
}
printf("\n"); return true;
}
int c = R[];
for(int i = R[]; i; i = R[i]) if(S[c] > S[i]) c = i;
delc(c);
for(int i = D[c]; i != c; i = D[i])
{
vis[sh] = X[i];
for(int j = R[i]; j != i; j = R[j])
delc(C[j]);
if(dfs(sh + )) return true;
for(int j = L[i]; j != i; j = L[j])
resc(C[j]);
}
resc(c);
return false;
} char str[][]; void build(int x, int y, int k)
{
ans1 = (x * + y - ) * + k;
add(ans1, x * + k);
add(ans1, * + (y - ) * + k);
add(ans1, * * + x * + y);
int block = (y - ) / * + x / ;
add(ans1, * * + block * + k); } int main()
{ while(~scanf("%s", str[]))
{
m = * * ;
init(); for(int i = ; i < ; i++)
{
rs(str[i]);
}
for(int i = ; i < ; i++)
for(int j = ; j <= ; j++)
{
if(str[i][j - ] == '-')
for(int k = ; k <= ; k++) build(i, j, k);
else
build(i, j, str[i][j - ] - ('A' - ));
}
dfs();
} return ;
}
Sudoku POJ - 3076的更多相关文章
- Sudoku POJ - 3076 (dfs+剪枝)
Description A Sudoku grid is a 16x16 grid of cells grouped in sixteen 4x4 squares, where some cells ...
- (简单) POJ 3076 Sudoku , DLX+精确覆盖。
Description A Sudoku grid is a 16x16 grid of cells grouped in sixteen 4x4 squares, where some cells ...
- POJ 3076 Sudoku DLX精确覆盖
DLX精确覆盖模具称号..... Sudoku Time Limit: 10000MS Memory Limit: 65536K Total Submissions: 4416 Accepte ...
- POJ 3076 / ZOJ 3122 Sudoku(DLX)
Description A Sudoku grid is a 16x16 grid of cells grouped in sixteen 4x4 squares, where some cells ...
- 【POJ 3076】 Sudoku
[题目链接] http://poj.org/problem?id=3076 [算法] 将数独问题转化为精确覆盖问题,用Dancing Links求解 [代码] #include <algorit ...
- POJ 3076 Sudoku
3076 思路: dfs + 剪枝 首先,如果这个位置只能填一种字母,那就直接填 其次,如果对于每一种字母,如果某一列或者某一行或者某一块只能填它,那就填它 然后,对于某个位置如果不能填字母了,或者某 ...
- POJ 3076 Sudoku (dancing links)
题目大意: 16*16的数独. 思路分析: 多说无益. 想说的就是dancing links 的行是依照 第一行第一列填 1 第一行第二列填 2 -- 第一行第十五列填15 第一行第二列填 1 -- ...
- Sudoku POJ - 2676(DLX)
Sudoku Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 25356 Accepted: 11849 Specia ...
- POJ 3076 SUKODU [Dangcing Links DLX精准覆盖]
和3074仅仅有数目的不同,3074是9×9.本来想直接用3074的.然后MLE,,,就差那么20M的空间,,. 从这里学习到了解法: http://www.cnblogs.com/ylfdrib/a ...
随机推荐
- python之间的基础
编程第一步 print('hello,world!') 变量名的命名的规则: 1:变量由字母,数字,下划线组成 2:变量不能以数字开头 3:禁止使用python中的关键字,如 'alse', 'Non ...
- Java 自动装箱与拆箱(Autoboxing and unboxing)
什么是自动装箱拆箱 基本数据类型的自动装箱(autoboxing).拆箱(unboxing)是自J2SE 5.0开始提供的功能. 一般我们要创建一个类的对象实例的时候,我们会这样: Class a = ...
- 小L记单词
题目描述 小L最近在努力学习英语,但是对一些词组总是记不住,小L小把这些词组中每一个单词的首字母都记一下,这样形成词组的缩写,通过这种方式小L的学习效率明显提高. 输入 输入有多行,每组测试数据占一行 ...
- C. Ayoub and Lost Array
链接 [https://codeforces.com/contest/1105/problem/C] 题意 给你n,表示数组长度,元素的值是l到r,问有多少种方案使得所有元素和整除3 分析 思维dp, ...
- Form的is_valid校验规则及验证顺序
一.验证顺序 查看form下的源码了解顺序 BaseForm为基类,中间包含了is_valid校验方法 @html_safe class BaseForm: ......... self.is_b ...
- java.lang.LinkageError: JAXB 2.0 API is being loaded from the bootstrap classloader
我的解决办法: 1.如果是application工程,则在程序中打印出 system.out.println(System.getProperty("java.endo ...
- Windows10下安装VMware虚拟机并搭建CentOS系统环境
转载: http://blog.51cto.com/10085711/2069270 操作系统 Windows 10专业版(64位) VMware虚拟机 产品:VMware® Workstation ...
- [转帖]LCD与LED的区别之背光原理与优缺点对比介绍
LCD与LED的区别之背光原理与优缺点对比介绍 http://m.elecfans.com/article/620376.html 时下液晶面板与液晶电视技术已经达到炉火纯青的境界,并已经成为大屏幕平 ...
- springboot+ELK+logback日志分析系统demo
之前写的有点乱,这篇整理了一下搭建了一个简单的ELK日志系统 借鉴此博客完成:https://blog.csdn.net/qq_22211217/article/details/80764568 设置 ...
- org.elasticsearch.client.transport.NoNodeAvailableException
SpringBoot连接elasticsearch异常 2018-09-11 16:03:43.692 ERROR 8684 --- [ main] o.s.boot.SpringApplicatio ...